Mastering Enemy Pathfinding in JavaFX Tower Defense
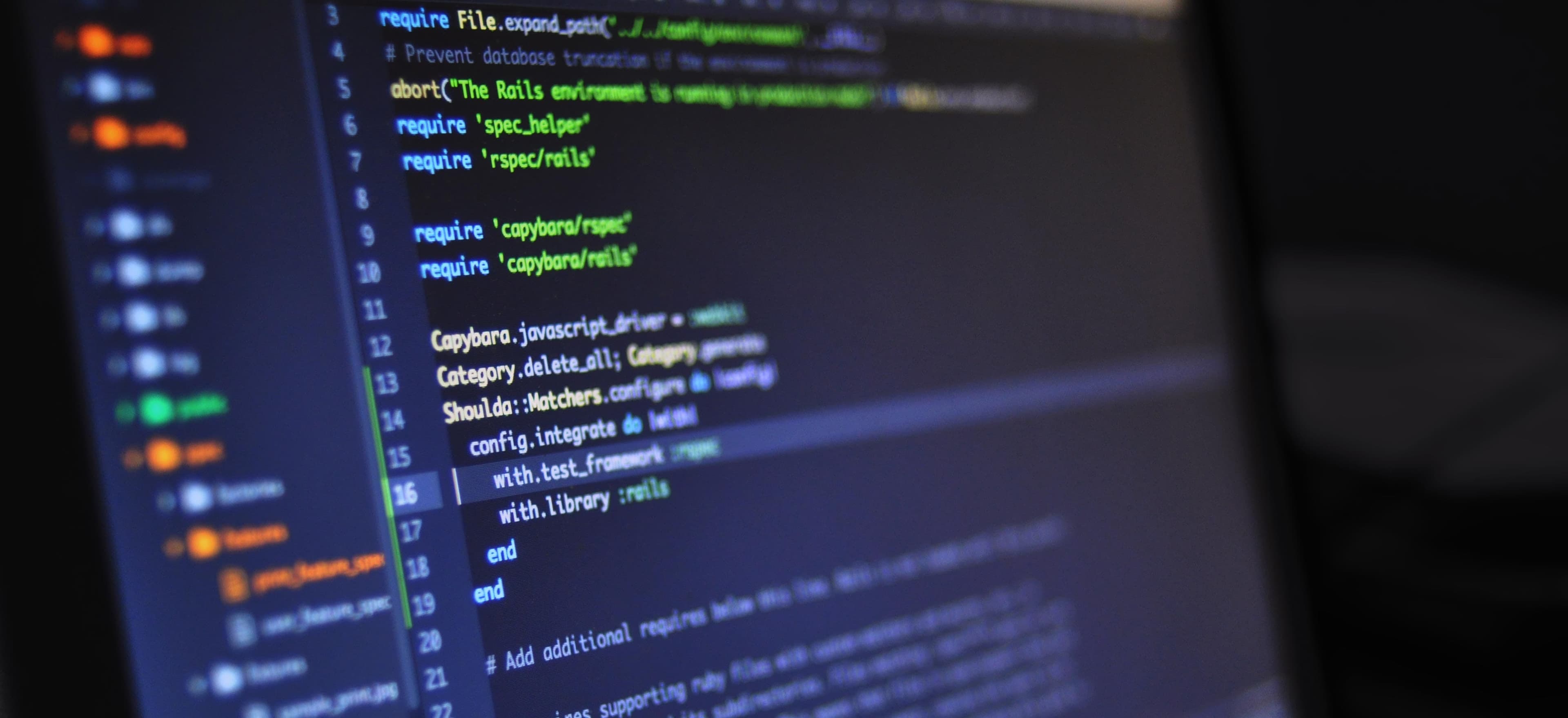
- Published on
Mastering Enemy Pathfinding in JavaFX Tower Defense
Tower defense games are not just about placing towers; they also involve sophisticated enemy AI that challenges players' strategies. One of the most crucial components of this AI is pathfinding. This blog post will explore how to implement enemy pathfinding in a JavaFX tower defense game, using the A* algorithm. We'll discuss the underlying principles of pathfinding, provide engaging examples, and offer code snippets that you can easily incorporate into your project.
Understanding the Basics: What is Pathfinding?
Pathfinding refers to the algorithms used by entities to navigate through a space, avoiding obstacles and finding the shortest or most efficient route to a target. In tower defense games, enemies need to reach the player's base while navigating a map filled with towers, walls, and other barriers.
Why Use A* Algorithm?
The A* (A-Star) algorithm combines the benefits of Dijkstra's algorithm and a heuristic approach to find the optimal path. Here are some reasons why A* is particularly suitable for tower defense games:
- Optimal Pathfinding: A* guarantees the shortest path to the destination.
- Heuristic Efficiency: It uses heuristics to prioritize nodes, resulting in faster computations.
- Versatile: A* can adapt to various types of graphs and environments, which is critical in a dynamic game.
Setting Up Your JavaFX Environment
Before diving into coding, ensure you have JavaFX set up in your development environment. You can follow these JavaFX Installation Guides to get started.
Basic Project Structure
Create a basic JavaFX project structure as follows:
/TowerDefense
├── src
│ ├── Main.java
│ ├── Game.java
│ ├── Enemy.java
│ └── Pathfinder.java
└── lib
Implementing the A* Algorithm
Now that we have our project structure, let's start implementing the A* algorithm in our Pathfinder
class.
Pathfinder.java
import java.util.*;
public class Pathfinder {
public List<Node> findPath(Node start, Node goal, Map<Node, List<Node>> graph) {
// Priority queue to hold nodes to explore
PriorityQueue<Node> openSet = new PriorityQueue<>(Comparator.comparingDouble(Node::getF));
Set<Node> closedSet = new HashSet<>();
start.setG(0);
start.setH(heuristic(start, goal));
openSet.add(start);
while (!openSet.isEmpty()) {
Node current = openSet.poll();
// Check if we reached the goal
if (current.equals(goal)) {
return reconstructPath(current);
}
closedSet.add(current);
for (Node neighbor : graph.get(current)) {
if (closedSet.contains(neighbor)) continue; // Ignore the neighbor which is already evaluated
double tentativeG = current.getG() + weight(current, neighbor);
if (tentativeG < neighbor.getG()) {
// This path is better
neighbor.setG(tentativeG);
neighbor.setH(heuristic(neighbor, goal));
neighbor.setParent(current);
if (!openSet.contains(neighbor)) {
openSet.add(neighbor);
}
}
}
}
return Collections.emptyList(); // No path found
}
// Helper methods
private double heuristic(Node a, Node b) {
return Math.abs(a.getX() - b.getX()) + Math.abs(a.getY() - b.getY());
}
private double weight(Node a, Node b) {
return 1; // Adjust according to your game dynamics
}
private List<Node> reconstructPath(Node current) {
List<Node> path = new ArrayList<>();
while (current != null) {
path.add(current);
current = current.getParent();
}
Collections.reverse(path);
return path;
}
}
Commentary on the Code
-
Node Class: Each
Node
represents a grid point in your game. This includes properties like coordinates, G and H scores for the A* algorithm, and a parent node for backtracking. -
Exploration Logic: This algorithm utilizes a priority queue to explore nodes with the lowest F cost first (F = G + H). It checks if the goal has been reached and reconstructs the path if so.
-
Heuristic Function (heuristic): The heuristic function calculates the distance from any given node to the goal. In this case, we use the Manhattan distance, which is perfect for a grid-based map.
Integrating Pathfinding into Your Game
With the A* algorithm implemented, it's time to visualize how enemies can navigate the path to the target. Below is the Enemy
class that uses our Pathfinder
.
Enemy.java
import javafx.scene.shape.Circle;
public class Enemy {
private Node currentNode;
private Circle appearance;
public Enemy(Node start) {
this.currentNode = start;
this.appearance = new Circle(10); // Radius of 10 for the enemy
// Initialize additional properties for visualization here
}
public void move(Node goal, Map<Node, List<Node>> graph) {
Pathfinder pathfinder = new Pathfinder();
List<Node> path = pathfinder.findPath(currentNode, goal, graph);
if (!path.isEmpty()) {
currentNode = path.get(0); // Move to the next node
// Move the enemy's visual representation accordingly
updateAppearance();
} else {
System.out.println("No path found to the target!");
}
}
private void updateAppearance() {
appearance.setTranslateX(currentNode.getX());
appearance.setTranslateY(currentNode.getY());
}
}
Key Points in the Enemy Class
-
Instantiation: The constructor initializes the enemy and its graphical representation.
-
Moving Logic: The
move
method interacts with thePathfinder
class to determine the next step towards the goal. If a path is found, the enemy updates its position. -
Visual Updates: We simulate movement by updating the
Circle
appearance based on the node's coordinates.
Enhancing the Experience with Additional Features
Dynamic Obstacles
In a tower defense game, towers can be placed by players, creating dynamic obstacles. Here is how you can adapt the pathfinding to account for these obstacles.
-
Dynamic Graph: Maintain a graph that updates in real-time as towers are placed. Remove nodes from the graph where towers are built.
-
Re-pathfinding: Whenever a tower is placed, call a method to re-calculate the paths for all enemies on the map.
Considerations for Performance Optimization
-
Caching Paths: Store previously computed paths. If an enemy is approaching the same destination, reuse the calculated path unless changes are made to the graph.
-
Limit Search Space: You can implement an area-of-influence or grid-based search algorithm that limits the exploration area based on the enemies' proximity.
The Closing Argument
By mastering enemy pathfinding through the A* algorithm in your JavaFX tower defense game, you not only elevate gameplay complexity but also create a more engaging experience for players. The combination of sound algorithmic principles with Java expertise allows you to craft an interactive environment that poses challenges for players to conquer.
Feel free to test and implement the example code snippets provided throughout this post. For common challenges in game development or algorithm optimization, be sure to check out resources like Game Development Stack Exchange for community advice. Happy coding, and may your tower defense games be ever strategic!
If you found this post helpful or have further questions, feel free to leave a comment, and I will assist you!
Checkout our other articles