Avoiding Common Pitfalls in JPA Query Validation
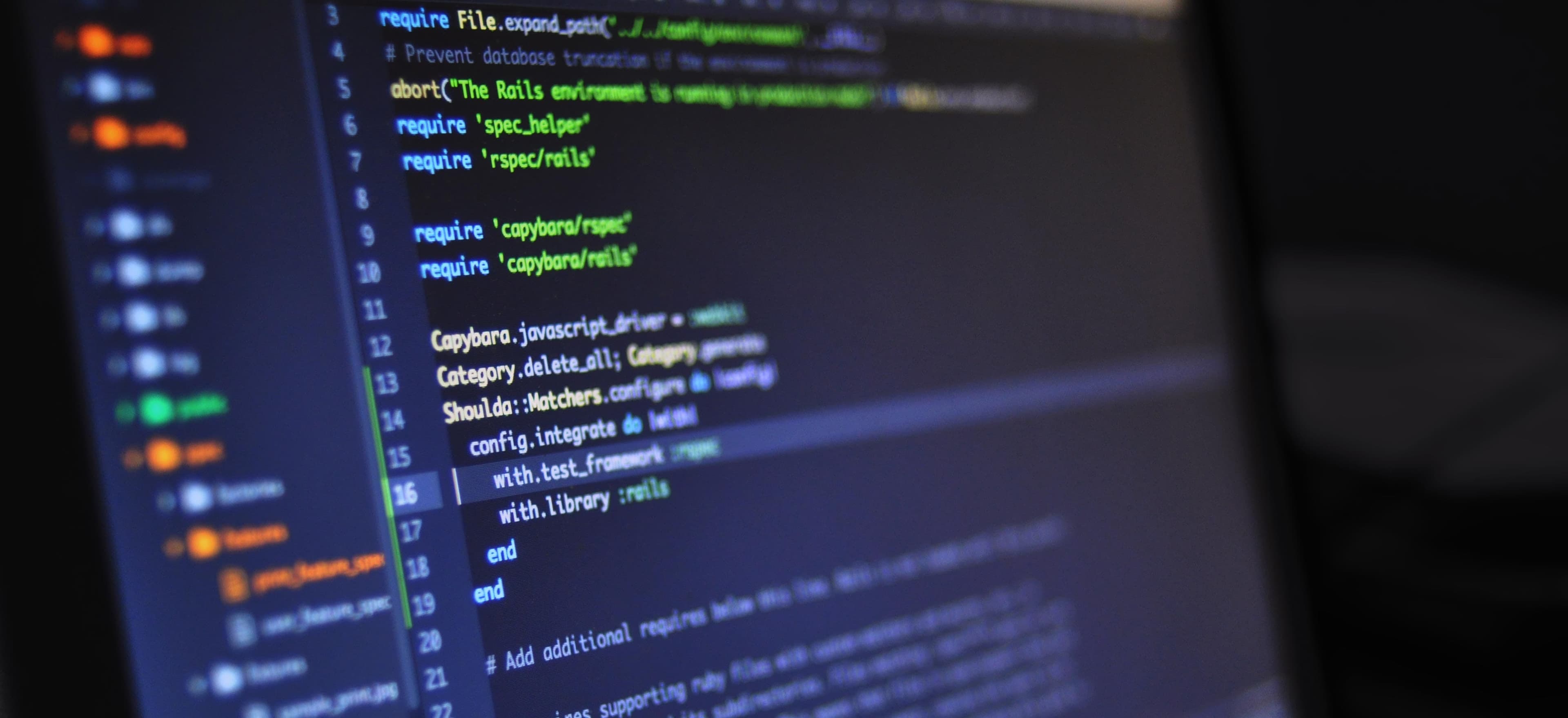
- Published on
Avoiding Common Pitfalls in JPA Query Validation
Java Persistence API (JPA) simplifies database interactions in Java applications, but it is not without its challenges. One common area that developers stumble upon is query validation. In this blog post, we will explore some of the typical pitfalls that arise when working with JPA queries, how to avoid these issues, and best practices for writing robust JPA queries.
By the end of this article, you will have a clearer understanding of best practices in JPA query validation, making your data access layer more reliable and maintainable.
What is JPA?
Java Persistence API (JPA) is a specification for accessing, persisting, and managing data between Java objects and relational databases. It provides a way for Java developers to interact with databases through an object-oriented model, eliminating much of the boilerplate code involved in traditional JDBC operations.
For more details about JPA, you can check the official documentation here.
Understanding JPA Queries
JPA allows developers to create queries using two primary methods:
- JPQL (Java Persistence Query Language) - A query language that operates on the entity object model rather than directly on the database tables.
- Criteria API - A programmatic way to construct queries in a type-safe manner.
Both methodologies have their pros and cons, especially when it comes to validation and error handling. Let's dive deeper into avoiding common pitfalls in JPA query validation.
Pitfall #1: Lack of Error Handling
The Issue
One of the most common mistakes developers make is neglecting proper error handling when executing JPA queries. This could be a result of a non-existent table, a syntax error, or a misconfiguration in the Entity or DataSource.
Resolution
Always wrap your query execution in try-catch blocks and handle specific exceptions appropriately. Utilize the EntityManager
and check for PersistenceException
or QueryTimeoutException
.
Example
import javax.persistence.EntityManager;
import javax.persistence.PersistenceException;
public void executeQuery(EntityManager entityManager) {
try {
entityManager.createQuery("FROM User").getResultList();
} catch (PersistenceException e) {
// Log the error or handle it
System.err.println("Error executing query: " + e.getMessage());
}
}
Why: This approach ensures that your application can gracefully handle issues during query execution, enhancing user experience and improving maintainability.
Pitfall #2: Ignoring Parameter Binding
The Issue
String concatenation can lead to SQL injection attacks, as well as syntax errors. Forgetting to bind parameters correctly can also lead to runtime exceptions.
Resolution
Always use parameter binding in your JPA queries. This protects against SQL injection and also makes your code cleaner and easier to read.
Example
String username = "john_doe";
try {
List<User> users = entityManager.createQuery("SELECT u FROM User u WHERE u.username = :username", User.class)
.setParameter("username", username)
.getResultList();
} catch (PersistenceException e) {
// Handle exception
}
Why: Binding parameters not only secures your application but also reduces syntax errors since the JPA implementation will ensure that the values are appropriately handled.
Pitfall #3: Using Raw SQL Queries
The Issue
While JPA supports raw SQL queries, overusing them can undermine the benefits of JPA, like improved readability and maintainability. Raw SQL also makes it harder to refactor or change the underlying database.
Resolution
Stick to JPQL or the Criteria API as much as possible. If you must use raw SQL, ensure to validate the SQL syntax separately and handle potential errors.
Example
String rawSql = "SELECT * FROM users WHERE username = ?";
Query query = entityManager.createNativeQuery(rawSql, User.class);
query.setParameter(1, username);
List<User> users = query.getResultList();
Why: While it might be tempting to use raw SQL for complex queries, it can introduce potential errors and vulnerabilities, as well as decrease the portability of your code across different databases.
Pitfall #4: Improper or Missing Transaction Management
The Issue
When dealing with multiple operations, especially writes, failing to manage transactions can lead to data inconsistency. A common mistake is executing queries without wrapping them in transaction boundaries.
Resolution
Ensure that all JPA operations that modify data are wrapped in transactions. This ensures that either all changes are saved or none, preserving data integrity.
Example
public void updateUser(User user) {
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
entityManager.merge(user);
transaction.commit();
} catch (PersistenceException e) {
transaction.rollback(); // Ensure rollback on error
System.err.println("Transaction failed: " + e.getMessage());
}
}
Why: Transaction management is essential for ensuring the reliability of your data operations. It prevents partial updates and maintains consistency across your database.
Pitfall #5: Forgetting to Flush or Clear
The Issue
When executing queries, the entity manager operates in a persistent context, which may lead to unexpected results if the context is not flushed or cleared appropriately.
Resolution
Use flush()
to synchronize the current state of persistence context with the database or clear()
to detach entities when necessary.
Example
public void saveUser(User user) {
entityManager.persist(user);
entityManager.flush(); // Ensure immediate synchronization
}
Why: Flushing can help in ensuring that the intended operations are performed immediately, making troubleshooting easier. Clearing the persistence context can also help in managing memory efficiently.
Best Practices for JPA Queries
-
Always Use Parameter Binding: Adhere to this to prevent SQL injection.
-
Practice Consistent Error Handling: Centralize your error-handling strategies for better maintainability.
-
Use JPQL/Criterion API: Avoid raw SQL wherever possible for maintainability and portability.
-
Manage Transactions: Always manage transactions carefully to avoid data inconsistency.
-
Optimize Fetching Strategies: Use appropriate fetching strategies (
EAGER
andLAZY
) to improve performance.
The Bottom Line
Understanding and avoiding the common pitfalls in JPA query validation is crucial for building robust applications. By following best practices like proper error handling, using parameter binding, managing transactions, and adhering to the query language best suited for your use case, you can significantly enhance the reliability of your data access layer.
As you develop your application, remember that thorough testing and consistent validation can go a long way in ensuring that your JPA queries execute correctly and efficiently.
For further reading on JPA and advanced querying techniques, you might find these resources useful:
Happy coding!