Unlocking Java Performance: The Biggest Tuning Mistake to Avoid
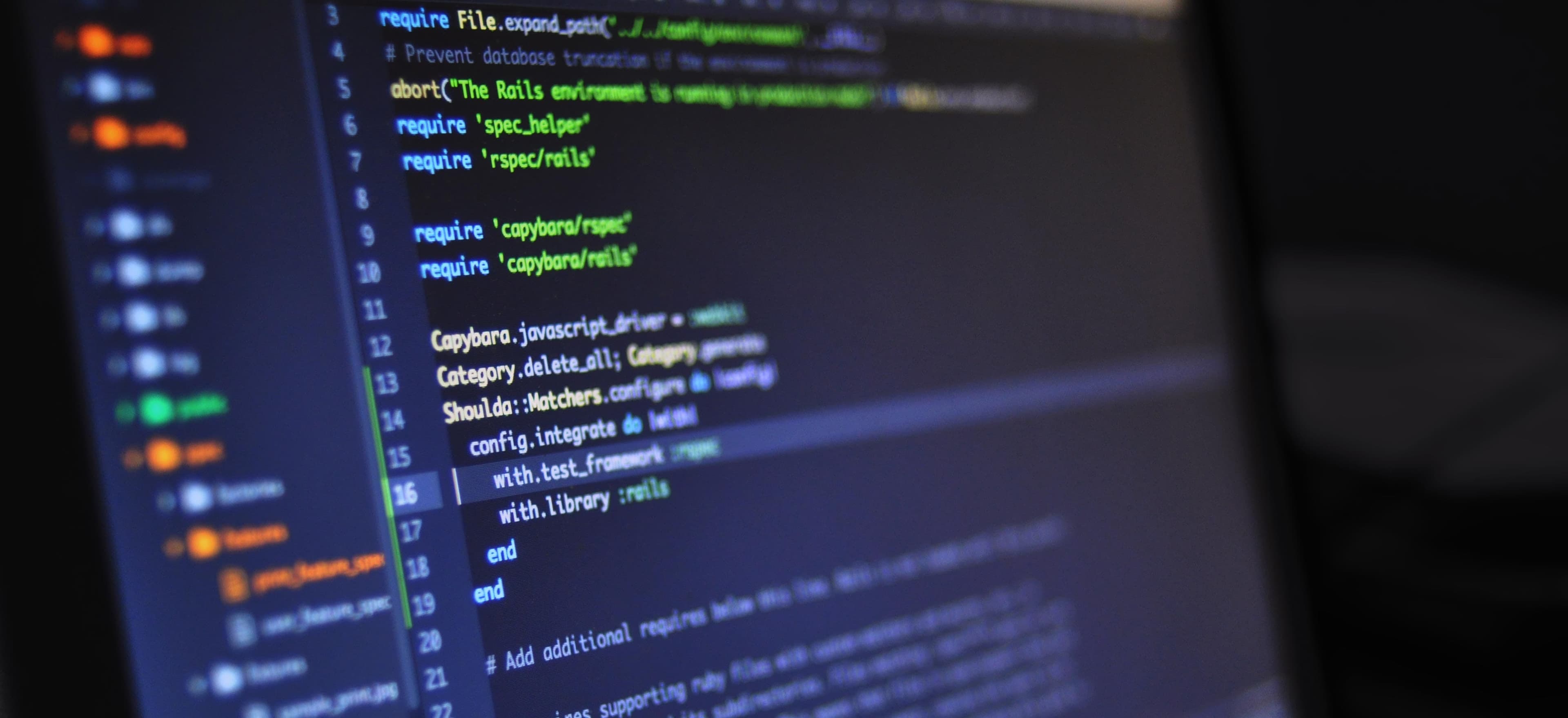
- Published on
Unlocking Java Performance: The Biggest Tuning Mistake to Avoid
Java is a robust programming language widely used for building scalable applications. Yet, as with any technology, achieving peak performance can be a daunting challenge. In this blog post, we will explore common performance issues and reveal the most significant tuning mistake developers make: premature optimization. By the end, you will have practical insights into Java performance tuning and how to avoid this pitfall.
Understanding Java Performance
Java applications are often perceived as slower than those written in languages like C or C++. However, Java's performance is not inherently poor. It is a misconception fueled by a misunderstanding of the Java Virtual Machine (JVM) and its garbage collection (GC) processes.
The JVM acts as an intermediate layer between Java applications and underlying hardware, providing features like Just-In-Time (JIT) compilation, memory management, and garbage collection. Hence, before diving into performance tuning, it is crucial to understand how the JVM operates.
Common Performance Pitfalls
Before we delve into the biggest tuning mistake to avoid, let's discuss some common performance pitfalls:
-
Inefficient Algorithms: Choosing the wrong algorithm can severely impact performance. Always analyze the algorithm you use and look for more efficient alternatives if needed.
-
Excessive Object Creation: Frequent creation and disposal of objects can lead to higher GC overhead, which can impact application responsiveness.
-
Ignoring Caching: Not using caches for frequently accessed data can slow down your application. Caching optimized data can save processing time.
-
Ineffective Multithreading: Improper use of threads can either overload the CPU or lead to contention for resources. Proper management of multithreading is essential for optimal performance.
While it’s essential to recognize these pitfalls, the most crucial mistake is still ahead.
The Tuning Mistake: Premature Optimization
The principle of "premature optimization" refers to making performance tweaks to code before truly understanding the bottlenecks. The phrase, attributed to Donald Knuth, states, "premature optimization is the root of all evil." Here’s why it matters in the context of Java performance tuning:
-
Misallocation of Resources: Spending time on areas of the code that do not significantly impact performance can lead to wasted resources and effort.
-
Increased Complexity: Tweaking code for performance can lead to increased complexity, making it harder to maintain and understand.
-
Hindering Future Changes: Optimizing too early can create tight coupling in your code, making future changes difficult and counterproductive.
Identifying Real Bottlenecks
Before jumping into any optimizations, profiling your application is essential. Profiling tools can help identify methods that consume excessive time or memory.
Example of Profiling Code with Java Mission Control
import java.util.Random;
public class PerformanceProfiler {
public static void main(String[] args) {
Random random = new Random();
long startTime = System.nanoTime();
// Simulate a heavy processing task
for (int i = 0; i < 100000; i++) {
random.nextInt();
}
long duration = System.nanoTime() - startTime;
System.out.println("Task completed in " + duration + " nanoseconds.");
}
}
In this example, we are simulating a heavy processing task to measure its execution time. The System.nanoTime()
method helps us get fine-grained timing, which is crucial for performance analysis.
Once you’ve analyzed the execution time and found your bottlenecks, you can focus on those areas that need attention.
Implementing Strategic Optimizations
Once you identify the real knowledge bottlenecks, it is time for targeted optimizations. Below are some strategies that can uplift your Java application's performance.
1. Optimize Garbage Collection
Understanding how garbage collection works in Java can significantly impact performance. Using the G1 Garbage Collector is often advisable for applications with large heaps.
You can enable G1 GC with the following parameters:
java -XX:+UseG1GC -Xmx4g -Xms4g -jar YourApp.jar
This setup allocates 4GB of memory for your application and uses the G1 Garbage Collector, which efficiently manages heap memory.
2. Use StringBuilder for Concatenation
String concatenation with the +
operator in loops can lead to performance degradation due to the creation of intermediate String objects. Instead, utilize StringBuilder
.
Here’s a simple implementation:
public class StringConcatenation {
public static void main(String[] args) {
StringBuilder builder = new StringBuilder();
for (int i = 0; i < 1000; i++) {
builder.append("Number: ").append(i).append("\n");
}
String result = builder.toString();
System.out.println(result);
}
}
By using StringBuilder
, you reduce the overhead of object creation and improve memory utilization.
3. Use Efficient Collections
Choosing the right collection can reduce time complexity and optimize performance. For example, using ArrayList
over LinkedList
is often more efficient for random access operations due to better cache locality.
import java.util.ArrayList;
import java.util.List;
public class CollectionExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
for (int i = 0; i < 1000000; i++) {
list.add("Item " + i);
}
String element = list.get(999999);
System.out.println("Last element was: " + element);
}
}
4. Parallel Processing with Streams
Java 8 introduced the Stream API, allowing for parallel processing of collections. Utilize parallel streams to take advantage of multi-core processors.
import java.util.ArrayList;
import java.util.List;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
for (int i = 0; i < 1_000_000; i++) {
numbers.add(i);
}
long count = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.count();
System.out.println("Count of even numbers: " + count);
}
}
Using parallelStream
in this example can lead to performance improvements, especially with large datasets.
The Bottom Line
Performance tuning in Java requires a methodical approach. Understanding the JVM and implementing optimizations only after identifying bottlenecks is crucial. Remember, premature optimization can lead to complex code and wasted resources.
Instead, focus on measuring, analyzing, and optimizing based on solid evidence. With the strategies outlined in this post, you should be better equipped to enhance Java application performance without falling into the trap of early optimization.
For more information on performance tuning in Java, consider checking out the Oracle Java Performance Tuning Guide.
Happy coding!
Checkout our other articles