Resolving Java Database Connection Issues in Migration Tasks
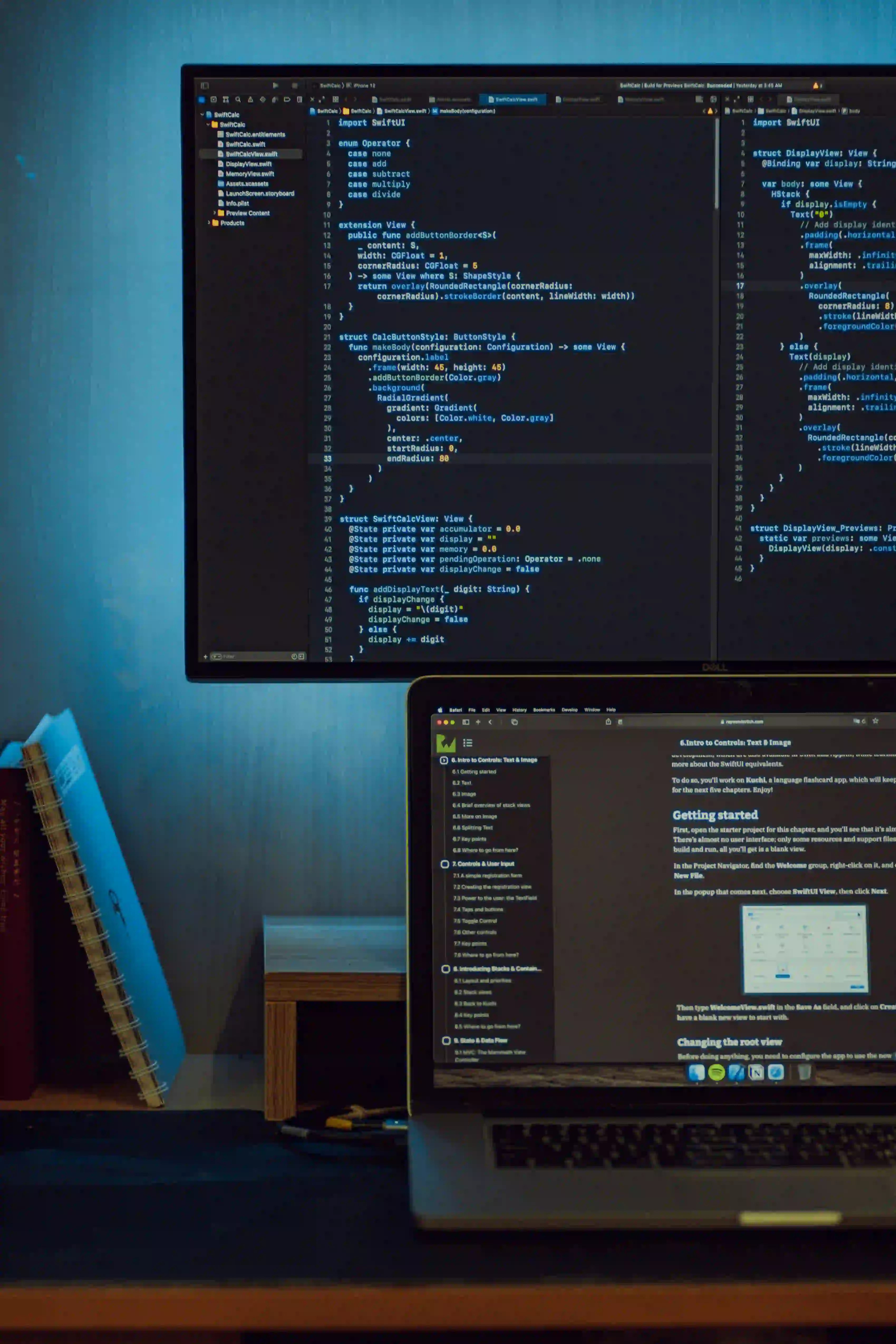
Resolving Java Database Connection Issues in Migration Tasks
Transferring a project, specifically involving databases, can be a daunting task for developers. Moving from a local environment to a live server introduces several challenges, especially regarding database connectivity. In this blog post, we will delve into common issues faced while establishing database connections in Java during migration processes, drawing on insights that can also be applied when migrating WordPress sites—similar to what is discussed in the article titled Migrating Local WordPress Site to Live Server: Database Connection Concern.
The Importance of Database Connectivity
Establishing a successful database connection is crucial. It allows your applications to interact with data effectively. In migration scenarios, any hiccup in connectivity can halt your project in its tracks. Java, being one of the most popular programming languages, provides various tools to manage these connections. However, developers often encounter a slew of issues that can make the process more complex.
Common Database Connection Issues
Before diving into solutions, let’s examine some frequent issues developers face when dealing with Java-based applications connecting to a database during migration:
- Incorrect Database URL
- Driver Class Not Found
- Authentication Failures
- Network Issues
- Database Permissions
1. Incorrect Database URL
When migrating, one of the most common errors is an incorrect database URL. The database URL is crucial as it defines where your application should connect.
Example Code
String dbUrl = "jdbc:mysql://localhost:3306/mydatabase";
Connection connection = DriverManager.getConnection(dbUrl, "username", "password");
In this code snippet, ensure you replace localhost
, 3306
, mydatabase
, username
, and password
with the actual details from your live server setup.
Why It Matters: The database URL points to your database's location. If it's incorrect, your application won't find the database, resulting in a failed connection attempt.
2. Driver Class Not Found
Java relies on JDBC (Java Database Connectivity) drivers to facilitate connectivity. If your application cannot find the appropriate driver, you'll be greeted with an error.
Example Code
try {
Class.forName("com.mysql.cj.jdbc.Driver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
This code attempts to load the MySQL driver class. Ensure you have the correct library included in your project’s dependencies, such as MySQL Connector/J.
Why It Matters: If the necessary driver is not available, your application won’t be able to communicate with the database, leading to connection failures.
3. Authentication Failures
Even when the URL and driver are correct, authentication failures can occur if the credentials provided are invalid.
Example Code
try {
Connection connection = DriverManager.getConnection(dbUrl, "username", "password");
} catch (SQLException e) {
System.out.println("Authentication failed: " + e.getMessage());
}
In this snippet, if the provided username and password are incorrect, you will see an authentication message.
Why It Matters: Your database's security is vital. Using incorrect credentials is a common pitfall that can block access entirely.
4. Network Issues
Network-related problems can arise, especially in cloud-based setups. Firewalls might block connections, or the server might not be reachable.
How to Diagnose
- Ping the Database Server: You can use the
ping
command to check connectivity. - Check Firewall Settings: Ensure that the required ports are open.
Why It Matters: You must confirm that your application can communicate with the database server over the network. If not, you won’t be able to establish a connection.
5. Database Permissions
Even with the right connection parameters, insufficient privileges can lead to failures. The database user must have appropriate permissions to access the database and perform required operations.
Example SQL Grant Statement
GRANT ALL PRIVILEGES ON mydatabase.* TO 'username'@'hostname' IDENTIFIED BY 'password';
FLUSH PRIVILEGES;
This SQL command grants all privileges to the specified user. Adjust the username and hostname according to your configuration.
Why It Matters: Ensuring proper permissions is similar to setting the right access levels during a website migration, as discussed in the WordPress migration article. Insufficient privileges can prevent your application from functioning correctly.
Debugging Connection Issues
If you encounter connection issues, here are some debugging steps to take:
- Log Connection Attempt Details: Capture and log every aspect of your connection attempt. This will help identify where the breakdown occurs.
- Use Try-Catch Blocks: This will help catch specific database exceptions and provide clarity on the issue.
- Test Connections Independently: Use a simple Java program to test database connectivity without the complexity of your main application.
Example Debug Code
public static void main(String[] args) {
String dbUrl = "jdbc:mysql://localhost:3306/mydatabase";
String username = "user";
String password = "pass";
try {
Connection connection = DriverManager.getConnection(dbUrl, username, password);
System.out.println("Connection Successful.");
} catch (SQLException e) {
System.out.println("Connection Failed: " + e.getMessage());
}
}
Why It Matters: Simplifying the code allows you to isolate connection issues and troubleshoot effectively.
Best Practices for Database Connectivity in Java
- Use Connection Pooling: This improves performance and handles multiple requests without exhausting resources.
- Implement Proper Error Handling: This aids in understanding connection failures and taking corrective actions.
- Close Your Connections: Always ensure that connections are closed after use to prevent leaks.
Connection Pooling Example
Using a library like HikariCP, you can easily implement connection pooling:
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("username");
config.setPassword("password");
HikariDataSource dataSource = new HikariDataSource(config);
// Use the dataSource to get connections
try (Connection connection = dataSource.getConnection()) {
// Use connection
}
Why It Matters: Connection pooling can lead to significant performance improvements, especially under load.
Key Takeaways
Navigating Java database connections during migration transitions is often fraught with challenges ranging from configuration errors to network issues. Understanding these common pitfalls and adhering to best practices can enable developers to establish robust and efficient database connections.
For deeper insights into connectivity challenges faced during website migrations, you might want to refer to the article Migrating Local WordPress Site to Live Server: Database Connection Concern, which outlines similar issues in a different context.
By staying mindful of these challenges and strategies, your migration task will be smoother, allowing you to focus on delivering value rather than troubleshooting connectivity issues.