How Automation Testing Reduces Release Delays
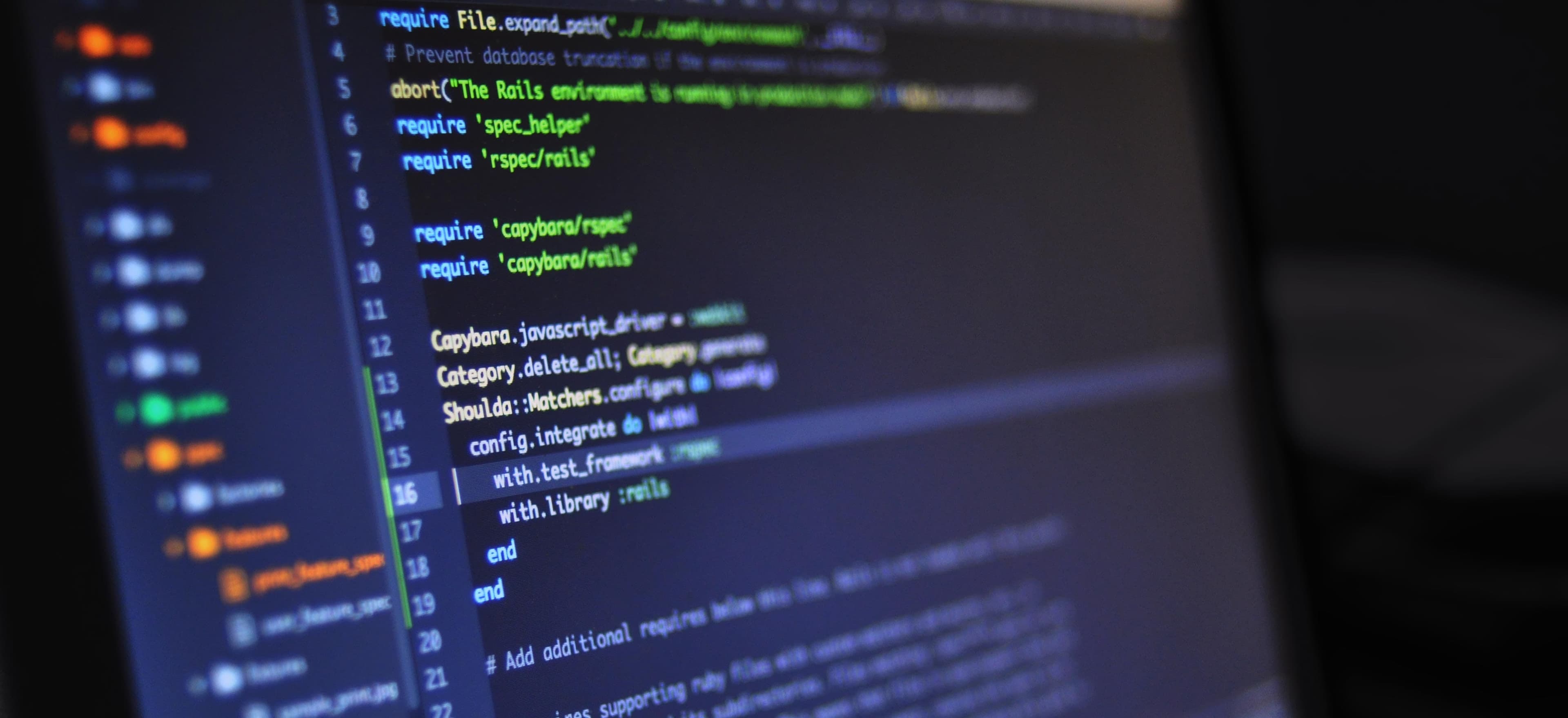
- Published on
How Automation Testing Reduces Release Delays
In the fast-paced world of software development, companies are continually challenged to deliver high-quality products more quickly. One effective way to meet this challenge is through automation testing. In this blog post, we'll explore how automation testing can significantly reduce release delays, its benefits, and some implementation strategies.
Understanding Automation Testing
Automation testing refers to the process of using software tools to execute pre-scripted tests on a software application before it is released into the production environment. While manual testing plays an essential role, automation allows for a broader range of testing scenarios, repeated executions, and faster feedback.
Why Automation Testing?
- Speed and Efficiency: Automated tests can run much faster than manual tests, which is critical in a CI/CD (Continuous Integration/Continuous Deployment) pipeline.
- Accuracy: Automated tests eliminate the risk of human error, ensuring more consistent results across testing cycles.
- Reusability: Once created, automated test scripts can be reused for different versions of the software, reducing the overall effort for regression testing.
The Link Between Automation Testing and Release Delays
1. Early Detection of Bugs
With automation testing, bugs can be identified early in the development lifecycle. This early detection prevents bugs from cascading into larger problems later in the project, reducing the time spent on rework.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
int result = calc.add(5, 3);
assertEquals(8, result);
}
}
Commentary: The above JUnit test checks the addition method of the Calculator class. This immediate feedback allows developers to address problems as they arise, rather than after the software is already in a more mature stage.
2. Parallel Execution of Tests
Automation testing facilitates parallel execution, where multiple test scripts can run simultaneously across different environments. This capability drastically cuts down the time needed for testing.
import org.testng.annotations.Test;
public class ParallelTests {
@Test(threadPoolSize = 3, invocationCount = 10)
public void testMethod() {
// This test method will run in parallel
System.out.println("Thread: " + Thread.currentThread().getId());
// Simulate test logic
}
}
Commentary: The above code uses TestNG to execute the testMethod()
in parallel. By harnessing the power of multi-threading, testing time can be significantly minimized.
3. Frequent Regression Testing
Every code change carries the risk of introducing new bugs. Automated tests enable continuous regression testing, ensuring new features do not disrupt existing functionality.
public class StringUtility {
public boolean isPalindrome(String input) {
String reverse = new StringBuilder(input).reverse().toString();
return input.equals(reverse);
}
}
public class StringUtilityTest {
@Test
public void testPalindrome() {
StringUtility util = new StringUtility();
assertTrue(util.isPalindrome("radar"));
assertFalse(util.isPalindrome("hello"));
}
}
Commentary: This example highlights testing the isPalindrome
method of the StringUtility
class. Automated regression tests ensure that modifications to code do not unintentionally affect core functionalities.
Best Practices for Implementing Automation Testing
To achieve the best results from automation testing and effectively reduce release delays, consider the following strategies:
1. Choose the Right Tools
Selecting the right automation tools can make or break your testing strategy. Popular options include Selenium for web applications and TestNG or JUnit for Java applications.
2. Start Small and Scale
Begin by automating critical test cases (like smoke tests) and gradually expand your test suite. Scaling in this manner minimizes initial overhead and allows for more manageable implementation.
3. Continuous Integration
Integrate automation testing into your CI/CD pipeline. CD tools like Jenkins or GitLab CI can trigger automated tests after every code commit, providing real-time feedback to developers.
4. Maintain Your Test Suite
Regularly review and update your test scripts to ensure they remain relevant and effective. Outdated tests can lead to false negatives, consuming valuable time.
The Impact of Automation Testing on Team Morale
Beyond reducing release delays, automation testing can positively influence team morale. Developers can concentrate more on creating new features rather than getting bogged down in tedious manual testing. This sense of innovation can lead to a more motivated and satisfied development team.
In Conclusion, Here is What Matters
In conclusion, automation testing is an invaluable approach that significantly reduces release delays in software development. By leveraging automated tests for speed, accuracy, and feedback, organizations can deliver high-quality products more efficiently.
If you want to delve deeper into automation testing, consider exploring more about Selenium or investing in TestNG. Adopting these tools and practices transitions teams toward a more streamlined, efficient development process.
Embrace automation to not only stay competitive in the market but also to foster a proactive development culture that thrives on constant improvement. The future of software development is automated, and those who adapt will undoubtedly lead the way.
Checkout our other articles