How to Tackle Common Issues in JMetro 5.3 Update
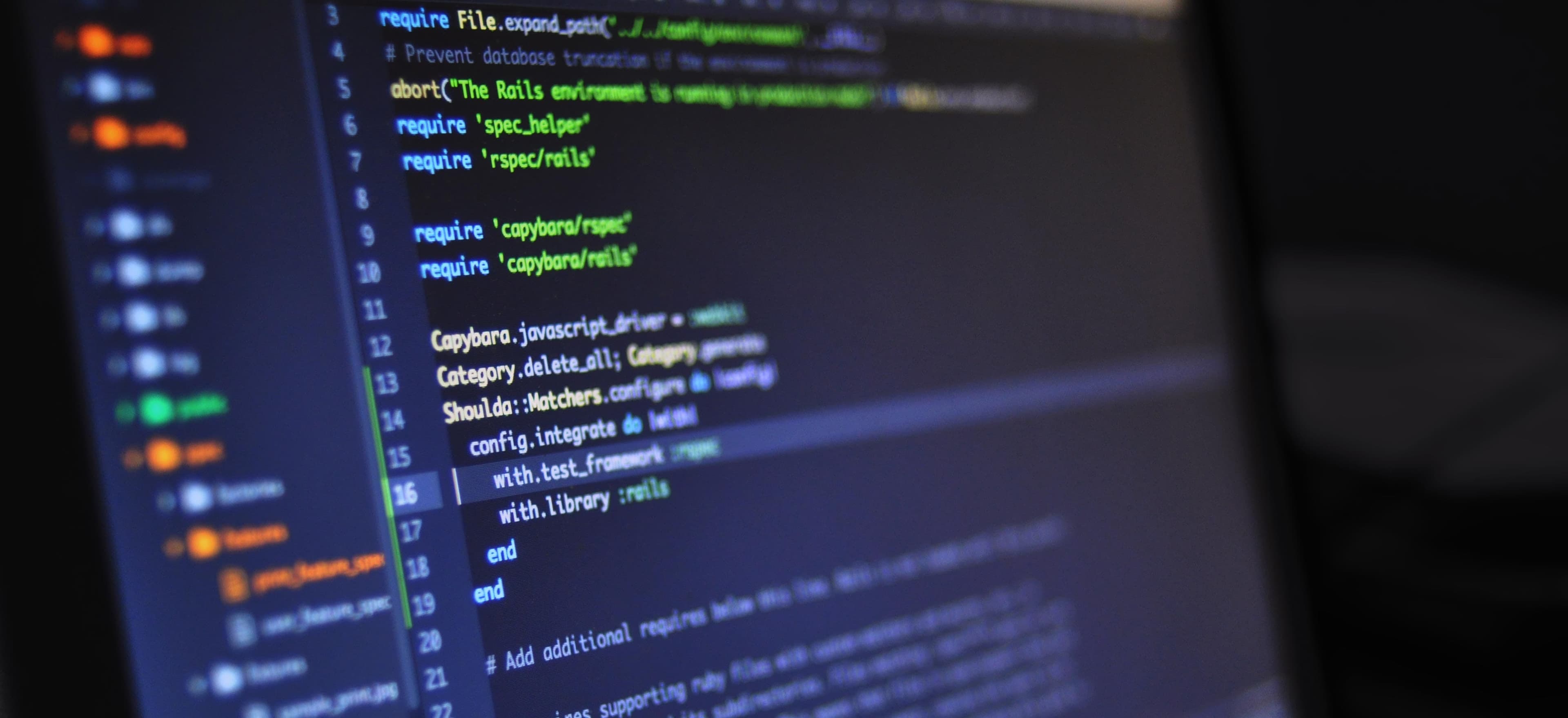
- Published on
How to Tackle Common Issues in JMetro 5.3 Update
JMetro is an increasingly popular library in the Java ecosystem that allows developers to harness the power of the JavaFX framework while also offering a modern interface reminiscent of the Material Design principles. With the recent JMetro 5.3 update, new functionalities and potential bug fixes were introduced; however, users may also face some common issues.
In this blog post, we will discuss how to troubleshoot and resolve these problems. We will also provide exemplary code snippets to help you effectively implement JMetro in your applications.
Why Choose JMetro?
Before diving into the common issues of JMetro 5.3, it's worth discussing why JMetro stands out as an essential library for Java developers.
-
Modern User Interfaces: JMetro allows you to create modern, sleek interfaces for your JavaFX applications, making them visually appealing.
-
Ease of Implementation: The library is friendly for developers of all experience levels. With clear documentation and a strong community, you are more likely to find solutions to problems quickly.
-
Cross-Platform Compatibility: One of Java's strong points is its platform independence, and JMetro adheres to this philosophy, allowing you to create applications that work seamlessly across different operating systems.
The combination of those factors makes it evident why developers gravitate toward JMetro. However, let’s address the challenges that may arise with this library, especially with the latest version update.
Common Issues in JMetro 5.3
Issue #1: Dependency Conflicts
One of the most common hurdles developers may face when updating to JMetro 5.3 is dependency conflicts. This occurs when different libraries require different versions of common dependencies.
Solution:
To tackle this, you can use Maven or Gradle dependency management. Here’s how to specify the right dependency in a Maven pom.xml
file:
<dependency>
<groupId>org.jmetro</groupId>
<artifactId>jmetro</artifactId>
<version>5.3.0</version>
</dependency>
You can ensure compatibility by using the Maven dependency plugin to analyze your project dependencies:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>3.1.2</version>
<executions>
<execution>
<id>analyze</id>
<goals>
<goal>analyze</goal>
</goals>
</execution>
</executions>
</plugin>
Issue #2: Theme Application Not Working
Another issue commonly reported with the JMetro 5.3 upgrade is the application of the theme not reflecting visually in the UI. This can be frustrating, especially if you have spent time designing an appealing layout.
Solution: The theme must be applied correctly in your JavaFX application. Below is a code snippet showcasing how to properly set a JMetro theme:
import org.jmetro.JMetro;
import org.jmetro.Style;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class JMetroApp extends Application {
@Override
public void start(Stage primaryStage) {
// Create a layout
StackPane root = new StackPane();
Scene scene = new Scene(root, 300, 250);
// Apply JMetro
JMetro jMetro = new JMetro(Style.LIGHT);
jMetro.setScene(scene);
primaryStage.setTitle("JMetro Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this example, we create a simple JavaFX application and use the JMetro
class to apply a LIGHT theme. Make sure that you're calling jMetro.setScene(scene)
before showing the primary stage.
Issue #3: Missing Fonts
JMetro relies on specific font resources, and upon upgrading, you might find that some fonts are missing or not rendering as expected.
Solution:
Ensure that you have all the necessary font files included in your project’s resources. You can include them by using the JavaFX Font.loadFont
method:
import javafx.scene.text.Font;
Font.loadFont(getClass().getResourceAsStream("/path/to/font.ttf"), 12);
Ensure the path to your font file is correct. You may consider using a popular web font service like Google Fonts to find suitable fonts for your application. For deeper insights, check out Google Fonts.
Issue #4: Performance Issues
With new features and additional complexities, some users experience performance issues in their applications post-update.
Solution:
To optimize performance, ensure that you efficiently manage UI components and layout rendering. For instance, if your UI contains a list or table, use virtualization. The JavaFX ListView
or TableView
already has virtualization enabled, which helps improve performance.
Example of a ListView
:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ListView;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.collections.FXCollections;
public class ListViewExample extends Application {
@Override
public void start(Stage primaryStage) {
ListView<String> listView = new ListView<>();
listView.setItems(FXCollections.observableArrayList("Item 1", "Item 2", "Item 3"));
StackPane root = new StackPane();
root.getChildren().add(listView);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("ListView Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
By following these best practices and utilizing JavaFX features, you can significantly enhance the performance of your JMetro application.
A Final Look
While the JMetro 5.3 update brings along its share of challenges, the benefits it offers in enabling the creation of modern JavaFX applications are worth overcoming these hurdles. By being aware of the common issues and their solutions presented in this post, you can streamline your development process and craft beautiful applications with relative ease.
As you continue to develop with JMetro, we encourage you to stay connected with the JMetro GitHub page for updates and additional resources related to the library.
Feel free to reach out in the comments below if you encounter other issues or have tips for fellow developers tackling JMetro! Happy coding!
Checkout our other articles