Debugging JavaScript Load Failures in Java Web Apps
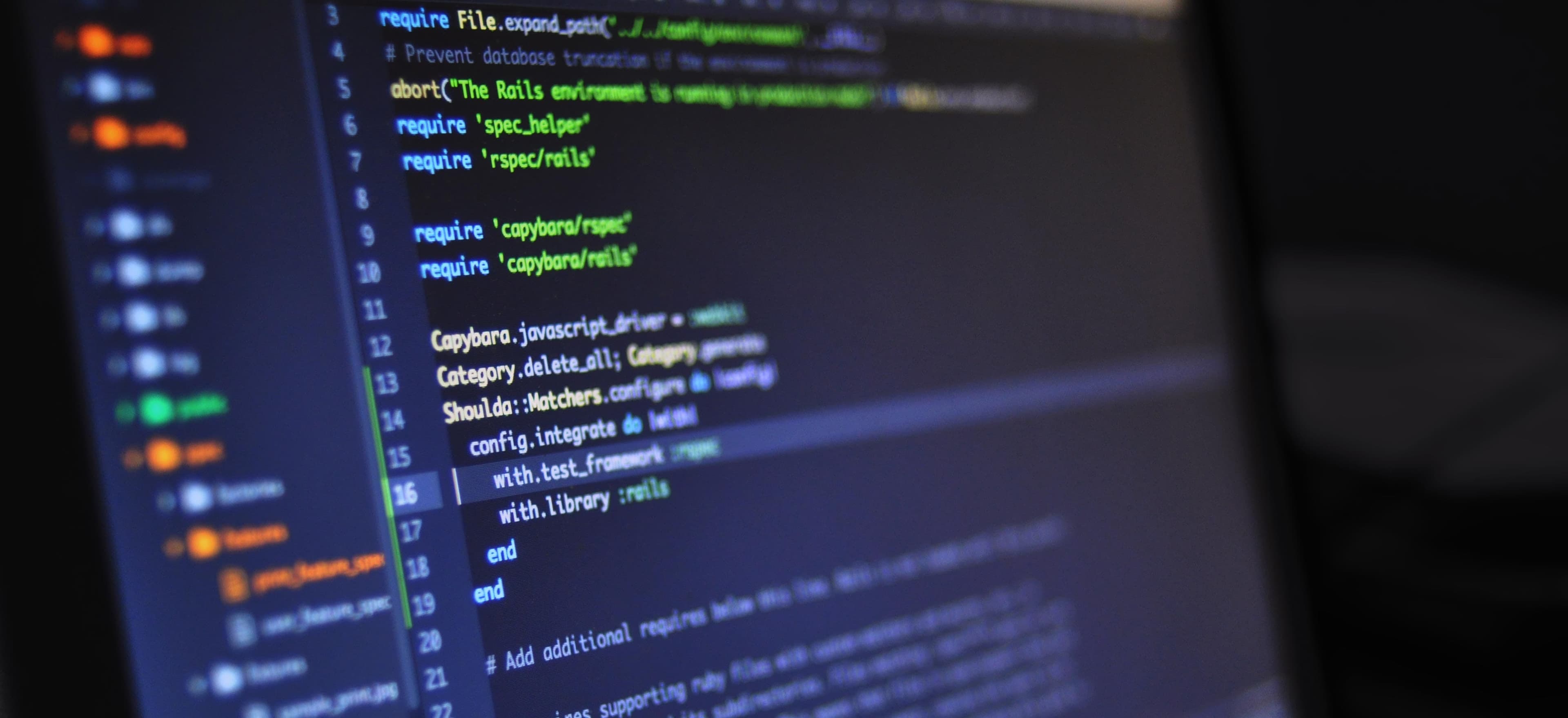
- Published on
Debugging JavaScript Load Failures in Java Web Apps
Java web applications enable dynamic web content and are often integrated with JavaScript for improved user interactivity. However, JavaScript load failures can pose significant issues, leading to a disrupted user experience and myriad bugs. In this post, we cruise through effective ways to tackle JavaScript load failures in your Java web apps, providing best practices and solutions.
Understanding JavaScript Load Failures
Load failures occur when JavaScript files or libraries embedded in your web application fail to execute properly. Several reasons can cause these failures:
- Network Issues: Temporary network outages can prevent JavaScript files from loading.
- Incorrect Paths: If the path to the JavaScript file is incorrect, browsers will fail to load it.
- Server Errors: 500 Internal Server Errors or 404 Not Found can halt the loading of scripts.
- Cross-Origin Resource Sharing (CORS): If your JavaScript or resources are hosted on a different domain, improper CORS settings can trigger load failures.
Understanding these common culprits allows developers to create robust web applications that gracefully handle errors and unexpected behavior.
Diagnosing Load Failures
Using Browser Developer Tools
Before diving into solutions, it's vital to diagnose the issue. One powerful tool at your disposal is the browser's developer tools, accessible via F12 or by right-clicking and selecting "Inspect".
-
Network Tab: Under the Network tab, you can see all resources loaded by the webpage and their status. Look for red messages indicating errors (404 or 500 statuses) next to your JavaScript files.
-
Console Tab: The Console tab displays JavaScript errors that may indicate why a particular script is not functioning as intended. Note any error messages, as they can provide insight into misbehaving code.
Example Code Snippet: Error Logging
window.onerror = function (msg, url, lineNo, columnNo, error) {
console.error("Error: " + msg + "\nURL: " + url + "\nLine: " + lineNo + "\nColumn: " + columnNo);
};
Why This Matters:
This code snippet sets a global error handler for your web application. When an error occurs, it prints the message, URL, and line number to the console. This allows for efficient debugging without needing to rely solely on browser tools.
Handling JavaScript Load Failures
Graceful Degradation
Graceful degradation is an essential principle in web design. It refers to the ability of a web application to function with reduced capability instead of failing entirely when JavaScript fails to load. Here’s how to implement it:
-
Inline Critical Functions: For essential functionality, you could consider inline scripts that embed critical JavaScript right in your HTML. This ensures that users can still access essential features without relying on external scripts.
-
CSS Fallbacks: If you include styles driven by JavaScript (like animations), consider providing static CSS alternatives.
Example: Basic Fallback Script
<script>
if (!window.MyLibrary) {
console.warn("MyLibrary failed to load. Using fallback.");
// Define a simple version or logic here
window.MyLibrary = {
greet: function() {
console.log("Hello, User!");
}
};
}
</script>
Why This Matters:
In the above code, we are checking if a third-party library has loaded. If not, we log a message in the console and define a simpler alternative. This enables functionality to continue without breaking the user experience.
Error Handling Strategies
- Try-Catch Blocks: Use try-catch blocks around critical sections of your JavaScript to gracefully handle errors during execution.
try {
riskyFunction(); // A function that could throw an error
} catch (error) {
console.error("Caught an error: ", error.message);
// Handle the error accordingly
}
Why This Matters:
Using try-catch ensures that an error in one part won’t crash the entire application. Instead, you can handle the error and keep the app running, albeit with limited features.
Validating Resource URLs
Check if the paths to your JavaScript resources are valid. Often, developers ignore file extensions or make typo errors in the URL.
Example: Java Servlet for Resource Serving
In a typical Java web application, you might be using a servlet to serve your resources.
@WebServlet("/scripts/*")
public class ScriptServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String resourcePath = request.getPathInfo();
// Serve the resource or throw 404 if not found
String fullPath = getServletContext().getRealPath("/resources" + resourcePath);
File resourceFile = new File(fullPath);
if (resourceFile.exists()) {
response.setContentType("application/javascript");
Files.copy(resourceFile.toPath(), response.getOutputStream());
} else {
response.sendError(HttpServletResponse.SC_NOT_FOUND);
}
}
}
Why This Matters:
In this example, we implement a servlet that serves JavaScript files dynamically. It checks if the resource exists and serves it, thus managing resource paths on the server side.
CORS Issues
CORS can easily trip up JavaScript functionality in your application, especially if resources are hosted cross-domain. Make sure your server settings are configured correctly to allow cross-origin requests.
Example: Setting CORS in Java
response.setHeader("Access-Control-Allow-Origin", "*");
Why This Matters:
By allowing all origins, you can prevent load failures due to CORS issues, at least temporarily. For a production scenario, specify allowed origins to enhance security.
In Conclusion, Here is What Matters
Debugging JavaScript load failures requires patience and a systematic approach. Utilizing browser tools, implementing error handling strategies, and serving resources properly are instrumental in ensuring that your Java web applications function smoothly.
For more on troubleshooting load failures, consider reading our referenced article Troubleshooting Iframe JavaScript Load Failures.
By investing time into understanding and preparing for these failures, you can elevate your web applications' resilience and user experience substantially. Keep these strategies in mind as you build robust Java web applications. Happy coding!
Checkout our other articles