Effortlessly Test Your Java Apps with AWS CDK Strategies
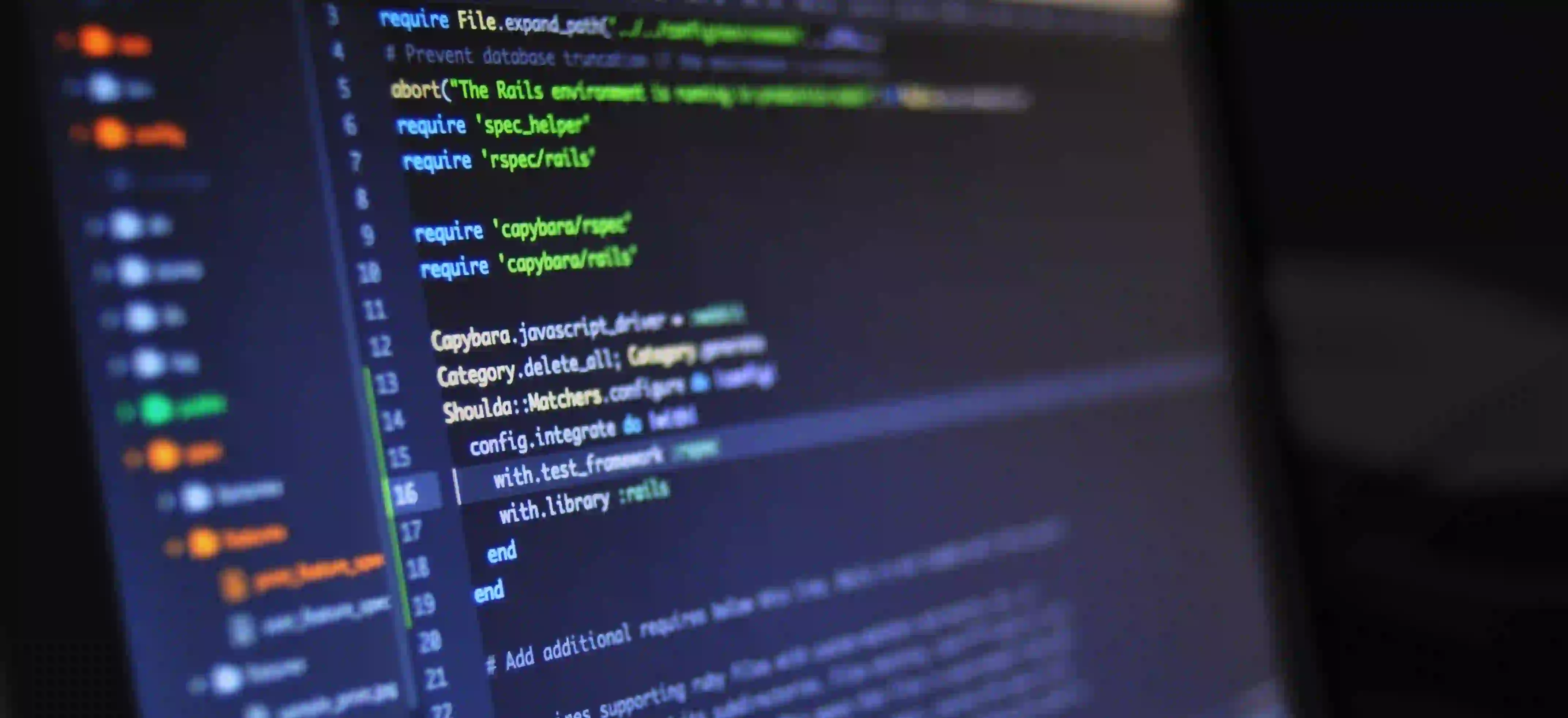
Effortlessly Test Your Java Apps with AWS CDK Strategies
Testing Java applications can be a daunting task, especially in cloud-native environments. Fortunately, with the AWS Cloud Development Kit (CDK), developers gain a powerful framework that simplifies infrastructure deployment and enhances testing capabilities. In this blog post, we will explore effective strategies for testing your Java applications using AWS CDK, helping you streamline your development workflow and improve software quality.
Why Use AWS CDK for Testing?
AWS CDK allows you to define cloud resources in a programming language you are already familiar with, such as Java. This simplifies the creation of infrastructure-as-code (IaC) and facilitates automated testing of your applications. By deploying your resources in a repeatable way, you can ensure that your tests run in a consistent environment, which is crucial for accurate testing results.
Moreover, a solid understanding of testing strategies within AWS CDK can help developers easily scale their applications while maintaining reliability.
Key Strategies for Testing Java Applications with AWS CDK
1. Unit Testing Your CDK Stacks
Unit testing is a critical part of any development process, and AWS CDK projects are no different. You can test your CDK stacks to verify that your infrastructure code behaves as expected.
Code Snippet: Unit Test for a CDK Stack
import static org.junit.jupiter.api.Assertions.assertEquals;
import software.amazon.awscdk.core.App;
import software.amazon.awscdk.core.Stack;
import software.amazon.awscdk.assertions.Template;
class MyStackTest {
@org.junit.jupiter.api.Test
void testStack() {
App app = new App();
Stack stack = new MyStack(app, "MyTestStack");
Template template = Template.fromStack(stack);
template.hasResourceProperties("AWS::S3::Bucket", properties -> {
assertEquals("myBucketName", properties.get("BucketName"));
return true;
});
}
}
Commentary: In this code snippet, we create a simple unit test using JUnit for a CDK stack that provisions an S3 bucket. The Template
class allows us to assert properties of resources defined in our stack, ensuring that our infrastructure is set up correctly.
2. Integration Testing with AWS Services
Integration tests allow you to validate that different components of your application work together as intended. AWS CDK makes it easier to deploy these services and run your integration tests.
Example: Testing an API Gateway with Lambda Function
Consider a scenario where you have a Lambda function triggered by an API Gateway:
import software.amazon.awscdk.core.*;
import software.amazon.awscdk.services.apigateway.*;
import software.amazon.awscdk.services.lambda.*;
public class ApiGatewayLambdaStack extends Stack {
public ApiGatewayLambdaStack(final Construct scope, final String id) {
super(scope, id);
Function myFunction = Function.Builder.create(this, "MyFunction")
.runtime(Runtime.JAVA_11)
.handler("com.example.MyHandler::handleRequest")
.code(Code.fromAsset("path/to/lambda/code"))
.build();
HttpApi httpApi = HttpApi.Builder.create(this, "Api")
.defaultIntegration(new LambdaProxyIntegration(LambdaProxyIntegrationProps.builder()
.handler(myFunction)
.build()))
.build();
}
}
Commentary: This stack creates a Lambda function and integrates it with an API Gateway. You can perform integration tests to ensure that calls to your API invoke the correct Lambda function and return the expected results.
3. End-to-End Testing
End-to-end testing is vital for verifying complete workflows in your application. You can deploy your entire stack using AWS CDK and run your acceptance tests against the live environment.
Running Tests Against a Deployed Stack
To conduct end-to-end tests, consider using testing frameworks like JUnit 5 or TestNG, along with an HTTP client like OkHttp.
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class EndToEndTest {
private static final String API_URL = "https://your-api-url.amazonaws.com/endpoint";
@Test
public void testApiResponse() throws Exception {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(API_URL)
.build();
try (Response response = client.newCall(request).execute()) {
assertEquals(200, response.code());
}
}
}
Commentary: This code uses OkHttp to send a request to the deployed API and checks that the response code is 200. Thus, verifying that the entire workflow behaves as expected.
4. Mocking AWS Services during Testing
Testing against actual AWS services can lead to high costs and increased test runtimes. AWS CDK offers ways to mock services to control costs and improve speed.
You can use tools like LocalStack to simulate AWS services locally. This allows you to run your tests against a local environment that mimics the behavior of the real AWS infrastructure.
Recommended Practices
-
Use CDK Constructs: Leverage CDK constructs that package functionality, making it reusable and easier to test.
-
Embrace CI/CD: Integrate your testing suite into your Continuous Integration and Continuous Deployment (CI/CD) pipeline. This ensures that tests will run automatically whenever you make changes.
-
Read the Right Resources: For deeper insights into the nuances of AWS CDK and testing, consider checking out articles like Mastering AWS CDK: Overcoming Testing Challenges.
-
Error Handling: Implement thorough error handling in your code to simplify debugging during tests. Making your tests expressive and clear will ultimately save you time.
-
Use Assertions Effectively: Use a wide variety of assertions to validate different aspects of your constructs. This ensures that all relevant cases are covered, improving the robustness of your tests.
Final Considerations
By leveraging AWS CDK for testing your Java applications, you can significantly improve your development workflow. The strategies outlined in this post provide a framework for effective testing, ensuring your applications behave as expected in various scenarios.
With the right tools and practices, testing can become a seamless part of your development process. Embrace these strategies, keep improving, and you'll find that testing can be not only manageable but also enjoyable.
Dive deeper into AWS CDK and make informed decisions to take your Java applications to new heights of stability and performance. Happy coding and testing!