Solving Java Database Connection Issues During Migration
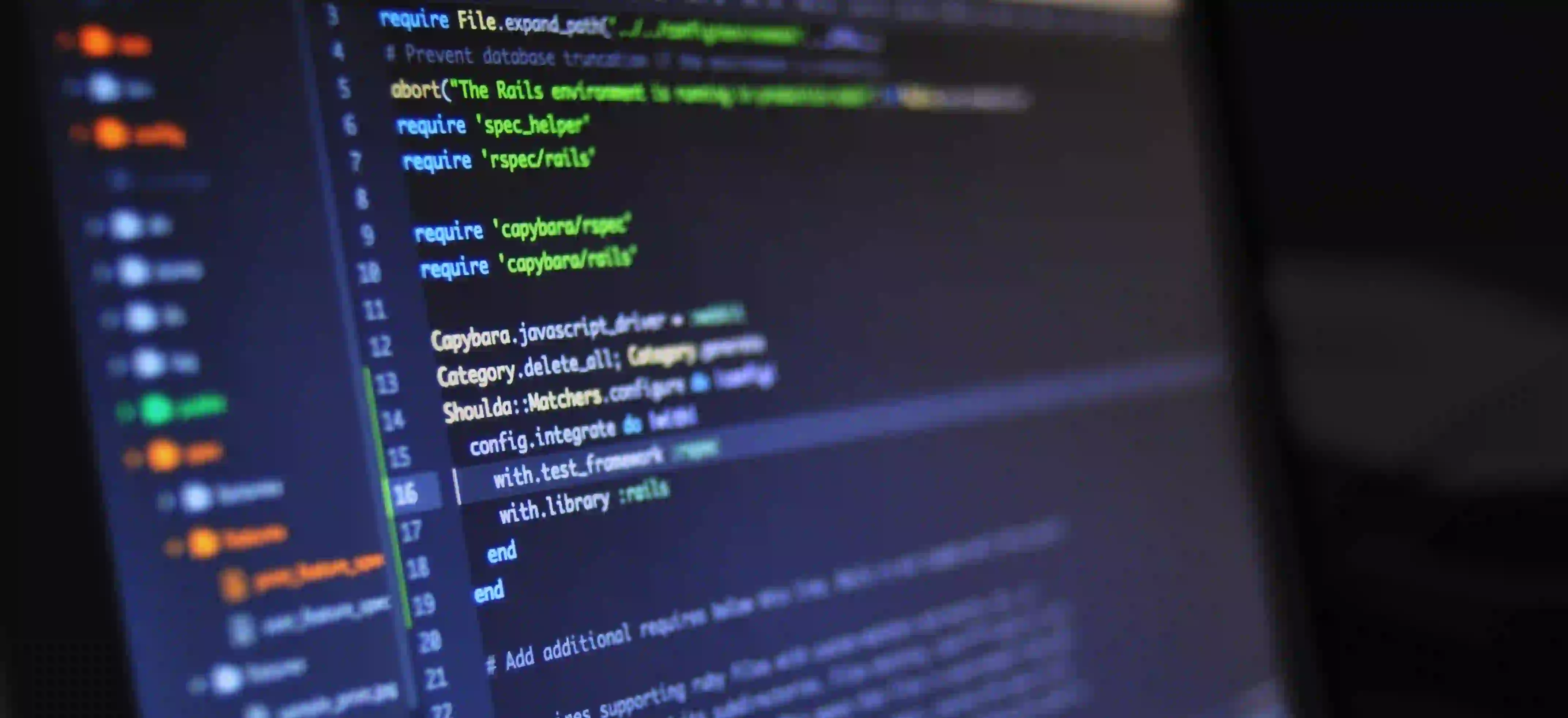
Solving Java Database Connection Issues During Migration
Migrating an application from a local environment to a live server is a common challenge faced by developers. In the world of Java, such migrations typically include securing an effective database connection. This guide explores common issues you may encounter during this process and provides effective solutions.
Why Is Database Connection Critical?
When you migrate a Java application, establishing a reliable database connection is paramount. It's the backbone of your application's functionality—enabling data retrieval, manipulation, and storage. A failure in this part of the application can lead to downtime, loss of data, or even corruption of the database.
Key Areas of Focus
- Database Configuration
- Connection Strings
- Driver Compatibility
- Environment Variables
- Error Handling
Let's dive into these areas.
Database Configuration
Before establishing a connection, ensure that your database allows connections from the live server. This might involve configuring the database server settings, enabling remote connections, and defining user access privileges.
Code Example: Checking Database Permissions
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseCheck {
public static void main(String[] args) {
String url = "jdbc:mysql://your-live-server:3306/yourDatabase";
String user = "yourUsername";
String password = "yourPassword";
try (Connection connection = DriverManager.getConnection(url, user, password)) {
System.out.println("Database connection successful!");
} catch (SQLException e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
}
In this code, we attempt to connect to the database. If there are permission issues or the server is misconfigured, the SQLException will catch the error with a relevant message.
Connection Strings
Connection strings are essential when connecting to a database. They must match the live server's configurations. Ensure that you use the correct hostname, port number, and database name.
Code Example: Verifying Connection String
public class ConnectionStringVerify {
private static final String DB_URL = "jdbc:mysql://your-live-server:3306/yourDatabase";
private static final String USER = "yourUsername";
private static final String PASS = "yourPassword";
public static void main(String[] args) {
if (DB_URL.contains("your-live-server")) {
System.out.println("Connection string is formatted correctly.");
} else {
System.err.println("Invalid connection string.");
}
}
}
In this example, we perform a quick check of the connection string to verify that it contains the correct server address.
Driver Compatibility
It's crucial to use the correct JDBC driver for the version of your database. Outdated or incompatible drivers can lead to connection failures. Ensure that the version you package with your application matches the database server version.
Code Example: Loading the JDBC Driver
try {
Class.forName("com.mysql.cj.jdbc.Driver");
System.out.println("Driver loaded.");
} catch (ClassNotFoundException e) {
System.err.println("Driver not found: " + e.getMessage());
}
Here, we load the MySQL driver required for database access. If the driver isn't compatible, ClassNotFoundException will inform you of the issue.
Environment Variables
Your development environment may contain hard-coded values for database connections that shouldn't be used in production. Utilizing environment variables helps you manage sensitive information securely and adapt to different environments easily.
Code Example: Using Environment Variables
public class EnvironmentVariableExample {
public static void main(String[] args) {
String dbUrl = System.getenv("DB_URL");
String dbUser = System.getenv("DB_USER");
String dbPassword = System.getenv("DB_PASSWORD");
if (dbUrl != null && dbUser != null) {
System.out.println("Environment variables loaded successfully.");
} else {
System.out.println("Failed to load environment variables.");
}
}
}
In this example, we capture database credentials using environment variables, reducing the hardcoding of sensitive data in your code.
Error Handling
During migration, it's essential to implement comprehensive error handling to capture and log any exceptions that occur during the database connection process.
Code Example: Robust Error Handling
public class RobustErrorHandling {
public static void main(String[] args) {
String url = "jdbc:mysql://your-live-server:3306/yourDatabase";
String user = "yourUsername";
String password = "yourPassword";
try (Connection connection = DriverManager.getConnection(url, user, password)) {
// Some operations...
} catch (SQLException e) {
logError(e);
}
}
private static void logError(SQLException e) {
System.err.println("Error Code: " + e.getErrorCode());
System.err.println("SQL State: " + e.getSQLState());
System.err.println("Message: " + e.getMessage());
}
}
Here, instead of simply printing the error message, we log detailed information—such as error code and SQL state—which can help during debugging.
Additional Resources
In addition to the aforementioned strategies, referring to resources on related topics can be beneficial. For instance, if you're dealing with a WordPress migration process, you might find this article helpful: Migrating Local WordPress Site to Live Server: Database Connection Concern.
Wrapping Up
Migrating a Java application and setting up a proper database connection requires careful attention to configuration, code quality, and error handling. By ensuring your connection string is correct, validating permissions, using the right driver, managing environment variables properly, and implementing error handling, you can pave the way for a successful deployment.
For further reading, consider examining the Java documentation on JDBC, which provides an in-depth look at Java database connectivity, best practices, and troubleshooting tips.
Prepare well, test extensively, and your migration will not only be smooth but also enhance your application's performance and reliability. Happy coding!