Performance Pitfalls in JavaFX Mobile Applications
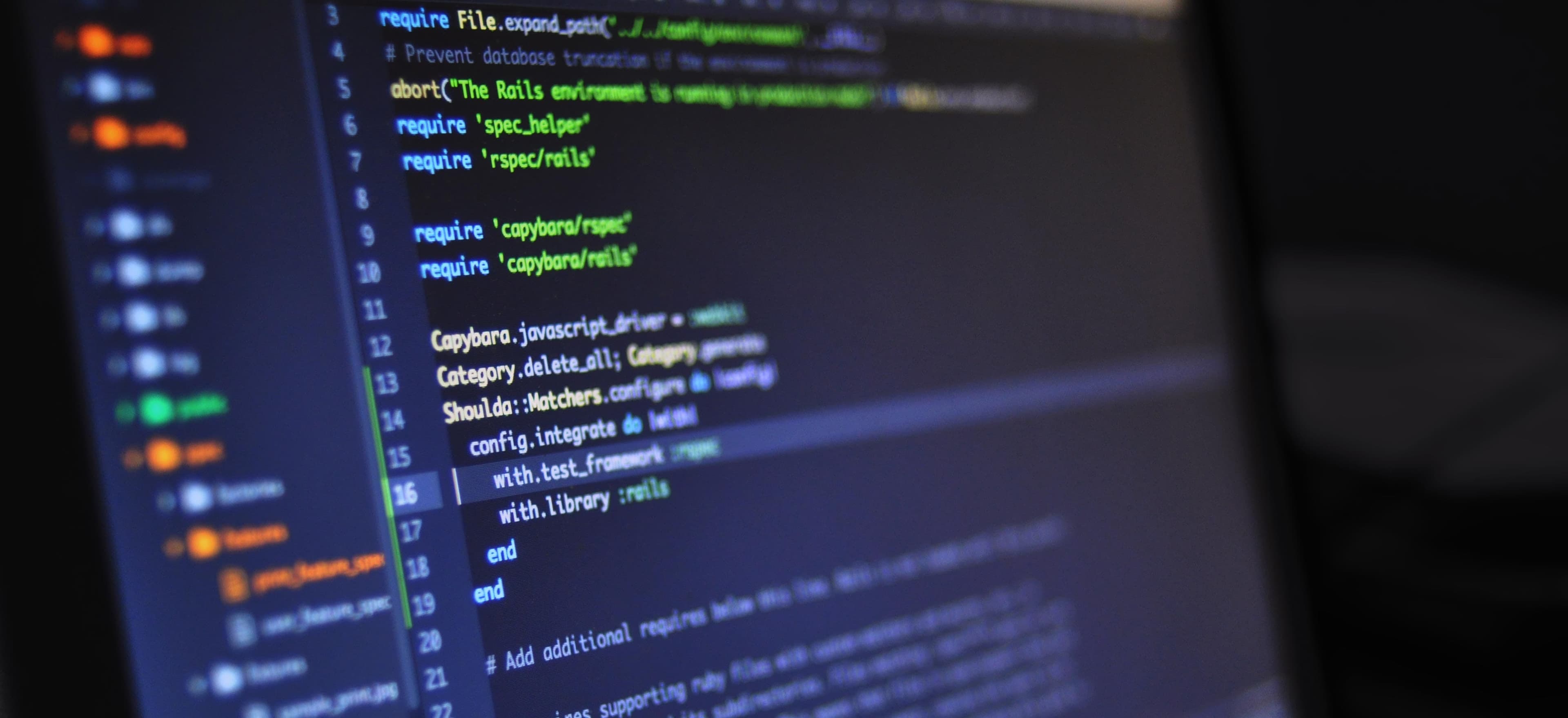
- Published on
Performance Pitfalls in JavaFX Mobile Applications
JavaFX has emerged as a popular framework for building rich internet applications, including mobile apps. Despite its robust capabilities, certain common pitfalls can significantly impact performance, leading to suboptimal user experiences. In this blog post, we will explore these performance pitfalls in JavaFX mobile applications, discuss potential causes, and provide effective solutions to improve performance.
Understanding JavaFX
Before diving into performance pitfalls, it's essential to understand what JavaFX is and why it's used. JavaFX is a powerful framework for building desktop and mobile applications in Java. Its features include:
- A rich set of UI controls.
- Advanced graphics and media capabilities.
- CSS styling and FXML for UI layout.
- Scene graph architecture for efficient rendering.
However, as with any framework, understanding its limitations and potential drawbacks is crucial for developing high-performance applications.
Common Performance Pitfalls
1. Overusing Animation
Why It Matters: Animations can enhance user experience when used correctly. However, excessive or poorly implemented animations can lead to sluggish performance.
Timeline timeline = new Timeline();
timeline.getKeyFrames().add(new KeyFrame(Duration.seconds(1),
new KeyValue(rectangle.translateXProperty(), 300)));
timeline.play();
Analysis: In this example, a timeline animation is utilized on a rectangle. While the animation is straightforward, if you implement numerous simultaneous animations, you may encounter frame drops, especially on lower-end devices.
Solution: Use animations sparingly and consider their necessity. Batch animations and selectively disable them on devices that can’t handle them.
2. Excessive Use of Bindings
Why It Matters: JavaFX offers a powerful binding mechanism that helps to keep the UI in sync with the underlying data model. However, overusing bindings can lead to performance degradation due to increased overhead.
label.textProperty().bind(nameField.textProperty());
Analysis: This code binds a label’s text to the text field. While useful, if you have numerous bindings—especially in a nested structure—it can lead to performance issues.
Solution: Limit the use of binding. Instead, use event listeners or manual updates when it's feasible to improve performance.
3. Inefficient Scene Graph Structure
Why It Matters: The scene graph in JavaFX is crucial for rendering. A poorly structured scene graph can result in long rendering times and unresponsiveness.
Example of a Poor Structure:
Group root = new Group();
for (int i = 0; i < 1000; i++) {
Rectangle rect = new Rectangle(10, 10);
root.getChildren().add(rect);
}
Analysis: Here, a Group is used to create a vast number of rectangles directly. This can make the rendering process heavy and slow.
Solution: Use other nodes, like Pane
or Canvas
, for better performance. Grouping multiple shapes into a single node can reduce the scene graph's complexity.
4. Using Heavy Graphics and Media Resources
Why It Matters: Heavy graphics or large media files can consume significant memory and processing power, leading to slow app performance.
Image image = new Image("large-image.png", true);
ImageView imageView = new ImageView(image);
Analysis: If the selected graphics assets are too large, they may hinder the app’s performance, especially on mobile devices.
Solution: Optimize images and media files for size and resolution before using them in your application. Use formats like SVG for vector graphics where possible.
5. Ignoring the Mobile Platform's Limitations
Why It Matters: Developing for mobile requires an understanding of the specific limitations of mobile platforms. Not considering these can result in poor performance.
Example: Unused resources
public void initResources() {
// Initializing resources...
// Loading high-resolution images that may not be necessary
}
Analysis: Loading resources that exceed device capabilities can hurt performance and lead to crashes.
Solution: Detect the device type at runtime and load resources according to the capabilities of the device. Use lower resolution resources on weaker devices.
Best Practices for Performance Optimization
Use JavaFX CSS Wisely
JavaFX supports CSS for styling applications. However, overusing complex styles can put a strain on the rendering engine:
.button {
-fx-font-size: 16px;
-fx-background-color: #ff0000;
}
Tip: Simplify stylesheets and avoid deep selector hierarchies.
Manage Memory Wisely
Garbage collection can hinder performance if not managed properly:
List<Circle> shapes = new ArrayList<>();
for (int i = 0; i < 10000; i++) {
shapes.add(new Circle(5));
}
Tip: Be cautious with large collections and also remove unneeded objects to allow garbage collection to reclaim memory proactively.
Profile Your Application
Java provides profiling tools, which help identify bottlenecks. Use tools like VisualVM or Java Mission Control.
Tip: Regularly profile your mobile application during development and testing to catch performance issues early.
Optimize Event Handling
While it's easy to create multiple event handlers, each handler incurs a performance cost:
Button button = new Button("Click me");
button.setOnAction(event -> System.out.println("Button clicked!"));
Tip: Minimize event listeners or handlers where possible, and consider handling events at a shared central point rather than for each element.
Key Takeaways
JavaFX offers a powerful platform for mobile development, but it is crucial to remain vigilant about performance pitfalls. From overusing animations and bindings to mismanaging resources, developers must be aware and proactive about addressing these areas. By adhering to best practices and optimizing your applications thoughtfully, you can enjoy all the benefits of JavaFX without compromising performance.
For further reading on JavaFX performance optimizations, consider checking out Oracle's official documentation and exploring community resources.
By focusing on these pitfalls and solutions, your JavaFX mobile applications can deliver a smooth and responsive user experience that keeps users engaged and satisfied.