Mastering Smooth Sprite Animation in JavaFX
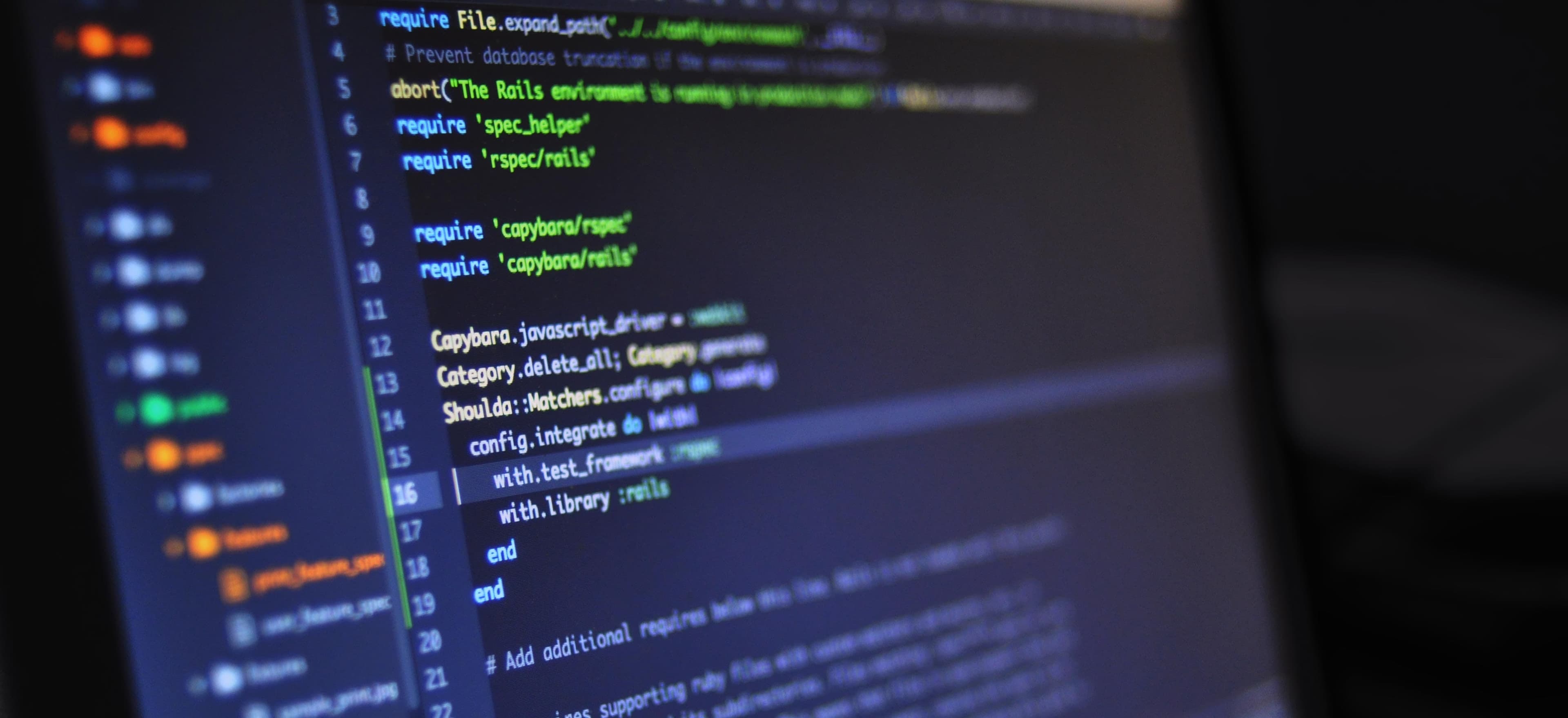
- Published on
Mastering Smooth Sprite Animation in JavaFX
Sprite animation is a vital component of game development, allowing for dynamic and visually appealing movement within an application. In this blog post, we'll dive deep into the world of sprite animation using JavaFX, one of the most popular frameworks for building rich user interfaces in Java. We’ll cover the concept of sprite sheets, how to implement sprite animation efficiently, and provide some exemplary code snippets to illustrate key concepts.
What are Sprites?
Sprites are two-dimensional images or animations integrated into a larger scene, commonly used in video games. Think of a cartoon character walking across the screen; that character is often a sprite animated through a series of images (frames).
Understanding Sprite Sheets
A sprite sheet is a large image containing several small images or frames representing different states or actions (e.g., walking, jumping, attacking). Using sprite sheets helps minimize the number of file loads - the system loads one image instead of many individual frames, enhancing performance.
!Sprite Sheet Example
Example of a sprite sheet displaying various character movements
Setting Up JavaFX
Before we dive into coding, ensure you have JavaFX set up in your development environment. Here’s a quick guide:
1. Installing JavaFX
- Download the latest version of JavaFX SDK from Gluon.
- Unzip the folder and note the path to the
lib
directory. - Make sure your IDE supports JavaFX. This guide assumes you’re using IntelliJ IDEA, but the principles hold for other IDEs.
2. Configuring Your Project
You'll need to configure your project to include JavaFX libraries. In IntelliJ IDEA, you can do this by:
- Going to File > Project Structure.
- Under Libraries, click the '+' button, and select 'Java'.
- Navigate to the
lib
directory of your JavaFX SDK and select all the.jar
files.
Implementing Sprite Animation
Now we can get into creating smooth sprite animations. The example below demonstrates setting up a simple sprite animation using a sprite sheet.
Code Snippet: Basic Animation Setup
import javafx.animation.Animation;
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
import javafx.util.Duration;
public class SpriteAnimationExample extends Application {
private static final int SPRITE_COUNT = 4; // Number of frames in the sprite sheet
private static final int FRAME_WIDTH = 64; // Width of each frame
private static final int FRAME_HEIGHT = 64; // Height of each frame
@Override
public void start(Stage primaryStage) {
Image spriteSheet = new Image("spritesheet.png"); // Your sprite sheet image
ImageView imageView = new ImageView(spriteSheet);
Timeline timeline = new Timeline(new KeyFrame(Duration.seconds(0.1), event -> {
int frameIndex = (int) ((event.getTarget()) % SPRITE_COUNT);
imageView.setViewport(new javafx.geometry.Rectangle2D(
frameIndex * FRAME_WIDTH, 0, FRAME_WIDTH, FRAME_HEIGHT));
}));
timeline.setCycleCount(Animation.INDEFINITE);
timeline.play();
Pane root = new Pane(imageView);
Scene scene = new Scene(root, 256, 256);
primaryStage.setTitle("Sprite Animation Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
- Imports: We import necessary JavaFX packages to work with the application.
- Constant Definitions: Constants define the number of frames and the dimensions of each frame in the sprite sheet.
- ImageView Setup: The
ImageView
component displays the sprite sheet. We set its viewport to modify where the ImageView pulls its content from. - Timeline Animation: A
Timeline
is used to manage the animation frames. Here, we set a KeyFrame that updates which frame is visible every 0.1 seconds. - Viewport Rectangle: The
setViewport
method adjusts which part of the sprite sheet is displayed, taking into account the width and height of each frame.
This snippet creates a basic sprite animation that continuously cycles through frames for a smoother effect.
Enhancing Animation
1. Adding Multiple Animations
If you have different sprite sheets for various actions (like walking or jumping), you can create separate ImageView
instances and manage them similarly within your main application.
2. An Example with Player Movement
Consider enhancing your sprite with player controls. You might want to only play the walking animation if the user presses arrow keys.
Code Snippet: Player Movement Example
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.input.KeyEvent;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
import javafx.animation.Animation;
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.util.Duration;
public class PlayerMovementExample extends Application {
private static final int SPRITE_COUNT = 4;
private static final int FRAME_WIDTH = 64;
private static final int FRAME_HEIGHT = 64;
private boolean isMoving = false;
@Override
public void start(Stage primaryStage) {
Image spriteSheet = new Image("spritesheet.png");
ImageView imageView = new ImageView(spriteSheet);
imageView.setViewport(new javafx.geometry.Rectangle2D(0, 0, FRAME_WIDTH, FRAME_HEIGHT));
Timeline timeline = new Timeline(new KeyFrame(Duration.seconds(0.1), event -> {
if (isMoving) {
int frameIndex = (int) ((event.getTarget()) % SPRITE_COUNT);
imageView.setViewport(new javafx.geometry.Rectangle2D(frameIndex * FRAME_WIDTH, 0, FRAME_WIDTH, FRAME_HEIGHT));
}
}));
timeline.setCycleCount(Animation.INDEFINITE);
timeline.play();
Pane root = new Pane(imageView);
Scene scene = new Scene(root, 256, 256);
scene.addEventFilter(KeyEvent.KEY_PRESSED, keyEvent -> {
switch (keyEvent.getCode()) {
case RIGHT:
isMoving = true;
break;
}
});
scene.addEventFilter(KeyEvent.KEY_RELEASED, keyEvent -> {
switch (keyEvent.getCode()) {
case RIGHT:
isMoving = false;
break;
}
});
primaryStage.setTitle("Player Movement Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
In this extended example, we’ve introduced user controls that dictate whether the sprite can move. Key points:
- Player Input Handling: The
addEventFilter
method captures key events and updates theisMoving
flag accordingly. - Animation Condition: The animation now only plays if the
isMoving
flag is true, providing feedback on user actions.
Closing the Chapter
Incorporating sprite animation in JavaFX is straightforward, yet offers vast opportunities for enhancing your applications, particularly in game development. This blog post showcased the basics, including setting up sprite sheets and controlling animations based on user input.
Further Reading
- For a more in-depth understanding of animations in JavaFX, check out JavaFX Animation Documentation.
- For advanced graphics in JavaFX, see JavaFX Graphics Documentation.
As you continue your journey with JavaFX, remember that practice is key. Experiment with different sprite sheets and animations to bring your characters to life! Good luck, and happy coding!
Checkout our other articles