Common Pitfalls When Using Google Gson for JSON Parsing
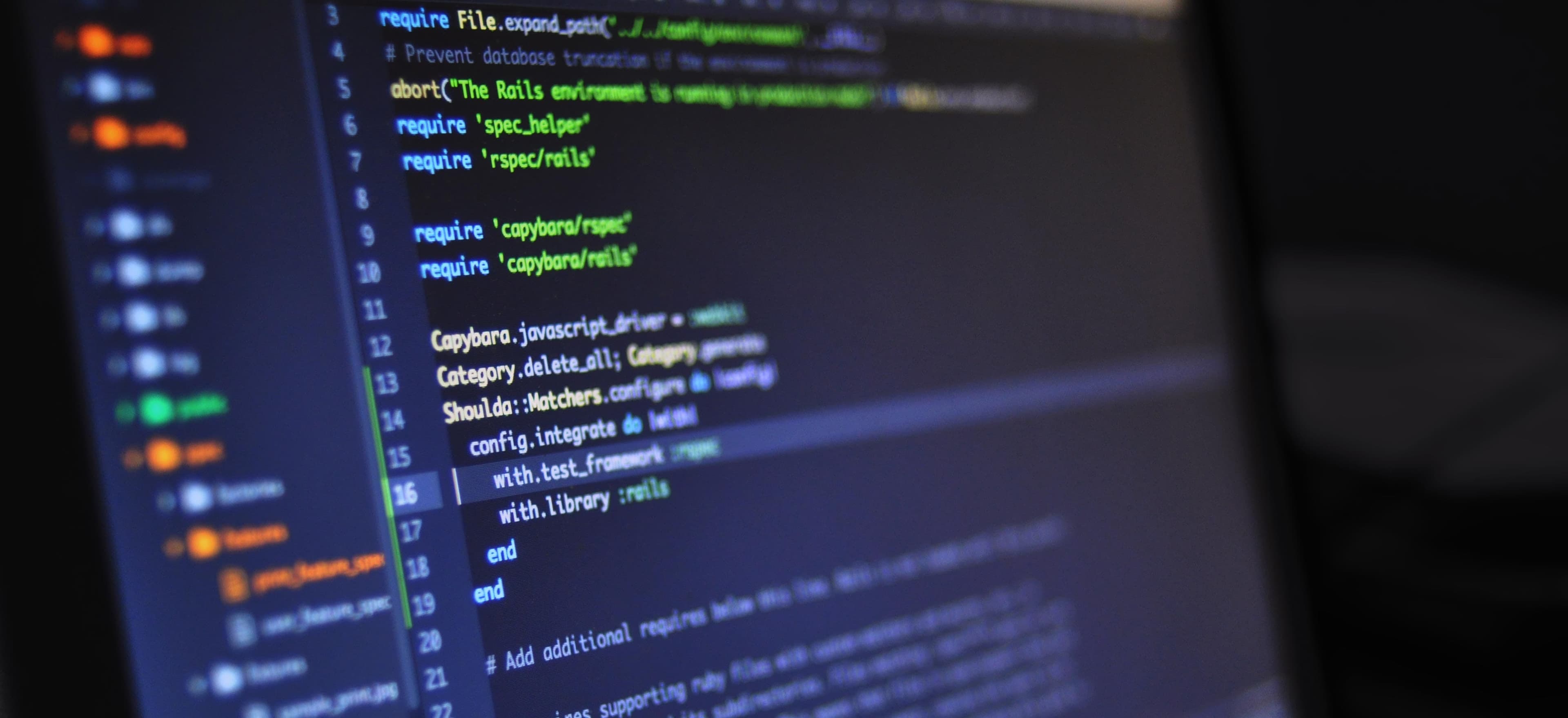
- Published on
Common Pitfalls When Using Google Gson for JSON Parsing
Parsing JSON in Java has become an essential skill for many developers, especially with the increasing reliance on APIs that communicate through this lightweight data interchange format. Google Gson is a powerful library that simplifies the process of converting Java objects to JSON and vice versa. However, like any powerful tool, it has its intricacies.
In this blog post, we will explore common pitfalls when using Google Gson for JSON parsing, provide insight into their significance, and furnish practical solutions to avoid them.
Understanding Google Gson
Google Gson is a popular library that allows developers to convert Java objects to JSON and parse JSON back into Java objects. It is simple, efficient, and widely used in modern Java-based applications.
Why Use Google Gson?
- Ease of Use: It requires minimal setup, allowing quick integration into projects.
- Flexibility: It can handle a variety of Java types, including collections and complex nested objects.
- Performance: Gson is optimized for performance, making it a suitable choice for large datasets.
Common Pitfalls
1. Ignoring Field Naming Conventions
One of the first obstacles developers encounter is the mismatch between Java field names and JSON key names. By default, Gson uses Java field names directly as JSON key names.
Example Code
public class User {
private String firstName;
private String lastName;
// Getters and Setters...
}
String json = "{\"firstName\": \"John\", \"lastName\": \"Doe\"}";
// Parsing
User user = new Gson().fromJson(json, User.class);
Why This Matters
If your JSON keys do not match the field names in your Java class (e.g., first_name
vs. firstName
), you'll end up with a user object with null fields.
Solution
To resolve this, you can use Gson's @SerializedName
annotation to specify the JSON key names.
public class User {
@SerializedName("first_name")
private String firstName;
@SerializedName("last_name")
private String lastName;
// Getters and Setters...
}
2. Misprinting Collection Types
When dealing with collections (like Lists or Maps), developers often misunderstand how to declare them, leading to runtime exceptions or unexpected results.
Example Code
public class User {
private List<String> roles;
// Getters and Setters...
}
// Incorrectly parsing
String json = "{\"roles\": \"admin\"}";
User user = new Gson().fromJson(json, User.class); // Throws JsonSyntaxException
Why This Matters
In this scenario, the roles
field in JSON is expected to be an array, but a string was provided instead. Deviations from expected data types can lead to exceptions in parsing.
Solution
Always ensure that your JSON structure matches the data types you’ve defined in your classes. The correct JSON input for the above case would be:
{"roles": ["admin", "user"]}
3. Failing to Handle Null Values
Another common pitfall is the assumption that Gson will handle null values gracefully. While Gson does parse nulls, the outcomes can sometimes lead to confusion.
Example Code
String json = "{\"firstName\": null, \"lastName\": \"Doe\"}";
User user = new Gson().fromJson(json, User.class);
// firstName will be set to null
Why This Matters
Sometimes developers expect null
fields to be excluded, which would create a discrepancy in the expected output.
Solution
To manage this, it may be beneficial to implement custom deserializers to handle default values or to check for nulls in your application logic.
4. Not Using a Custom Date Format
Java has several date formats, but Gson lacks a default mechanism for converting between them and JSON strings. If you inherit this behavior, your date fields may become problematic.
Example Code
public class Event {
private Date eventDate;
// Getters and Setters...
}
// Default deserialization may fail
String json = "{\"eventDate\": \"2023-12-31\"}";
Event event = new Gson().fromJson(json, Event.class); // Returns null
Why This Matters
Using the incorrect date format during deserialization may lead to null
values or runtime exceptions due to incompatible formats.
Solution
Utilize GsonBuilder()
to set a custom date format for proper serialization and deserialization.
Gson gson = new GsonBuilder()
.setDateFormat("yyyy-MM-dd")
.create();
Event event = gson.fromJson(json, Event.class);
5. Neglecting Error Handling
When parsing JSON, it's crucial to anticipate and handle potential parsing errors. Ignoring this can lead to unhandled exceptions and application crashes.
Example Code
String json = "{\"firstName\": \"John\"}"; // Correct JSON
User user = new Gson().fromJson(json, User.class);
However, if the JSON structure changes or contains errors, the above parsing could fail, resulting in an application throwing a runtime exception.
Why This Matters
Failing to implement error handling can spiral into the user experience suffering due to abrupt application failures.
Solution
Wrap your parsing logic within a try-catch block, allowing you to intercept errors and respond appropriately.
try {
User user = new Gson().fromJson(json, User.class);
} catch (JsonSyntaxException e) {
e.printStackTrace(); // Handling the error
}
Closing Remarks
While Google Gson is an adept tool for JSON parsing in Java, comprehension and adherence to best practices are necessary to leverage its full potential. Overlooking common pitfalls like field naming conventions, collection types, null values, custom date formats, and error handling can lead to frustrating debugging sessions.
By understanding these challenges and applying the outlined solutions, developers can create robust applications that efficiently manage JSON data.
For further information on Gson, visit Gson User Guide and consider exploring JSON and Java for a deeper dive into practical applications.
Arming yourself with this knowledge not only aids in writing better code but also contributes to more reliable and maintainable applications. Happy coding!