When Convention Over Configuration Leads to Inflexibility
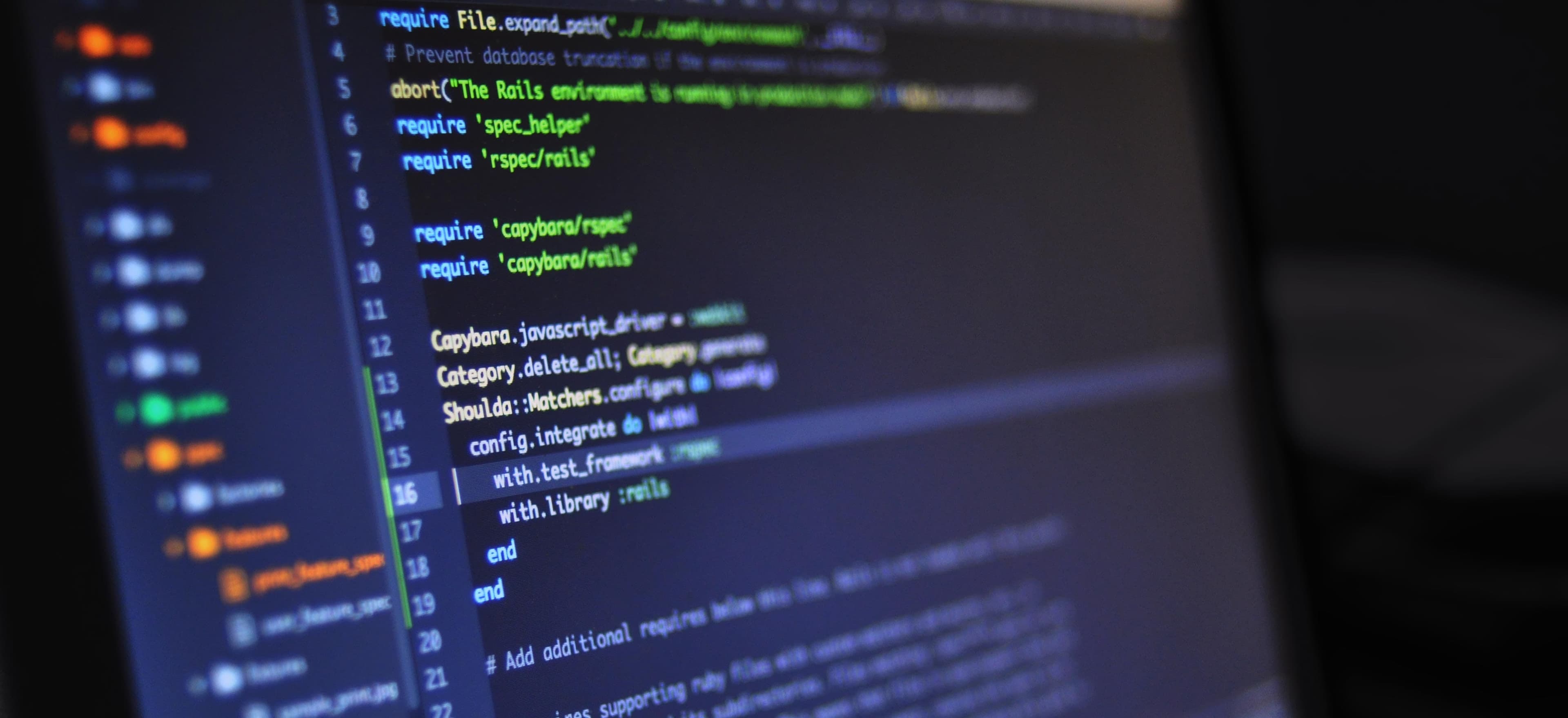
- Published on
When Convention Over Configuration Leads to Inflexibility in Java Development
In the world of software development, how we design our applications can either empower or hinder our productivity. One popular philosophy, particularly in frameworks like Ruby on Rails and, though more indirectly, Java-based frameworks such as Spring, is the concept of Convention Over Configuration. While this approach has many bona fide benefits, it can sometimes lead to inflexibility. This blog post aims to dissect the implications of this principle in Java development and provide some practical insights.
Understanding Convention Over Configuration
Convention Over Configuration (CoC) is a design paradigm aimed at increasing productivity by reducing the number of settings that developers need to specify to get an application up and running. This principle suggests that software should have sensible defaults, which allows developers to focus on writing application features instead of configuration code.
For Java developers, frameworks like Spring Boot exemplify this principle. With Spring Boot, you can bootstrap applications quickly by following conventions rather than writing extensive configuration files.
Advantages of Convention Over Configuration
-
Reduced Boilerplate: CoC minimizes boilerplate code, making it easier to start a project and write the code that truly matters.
-
Faster Development: Default behaviors, such as automatic dependency injection, allow developers to focus on business logic rather than infrastructure.
-
Ease of Use: New developers can ramp up quickly without needing to learn intricate configuration procedures.
Example: Spring Boot Configuration
Here is an example of a Spring Boot application that illustrates how Convention Over Configuration streamlines project setup:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
This minimal setup allows you to get your application running with just a few lines of code. By annotating the MyApplication
class with @SpringBootApplication
, you instruct Spring to configure many aspects of your application automatically.
Why This Matters
In this example, the beauty lies in not having to create XML configuration files or extensive setup classes. The convention is powerful because it leverages opinions of the framework, which are usually well thought out from real-world experiences. This is advantageous when you have the consensus of best practices baked into the framework itself.
The Downside: Inflexibility in Certain Situations
Despite its merits, there are situations where Convention Over Configuration can lead to inflexibility. This inflexibility can manifest in several ways, and understanding them is crucial for designing maintainable and scalable applications.
1. Over-Designed Framework Opinions
CoC typically relies on "sensible defaults" built into the framework. However, these defaults may not match the unique requirements of every application. When developers encounter situations where the default behavior doesn't align with their needs, they often find themselves wrestling against the framework.
Case Study: Database Configuration in Spring Boot
Consider a scenario where you need to configure a database connection that isn’t supported by Spring Boot’s default settings. If you’re working with a less common database or your database conventions diverge significantly from Spring's expectations, you may end up with extensive configurations.
spring:
datasource:
url: jdbc:yourdatabase://localhost:5432/mydb
username: myuser
password: mypass
The default behavior assumes certain conventions aligned with popular databases like MySQL or PostgreSQL. If you're using an unconventional database, Spring may not provide the flexibility required, leading to complex and cumbersome configurations.
2. Hurdles When Switching Contexts
Switching from one framework to another may become increasingly difficult when the chosen framework relies heavily on conventions. In tightly coupled systems, losing that implicit knowledge can slow down migration and development.
For instance, if you’re deep into a Spring Boot application that heavily leverages its conventions, migrating to a more unopinionated framework or switching to a microservices architecture requires rethinking many canonical approaches you've come to depend on.
3. Loss of Control and Transparency
By defaulting to conventions, the underlying mechanisms of the framework may become obscured. Developers find themselves using features without understanding how they work, leading to difficulties in debugging and enhancing applications. The hands-off approach might overlook particular performance optimizations needed for specific use cases.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByEmail(String email);
}
Using Spring Data, you can attain the functionality with minimal code. However, if performance becomes an issue, you may need to look under the hood and construct custom queries—a process that goes against the streamlined initial setup.
Striking a Balance
While CoC provides a powerful alternative to extensive configuration, it’s crucial to strike a balance between convention and flexibility. Here are some actionable strategies for achieving this:
1. Utilize Configuration Overrides
When you run into a situation where default settings aren't quite right, don't hesitate to override them. Most frameworks offer the ability to configure components manually when necessary. In Spring Boot, you can override auto-configurations via properties or by defining your own beans.
2. Stay Informed of Framework Updates
Frameworks are continually evolving. New features that increase flexibility are regularly introduced. Keep an eye on official documentation and community updates. Being aware of these changes can help you leverage tools that might better meet your application's needs.
3. Build with Composition
Creating small, composable components that can work together allows you to circumvent the rigidity of convention-based designs. Instead of relying entirely on framework defaults, construct your features in a modular way that allows for independent evolution and testing.
Example of a Composable Component
Consider defining a custom service that encapsulates business logic:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserByEmail(String email) {
return userRepository.findByEmail(email)
.orElseThrow(() -> new UserNotFoundException(email));
}
}
With this approach, if your requirements change—like needing to integrate with an external user system—you can refactor and update the UserService
without overhauling everything in your application.
My Closing Thoughts on the Matter
Convention Over Configuration can greatly enhance productivity and simplify initial project setup for Java applications, especially when relying on frameworks like Spring Boot. However, it's vital to recognize its limitations. Understanding when to apply conventions and when to exercise flexibility is key to creating robust, maintainable code.
Through thoughtful design and awareness of the complexities at play, Java developers can harness the power of both conventions and configurations. Balancing these approaches leads to applications that are not only quick to develop but can seamlessly adapt to emergent requirements.
For further reading on Spring Boot and its conventions, you might find the official Spring Boot Documentation useful. This will provide deeper insights and further examples to enrich your understanding and application of these principles in your own projects.