Streamlining Your Testing Process: Tips for Speed and Efficiency
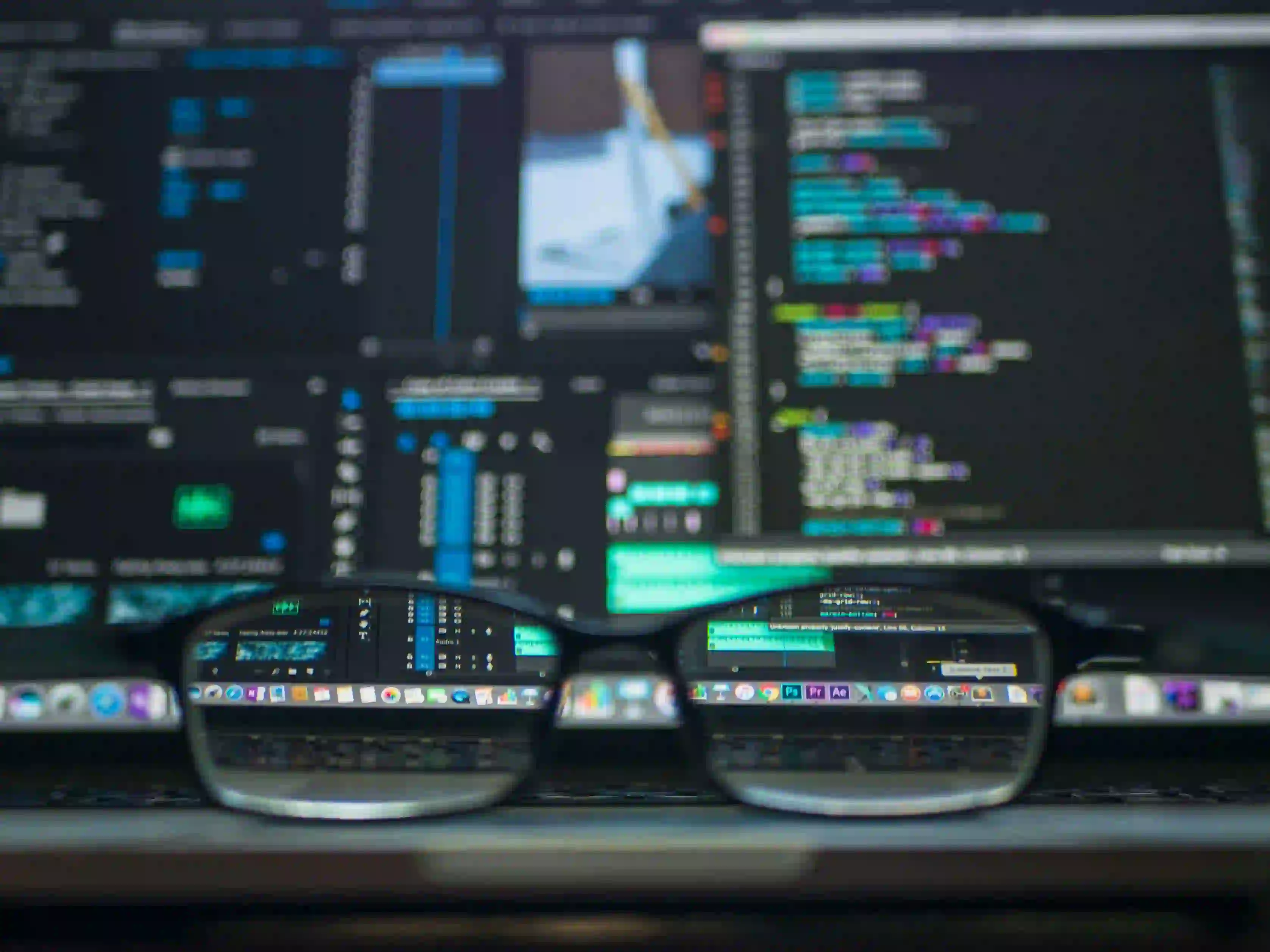
Streamlining Your Testing Process: Tips for Speed and Efficiency
In today's fast-paced software development environment, efficient testing is crucial for ensuring quality and maintaining a competitive edge. This blog post explores practical tips for streamlining your testing process, focusing on both speed and efficiency. Whether you are a seasoned developer or just beginning your journey, these strategies will help you streamline your testing workflow.
Why Testing is Essential
Testing is not just a phase; it is an integral part of the software development lifecycle. It ensures that your application is free of bugs and functions as intended. Moreover, thorough testing enhances user satisfaction and boosts your reputation in the market. Here are some benefits of an efficient testing process:
- Faster Time to Market: Speedy testing allows quicker feedback, aiding in rapid deployment.
- Improved Quality: Consistent testing leads to fewer defects and higher-quality products.
- Cost Efficiency: Identifying and fixing bugs early in the development process saves money.
1. Automate Where Possible
Automation is your best friend when it comes to streamlining tests. By automating repetitive tests, you save time and resources, allowing your team to focus on more complex areas.
Code Snippet: Simple Test Automation with JUnit
Here’s a simple example of how to use JUnit for automated testing in Java:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(5, 3);
assertEquals(8, result);
}
}
Why Use JUnit? JUnit provides a straightforward framework for unit testing in Java. It allows you to run tests easily and integrates seamlessly with build tools like Maven.
More Resources
- JUnit Documentation
- Test Automation Best Practices
2. Implement Test-Driven Development (TDD)
Test-Driven Development is a software development approach in which you write tests before coding the actual functionality. This method ensures that your code is continually tested and minimizes bugs in the long run.
Fundamental Steps of TDD
- Write a test for the functionality you are about to implement.
- Run the test to confirm it fails (as expected).
- Write the minimal code necessary for the test to pass.
- Refactor the code, ensuring it remains clean and efficient.
Example: TDD in Action
Let's say you want to implement a method that multiplies two numbers:
// Step 1: Write the test
@Test
public void testMultiplication() {
Calculator calculator = new Calculator();
int result = calculator.multiply(5, 3);
assertEquals(15, result);
}
// Step 2: Implement the method in the Calculator class
public class Calculator {
public int multiply(int a, int b) {
return a * b;
}
}
Why TDD? TDD reduces defects and leads to well-tested code even before the implementation starts. This practice can significantly enhance speed and efficiency.
More Resources
- Understanding TDD
- Practical TDD
3. Use Continuous Integration (CI)
Integrating CI into your workflow ensures that code changes are automatically tested. This minimizes integration problems and ensures that defects are found early.
Benefits of CI
- Immediate Feedback: Developers receive rapid feedback on their changes.
- Reduced Integration Issues: Regularly integrating and testing code helps to catch bugs early.
Example CI Tools
- Jenkins: An open-source automation server.
- Travis CI: A cloud-based CI service.
Setting Up CI with Jenkins
# Install Jenkins using apt
sudo apt-get install jenkins
Why CI? CI fosters a culture of collaboration and enables faster releases, enhancing overall productivity.
More Resources
- Jenkins Official Documentation
- Continuous Integration Explained
4. Optimize Test Cases
Not all test cases are created equal. You should focus on writing tests that provide valuable feedback while eliminating redundant tests.
Strategies for Optimizing Test Cases
- Prioritize Critical Paths: Focus on scenarios that are crucial for business.
- Eliminate Flaky Tests: Remove tests that often fail for non-code-related reasons.
- Use Parameterized Tests: Run a single test with multiple input values.
Example: Parameterized Tests with JUnit
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
@RunWith(Parameterized.class)
public class ParameterizedCalculatorTest {
private int a;
private int b;
private int expected;
public ParameterizedCalculatorTest(int a, int b, int expected) {
this.a = a;
this.b = b;
this.expected = expected;
}
@Parameterized.Parameters
public static Object[][] data() {
return new Object[][] {
{ 2, 3, 5 }, { 10, 5, 15 }, { 0, 0, 0 }
};
}
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(expected, calculator.add(a, b));
}
}
Why Parameterized Tests? They reduce duplication and allow testing multiple conditions efficiently, thus saving time.
More Resources
5. Maintain an Organized Test Suite
A well-structured test suite not only enhances clarity but also saves time in locating and running tests.
Tips for Managing Your Test Suite
- Group Similar Tests: Use packages or directories to categorize tests.
- Implement Naming Conventions: Choose meaningful names for test methods that describe their functionality.
- Regularly Review Tests: Periodically evaluate test relevance and effectiveness.
Example Directory Structure
src
├── main
│ └── java
│ └── com
│ └── example
│ └── calculator
│ └── Calculator.java
├── test
│ └── java
│ └── com
│ └── example
│ └── calculator
│ ├── CalculatorTest.java
│ └── ParameterizedCalculatorTest.java
Why Organize? An organized test suite increases the team's productivity by making it easier to update, run, and maintain tests.
More Resources
- How to Organize Your Test Suite
Final Thoughts
Incorporating these tips into your testing process will enhance both speed and efficiency. From automating tests to implementing TDD and CI, each strategy minimizes the chance of bugs and improves code quality. Remember, the ultimate goal is to deliver a robust product in the shortest possible time frame while maintaining high standards.
By refining your testing strategies, you pave the way for a successful development cycle, satisfied users, and a stellar reputation in the marketplace. Start making these changes now, and you'll see improvement in your workflow and product quality!
Feel free to reach out with questions or share your experiences in streamlining your testing process. Happy coding!