Common Garbage Collection Issues in Java and Their Fixes
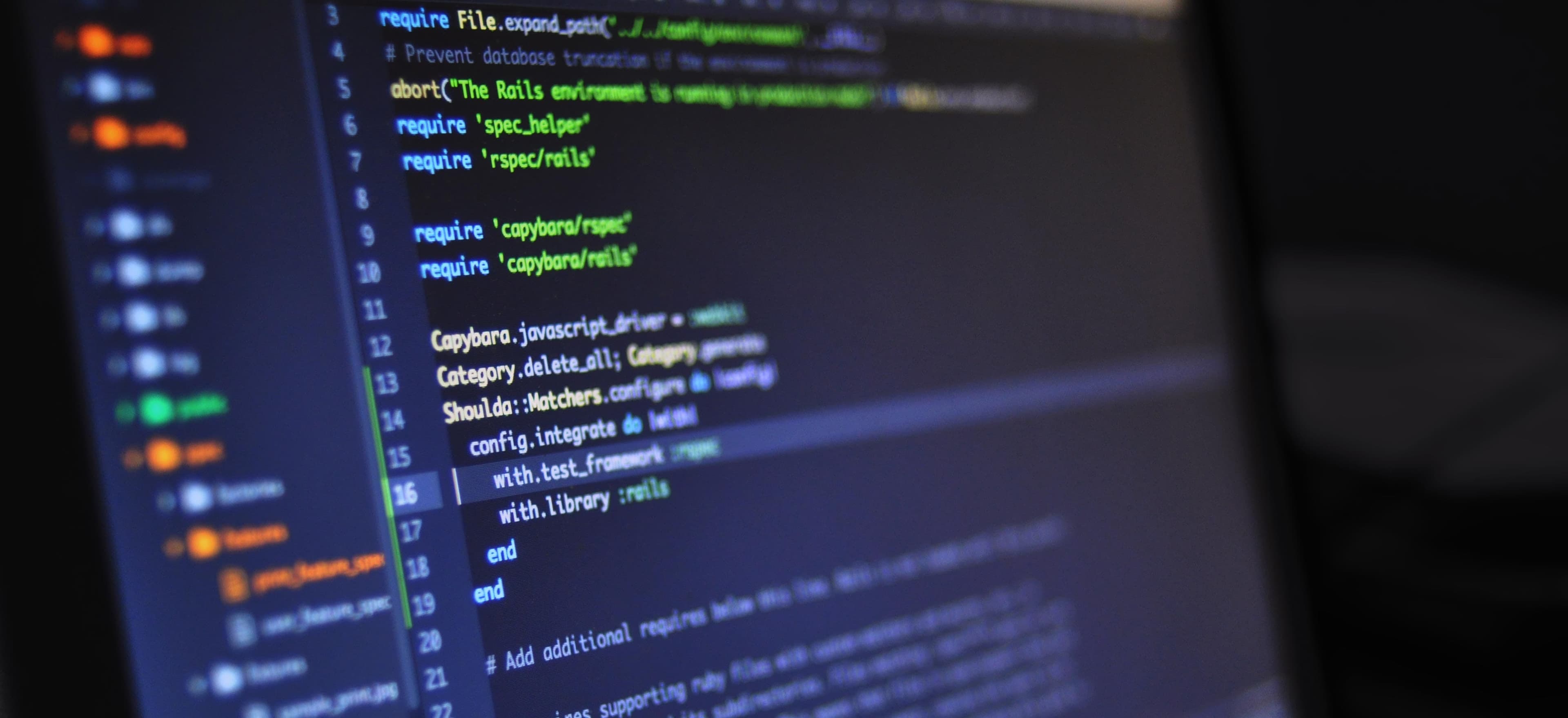
- Published on
Common Garbage Collection Issues in Java and Their Fixes
Garbage Collection (GC) is a critical aspect of Java programming that ensures efficient memory management. While it automatically cleans up unused objects, developers often face issues that hinder performance. In this blog post, we will explore common garbage collection problems in Java, analyze their causes, and discuss effective strategies for resolution. Our aim is to empower you to enhance your application performance through better memory management.
Understanding Garbage Collection in Java
Before we dive into the common issues, let's refresh our understanding of how garbage collection works in Java. Java's GC is responsible for reclaiming memory occupied by objects that are no longer in use. It operates in the background, allowing developers to focus on building applications rather than managing memory directly.
Java offers several GC algorithms, including:
- Serial GC: Suitable for small applications.
- Parallel GC: Utilizes multiple threads to speed up GC processes. Best for multi-core machines.
- Concurrent Mark-Sweep (CMS): A low-pause collector ideal for applications requiring quick response times.
- G1 (Garbage First): A modern collector that aims to balance latency and throughput.
Understanding these algorithms can help us tackle their associated issues more effectively.
Common Garbage Collection Issues
1. Long Garbage Collection Pauses
Problem
Long pauses during garbage collection can degrade application performance, especially in latency-sensitive applications. These pauses occur when the GC takes a significant amount of time to reclaim memory, leading to delays that users might notice.
Why It Happens
- Large heaps can require extensive time to scan for objects to reclaim.
- A high allocation rate can lead to frequent GC cycles.
Fixes
-
Tune Heap Size: Adjusting the Java heap size can mitigate long GC pauses. You can use the
-Xms
(initial heap size) and-Xmx
(maximum heap size) flags.java -Xms512m -Xmx2048m -jar myapp.jar
-
Use G1 Garbage Collector: G1 is designed to minimize pause times. You can enable it with:
java -XX:+UseG1GC -jar myapp.jar
2. Frequent Full GCs
Problem
Frequent full garbage collections can lead to application performance issues due to the lengthy duration of the full GC process. This is particularly troublesome in production environments.
Why It Happens
- Insufficient heap size because of memory leaks.
- High memory allocation rates leading to frequent exhaustion of the Eden space.
Fixes
- Profile Your Application: Use tools like VisualVM or Java Mission Control to identify memory leaks and analyze memory usage patterns.
- Increase Heap Size: Similar to the previous fix, increasing
-Xmx
can help reduce the frequency of full GCs.
3. Memory Leaks
Problem
Memory leaks occur when objects that are no longer required are still referenced, preventing the garbage collector from reclaiming their memory. This can lead to OutOfMemoryErrors.
Why It Happens
- Unintentional object retention through static references or unintended collections.
- Poor management of collections like Lists and Maps.
Fixes
-
Use Weak References: For caching or listeners, use
WeakReference
, which allows the garbage collector to reclaim memory when needed.class Cache { private final Map<String, WeakReference<Object>> cacheMap = new HashMap<>(); public void put(String key, Object value) { cacheMap.put(key, new WeakReference<>(value)); } public Object get(String key) { WeakReference<Object> weakRef = cacheMap.get(key); return weakRef != null ? weakRef.get() : null; } }
-
Track Object Lifecycle: Use profiling tools to monitor and optimize the object lifecycle in your application.
4. Increased Memory Consumption
Problem
Sudden spikes in memory usage can result from inefficient garbage collection, causing the application to consume more memory than necessary.
Why It Happens
- Excessive object creation and high allocation rates can lead to increased memory consumption.
Fixes
-
Optimize Object Creation: Reduce the number of temporary objects created within frequently called methods. For example, instead of creating new strings:
// Bad Practice StringBuilder builder = new StringBuilder(); for (int i = 0; i < 1000; i++) { builder.append("Item ").append(i).append("\n"); } String result = builder.toString();
Consider reusing existing objects.
-
Utilize Object Pools: Implementing object pooling for expensive objects can minimize memory spikes.
5. Genotype Bloat
Problem
In generational garbage collection, objects are divided into different generations (Young, Survivor, Old). If the birth rate of objects in the young generation is dramatically higher than the rate of recycling, it can cause the old generation to grow uncontrolled.
Why It Happens
- Rapid object creation and late promotion to the old generation remain unprocessed.
Fixes
-
Monitor Promotion Rates: Configure the size of your young and old generations based on application needs. For example:
java -XX:NewRatio=2 -jar myapp.jar
This will ensure that the young generation is twice as large as the old generation.
My Closing Thoughts on the Matter
Garbage collection in Java is essential for memory management, but it is not without its challenges. By understanding the common issues and employing the fixes outlined in this article, developers can significantly optimize their Java applications for better performance. Remember to regularly profile your application and refine memory settings based on its unique behavior.
For further reading on Java memory management, consider visiting Java Performance Tuning and explore best practices to ensure your application is both efficient and robust.
Embrace these strategies, and watch as your Java applications run smoother and faster, free from the burdens of common garbage collection issues.
Checkout our other articles