Overcoming Procrastination: Java for Lazy Developers
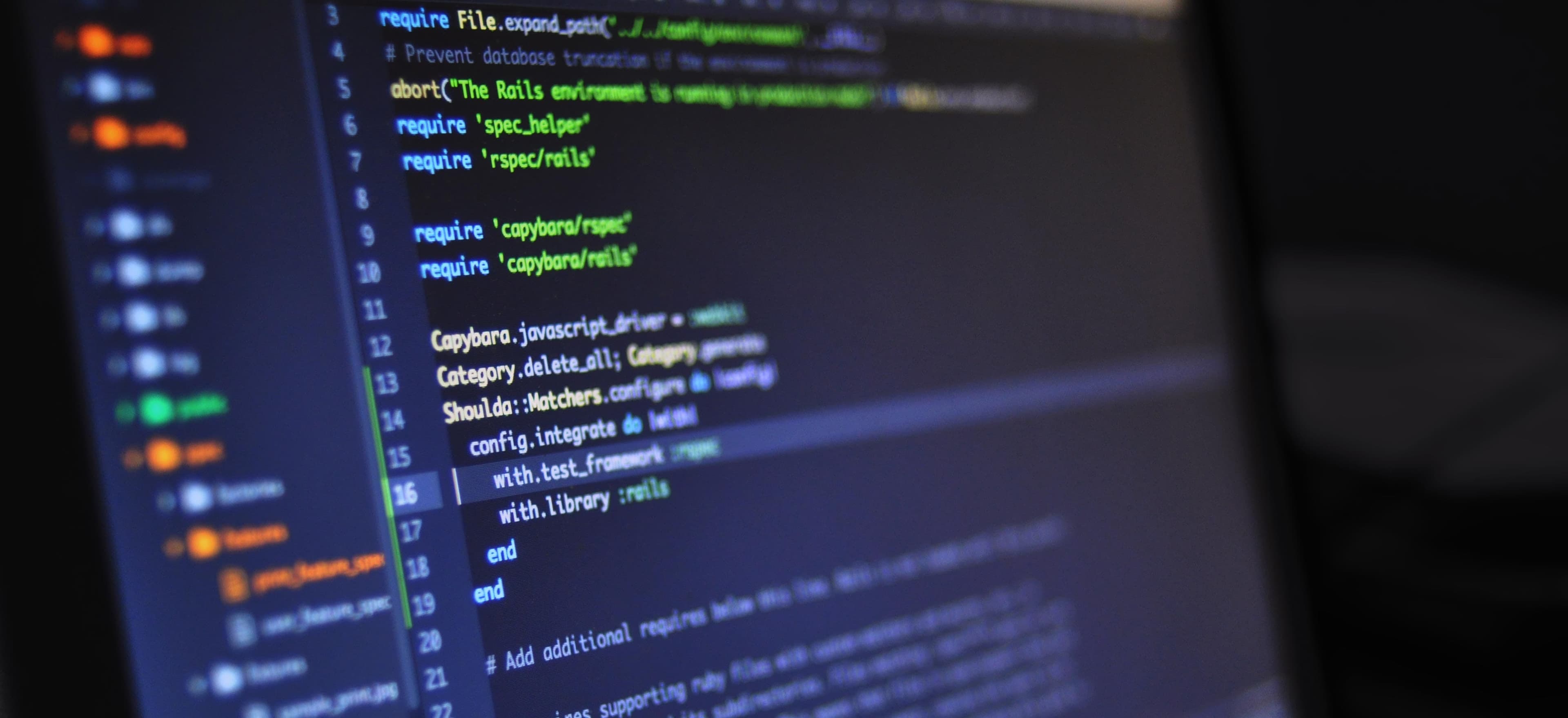
- Published on
Overcoming Procrastination: Java for Lazy Developers
Procrastination can be a significant barrier for many developers. It may stem from various sources, such as fear of failure, lack of motivation, or overwhelming tasks. However, in the world of Java programming, there are strategies and tools that can help even the laziest developers overcome procrastination. This blog post aims to explore these solutions while providing practical Java code examples that demonstrate effective techniques to boost productivity.
Understanding Procrastination
Before diving deep into the Java solutions, it's essential to understand what procrastination is. It’s the act of delaying action or decision, often leading to stress and poor performance. According to research, procrastination often arises from a desire to avoid unpleasant tasks. However, procrastinating can worsen anxiety and reduce efficiency. For a developer, the consequences can be even more pronounced—missed deadlines, bugs slipping through the cracks, and a backlog of work can pile up.
Why Java?
Java has long been a popular programming language for a variety of applications—from web development to mobile applications. Its versatility allows developers to focus on designing efficient solutions without being bogged down by unnecessary complexity. Additionally, Java's extensive libraries and frameworks provide tools that can help streamline tasks and automate processes, making it an excellent choice for those struggling with procrastination.
Strategies to Tackle Procrastination
1. Break Down Tasks
One of the most effective strategies for overcoming procrastination is to break down larger tasks into manageable pieces. This technique reduces the overwhelming feeling that often accompanies large projects.
Here’s a simple Java program to help exemplify this approach:
import java.util.ArrayList;
import java.util.List;
public class TaskManager {
private List<String> tasks;
public TaskManager() {
tasks = new ArrayList<>();
}
public void addTask(String task) {
tasks.add(task);
}
public void printTasks() {
System.out.println("Your Tasks:");
for (int i = 0; i < tasks.size(); i++) {
System.out.println((i + 1) + ". " + tasks.get(i));
}
}
public static void main(String[] args) {
TaskManager taskManager = new TaskManager();
taskManager.addTask("Implement user authentication");
taskManager.addTask("Write unit tests for authentication");
taskManager.addTask("Document the authentication process");
taskManager.printTasks();
}
}
Why This Matters:
This TaskManager
class allows developers to add tasks easily and print them out. By interacting with this simple application, you can visualize your programmatic endeavors, making it easier to prioritize and complete your tasks.
2. Automated Reminders
Automating reminders is another effective way to keep procrastination at bay. You can use Java's scheduling mechanism to remind you of upcoming tasks or deadlines.
Here’s a basic example using java.util.Timer
:
import java.util.Timer;
import java.util.TimerTask;
public class Reminder {
private Timer timer;
public Reminder(int seconds) {
timer = new Timer();
timer.schedule(new RemindTask(), seconds * 1000);
}
class RemindTask extends TimerTask {
public void run() {
System.out.println("Time to get back to work!");
timer.cancel(); // Terminate the Timer thread
}
}
public static void main(String[] args) {
System.out.println("You have set a timer for 5 seconds.");
new Reminder(5);
}
}
Why This Matters:
In this Reminder
class, a timer notifies you to refocus on your work after a set number of seconds. This simple yet powerful reminder can break a cycle of distraction and encourage you to tackle those tasks you've been neglecting.
3. Utilize Libraries and Frameworks
Java offers numerous libraries and frameworks that can significantly reduce the workload for developers. Utilizing these can boost your productivity and minimize the hours spent on tedious tasks.
For example, using the Spring Framework can help streamline complex application development. Check out the Spring Boot documentation for a comprehensive guide.
Here’s a quick way to initialize a simple web application using Spring Boot:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
System.out.println("Application started successfully!");
}
}
Why This Matters:
In this code, we can see the power of Spring Boot—developers can get a web application up and running with a few lines of code, eliminating unnecessary boilerplate code and letting you focus on the core logic of your application.
4. Code Reviews and Pair Programming
Engaging with peers through code reviews or pair programming sessions can help alleviate procrastination-related issues. Having someone to share the workload and provide constructive criticism can be motivating.
Here’s how you can simulate a basic code review session in Java:
import java.util.ArrayList;
import java.util.List;
class CodeReview {
private List<String> reviewers;
public CodeReview() {
reviewers = new ArrayList<>();
}
public void addReviewer(String reviewer) {
reviewers.add(reviewer);
}
public void printReviewers() {
System.out.println("Reviewers for this code:");
for (String reviewer : reviewers) {
System.out.println("- " + reviewer);
}
}
public static void main(String[] args) {
CodeReview codeReview = new CodeReview();
codeReview.addReviewer("Alice");
codeReview.addReviewer("Bob");
codeReview.printReviewers();
}
}
Why This Matters:
By using the CodeReview
class, a developer can easily keep track of who will be reviewing their code, thus enhancing accountability. Such engagements can sidestep procrastination since deadlines become more potent when others are involved.
5. Time Management Techniques
Time management techniques—such as the Pomodoro technique—can increase focus and reduce procrastination. Developers can write a small Java application to track work sessions and breaks.
import java.util.Timer;
import java.util.TimerTask;
public class Pomodoro {
private Timer timer;
public void startPomodoro(int workMinutes, int breakMinutes) {
// Work Session
System.out.println("Work session started for " + workMinutes + " minutes.");
timer = new Timer();
timer.schedule(new TimerTask() {
public void run() {
System.out.println("Time for a break!");
// Break Session
startBreak(breakMinutes);
timer.cancel();
}
}, workMinutes * 60000); // convert minutes to ms
}
private void startBreak(int breakMinutes) {
System.out.println("Break session started for " + breakMinutes + " minutes.");
timer = new Timer();
timer.schedule(new TimerTask() {
public void run() {
System.out.println("Break is over! Get back to work!");
timer.cancel();
}
}, breakMinutes * 60000);
}
public static void main(String[] args) {
Pomodoro pomodoro = new Pomodoro();
pomodoro.startPomodoro(25, 5); // 25 mins work, 5 mins break
}
}
Why This Matters:
The Pomodoro
class is an example of how you can manage your time effectively. By structuring your work in focused bursts, you enhance efficiency and minimize procrastination.
Final Thoughts
Procrastination can be a hurdle in any developer's journey, but Java provides a plethora of tools and methodologies for overcoming this challenge. By breaking tasks down into manageable pieces, automating reminders, utilizing frameworks, engaging in code reviews, and practicing effective time management techniques, you can significantly enhance your productivity.
Remember: the key is to act—sometimes the hardest part is just getting started. So fire up your IDE, implement the discussed strategies, and watch your productivity soar. After all, the best time to start overcoming procrastination is right now.
For more insights on Java programming, feel free to explore Oracle's Java Tutorials and discover diverse resources that can elevate your coding skills. Happy coding!
Checkout our other articles