Top 5 Common Pitfalls When Using Google Guava
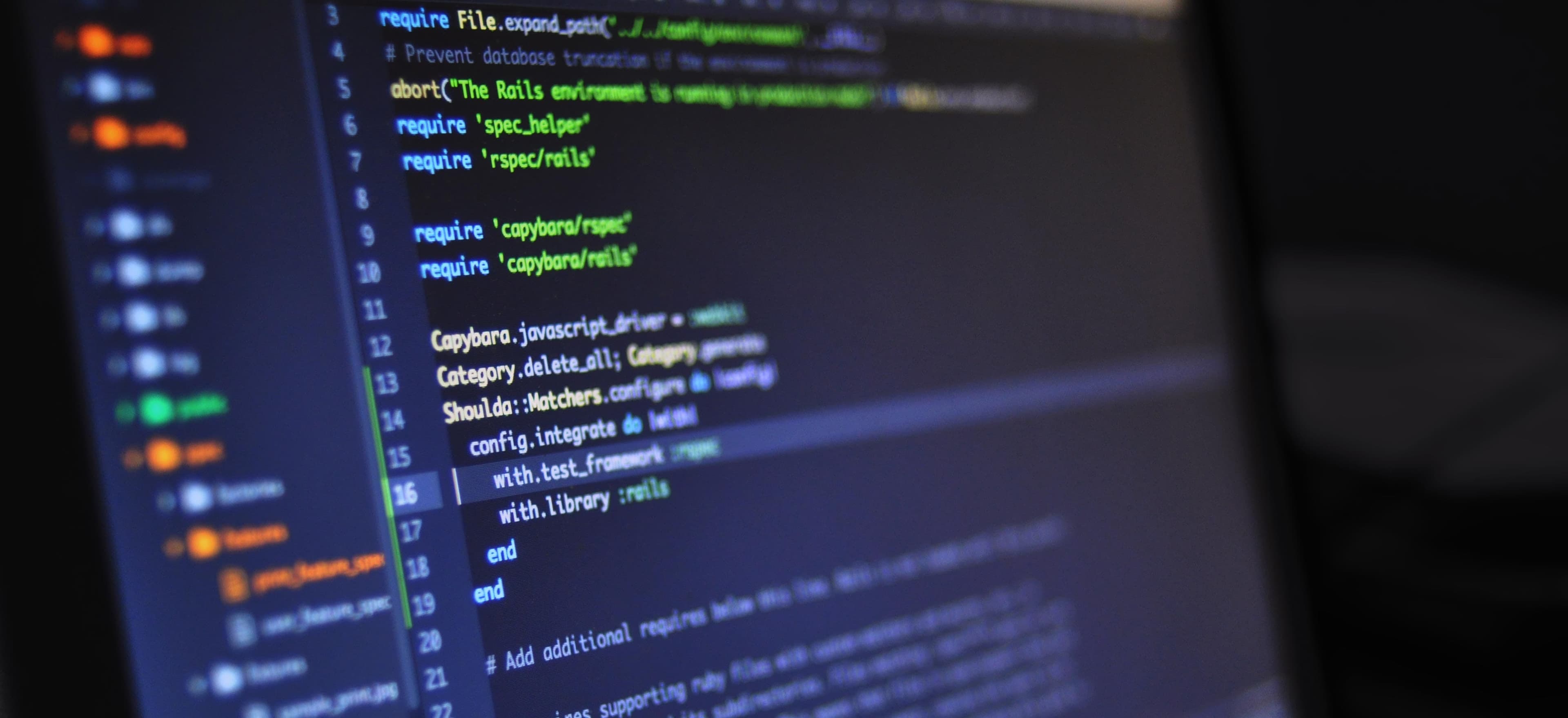
- Published on
Top 5 Common Pitfalls When Using Google Guava
Google Guava is a powerful set of core libraries that extend Java's standard libraries. With its rich collections, caching, primitives support, concurrency utilities, and much more, Guava aims to help you write clean, efficient, and maintainable code. However, like any library, there are certain pitfalls that developers often encounter. In this post, we'll explore the top five common pitfalls when using Google Guava and how to avoid them, enhancing your coding practices along the way.
1. Overusing Immutable Collections
The Pitfall
One of Guava's standout features is its support for immutable collections. While immutability is great for thread safety and protecting your data from unintended modifications, creating an immutable collection for every use case can lead to performance issues.
The Solution
Use immutable collections when you truly need data integrity and don’t anticipate further modifications. For example, if your data set will remain constant throughout its lifetime, use ImmutableList
:
import com.google.common.collect.ImmutableList;
ImmutableList<String> immutableList = ImmutableList.of("Java", "Guava", "Programming");
In contrast, if you need to perform many modifications, a mutable collection like ArrayList
is more efficient:
import java.util.ArrayList;
import java.util.List;
List<String> mutableList = new ArrayList<>();
mutableList.add("Java");
mutableList.add("Guava");
mutableList.add("Programming");
Why This Matters
Using immutable collections unnecessarily can create performance bottlenecks, especially in scenarios involving frequent updates. Note that immutability should be a choice based on your data's nature and manipulation requirements.
2. Ignoring Optional
The Pitfall
Guava provides the Optional
class as a neat way to handle nullable values and avoid NullPointerExceptions
. However, many developers either overlook its use or misuse it by wrapping non-nullable values, disregarding its primary purpose.
The Solution
Use Optional
to represent a value that may or may not be present. Here’s how to utilize it correctly:
import com.google.common.base.Optional;
Optional<String> optionalString = Optional.of("Hello, Guava!");
If you might have a null value:
String value = getSomeString(); // This method might return null
Optional<String> optionalString = Optional.fromNullable(value);
Why This Matters
By avoiding the NullPointerException
and using Optional
, you make your intentions clear and your code more robust. Optional
serves as a signal to both developers and users that a value might not be provided, promoting better design.
3. Misusing Caching with CacheBuilder
The Pitfall
Guava's caching utilities provide a straightforward way to store and retrieve data efficiently. Misconfiguration, however, can lead to memory leaks or excessive memory use.
The Solution
Use CacheBuilder
wisely to establish the right cache size, expiration time, and eviction policies. Here's an example of an optimal cache setup:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.maximumSize(1000) // Limit to 1000 entries
.expireAfterWrite(10, TimeUnit.MINUTES) // Expire entries after 10 minutes
.build(new CacheLoader<String, String>() {
@Override
public String load(String key) {
return fetchFromDatabase(key);
}
});
Why This Matters
Setting limits and expirations prevents endless memory consumption. If your cache grows unbounded, the performance can degrade, leading to increased latency and potential server crashes.
4. Using Joiner Without Proper Formatting
The Pitfall
Guava’s Joiner
makes it easy to join collections into a single string. However, developers often forget to properly handle nulls and whitespace, which can lead to unexpected results.
The Solution
Utilize Joiner
’s built-in methods for cleaner results. Here's how you can join a collection of strings with proper null handling:
import com.google.common.base.Joiner;
List<String> strings = Arrays.asList("Java", null, "Guava", " ");
// This will ignore nulls and trim whitespace
String result = Joiner.on(", ").skipNulls().join(strings);
Why This Matters
Failing to handle nulls might yield a non-informative output, such as Java, , Guava,
. Use the available options to ensure your output maintains the desired format.
5. Misunderstanding Function Types
The Pitfall
Guava provides various functional types for cleaner code such as Function
, Predicate
, and Transformer
. New users often misuse these or choose the wrong type, leading to complex and unoptimized code.
The Solution
Understanding and correctly applying these function types is crucial. Here’s an example of using Function
correctly:
import com.google.common.base.Function;
import com.google.common.collect.Lists;
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<Integer> nameLengths = Lists.transform(names, new Function<String, Integer>() {
@Override
public Integer apply(String name) {
return name.length();
}
});
Why This Matters
Choosing the correct functional type can enhance code readability and maintainability. Using them properly allows you to write declarative and concise code, reducing boilerplate and potential errors.
Final Thoughts
Google Guava is an extensive library that can significantly enhance your Java development experience. However, its misuse can lead to pitfalls that hinder performance and clarity. By being mindful of these common errors—overusing immutable collections, ignoring Optional
, misconfiguring caches, misusing Joiner
, and misunderstanding function types—you can harness Guava's full potential.
For further reading about Google Guava, check out the official documentation for detailed insights and a deeper understanding of the library. Happy coding!
By following these guidelines, you will be able to make more informed decisions while using Google Guava in your Java projects. If you have further tips or experiences dealing with these pitfalls, feel free to share them in the comments below!
Checkout our other articles