Mastering jOOQ: Common SQL Function Pitfalls to Avoid
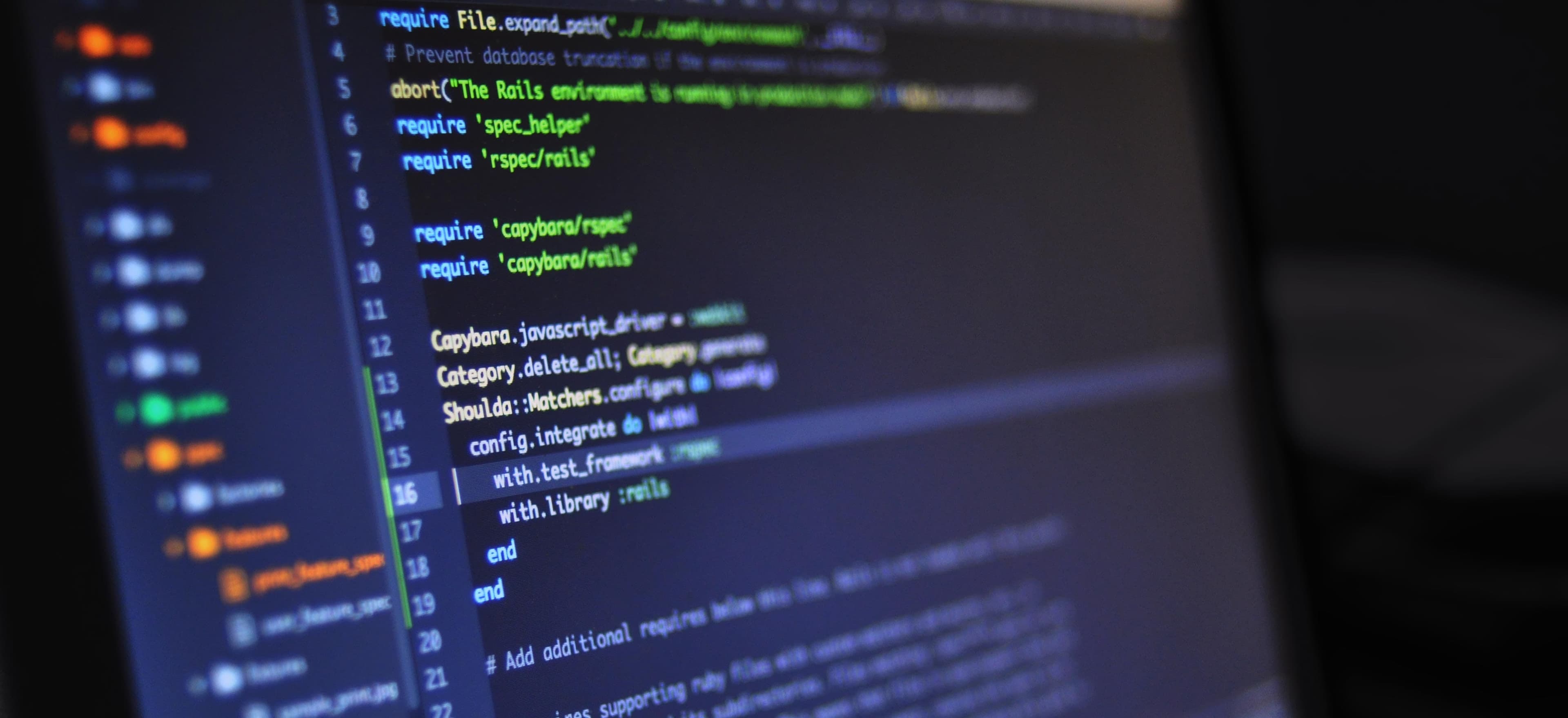
- Published on
Mastering jOOQ: Common SQL Function Pitfalls to Avoid
In the world of database management, SQL functions play a crucial role in data manipulation and retrieval. With libraries like jOOQ (Java Object Oriented Querying), developers can write type-safe SQL in a Java-centric way. However, working with SQL functions in jOOQ can pose challenges. In this blog post, we will explore some common pitfalls developers face while using jOOQ for SQL functions and how to avoid them.
Understanding jOOQ
Before we dive into the pitfalls, let's briefly discuss what jOOQ is and why it's beneficial for Java developers. jOOQ is a popular open-source library that allows you to write typesafe SQL queries directly in Java. It elegantly bridges the gap between object-oriented programming and relational database management, enabling a fluent API style for constructing queries.
For further insights on jOOQ, you can check out the official jOOQ website.
Common SQL Function Pitfalls
1. Misusing SQL Aggregate Functions
SQL aggregate functions like SUM
, AVG
, and COUNT
might seem straightforward, but it's easy to misuse them. One common mistake is forgetting to use them with appropriate grouping, leading to inaccurate results.
Example:
Let’s consider a scenario where you want to calculate the total sales for each product category. Here’s how it could be misused:
dslContext.select(sum(SALES.AMOUNT))
.from(SALES)
.where(SALES.YEAR.eq(2023))
.fetch();
Pitfall: The above query will throw an error or return an incorrect total if there are multiple categories. Without a GROUP BY
clause, the SUM
function aggregates all sales without any distinction.
Solution:
To correct this, use the GROUP BY
clause accordingly:
dslContext.select(CATEGORY.NAME, sum(SALES.AMOUNT))
.from(SALES)
.join(CATEGORY).on(SALES.CATEGORY_ID.eq(CATEGORY.ID))
.where(SALES.YEAR.eq(2023))
.groupBy(CATEGORY.NAME)
.fetch();
By grouping by CATEGORY.NAME
, we ensure the sums are calculated for each category separately.
2. Neglecting Parameter Binding
When constructing queries, always use parameter binding for dynamic inputs. It enhances security by preventing SQL injection attacks and improves performance.
Example:
Instead of writing:
String year = "2023";
Result<?> result = dslContext.select()
.from(SALES)
.where(SALES.YEAR.eq(year))
.fetch();
Pitfall: This string interpolation can be risky. An attacker might exploit potential vulnerabilities if year
is derived from user input.
Solution:
Utilize jOOQ's parameter binding features:
int year = 2023;
Result<?> result = dslContext.select()
.from(SALES)
.where(SALES.YEAR.eq(val(year)))
.fetch();
Using val(year)
securely binds the input, mitigating SQL injection risks.
3. Ignoring Database-Specific Functions
Different databases offer various functions that can significantly enhance your query performance or capabilities. Relying only on standard SQL functions may lead you to miss out on potential optimizations.
Example:
Assuming you want to retrieve the current timestamp but not utilizing the specific database function can be limiting:
dslContext.select(currentTimestamp())
.from(DUAL) // DUAL is specific to Oracle
.fetch();
Pitfall: By not recognizing that some databases support custom functions, you may experience inconsistencies or suboptimal functionality.
Solution:
Always refer to your database's documentation for function availability. For instance, in PostgreSQL, you can directly use:
dslContext.select(pgCurrentTimestamp())
.from(DSL.table("my_table"))
.fetch();
4. Incorrectly Handling Null Values
Null values are common in databases, and mishandling them can lead to unexpected results.
Example:
Consider the case where you want to count non-null entries:
int count = dslContext.select(count(SALES.AMOUNT))
.from(SALES)
.where(SALES.AMOUNT.isNotNull())
.fetchOne(0, Integer.class);
Pitfall: The count might still include entries if handled incorrectly, leading to misleading metrics.
Solution:
Make sure to use the isNotNull()
condition properly, but also consider using coalesce()
to handle null cases effectively:
int totalAmount = dslContext.select(coalesce(sum(SALES.AMOUNT), 0))
.from(SALES)
.fetchOne(0, Integer.class);
This safeguards against possible null returns.
5. Overlooking Performance Optimization
When writing complex queries, performance can become an issue if not carefully managed. Joining too many tables or failing to index can slow down your application.
Example:
This jOOQ query joins several tables without considering performance:
dslContext.select()
.from(SALES)
.join(CATEGORY).on(SALES.CATEGORY_ID.eq(CATEGORY.ID))
.join(PRODUCT).on(SALES.PRODUCT_ID.eq(PRODUCT.ID))
.where(PRODUCT.STATUS.eq("ACTIVE"))
.fetch();
Pitfall: As the number of records grows, performance can degrade significantly.
Solution:
- Always check your database's execution plans.
- Index the necessary columns.
- Simplify queries where possible.
Final Thoughts
By avoiding the aforementioned pitfalls, you can significantly improve your proficiency with jOOQ and SQL functions in general. Understanding aggregation, parameter binding, database-specific functions, null handling, and performance optimization will lead to more robust and efficient applications.
For further reading on jOOQ best practices, consider diving into jOOQ's official documentation which provides comprehensive coverage of its capabilities and functions.
By mastering these techniques, you'll elevate your database-related tasks, ultimately leading to a smoother development experience. Happy coding!
Checkout our other articles