Streamlining Object Mapping for NetSuite Data in Java
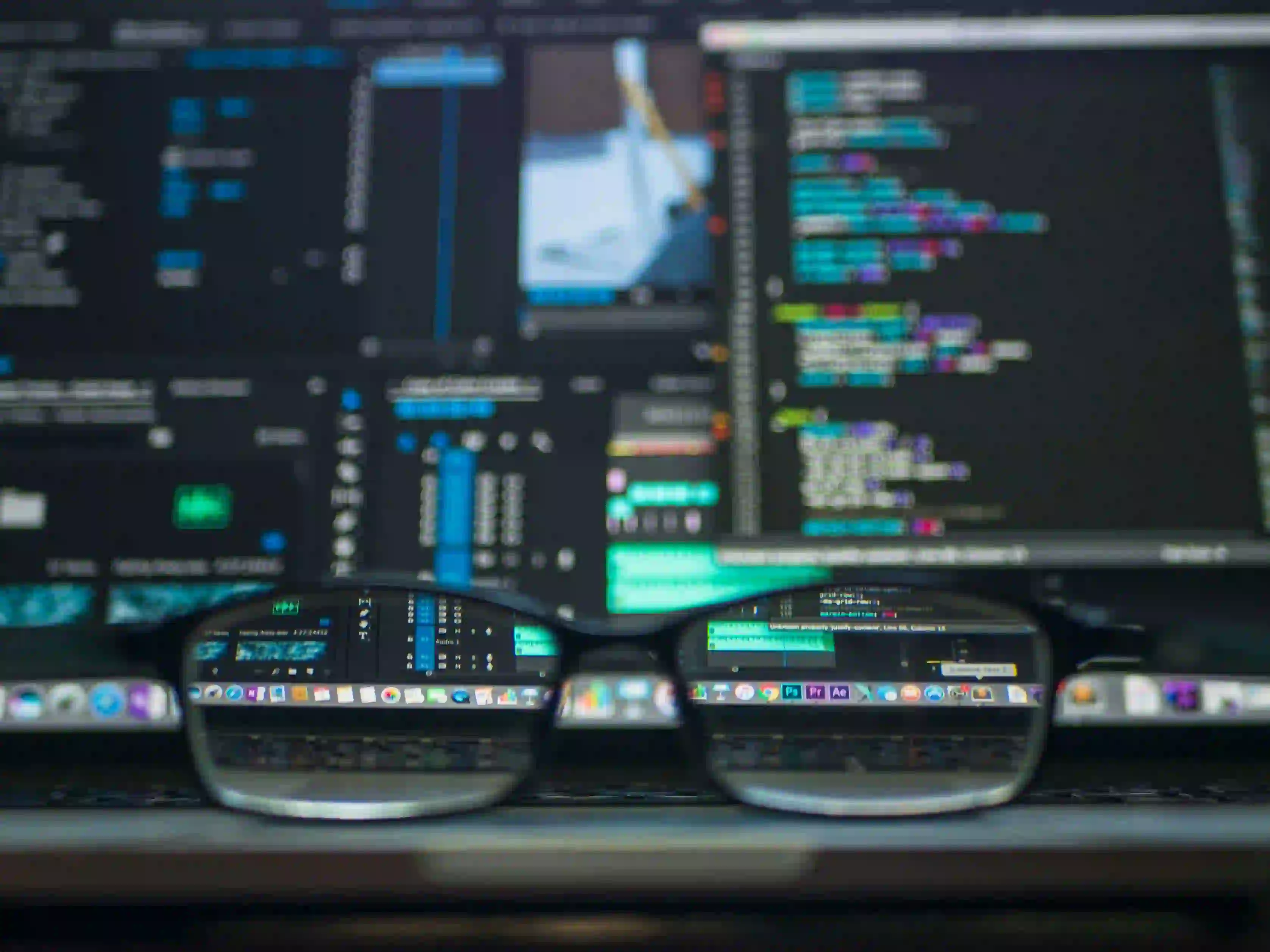
Streamlining Object Mapping for NetSuite Data in Java
As businesses grow, the need for effective data management becomes critical. Organizations that use systems like NetSuite can often find themselves overwhelmed with the amount of data flowing in and out of their applications. Java, being one of the most widely used programming languages, offers various frameworks and libraries that can help streamline data processing. This blog post explores how to simplify object mapping for NetSuite data in Java, ensuring efficient data handling without compromising on performance or readability.
Understanding Object Mapping
What is Object Mapping?
Object mapping is the process of converting data objects from one form to another. In the context of Java and NetSuite, it involves mapping data from an external source, like the NetSuite API, into Java objects that can be easily manipulated. This makes it easier for developers to work with the data, apply business logic, and perform operations without dealing with low-level details.
Why is it Important?
-
Maintainability: By using object mapping, you abstract the complexity of the data structure. This makes your code easier to read and maintain.
-
Performance: Efficient mapping reduces the overhead associated with data handling, which is essential for high-performance applications.
-
Code Reusability: With well-defined objects, you can reuse code across multiple methods and classes, reducing redundancy.
Setting Up Your Java Environment
Before jumping into object mapping, we need to ensure that your Java environment is ready. Here's a recommended setup:
- Java JDK: Make sure you have the latest Java Development Kit installed.
- Maven: Use Maven for dependency management to pull required libraries easily.
- NetSuite SDK: Integrate the NetSuite SDK in your project for seamless API interactions.
Sample Maven Dependency for NetSuite SDK
<dependency>
<groupId>com.netsuite</groupId>
<artifactId>netsuite-sdk</artifactId>
<version>2023.1.0</version>
</dependency>
Choosing a Mapping Strategy
There are several strategies for object mapping in Java when dealing with NetSuite data, including:
- Manual Mapping: Writing custom code to map NetSuite data to Java objects.
- Using an ORM: Using Object Relational Mapping tools like Hibernate, which can automatically handle data mapping.
- Data Transformation Libraries: Using libraries that specialize in transforming data between different formats.
In this post, we will focus on a more manual but structured approach for better understanding and control over the mapping process.
Creating Java Classes for NetSuite Data
To begin the mapping process, we will define Java classes that represent the entities we will retrieve from NetSuite. Let’s consider a simple example where we're retrieving customer data.
Customer Class
Here’s a sample class to represent customer data:
public class Customer {
private String id;
private String firstName;
private String lastName;
private String email;
public Customer(String id, String firstName, String lastName, String email) {
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
// Getters and setters omitted for brevity
@Override
public String toString() {
return "Customer{id='" + id + "', firstName='" + firstName + "', lastName='" + lastName + "', email='" + email + "'}";
}
}
Explanation
-
Attributes: The class has four attributes representing the customer's ID, first name, last name, and email.
-
Constructor: The constructor initializes these attributes, ensuring that all customer objects start with valid data.
-
toString Method: This method provides a readable string representation of the Customer data, which is helpful for debugging.
Fetching Data From NetSuite
To retrieve customer data from NetSuite, we would typically make a query through their API. Below is a simplified example for fetching a list of customers:
import com.netsuite.webservices.platform.core.RecordRef;
import com.netsuite.webservices.lists.customer.CustomerSearch;
import com.netsuite.webservices.lists.customer.CustomerSearchAdvanced;
import com.netsuite.webservices.core.Status;
import java.util.ArrayList;
import java.util.List;
public class CustomerService {
public List<Customer> getAllCustomers() {
List<Customer> customers = new ArrayList<>();
// Assume searchCustomers is a method that performs an actual search
CustomerSearch customerSearch = new CustomerSearch();
// Perform the search
CustomerSearchAdvanced searchResult = searchCustomers(customerSearch);
// Check for success
if (searchResult.getStatus() == Status.SUCCESS) {
for (var record : searchResult.getRecordList()) {
RecordRef recordRef = (RecordRef) record;
customers.add(mapRecordToCustomer(recordRef));
}
}
return customers;
}
private Customer mapRecordToCustomer(RecordRef recordRef) {
// Assuming RecordRef has methods to get necessary info
String id = recordRef.getInternalId();
String firstName = recordRef.getFirstName();
String lastName = recordRef.getLastName();
String email = recordRef.getEmail();
return new Customer(id, firstName, lastName, email);
}
}
Explanation
-
Data Retrieval: The
getAllCustomers
method performs a search on NetSuite's customer records. -
Mapping Records: The
mapRecordToCustomer
method converts aRecordRef
object to aCustomer
object. This separation of concerns keeps your code clean and easy to debug. -
List Creation: The resulting customers are stored in a List, ready for further processing or return.
Error Handling in Object Mapping
Handling errors is vital when dealing with data mapping. When retrieving data from an API, you might encounter various errors, such as network issues or data integrity errors. Here’s how you can implement effective error handling:
public List<Customer> getAllCustomers() {
List<Customer> customers = new ArrayList<>();
try {
// Search logic
} catch (ApiException e) {
// Log the exception
System.err.println("API error occurred: " + e.getMessage());
} catch (Exception e) {
// Catch all other exceptions
System.err.println("An unexpected error occurred: " + e.getMessage());
}
return customers;
}
Best Practices
-
Logging: Use a logging framework to record errors. This will help in troubleshooting.
-
Custom Exceptions: Create custom exceptions tailored to your application's needs.
-
Validation: Before mapping data, perform necessary validation checks to avoid incorrect data mapping.
Testing Object Mapping
In Java, unit testing is critical for ensuring your mapping functions work as intended. Using JUnit or another testing framework, you can create tests for your mapping logic.
Example Test Case
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CustomerServiceTest {
@Test
public void testMapRecordToCustomer() {
RecordRef recordRef = new RecordRef("123", "John", "Doe", "john.doe@example.com");
CustomerService service = new CustomerService();
Customer customer = service.mapRecordToCustomer(recordRef);
assertEquals("123", customer.getId());
assertEquals("John", customer.getFirstName());
assertEquals("Doe", customer.getLastName());
assertEquals("john.doe@example.com", customer.getEmail());
}
}
Explanation
-
Assertions: Using assertions allows you to verify that the mapping logic produces the desired results.
-
Isolation: The test isolates the mapping logic from the rest of the application, allowing for focused testing and debugging.
Key Takeaways
Streamlining object mapping for NetSuite data in Java is essential for any organization looking to manage its data effectively. By using proper Java classes, handling errors gracefully, implementing testing strategies, and following best practices, your data handling becomes more efficient and secure.
For those looking to explore more about Java and NetSuite integration, consider diving into the official NetSuite documentation and exploring available Java libraries that help streamline development processes.
With these best practices and methodologies, you can enhance your business’s data workflow significantly. Happy coding!