Understanding Function Chaining vs. Function Composition
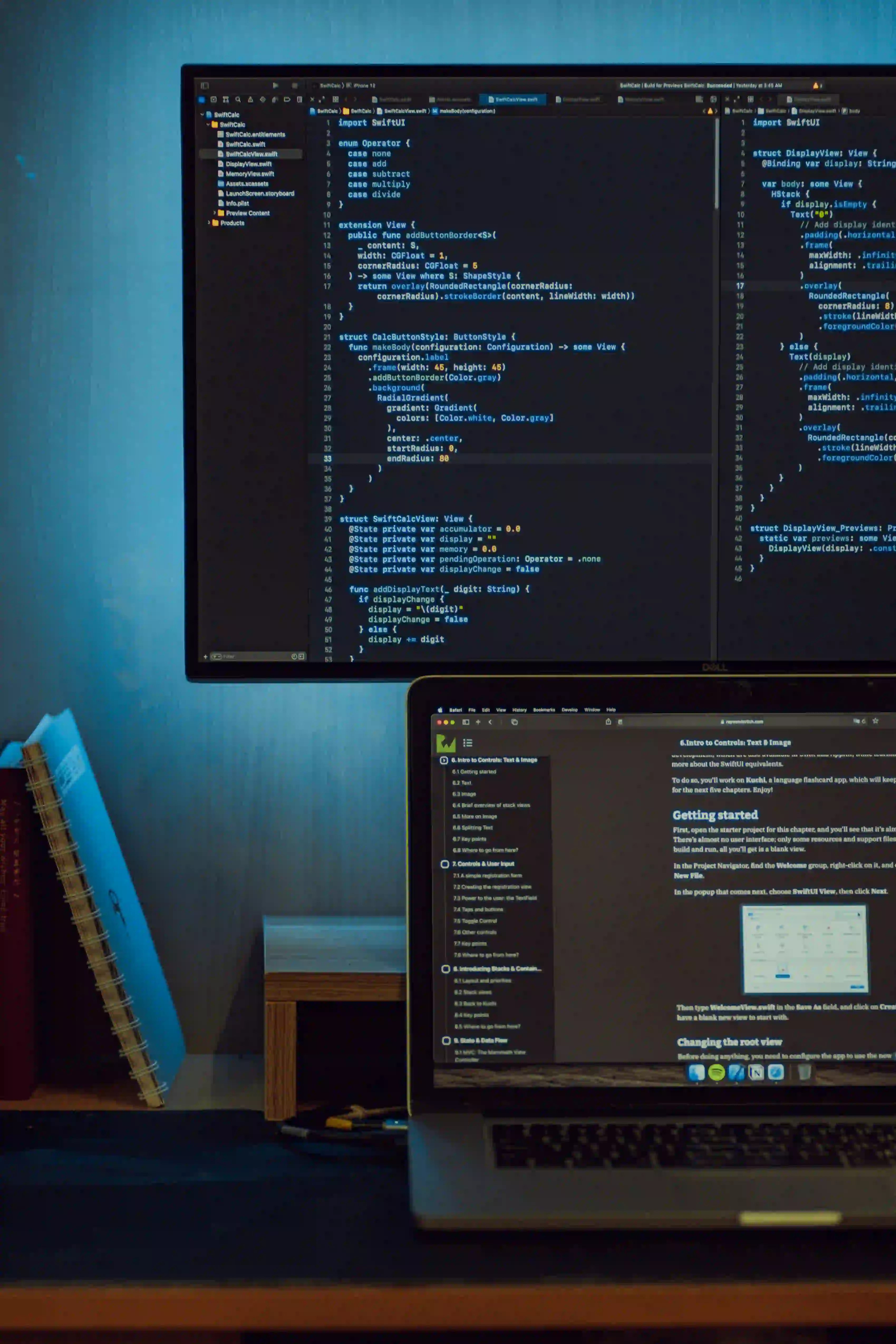
Understanding Function Chaining vs. Function Composition
In programming, the way we structure our code can significantly affect its readability and maintainability. Java, with its object-oriented features, offers developers multiple paradigms to enhance code organization and flow. Two popular concepts in Java are function chaining and function composition. While they may seem similar, they have distinct characteristics and applications.
In this blog post, we will delve into function chaining and function composition, identifying their definitions, differences, advantages, and use cases. Along the way, we'll look at code snippets and discuss the 'why' behind each implementation.
What is Function Chaining?
Function chaining, also known as method chaining, is a technique that allows multiple method calls on the same object to be chained together in a single statement. This is made possible by having each method return a reference to the current object (often referred to as this
).
Why Use Function Chaining?
- Conciseness: It reduces code verbosity.
- Readability: Related actions can be grouped together.
- Fluency: It enhances the sense of a streamlined flow.
Example of Function Chaining in Java
class StringBuilderExample {
private StringBuilder value;
public StringBuilderExample() {
value = new StringBuilder();
}
public StringBuilderExample append(String str) {
value.append(str);
return this; // Returning current instance for chaining
}
public StringBuilderExample capitalize() {
String str = value.toString();
value.setLength(0); // Clear current value
value.append(str.substring(0, 1).toUpperCase()).append(str.substring(1));
return this; // Returning current instance for chaining
}
public String build() {
return value.toString();
}
}
public class FunctionChainingDemo {
public static void main(String[] args) {
String result = new StringBuilderExample()
.append("hello ")
.capitalize()
.append("world")
.build();
System.out.println(result); // Outputs: Hello world
}
}
In the above example, StringBuilderExample
allows methods such as append
and capitalize
to be called in a chain. The key takeaway here is the return this;
statement, which is crucial for allowing the chaining of methods. By returning the current instance, we maintain context.
What is Function Composition?
Function composition involves combining two or more functions to produce a new function. In Java, this is frequently achieved through functional interfaces such as Function<T, R>
. Each function takes input and outputs a new result that can be passed to another function.
Why Use Function Composition?
- Decoupling: It promotes separation of concerns and reusability.
- Flexibility: You can easily change or swap out functions without modifying the composition logic.
- Clarity: It abstracts away low-level details.
Example of Function Composition in Java
import java.util.function.Function;
public class FunctionCompositionDemo {
public static void main(String[] args) {
Function<String, String> addHello = str -> "Hello, " + str;
Function<String, String> addWorld = str -> str + " world";
// Composing the functions
Function<String, String> helloWorld = addHello.andThen(addWorld);
String result = helloWorld.apply("Java");
System.out.println(result); // Outputs: Hello, Java world
}
}
In this example, we defined two functions: addHello
and addWorld
. The andThen
method is used to compose these functions. The resulting function can then be applied to produce a coherent output. As demonstrated, function composition provides a high level of modularity.
Key Differences Between Function Chaining and Function Composition
| Aspect | Function Chaining | Function Composition | |-----------------------|---------------------------------------------------------|---------------------------------------------------------| | Mechanism | Chaining method calls on the same object | Combining multiple functions to create a new function | | Focus | Enhances readability and fluency of method calls | Promotes reusability and separation of logic | | Return Value | Returns the same object for chaining | Returns a new function | | Use Case | Typically in constructors and builders | Often in functional programming and functional pipelines |
Advantages and Disadvantages
Advantages of Function Chaining
- Simplicity: Easy to follow flow of operations.
- Reduced Boilerplate: Fewer temporary variables needed.
Disadvantages of Function Chaining
- Complexity with Deep Chains: Can become hard to read with extensive chains.
- State Management: Can lead to mismanagement of the object's state.
Advantages of Function Composition
- Reusable Logic: Functions can be reused across different contexts.
- Testability: Smaller functions are easier to test individually.
Disadvantages of Function Composition
- Overhead: Can introduce performance overhead due to multiple function calls.
- Learning Curve: Understanding functional programming concepts may not be straightforward for all developers.
When to Use Function Chaining vs. Function Composition
-
Use Function Chaining when working with method-heavy APIs, such as builders or where readability in object state transformation is key.
-
Use Function Composition when working on functional programming paradigms, particularly for data transformations, where each function encapsulates a specific piece of logic.
Lessons Learned
Both function chaining and function composition are valuable techniques in Java that enhance code quality and structure. Depending on the context and requirements, each approach offers distinct advantages.
Understanding these paradigms allows developers to choose the right approach for their particular use cases, leading to clearer, more maintainable code. By mastering these concepts, you'll be better equipped to write elegant Java applications.
For further reading, you can check out Java's Functional Interfaces to learn more about function composition. If you're interested in learning about builder patterns, consider exploring the Builder Pattern.
Feel free to implement these patterns in your project. Happy coding!