Mastering Java 8 Lambda Expressions: Common Pitfalls to Avoid
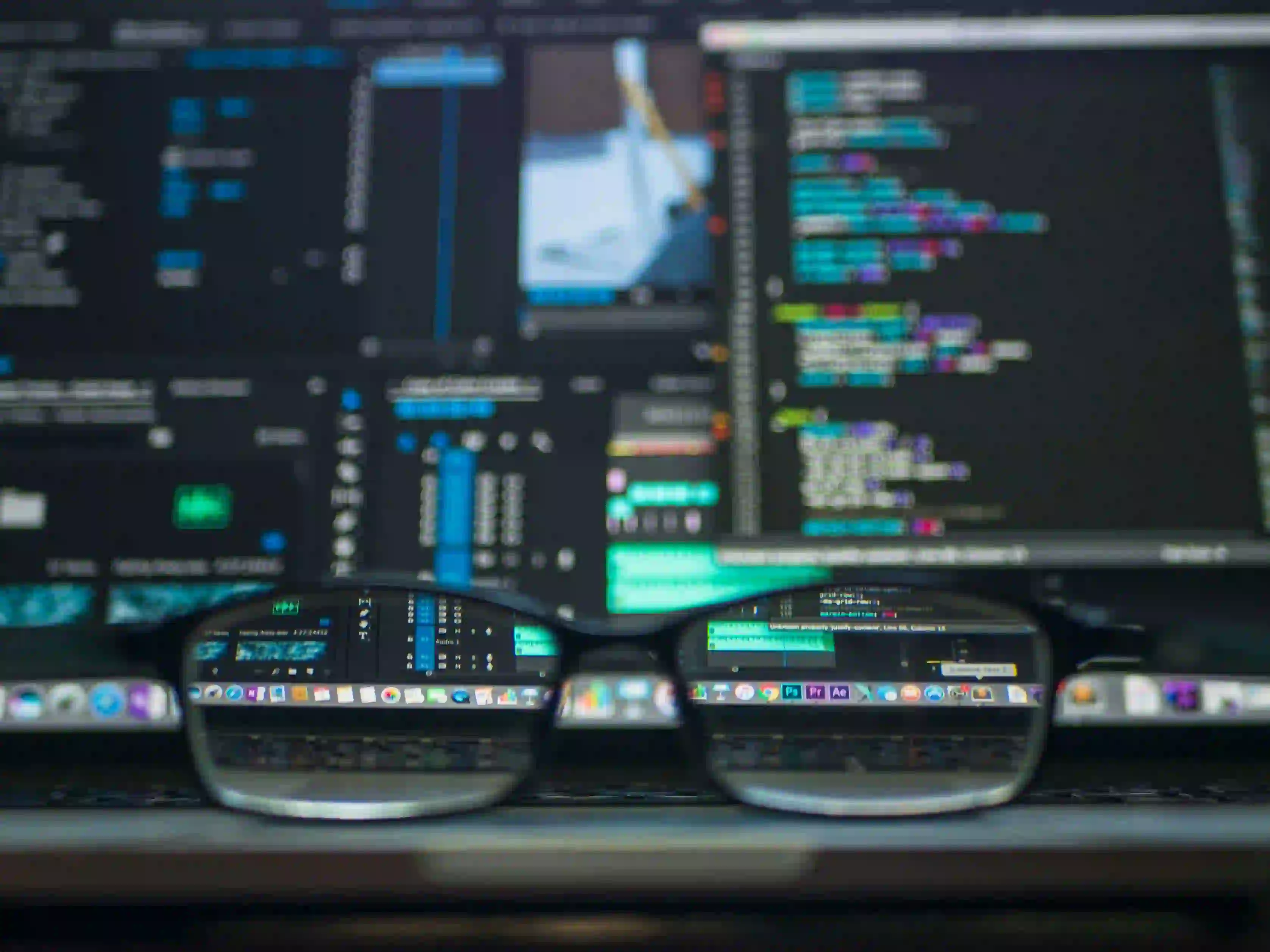
Mastering Java 8 Lambda Expressions: Common Pitfalls to Avoid
Java 8 brought significant enhancements to the Java programming language, with one of the most impactful being Lambda Expressions. They allow developers to write cleaner, more concise code, especially when working with Collections and functional interfaces. While Lambda expressions provide great flexibility, they also introduce nuances that can confuse even seasoned developers. In this article, we will explore common pitfalls of Lambda expressions in Java 8 and how to avoid them.
What Are Lambda Expressions?
In simple terms, a Lambda expression is a block of code which can be passed around and executed later. It consists of:
- Parameter List: The inputs that the lambda will accept.
- Arrow Token: The
->
symbol which separates the parameters from the body. - Body: The code that gets executed.
Syntax of Lambda Expressions
(parameter1, parameter2) -> { // code block }
A straightforward Lambda can also be expressed in a single line if it contains only one statement:
parameter -> expression
Example of a Simple Lambda
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
This code iterates over a list of names and prints each name to the console. The forEach()
method is a perfect example of using a Lambda expression for functional-style programming.
Common Pitfalls and How to Avoid Them
1. Not Understanding Scope
One of the most common pitfalls in using Lambda expressions is their interaction with variable scope. Unlike anonymous inner classes, Lambda expressions can access variables from their enclosing scope but must be effectively final.
Code Example
String name = "Alice";
Runnable r = () -> System.out.println(name);
name = "Bob"; // Error: name must be effectively final
Why This Matters
If you try to reassign name
after it's used in a Lambda expression, it will result in a compilation error. This is because the variable must not change its value. To avoid this, ensure variables referenced within a Lambda are immutable.
2. Using State in Lambdas
Using mutable shared state in Lambdas can lead to unexpected behavior. Java's Lambdas capture variables by value, not by reference. This means that if you change the variable outside the Lambda, it won't reflect inside the Lambda.
Code Example
IntStream.range(0, 10).forEach(i -> {
// avoid manipulating shared state
System.out.println(i);
});
Instead of modifying a shared variable inside a Lambda, opt for a data structure that can encapsulate state independently.
3. Type Inference Confusion
Java's type inference can sometimes lead to confusion. The compiler tries to infer the type of parameters, which may result in a mismatch. This is particularly troublesome in method references.
Code Example
Function<String, String> toUpperCase = str -> str.toUpperCase();
Here, the inferred type is clear. But in certain contexts, you might encounter compilation errors if the expected type isn’t explicit:
Function<String, String> toUpperCase = (String str) -> str.toUpperCase(); // Explicit types can help
Tips
Be explicit with types when necessary to help the compiler and improve code readability.
4. Lambda vs. Method Reference Confusion
Lambdas can sometimes be confusing when deciding if a method reference is appropriate. While they serve similar purposes, using method references can lead to clearer and more elegant code.
Example of Method Reference
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(System.out::println);
Using System.out::println
increases readability over writing out the Lambda directly.
When to Use Each
Use Lambdas for custom implementations that don’t have suitable method references. For direct mappings, prefer method references for cleaner code.
5. Avoid Complex Logic in Lambdas
Lambda expressions are best suited for small, simple operations. Placing complex logic can reduce code readability and maintainability.
Code Example
Predicate<String> filter = str -> {
// Complex logic should be avoided
return str.length() > 5 && str.startsWith("A") && !str.contains("Z");
};
Instead, refactor complex logic into separate methods:
private boolean isValid(String str) {
return str.length() > 5 && str.startsWith("A") && !str.contains("Z");
}
Predicate<String> filter = this::isValid;
6. Performance Considerations
While Lambda expressions can help create concise code, they might come with performance implications. Java creates a bridge between Lambdas and anonymous classes, potentially impacting performance under heavy usage.
Example
In high-performance scenarios, avoid overusing Lambdas, particularly in tight loops. Instead, use loops or more conventional approaches where necessary.
The Closing Argument
Lambda expressions in Java 8 are powerful tools that simplify programming. However, understanding their nuances is critical to avoid common pitfalls. Always remember:
- Be conscious of variable scope and immutability.
- Avoid mutable shared states.
- Use type inference judiciously.
- Prefer method references for simpler mappings.
- Keep Lambdas simple, and refactor complex logic.
- Consider performance, especially in performance-critical applications.
By being aware of these pitfalls and strategies, you can fully harness the potential of Lambda expressions in your Java projects.
If you want to dive deeper into Java 8 features, consider reading about the Streams API, which works hand-in-hand with Lambda expressions to facilitate functional-style programming.
Ultimately, mastering Lambda expressions will not only make your code cleaner but also enhance your coding efficiency. Happy coding!