Testing AWS CDK Applications with Java: A Practical Guide
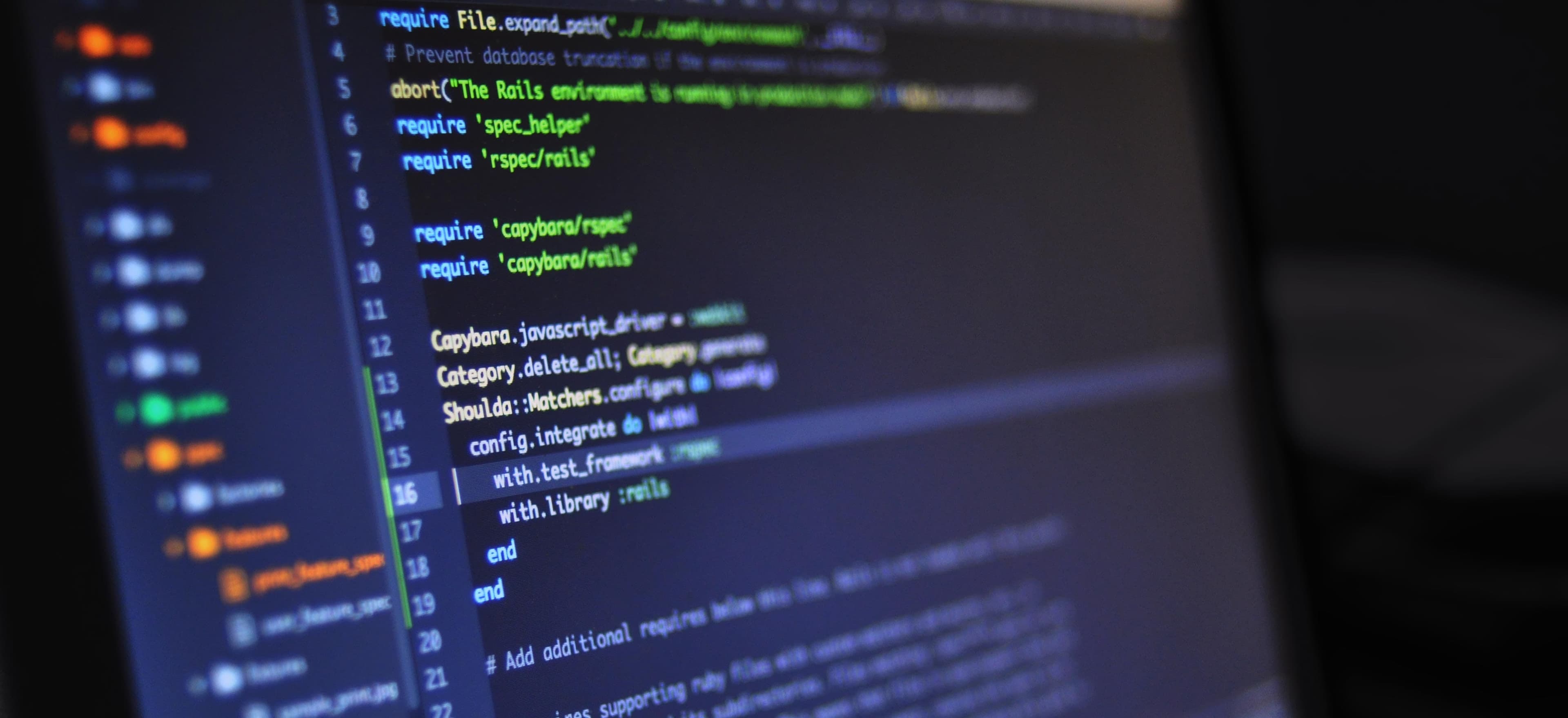
- Published on
Testing AWS CDK Applications with Java: A Practical Guide
When developing cloud applications using AWS Cloud Development Kit (CDK), testing is crucial to ensure that your infrastructure-as-code (IaC) operates flawlessly before deployment. In this post, we will delve into the intricacies of testing AWS CDK applications written in Java. We’ll cover the types of tests you can perform, best practices, and helpful examples to ensure your AWS resources are deployed correctly.
Stepping into the Topic
AWS CDK allows developers to model cloud infrastructure in a familiar programming language. With Java being one of the supported languages, developers can leverage its robust ecosystem and features. Most importantly, testing CDK applications helps to catch errors early in the development cycle and aids in maintaining a resilient infrastructure.
Why Should You Test Your CDK Applications?
- Validation of Infrastructure: Ensure that the infrastructure works as expected.
- Preventing Costly Errors: Catch mistakes before deploying to production, which helps avoid unexpected costs.
- Easier Maintenance: With proper tests, refactoring becomes safer and easier.
- Confidence in Changes: Tests provide assurance that new features or updates won't break existing functionalities.
For an in-depth examination of testing challenges in AWS CDK, refer to the article "Mastering AWS CDK: Overcoming Testing Challenges".
Types of Tests for AWS CDK Applications
When working with AWS CDK applications, you can implement various types of testing strategies:
1. Unit Testing
Unit tests verify the smallest parts of your application in isolation. In the context of CDK, this means testing individual constructs to ensure they produce the correct CloudFormation template.
Code Example: Unit Test
Here’s an example of how to write a unit test using JUnit for a CDK stack in Java.
import software.amazon.awscdk.core.Stack;
import software.amazon.awscdk.core.App;
import software.amazon.awscdk.assertions.Template;
import org.junit.jupiter.api.Test;
public class MyStackTest {
@Test
public void testLambdaFunctionCreated() {
App app = new App();
Stack stack = new MyStack(app, "MyTestStack");
Template template = Template.fromStack(stack);
template.hasResourceProperties("AWS::Lambda::Function", Map.of(
"Runtime", "java11"
));
}
}
Why This Code Works:
- We use JUnit to create a test class.
- The method
testLambdaFunctionCreated
initializes the application and creates a stack instance. Template.fromStack(stack)
captures the CloudFormation template generated by the stack.- Finally, we verify that the stack contains a Lambda function with the specified properties using
hasResourceProperties
.
2. Integration Testing
Integration tests evaluate the complete configuration of your application, including connections and interactions with other systems.
Code Example: Integration Test
We can use the AWS SDK along with our CDK constructs to verify that resources interact correctly with external services:
import com.amazonaws.services.lambda.invoke.LambdaInvokerFactory;
import org.junit.jupiter.api.Test;
public class IntegrationTest {
@Test
public void testApiGatewayIntegration() {
MyService service = LambdaInvokerFactory.builder()
.lambdaClient(AWSLambdaClientBuilder.defaultClient())
.build(MyService.class);
String response = service.invokeMyFunction("testInput");
assertEquals("expectedOutput", response);
}
}
Why This Code Works:
- Using
LambdaInvokerFactory
, you set up a proxy for the Lambda function. - The test condition verifies that the function returns the expected output when invoked with a known input.
3. End-to-End Testing
End-to-end tests encompass all components, ensuring that various services work together as intended. This is especially important in microservices architectures.
A common approach is to create a staging environment where features can be tested under conditions that mimic production.
Best Practices for Testing CDK Applications
- Keep Tests Isolated: Ensure that tests do not affect each other, which can lead to flaky behavior.
- Automate Testing: Use CI/CD pipelines to automate running your tests on each code change.
- Use Mocks and Stubs: For services that interact with AWS SDK, consider using mocks to simulate behavior without making actual calls.
- Document Tests Clearly: Clear documentation and comments help future maintainers understand the purpose and scope of each test.
- Version Control Your Tests: Keep your tests reviewed and updated as the application evolves.
Lessons Learned
Testing AWS CDK applications written in Java is vital for ensuring that your cloud infrastructure operates correctly and efficiently. By employing unit, integration, and end-to-end tests, you can have the confidence that your deployment will meet your application's requirements.
As you develop your AWS CDK applications, take the time to implement structured tests, adhere to best practices, and stay informed about evolving methodologies in testing.
For detailed insights into the complexities of testing AWS CDK applications, check out "Mastering AWS CDK: Overcoming Testing Challenges".
By taking a comprehensive and practical approach to testing, you'll be able to deploy AWS resources effectively while ensuring high-quality cloud applications. Embrace these testing strategies and make them part of your development workflow for successful CDK applications.
Checkout our other articles