Tackling Java Integration Testing with AWS CDK Tools
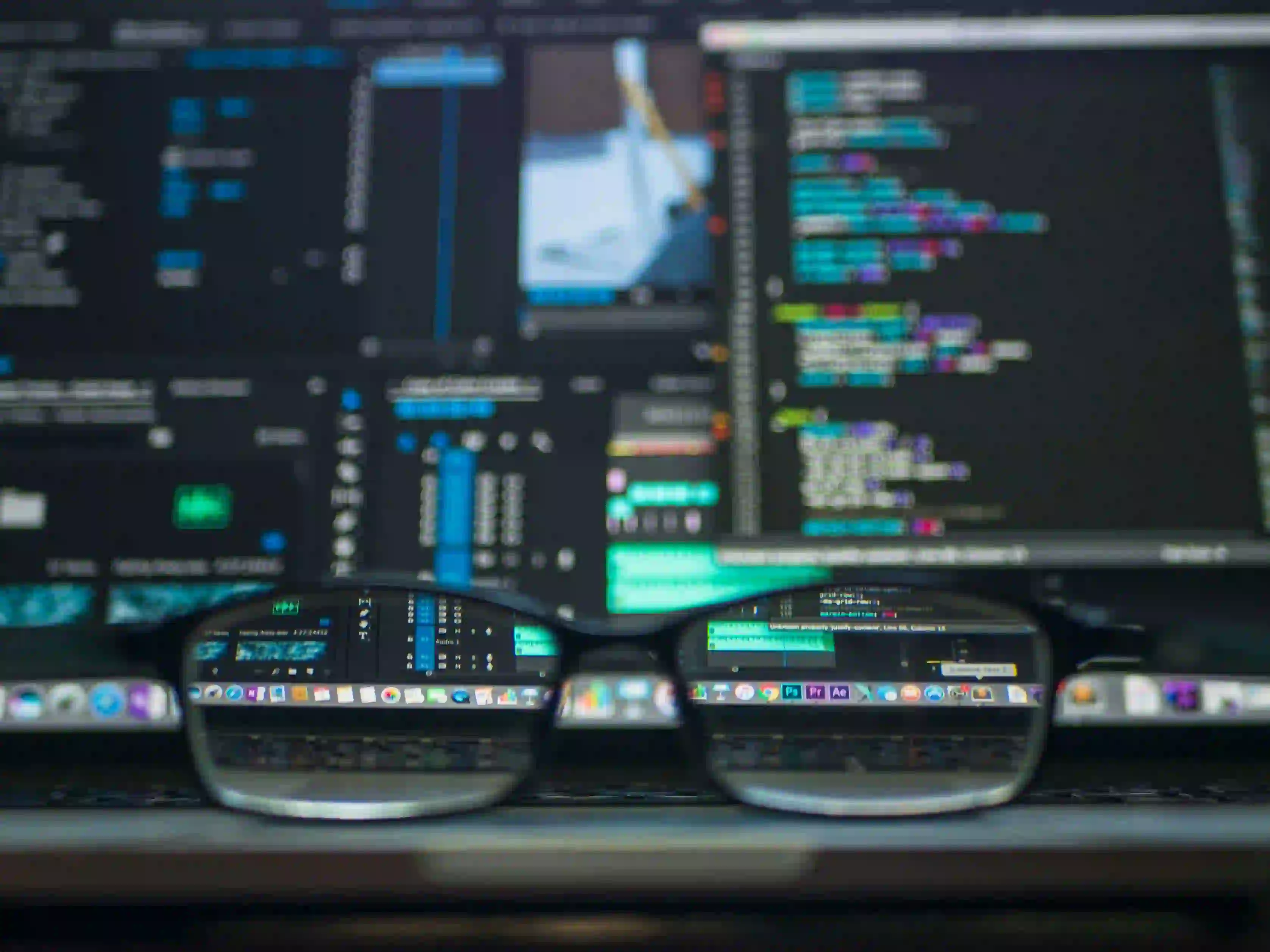
Tackling Java Integration Testing with AWS CDK Tools
Integration testing forms a critical part of the software development lifecycle, particularly in cloud applications. In Java projects, such testing ensures that various components work together as intended. When combined with AWS Cloud Development Kit (CDK), it provides powerful capabilities for setting up and managing cloud environments. In this blog post, we will explore how to effectively tackle integration testing in Java using AWS CDK tools.
Understanding Integration Testing
Before diving into the specifics of using AWS CDK with Java, let's briefly discuss what integration testing entails. Integration testing is the phase in software testing where different modules or services are tested as a combined entity. It helps identify issues in the interaction between integrated components.
The primary goals of integration testing include:
- Verifying Component Interactions: Ensuring that different services communicate correctly.
- Discovering Interface Errors: Catching issues where components interact (e.g., data format mismatches).
- Performance Testing: Assessing the overall performance of integrated services.
Why Use AWS CDK?
AWS CDK allows developers to define cloud infrastructure using familiar programming languages, including Java. By using CDK, you can programmatically configure AWS resources, which simplifies environment creation and makes integration testing more consistent and reliable.
Advantages of AWS CDK for Java Integration Testing:
- Infrastructure as Code: Define your infrastructure in Java, making it easy to version control.
- Rapid Provisioning: Quickly set up AWS resources needed for integration testing.
- Automatic Cleanup: Resources can be easily removed after testing, preventing unnecessary costs.
Prerequisites
Before we start crafting our integration tests, ensure you have the following prerequisites:
- Java Development Kit (JDK) installed (preferably version 11 or later).
- AWS CDK installed globally. You can use npm for installation:
🔧snippet.sh
npm install -g aws-cdk
- AWS CLI configured with the necessary permissions to deploy resources.
Setting Up a Basic CDK Project in Java
First, let's set up a basic AWS CDK project. If you haven't done it before, follow these steps:
Step 1: Create a New CDK Project
mkdir my-java-cdk-project
cd my-java-cdk-project
cdk init app --language java
This command sets up a Java CDK project with boilerplate code. You will see a directory structure with a few generated files.
Step 2: Add Dependencies
Modify the pom.xml
to include dependencies for AWS services you plan to use. Here’s an example for AWS Lambda and S3:
<dependencies>
<dependency>
<groupId>software.amazon.awscdk</groupId>
<artifactId>aws-lambda</artifactId>
<version>1.133.0</version>
</dependency>
<dependency>
<groupId>software.amazon.awscdk</groupId>
<artifactId>aws-s3</artifactId>
<version>1.133.0</version>
</dependency>
</dependencies>
Creating AWS Resources
Let’s create a sample AWS Lambda function that interacts with S3. This is essential for testing how our components will work together in the AWS environment.
Step 3: Define AWS Resources
Open the main CDK application file, usually in MyStack.java
, and add the following code:
import software.amazon.awscdk.core.*;
import software.amazon.awscdk.services.lambda.*;
import software.amazon.awscdk.services.s3.*;
public class MyStack extends Stack {
public MyStack(final Construct scope, final String id) {
super(scope, id);
// Create S3 bucket
Bucket bucket = Bucket.Builder.create(this, "MyTestBucket")
.versioned(true)
.build();
// Create Lambda function
Function lambdaFunction = Function.Builder.create(this, "MyLambdaFunction")
.runtime(Runtime.JAVA_11) // Set the Lambda runtime
.handler("com.example.MyHandler") // Define handler method
.code(Code.fromAsset("lambda")) // Point to your lambda code
.environment(Map.of("BUCKET_NAME", bucket.getBucketName())) // Pass Bucket name as environment variable
.build();
// Grant Lambda permission to read from the S3 bucket
bucket.grantRead(lambdaFunction);
}
}
Commentary on the Code
- Bootstrap the Stack: With the
MyStack
constructor, we initialize our stack. - S3 Bucket: Create a versioned S3 bucket that acts as our storage layer. Using versioning is a good practice when dealing with mutable data.
- Lambda Function: This defines an AWS Lambda function that can process events, linked to our S3 bucket.
- Environment Variables: Pass the S3 bucket name to the Lambda function using environment variables for easy access during execution.
- Permissions: Grant necessary permissions to the Lambda function so it can read from the S3 bucket.
Writing Integration Tests in Java
Now that we have our AWS resources defined, it's time to set up our integration tests.
Step 4: Setting Up Test Framework
You will need a testing framework. We’ll use JUnit along with AWS SDK for Java to handle our integration tests. Ensure you have JUnit configured in your pom.xml
:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
Step 5: Writing the Test Case
Create a new test class, e.g., LambdaIntegrationTest.java
, and define your test case:
import org.junit.jupiter.api.Test;
import software.amazon.awssdk.services.s3.S3Client;
import software.amazon.awssdk.services.s3.model.PutObjectRequest;
import software.amazon.awssdk.services.s3.model.PutObjectResponse;
import static org.junit.jupiter.api.Assertions.assertNotNull;
public class LambdaIntegrationTest {
private static final String BUCKET_NAME = System.getenv("BUCKET_NAME");
@Test
public void testLambdaFunction() {
S3Client s3 = S3Client.create();
// Create a sample file and upload it to S3
PutObjectRequest putRequest = PutObjectRequest.builder()
.bucket(BUCKET_NAME)
.key("test.txt")
.build();
PutObjectResponse putResponse = s3.putObject(putRequest, RequestBody.fromString("Hello, AWS CDK!"));
assertNotNull(putResponse);
// Add additional logic to trigger the lambda and check its response
}
}
Commentary on the Test Code
- S3 Client: Utilizes the AWS SDK for Java to create an S3 client and interact with the S3 bucket.
- Upload Test Object: We create a simple text file and upload it to S3 as part of the test.
- Assertions: Validate that the response from S3 is not null, indicating the object was successfully uploaded.
Step 6: Running Tests
Make sure you provision your resources before running tests:
cdk deploy
After the resources are up, run your tests:
mvn test
Clean Up Resources
Once the integration testing is done, remember to clean up the resources:
cdk destroy
Wrapping Up
Integration testing in Java using AWS CDK tools makes it easier to deploy and manage cloud resources programmatically. The combination allows you to validate end-to-end scenarios effectively.
For further insights into overcoming challenges in testing with AWS CDK, refer to Mastering AWS CDK: Overcoming Testing Challenges.
By following the steps laid out in this post, you can enhance your development workflow and improve the reliability of your applications. Be sure to continuously integrate your tests within your CI/CD pipeline to reap the full benefits!
Additional Resources
Experiment with these tools, adjust your infrastructure as needed, and happy coding!