Mastering Optional Values in Hands-On Programming
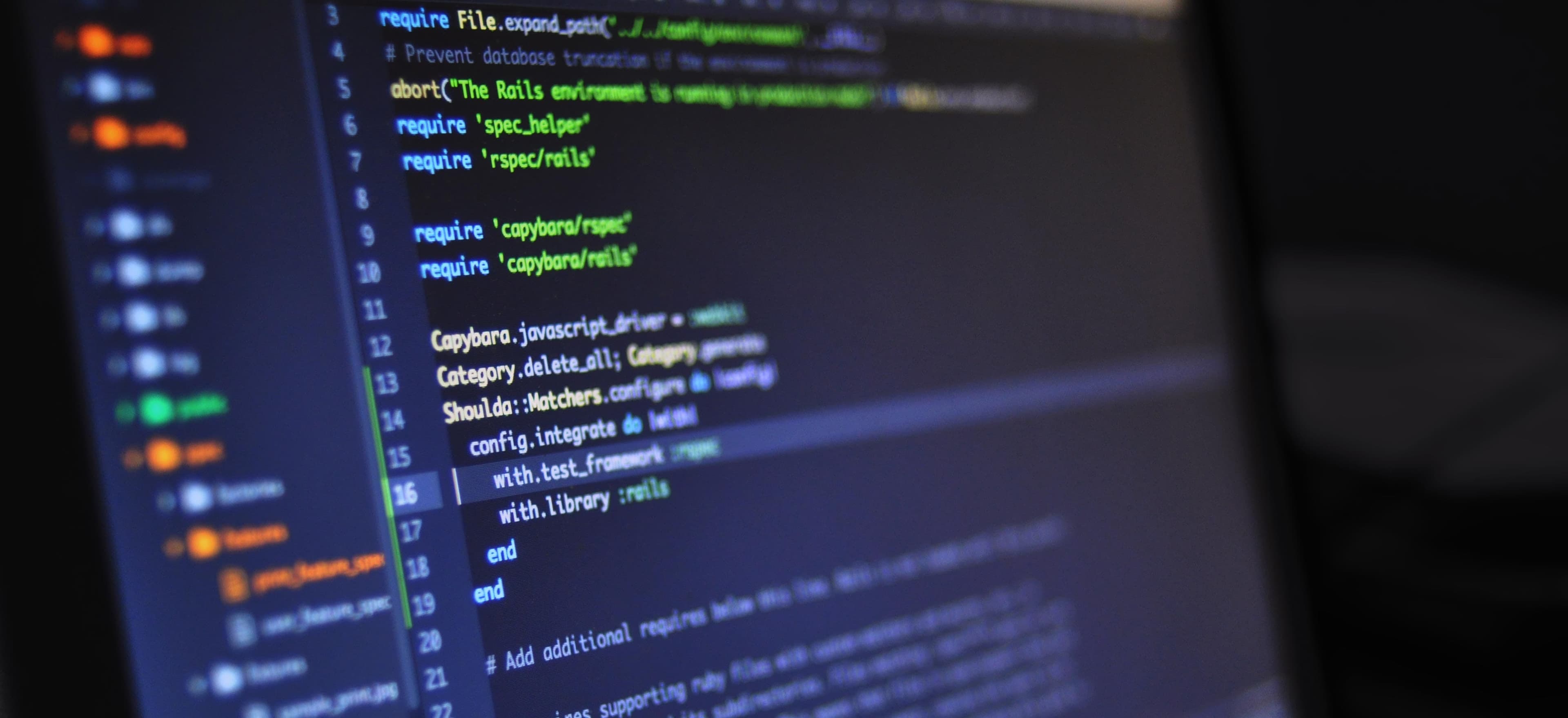
- Published on
Mastering Optional Values in Hands-On Programming
In the realm of Java programming, handling values that may or may not be present is a fundamental challenge that developers frequently encounter. This is where Optional values come into play. Introduced in Java 8, the Optional
class is a container that may or may not hold a non-null value. By leveraging Optional
, Java developers can write more robust, readable, and maintainable code.
In this blog post, we will delve into the concept of Optional
, explore its properties, methods, and best practices, and provide hands-on code snippets to solidify understanding.
What is Optional?
An Optional
is often said to be a better tool for handling null-checking. Instead of returning null from methods (which can lead to NullPointerExceptions
), a method can return an Optional
. This signals the intention that the result may or may not be present.
Why Use Optional?
- Clarity: Using
Optional
makes it clear to the caller that the return value may not be present. - Avoids NullPointerExceptions: Eliminates many common sources of errors related to null handling.
- Functional Style: Supports functional programming constructs such as streams and lambda expressions.
For more background on the need for Optional
, you can check out this guide.
Creating Optional Instances
Creating an Optional
instance is straightforward. Here are the most common ways to do so:
Using Optional.of()
This method requires a non-null value.
String value = "Hello, World!";
Optional<String> optionalValue = Optional.of(value);
Why: It guarantees that the provided value is not null. If it is, it throws a NullPointerException
, hence enforcing non-null values.
Using Optional.ofNullable()
This method can accept both null and non-null values.
String nullableValue = null;
Optional<String> optionalNullable = Optional.ofNullable(nullableValue);
Why: This is useful when you have a value that may or may not be present and you want to handle both scenarios gracefully.
Using Optional.empty()
If you need an empty Optional
, you can use:
Optional<String> emptyOptional = Optional.empty();
Why: It serves as a placeholder for when no value is available, making your intention explicit.
Accessing Optional Values
Once we have an Optional
, we need to know how to access the value inside it. Below are the key methods for accessing the content of an Optional
.
isPresent()
To check if a value is present, you can use isPresent()
.
if (optionalValue.isPresent()) {
System.out.println(optionalValue.get());
} else {
System.out.println("Value not present");
}
Why: This check prevents NoSuchElementException
when attempting to retrieve the value.
ifPresent()
A more functional approach to handle presence can be accomplished using ifPresent()
.
optionalValue.ifPresent(value -> System.out.println("Value: " + value));
Why: This approach allows you to execute a block of code only if the value is present, making your code more concise.
orElse() and orElseGet()
To retrieve a value or provide a default, use orElse()
or orElseGet()
.
String defaultValue = optionalNullable.orElse("Default Value");
System.out.println(defaultValue);
Why: These methods enable a fallback mechanism in a single line. This helps maintain cleaner code and avoids cumbersome null checks.
Stream Support
The Optional
class also supports Streams.
optionalValue.stream().forEach(System.out::println);
Why: This can lead to even more concise code in scenarios where you would typically use collections.
Transforming Optional Values
map()
You can transform an Optional
into another using the map()
method:
Optional<String> upperOptional = optionalValue.map(String::toUpperCase);
upperOptional.ifPresent(System.out::println);
Why: This allows for a composed function approach, where transformations or calculations can be chained.
flatMap()
Unlike map()
, flatMap()
is used when your mapping function returns another Optional
.
Optional<Integer> lengthOptional = optionalValue.flatMap(s -> Optional.of(s.length()));
lengthOptional.ifPresent(System.out::println);
Why: This prevents nested Optional
values, enabling clearer and cleaner code.
Best Practices
- Prefer Optional over null: Use
Optional
as an alternative to returning null, especially in method signatures. - Avoid Optional for Fields: Use
Optional
for method return types instead of class fields to avoid complications. - Chains over null checks: Utilize functional methods like
map()
,flatMap()
, andifPresent()
to reduce clutter in your code. - Avoid use in collections: Using
Optional
as part of collections can lead to complex code. Instead, use it as a return type.
A Final Look
In mastering the Optional
class, Java developers gain a powerful tool to handle values that may or may not exist. By implementing Optional
in your code, you not only improve its robustness but also enhance readability and maintainability. Understanding its methods and how to use them effectively enjoys a decisive advantage in modern Java programming.
For a deeper dive into the Optional
class and its use cases, you can explore the official Java documentation.
In your next Java project, consider integrating Optional
where applicable and experience the clarity and safety it brings to your programming practice. Happy coding!