Overcoming Lazy Initialization Issues in Your Code
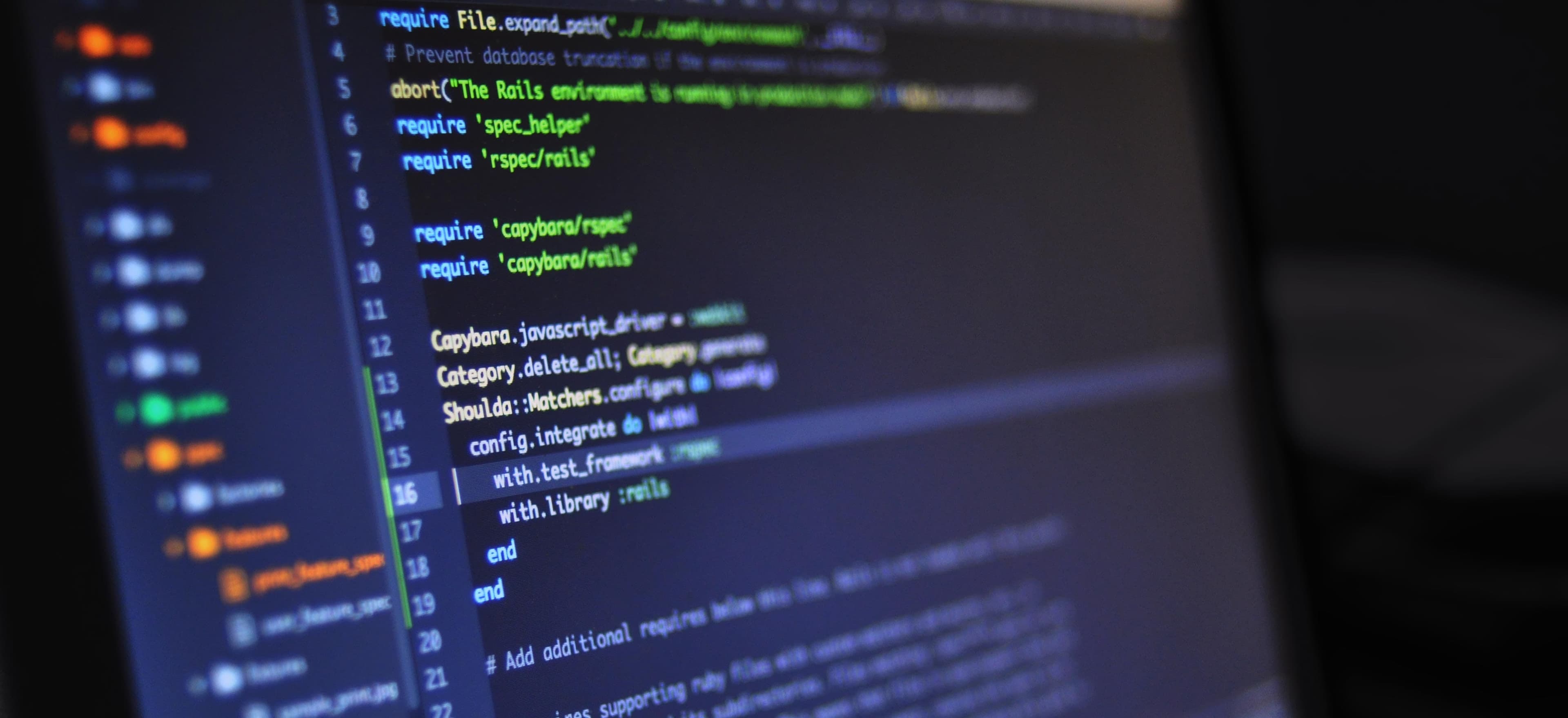
- Published on
Overcoming Lazy Initialization Issues in Your Code
Lazy initialization is an optimization technique commonly used in programming, where the creation of an object is deferred until it's actually needed. This can lead to performance improvements, particularly in applications where resources are expensive to create. However, lazy initialization can also introduce issues and complexities, especially in multi-threaded environments.
In this blog post, we will explore the nuances of lazy initialization in Java, how to effectively implement it, potential pitfalls, and best practices for overcoming these issues.
Understanding Lazy Initialization
Lazy initialization essentially means that an object’s instantiation is postponed until its first usage. The key advantages include reduced memory consumption and enhanced performance, especially when dealing with large data structures or resources that may never be used during the application's lifecycle.
Example of Lazy Initialization
Here's a simple example to illustrate lazy initialization in Java:
public class LazyLoadedSingleton {
private static LazyLoadedSingleton instance;
private LazyLoadedSingleton() {
// private constructor to prevent instantiation
}
public static LazyLoadedSingleton getInstance() {
if (instance == null) {
instance = new LazyLoadedSingleton();
}
return instance;
}
}
In this example, the LazyLoadedSingleton
class defers the creation of its instance (LazyLoadedSingleton instance
) until the getInstance()
method is called for the first time. If this instance has not been created yet, it initializes the object and returns it.
Pitfalls of Lazy Initialization
While lazy initialization can improve performance, it also comes with its own set of problems:
-
Thread Safety Issues: If multiple threads access the
getInstance()
method simultaneously, it may result in multiple instances of the singleton being created. -
Complexity: The logic in your codebase can become more convoluted, leading to maintenance difficulties.
-
Delayed Performance Costs: The first call to
getInstance()
can cause temporary delays due to object creation, which might be a concern in time-sensitive applications.
Overcoming Lazy Initialization Issues
-
Making Lazy Initialization Thread-Safe
One of the biggest challenges with lazy initialization is ensuring thread safety. Without proper synchronization, lazy initialization in a multi-threaded environment can lead to multiple instances of the object being created.
Using the synchronized keyword is one approach to achieving thread safety:
public class SynchronizedLazyLoadedSingleton {
private static SynchronizedLazyLoadedSingleton instance;
private SynchronizedLazyLoadedSingleton() {
// private constructor to prevent instantiation
}
public static synchronized SynchronizedLazyLoadedSingleton getInstance() {
if (instance == null) {
instance = new SynchronizedLazyLoadedSingleton();
}
return instance;
}
}
Here, the synchronized
modifier ensures that only one thread can execute the getInstance()
method at any time. This eliminates the risk of concurrent threads creating multiple instances.
-
Double-Checked Locking
While using synchronized
ensures thread safety, it can also reduce performance since every call to getInstance()
results in synchronized access. A common pattern to improve performance is called double-checked locking:
public class DoubleCheckedLockingSingleton {
private static volatile DoubleCheckedLockingSingleton instance;
private DoubleCheckedLockingSingleton() {
// private constructor to prevent instantiation
}
public static DoubleCheckedLockingSingleton getInstance() {
if (instance == null) {
synchronized (DoubleCheckedLockingSingleton.class) {
if (instance == null) {
instance = new DoubleCheckedLockingSingleton();
}
}
}
return instance;
}
}
In this implementation, we first check if the instance is null before entering the synchronized block. Once inside, we perform the null check again. This approach allows threads to bypass the costly synchronized block once the instance is initialized.
Why 'volatile'?
The use of the volatile
keyword prevents the instruction reordering that can lead to two threads getting different views of the instance variable. This is crucial because without it, the instance might be partially constructed, leading to unexpected behaviors.
-
Enum Singleton
Another effective way to implement lazy initialization with thread safety in Java is through an enum. This is a more modern approach, which can protect against multiple instance creation and serialization issues:
public enum EnumSingleton {
INSTANCE;
public void performAction() {
// logic here
}
}
Using an enum inherently ensures that only one instance of EnumSingleton
exists, making it a simple yet robust solution for a singleton pattern.
-
Lazy Initialization with Holder Classes
Another pattern is to use the Initialization-on-demand holder idiom. This method leverages static inner classes to achieve lazy loading and is thread-safe without requiring synchronization:
public class HolderLazyLoadedSingleton {
private HolderLazyLoadedSingleton() {
// private constructor to prevent instantiation
}
private static class Holder {
private static final HolderLazyLoadedSingleton INSTANCE = new HolderLazyLoadedSingleton();
}
public static HolderLazyLoadedSingleton getInstance() {
return Holder.INSTANCE;
}
}
In this case, the Singleton instance is created only when getInstance()
is called, holding off the initialization until it is actually needed. This also guarantees that no additional synchronization overhead is required.
The Closing Argument
Lazy initialization can be a powerful tool in your Java programming toolkit, offering performance benefits when used correctly. However, it also comes with challenges, particularly concerning thread safety and code complexity.
By following the best practices outlined in this post—such as using synchronized methods, double-checked locking, enum-based singletons, or holder classes—you can avoid common pitfalls and ensure your application runs smoothly under concurrent operations.
To further explore threading and synchronization in Java, you may find these resources helpful: Java Concurrency in Practice and Oracle's Java Tutorials on Concurrency.
By understanding and implementing these techniques, you're on your way to mastering lazy initialization and enhancing the performance of your Java applications.
Checkout our other articles