Overcoming Common Challenges in OOP Design Principles
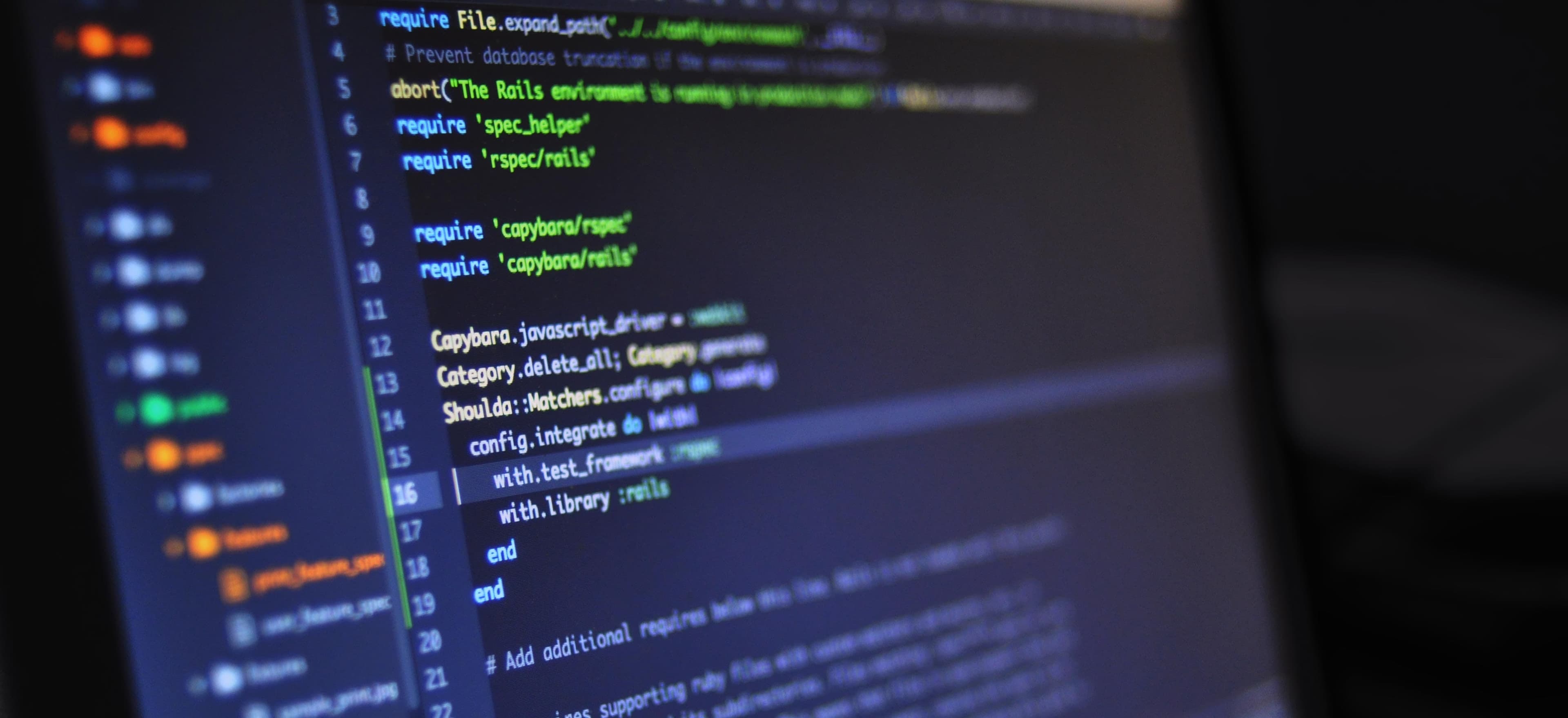
- Published on
Overcoming Common Challenges in OOP Design Principles
Object-Oriented Programming (OOP) is a powerful paradigm that can help create robust, reusable, and maintainable code. While OOP has numerous benefits, developers often encounter challenges in applying its principles effectively. This blog post explores these common challenges and provides actionable solutions, illustrated with Java code snippets.
Table of Contents
- Understanding OOP Design Principles
- Common Challenges in OOP
- Inheritance Complexity
- Code Duplication
- Tight Coupling
- Lack of Proper Abstraction
- Solutions to Overcome Challenges
- Effective Use of Inheritance and Composition
- Utilizing Design Patterns
- Implementing Interfaces and Abstract Classes
- Conclusion
Understanding OOP Design Principles
Before diving into the challenges, let's briefly revisit the core principles of OOP:
- Encapsulation: Bundling data and methods operating on that data into a single unit.
- Abstraction: Hiding complex implementation details and showing only essential features.
- Inheritance: Creating new classes based on existing ones, promoting code reuse.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
These principles provide a foundational structure for building applications. However, their correct implementation is crucial to avoiding potential pitfalls.
Common Challenges in OOP
Inheritance Complexity
Inheritance can lead to complicated class hierarchies. While it promotes code reuse, a deep inheritance tree can make it challenging to understand the relationships between classes.
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
class Dog extends Animal {
void bark() {
System.out.println("The dog barks.");
}
}
class Beagle extends Dog {
void sniff() {
System.out.println("The Beagle sniffs.");
}
}
In this example, you'd need to look up the entire chain to understand what an instance of Beagle
can do, which adds unnecessary complexity.
Code Duplication
Another common issue arises when developers try to implement similar functionality across multiple classes. This violation of the DRY (Don't Repeat Yourself) principle leads to code duplication and maintenance headaches.
class Car {
void start() {
System.out.println("Car started.");
}
}
class Bike {
void start() {
System.out.println("Bike started.");
}
}
The start
method's functionality is duplicated here, which is not ideal.
Tight Coupling
Overly interconnected classes can lead to tight coupling, making it difficult to modify one part of the code without affecting others. This can hinder scalability and make unit testing cumbersome.
class Engine {
void startEngine() {
System.out.println("Engine started.");
}
}
class Car {
private Engine engine = new Engine();
void start() {
engine.startEngine();
}
}
In this case, the Car
class relies directly on the Engine
class, creating a tightly coupled design.
Lack of Proper Abstraction
OOP aims to simplify complex systems through abstraction. A failure to abstract can result in systems that are hard to understand and maintain.
class DataManager {
void saveData(String data) {
// Implementation details
}
}
class ReportManager {
void createReport() {
// Uses DataManager
}
}
Here, DataManager
does not provide an abstract interface for data handling, complicating interactions for ReportManager
.
Solutions to Overcome Challenges
Effective Use of Inheritance and Composition
A balanced approach to inheritance and composition can mitigate complexity. Prefer composition over inheritance, especially for "has-a" relationships.
class Engine {
void start() {
System.out.println("Engine started.");
}
}
class Car {
private Engine engine; // Composition
Car() {
engine = new Engine();
}
void start() {
engine.start();
}
}
This design simplifies the relationships between classes, allowing for better flexibility and easier testing.
Utilizing Design Patterns
Design patterns provide standard solutions to common design problems. For instance, the Strategy Pattern can help reduce repetitive code and create clear abstractions.
interface EngineStrategy {
void startEngine();
}
class PetrolEngine implements EngineStrategy {
public void startEngine() {
System.out.println("Petrol engine started.");
}
}
class DieselEngine implements EngineStrategy {
public void startEngine() {
System.out.println("Diesel engine started.");
}
}
class Car {
private EngineStrategy engineStrategy;
Car(EngineStrategy strategy) {
this.engineStrategy = strategy;
}
void start() {
engineStrategy.startEngine();
}
}
By using the Strategy Pattern, you can swap out engines without altering the Car
class, ensuring flexibility and adherence to the Open/Closed Principle.
Implementing Interfaces and Abstract Classes
Utilizing abstract classes and interfaces can help reduce tight coupling and strengthen your code's architecture.
interface Shape {
double area();
}
class Circle implements Shape {
private double radius;
Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
Through the use of interfaces, you are creating a greater degree of flexibility. The Shape
interface decouples the code from specific implementations, making it easier to incorporate new shapes without altering existing code.
Final Considerations
Object-Oriented Programming is an exceptionally powerful paradigm, but it is not without its challenges. By focusing on effective use of inheritance and composition, applying design patterns judiciously, and implementing interfaces and abstract classes correctly, developers can significantly mitigate these challenges.
The success of an OOP design lies in the code's maintainability and extendability over time. By overcoming these common challenges, you can build software that not only meets its current requirements but is also prepared for future changes.
Whether you are a Java beginner or an experienced developer, revisiting and refining your understanding of OOP principles will always yield benefits. For more in-depth discussions on Java and OOP, feel free to explore Java Documentation and Design Patterns Book.
Transform your code today by prioritizing clear structure and design principles; your future self will thank you!