Understanding the Pitfalls of Class-Variable Overrides
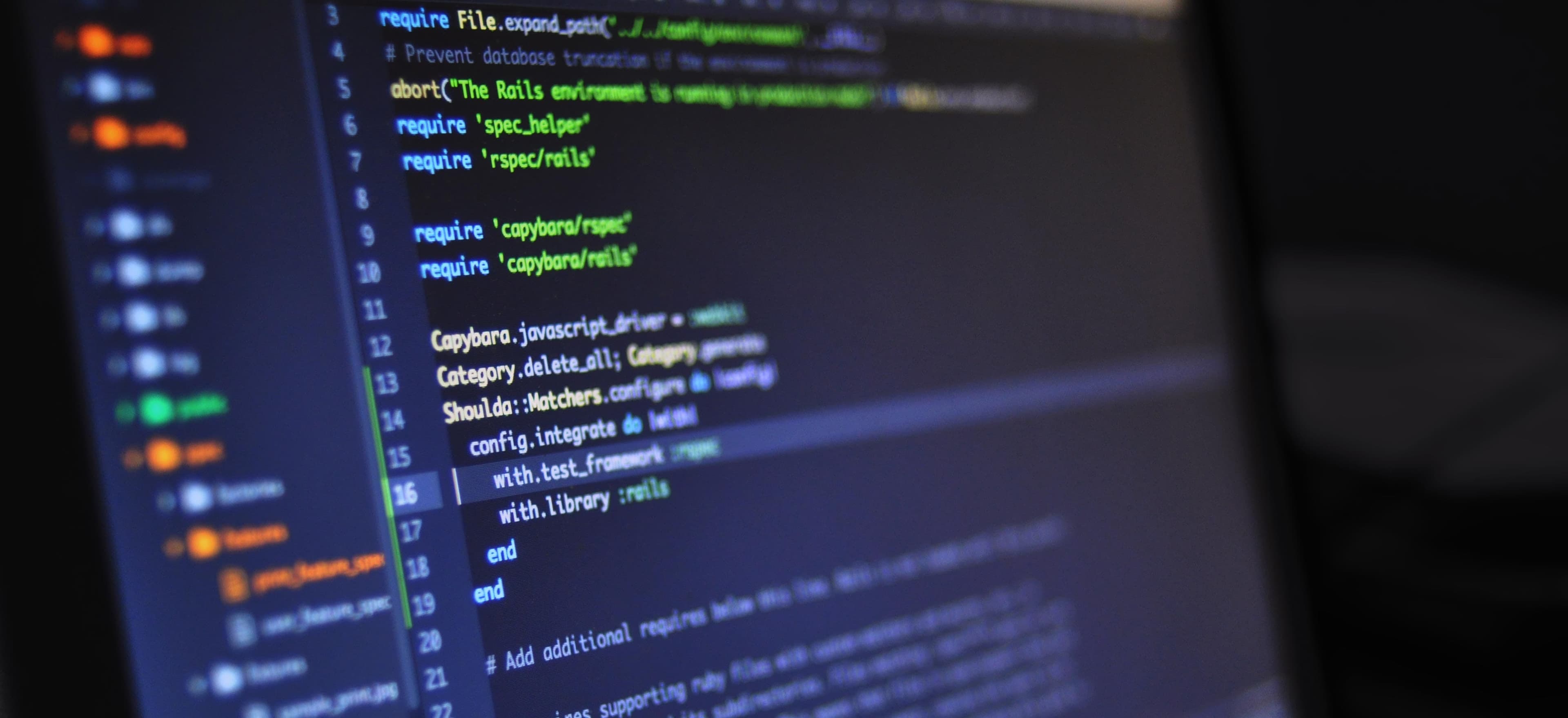
- Published on
Understanding the Pitfalls of Class-Variable Overrides in Java
Java, renowned for its object-oriented programming paradigm, allows developers to create robust applications with reusable code. However, one aspect that can lead to confusion and bugs is the overriding of class variables. In this blog post, we delve into the nuanced behavior of class-variable overrides, exploring common pitfalls, illustrating concepts with code snippets, and providing practical guidance for developers.
What are Class Variables?
In Java, class variables, often referred to as static variables, are shared among all instances of a class. This means that if one instance modifies a class variable, the change is reflected across all instances. It is crucial to comprehend how these variables behave, particularly with inheritance, since it can lead to unexpected results if overridden incorrectly.
Class Variable Example
Here's an illustrative example to clarify the behavior of class variables:
class BaseClass {
static String classVar = "Base Class Value";
void printClassVar() {
System.out.println(classVar);
}
}
class SubClass extends BaseClass {
static String classVar = "Sub Class Value";
}
public class Main {
public static void main(String[] args) {
BaseClass base = new BaseClass();
BaseClass subAsBase = new SubClass();
base.printClassVar(); // Output: Base Class Value
subAsBase.printClassVar(); // Output: Base Class Value
System.out.println(SubClass.classVar); // Output: Sub Class Value
}
}
Why is this Important?
In the example above, we have a BaseClass
with a static variable classVar
. The SubClass
overrides this variable with its own static version. However, when we invoke printClassVar
on subAsBase
, which is of type BaseClass
, it accesses the classVar
of BaseClass
, not SubClass
. This is due to the way static variables work—they belong to the class, not the instance.
The Pitfall of Assumptions
One of the most significant pitfalls developers encounter is assuming that static variables can be overridden like instance variables. This misconception can lead to bugs that can be challenging to pinpoint.
Imagine if, in a business application, the classVar
was supposed to represent some configuration shared across multiple components. If a developer mistakenly believes they have overridden the variable but have actually created a separate static variable, it can lead to unexpected behavior in the application.
Correct Use of Class Variables
To avoid the pitfalls of overriding static variables, adhere to the following best practices:
-
Avoid Shadowing: Instead of creating a static variable in a subclass that uses the same name, consider using instance variables or completely different names.
-
Understand Scope: Remember that the class variable belongs to the class itself. Therefore, be cautious about its scope.
Here’s a revised approach that avoids the pitfalls of shadowing:
class BaseClass {
static String classVar = "Base Class Value";
void printClassVar() {
System.out.println(classVar);
}
}
class SubClass extends BaseClass {
// Instead of shadowing, use a different name
static String subclassVar = "Sub Class Value";
void printSubClassVar() {
System.out.println(subclassVar);
}
}
public class Main {
public static void main(String[] args) {
BaseClass base = new BaseClass();
SubClass sub = new SubClass();
base.printClassVar(); // Output: Base Class Value
sub.printSubClassVar(); // Output: Sub Class Value
}
}
Why This Matters
This revised approach leads to code clarity. Each class variable has its distinct purpose and identity, thus eliminating confusion.
Instance vs Static Variables
To better understand the concept, we should also clarify the difference between instance variables and class variables. Here’s a quick comparison:
- Class Variables (Static): Shared among all instances. Changes made via one instance reflect across all instances.
- Instance Variables: Unique to each instance. Changes made via one instance do not affect other instances.
Example to Illustrate
Here’s a comparison of the two types of variables:
class Example {
static String classVar = "Static Variable";
String instanceVar;
Example(String value) {
this.instanceVar = value;
}
}
public class Main {
public static void main(String[] args) {
Example obj1 = new Example("Instance 1");
Example obj2 = new Example("Instance 2");
System.out.println(Example.classVar); // Output: Static Variable
System.out.println(obj1.instanceVar); // Output: Instance 1
System.out.println(obj2.instanceVar); // Output: Instance 2
Example.classVar = "New Static Value"; // Change class variable
System.out.println(Example.classVar); // Output: New Static Value
}
}
Here, changing classVar
affects all instances, while instanceVar
remains unique to each Example object.
Best Practices for Working with Class Variables
To avoid the pitfalls associated with class-variable overrides, you should incorporate the following strategies into your coding practices:
-
Clear Naming Convention: Use distinct names for class and instance variables to avoid unintentional shadowing.
-
Understanding Inheritance: Familiarize yourself with how static variables are resolved in inheritance hierarchies. Utilize tools like the Java Documentation to clarify uncertainties.
-
Favor Composition Over Inheritance: If a variable's behavior is better expressed through an instance variable, consider composition as a viable alternative.
-
Utilize Comments: Always add comments to explain the purpose of static members and their intended scope, especially in larger teams.
-
Code Reviews: Engage in code reviews to get peer feedback on how class and instance variables are utilized.
For further reading on Java's inheritance model, check out the official Oracle documentation.
A Final Look
In summary, understanding class-variable overrides in Java is crucial for avoiding common pitfalls and writing clear, maintainable code. Class variables belong to the class itself, and overriding them can lead to confusion, particularly in an object-oriented context. By following best practices, using clear naming conventions, and fostering a thorough understanding of the inheritance mechanism, you can mitigate the risks associated with overriding class variables.
Implementing these concepts can save developers from troubleshooting bugs that stem from variable mismanagement, leading to a more efficient development process. Your mastery of these principles ultimately enhances not only your coding skills but also the quality of your software projects. For more insights on Java programming fundamentals, stay tuned to our blog. Happy coding!