Turning Legacy Code into a Testing Dream: A Step-by-Step Guide
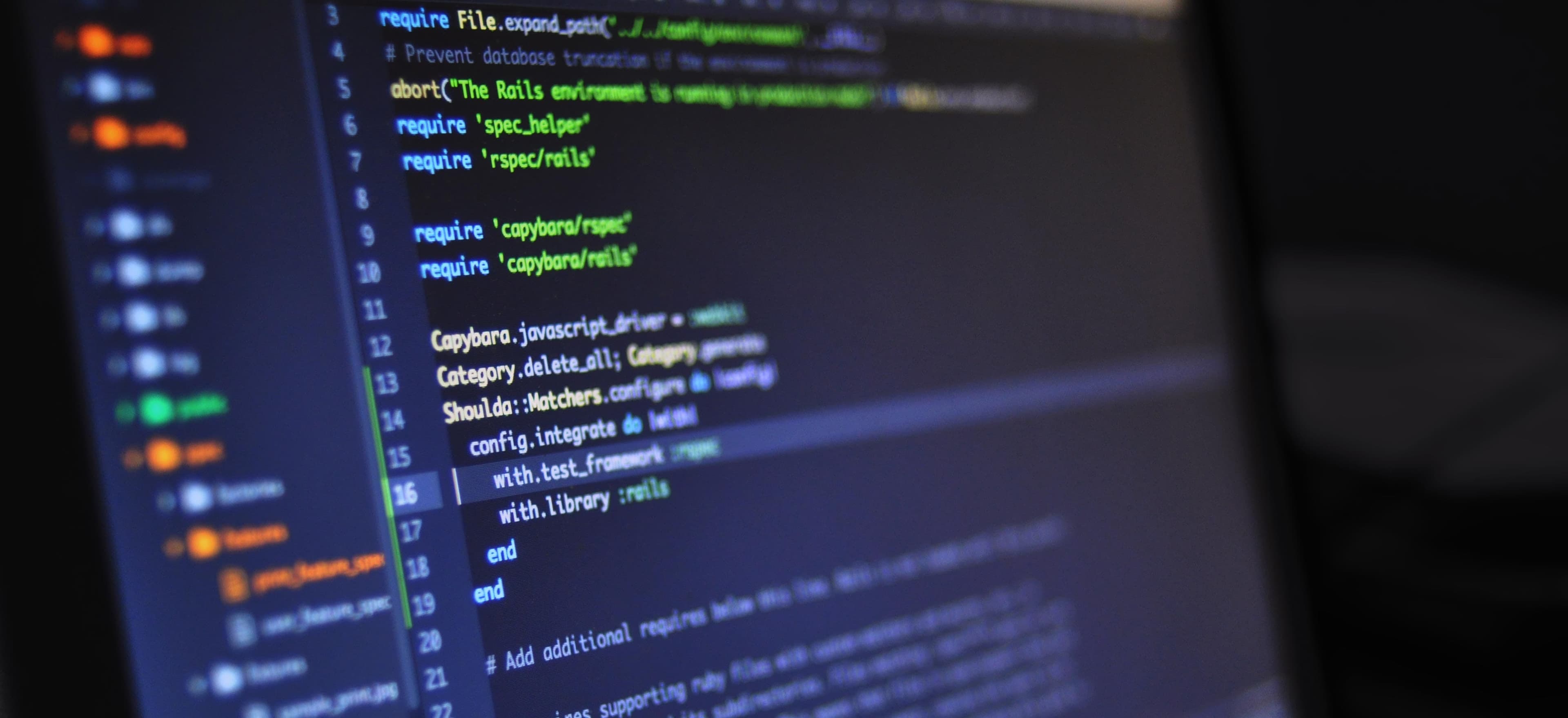
- Published on
Turning Legacy Code into a Testing Dream: A Step-by-Step Guide
Legacy code can often feel like a tangled web of confusion and outdated practices. While it may have served its purpose for years, the absence of tests around it can hamper your ability to maintain and enhance it. However, transforming this legacy code into a robust, test-friendly ecosystem is achievable. In this guide, we will walk through the steps involved in modernizing legacy code with an emphasis on writing unit tests.
Why Test Legacy Code?
Before we dive in, let's discuss why testing legacy code is vital. Here are a few key points:
- Facilitating Change: Tests act as a safety net during refactoring, ensuring that your code changes don’t introduce new bugs.
- Documentation: Tests serve as a form of documentation. They clarify how the code is intended to work.
- Preventing Regression: Automated tests help catch bugs that might occur when functionalities are altered or added.
Step 1: Understand the Legacy Code
First and foremost, you need to get familiar with the codebase. Here’s how to approach it:
- Read the Code: Try to understand the architecture, logic flow, and key components. Identifying the primary functionalities is critical.
- Run the Application: Set up the application in your local environment to see how it works. This firsthand experience can provide insights into areas that might need testing.
- Identify Key Components: Start mapping out which components are critical and need the highest priority for testing.
Example Code Snippet
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
Commentary: This simple Calculator
class might seem straightforward, but it encapsulates functionality that could affect larger systems depending on how it is used. Understanding this code is fundamental before writing tests.
Step 2: Set Up a Testing Framework
Choosing the right testing framework depends on your tech stack. For Java, popular frameworks include:
To get started with JUnit, add the following dependency if you're using Maven:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
This setup allows you to run your tests with the JUnit engine.
Step 3: Write Your First Test
Writing tests for legacy code can feel daunting. Start simple. Here’s how to write a unit test for our Calculator
class:
JUnit Test Example
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
@Test
public void testSubtract() {
Calculator calculator = new Calculator();
assertEquals(1, calculator.subtract(3, 2), "3 - 2 should equal 1");
}
}
Commentary: This code uses JUnit’s assertions to verify the output of the add
and subtract
methods in the Calculator
class. Notice how we provide a message that explains what is being tested; this can be invaluable for troubleshooting.
Step 4: Make Legacy Code Testable
Some legacy code may not be inherently testable due to its structure. If it tightly couples components or has side effects, you might need to refactor it first.
Refactoring Example
Say your Calculator
depends on external services (like logging or databases), which hinders testing. You can refactor by introducing interfaces.
public interface Logger {
void log(String message);
}
public class ConsoleLogger implements Logger {
public void log(String message) {
System.out.println(message);
}
}
public class Calculator {
private final Logger logger;
public Calculator(Logger logger) {
this.logger = logger;
}
public int add(int a, int b) {
int result = a + b;
logger.log("Adding: " + result);
return result;
}
}
Commentary: Now you can create mock objects for Logger
during unit testing, enabling you to isolate the Calculator
functionality.
Step 5: Run Your Tests
After writing your tests, execute them. If using an IDE like IntelliJ or Eclipse, look for the option to run tests. You can also run them from the command line using Maven:
mvn test
Monitor the output to ensure all tests pass. If a test fails, scrutinize the error messages and debug accordingly.
Step 6: Gradually Increase Test Coverage
To effectively transition your legacy codebase into a testing dream, don’t try to tackle everything at once. Focus on high-priority components first, gradually increasing your test coverage:
- Identify Risky Areas: Use tools like JaCoCo to analyze which parts of your codebase are untested.
- Implement Tests Iteratively: Follow practices like Test-Driven Development (TDD) or Behavior-Driven Development (BDD) to create a sustainable testing strategy.
Step 7: Continuous Integration
Incorporate your tests within a CI/CD pipeline. Tools like Jenkins or GitHub Actions ensure that every change gets tested, maintaining code quality over time.
Example GitHub Action
name: Java CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Maven Build
run: mvn clean install
Commentary: This simple GitHub Action script will run tests every time a commit is pushed to the repository. It promotes quality and keeps your codebase stable.
Lessons Learned
Turning legacy code into a testing dream requires patience and strategy. By understanding the legacy systems, setting up a robust testing framework, refactoring where necessary, and leveraging modern practices like CI, you can significantly enhance code quality.
This transformation not only eases the burden of maintaining the existing code but also fosters a culture of quality and continuous integration within your development team. As you embark on this journey, remember that every step, no matter how small, brings you closer to a more maintainable and trustworthy codebase.
For more on transforming code, consider looking into Clean Code by Robert C. Martin or Refactoring by Martin Fowler. Happy coding!
This blog post was crafted to provide you with actionable steps and knowledge about navigating legacy code and transforming it into a testable structure. By implementing these methods, you'll not only improve the immediate codebase but also set a precedent for best practices within your team.