Resolving JavaScript Load Issues in Java Web Applications
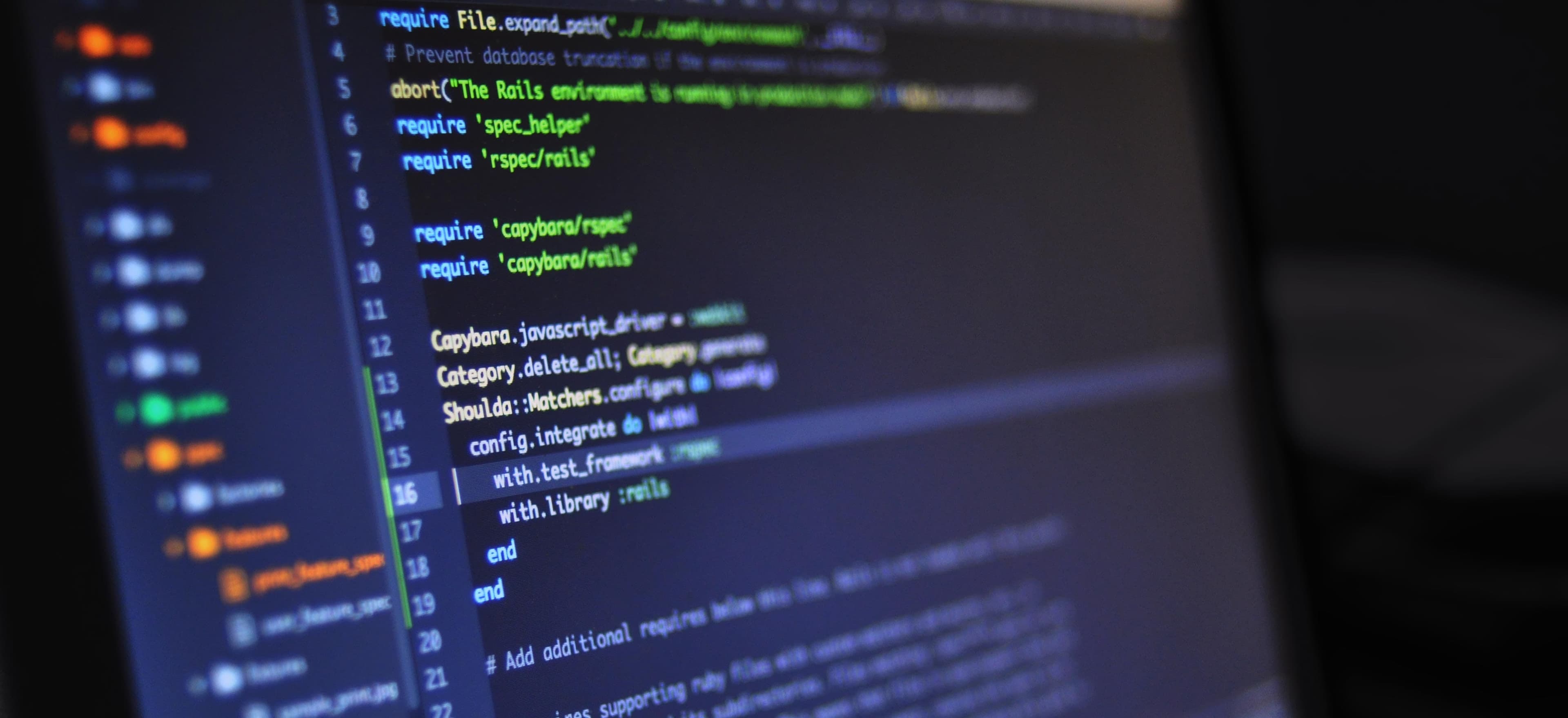
- Published on
Resolving JavaScript Load Issues in Java Web Applications
In the world of web development, Java and JavaScript often work hand in hand. Java backend frameworks like Spring Boot, JavaServer Faces (JSF), or servlet-based applications frequently integrate JavaScript to provide a seamless user experience. However, developers may encounter JavaScript load failures, which can impact application performance and usability.
In this post, we will explore common sources of JavaScript load issues in Java web applications and provide actionable solutions. This information will not only enhance your debugging skills but also ensure a smoother development process.
Understanding JavaScript Load Issues
JavaScript load issues happen when the browser fails to execute JavaScript code as intended. This can lead to features not working properly, or worse, the application crashing. Common symptoms include:
- Error messages in the browser console.
- Features that don't respond to user interactions.
- Application performance issues.
These issues can stem from a variety of sources, including incorrect file paths, incorrect MIME types, or issues with external libraries.
You may find Troubleshooting Iframe JavaScript Load Failures particularly helpful for understanding how iframes can influence JavaScript load behavior in your web apps.
Common Causes and Solutions
1. Incorrect File Paths
One of the most common reasons JavaScript fails to load is incorrect file paths. When linking to external JavaScript files, ensure the paths are correct.
Example:
<script src="assets/js/script.js"></script>
Why this matters: If the file path is incorrect, the browser will not be able to find the JavaScript file, leading to load failures.
Solution: Double-check the file paths. Use browser developer tools (like the network tab in Chrome) to see if the resources are being loaded correctly.
2. Script Loading Order
JavaScript execution is affected by the order of script inclusion. If a script that depends on another is loaded before that script, it will cause errors.
Example:
<script src="assets/js/dependency.js"></script>
<script src="assets/js/main.js"></script>
Why this matters: If main.js
depends on dependency.js
, trying to access a variable or function from dependency.js
in main.js
will result in a ReferenceError
.
Solution: Ensure that scripts are loaded in the correct order, or use the defer
attribute to indicate a script should only be executed once the document is fully parsed.
<script src="assets/js/dependency.js" defer></script>
<script src="assets/js/main.js" defer></script>
3. MIME Type Issues
Browsers enforce strict rules regarding the MIME types of resources. If your server is incorrectly configured and serves JavaScript files with the wrong MIME type, the browser will refuse to execute them.
Solution: Ensure your server is set to serve JavaScript files with the correct MIME type application/javascript
. Here’s an Apache configuration example:
AddType application/javascript .js
4. CORS Policies
Cross-Origin Resource Sharing (CORS) policies can also cause JavaScript load issues when fetching resources from different origins.
Why this matters: If your Java web application attempts to load a JavaScript file from a different domain without appropriate CORS headers, it will be blocked.
Solution: Ensure the server hosting the JavaScript file includes the appropriate headers. Here’s a simple example using Spring Boot:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("http://yourdomain.com");
}
}
5. Minified Files
Using minified or concatenated files can also lead to load issues, especially if there are syntax errors or if references are broken during the minification process.
Solution: Always test your application in a development environment before deploying minified files. Here’s how to include both minified and non-minified files:
<!-- Non-minified for development -->
<script src="assets/js/main.js"></script>
<!-- Minified for production -->
<script src="assets/js/main.min.js"></script>
6. JavaScript Errors
Miswritten JavaScript code is often the root cause of load issues. These can manifest as syntax errors, function reference errors, etc.
Example:
function myFunction() {
console.log("This is a function without an ending bracket";
}
Why this matters: A simple missing bracket can cause the entire script to fail, rendering features inoperable.
Solution: Always check the console for any JavaScript errors, and utilize linting tools to catch issues before they make it to production.
7. Dependencies on External Libraries
When utilizing external libraries (like jQuery or Bootstrap), ensure that they are loaded correctly before your own scripts.
Solution: Include the library in your HTML before including your JavaScript files:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="assets/js/myScript.js"></script>
8. Browser Compatibility
JavaScript is generally consistent, but there can be discrepancies between browser implementations.
Why this matters: Some features may not work in older browsers or certain versions.
Solution: Use feature detection (via libraries like Modernizr) to check if a feature is supported before using it:
if (Modernizr.fetch) {
// Safe to use the Fetch API
} else {
// Fallback
}
Debugging Techniques
In addition to the solutions provided, here are some debugging techniques that can help you quickly diagnose JavaScript load issues:
-
Use Console Logs: Add console logs liberally throughout your JavaScript to trace execution.
-
Browser Dev Tools: Utilize the network and console tabs to spot loading issues and JavaScript errors.
-
Simplify Your Code: If you encounter an issue, try isolating code blocks or reducing the complexity until the error disappears.
-
Network Analysis: Check for failed requests in the network tab of developer tools and review the status codes.
-
Versioning: Keep in mind the versions of libraries and frameworks you are using. Sometimes, breaking changes occur in new versions.
-
Report Issues: If you discover an issue with a library, don’t hesitate to report it. The open-source community thrives on collaborative problem-solving.
A Final Look
JavaScript load issues can be debilitating for Java web applications, but with the right knowledge and troubleshooting techniques, you can resolve these issues efficiently. From checking file paths to understanding CORS policies, each step is vital for ensuring your application runs smoothly.
Whether you are debugging a simple feature or a complex multi-faceted application, the right approach can save you hours of frustration. Don't forget to refer to resources like Troubleshooting Iframe JavaScript Load Failures for deeper insights into specific scenarios.
In your journey as a developer, encountering and resolving these issues will only enhance your skill set. Stay diligent, test often, and happy coding!