Common Mistakes When Using Java HashMap Explained
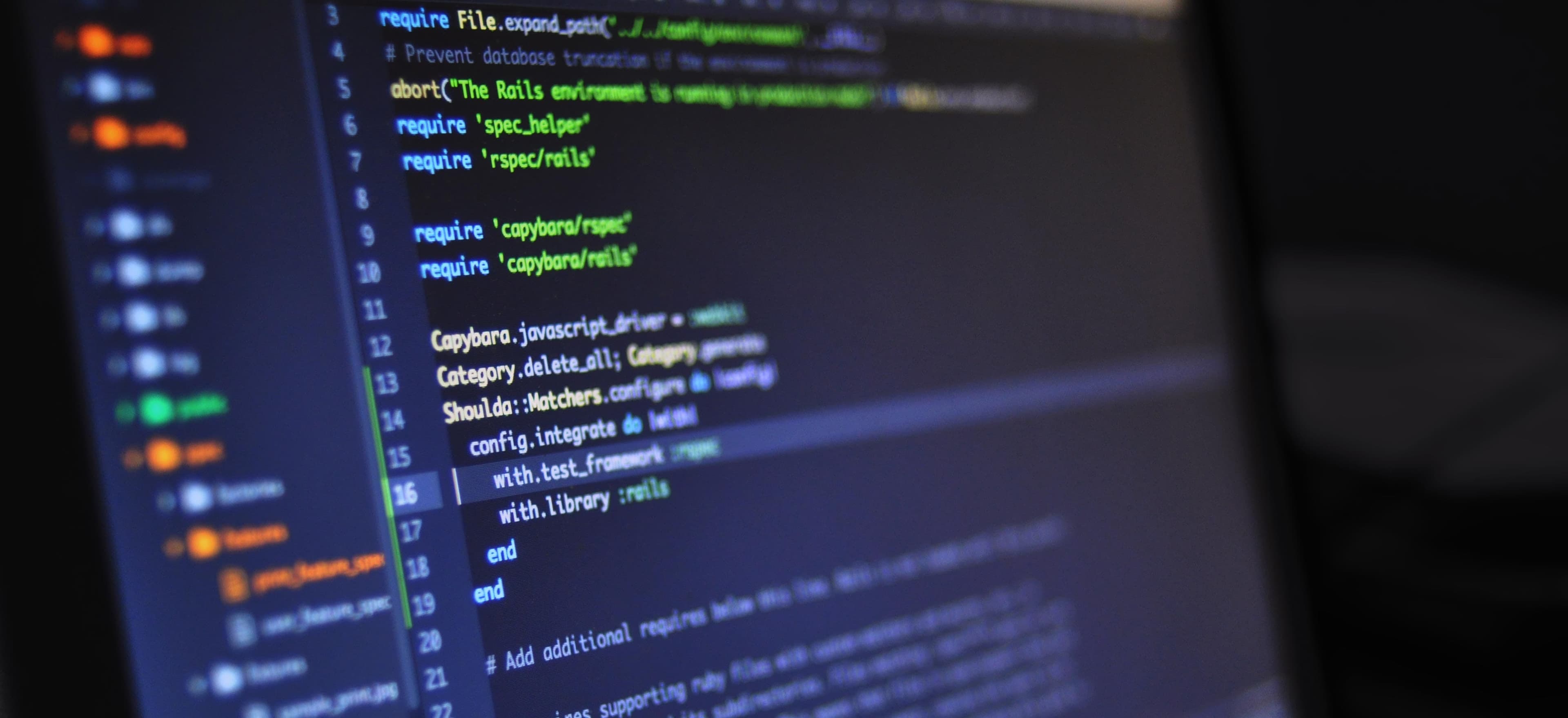
- Published on
Common Mistakes When Using Java HashMap Explained
In the realm of Java programming, the HashMap
class is a go-to solution for many developers. Its ease of use and efficiency make it a powerful tool for storing key-value pairs. However, missteps in utilizing HashMap
can lead to inefficiencies and bugs. This blog post will dive deep into common mistakes developers make when using HashMap
and how to avoid them.
What is a HashMap?
Before we delve into the mistakes, let's briefly understand what a HashMap
is. A HashMap
is part of the Java Collection Framework and represents a collection that maps keys to values. It allows null values and the null key. It is based on a hash table, and the basic operations—adding, removing, and searching—can be performed in constant time on average.
Common Mistakes with Java HashMap
1. Ignoring the Importance of hashCode()
One common mistake is failing to properly override the hashCode()
method for custom key classes. The performance and correctness of HashMap
rely heavily on the correct implementation of the hashCode()
and equals()
methods.
Example:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return age == person.age && Objects.equals(name, person.name);
}
}
Why Avoiding This Is a Mistake:
If you do not override hashCode()
as shown, two different instances of Person
with the same name and age will occupy different buckets in the HashMap
, leading to unexpected results during retrieval.
2. Changing Key Objects
Another frequent error is modifying a key object after it has been added to the HashMap
.
Example:
Map<Person, String> map = new HashMap<>();
Person person = new Person("Alice", 30);
map.put(person, "Engineer");
// Changing the state of the `person` object
person.age = 31;
// Now, retrieving the value won't work as intended
System.out.println(map.get(person)); // throws null
Why Avoiding This Is a Mistake:
Once a key object is placed in a HashMap
, changing its internal state affects its hash code and, more importantly, its position in the hash table, making it impossible to retrieve the value.
3. Using null
Keys and Values Incorrectly
HashMap
allows one null key and multiple null values. However, improper use might lead to confusion or errors.
Example:
Map<String, String> map = new HashMap<>();
map.put(null, "No Key!");
System.out.println(map.get(null)); // Works perfectly
Why Avoiding This Is a Mistake:
Over-reliance on null keys can make code unreadable and difficult to manage. It is advisable to avoid using null keys unless absolutely necessary.
4. Not Understanding Initial Capacity and Load Factor
While you can create a HashMap
with a default initial capacity of 16 and a load factor of 0.75, ignoring these parameters can lead to performance issues. If your HashMap
grows past those thresholds, it will resize, which is an expensive operation.
Example:
Map<String, String> map = new HashMap<>(100, 0.9f);
Why Avoiding This Is a Mistake:
Setting appropriate initial capacity and load factor can minimize resizing. If you expect your HashMap
to have a significant number of entries, initializing it correctly will improve the performance.
5. Underestimating the containsKey
Method
Some developers rely on catching NullPointerExceptions
rather than using containsKey
.
Example:
if (map.get("key") != null) {
// Do something
}
Why Avoiding This Is a Mistake:
If the key doesn't exist in the HashMap
, get
returns null. This could lead to false positives if null is a valid value associated with a key. Instead, use containsKey
.
if (map.containsKey("key")) {
// Safe to use map.get("key")
}
6. Failing to Synchronize for Multi-threading
Using HashMap
in a multi-threaded environment without synchronization can result in unpredictable behavior and data inconsistency.
Example:
Map<String, String> map = new HashMap<>();
Runnable task = () -> {
map.put("key", "value");
};
// Two threads trying to operate on the same HashMap.
new Thread(task).start();
new Thread(task).start();
Why Avoiding This Is a Mistake:
Explore ConcurrentHashMap
as a thread-safe alternative. It allows concurrent reads and writes without the need for external synchronization, providing better performance in a multi-threaded context.
7. Inefficient Key Searches
Sometimes developers do a search for a key without taking advantage of the underlying benefits of HashMap
. Using a linear search on entries can drastically reduce performance.
Example:
for (Map.Entry<String, String> entry : map.entrySet()) {
if (entry.getKey().equals("key")) {
// Do something
}
}
Why Avoiding This Is a Mistake:
Instead, make use of the containsKey
method:
if (map.containsKey("key")) {
// Do something
}
Bringing It All Together
A HashMap
is an excellent tool in the Java developer's toolkit, but it comes with its own set of pitfalls. Understanding and avoiding these common mistakes will enhance the efficiency and readability of your Java code. By properly managing keys, employing good practices with hashCode()
and equals()
, and utilizing thread-safe alternatives where needed, you can leverage the full power of HashMap
in Java.
For further reading on HashMaps, you might explore the official Java documentation here or an in-depth article on Java Collections. Happy coding!