Mastering Autosizing for Tree Table Columns in JavaFX
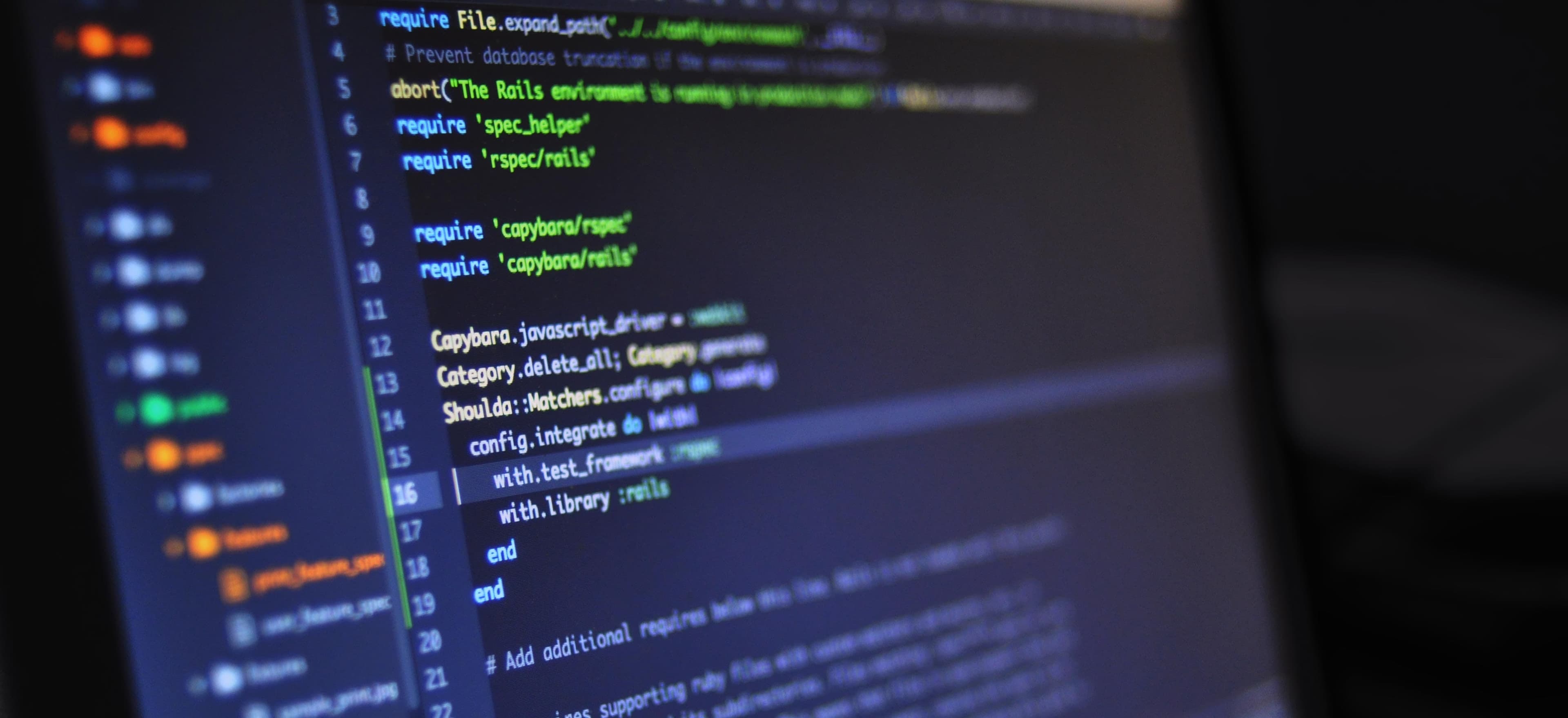
- Published on
Mastering Autosizing for Tree Table Columns in JavaFX
JavaFX is a powerful framework for building rich user interfaces, and one of its often-overlooked features is the TreeTableView
. This control allows you to display hierarchical data in a tabular format, making it an ideal choice for applications that require a structured view of complex datasets.
One of the challenges developers face when using TreeTableView
is determining an efficient way to size the columns based on the content they hold. In this blog post, we will delve into the concept of autosizing for tree table columns in JavaFX. We'll explore how to implement autosizing effectively, with clear code snippets and patterns that will help you create a polished and user-friendly application.
Understanding TreeTableView
Before we jump into autosizing techniques, let’s briefly understand what TreeTableView
is. A TreeTableView
is a type of table view that combines features of both a tree view and a table view. It allows for displaying hierarchical data in a tabular format. Each row can have a child node, which makes it suitable for presenting complex relationships between data entities, such as files in a directory structure or organizational charts.
Setting Up a TreeTableView
To get started, let’s set up a simple TreeTableView
in JavaFX to visualize some hierarchical data.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TreeItem;
import javafx.scene.control.TreeTableColumn;
import javafx.scene.control.TreeTableView;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class TreeTableViewExample extends Application {
@Override
public void start(Stage primaryStage) {
TreeTableView<String> treeTableView = new TreeTableView<>();
// Create the root node
TreeItem<String> rootNode = new TreeItem<>("Root");
treeTableView.setRoot(rootNode);
treeTableView.setShowRoot(true);
// Column definition
TreeTableColumn<String, String> column = new TreeTableColumn<>("Node");
column.setPrefWidth(200); // Set preference width for initial sizing
treeTableView.getColumns().add(column);
// Adding items
TreeItem<String> child1 = new TreeItem<>("Child 1");
TreeItem<String> child2 = new TreeItem<>("Child 2");
rootNode.getChildren().addAll(child1, child2);
VBox vBox = new VBox(treeTableView);
Scene scene = new Scene(vBox, 300, 250);
primaryStage.setScene(scene);
primaryStage.setTitle("TreeTableView Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of Code
- Root Node: We create a root node for our
TreeTableView
and set it to be visible. - Column Definition: A column is defined to display the nodes. We set an initial preferred width which we will modify later based on the content using autosizing.
- Adding Nodes: We add child nodes to the root node.
The Importance of Autosizing
Autosizing ensures that your tree table columns are optimally sized according to the content they display. This improves user experience significantly by preventing text truncation, making every piece of data visible at a glance. Automatic sizing can greatly enhance the readability of your UI.
Implementing Autosizing for Columns
Now, let’s implement the autosizing feature for the columns in our TreeTableView
. Below, we expand on our previous example by adding a method to perform autosizing based on the contents of the cells.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TreeItem;
import javafx.scene.control.TreeTableColumn;
import javafx.scene.control.TreeTableView;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class AutosizingTreeTableViewExample extends Application {
@Override
public void start(Stage primaryStage) {
TreeTableView<String> treeTableView = createTreeTableView();
autoSizeColumns(treeTableView); // Call autosizing method
VBox vBox = new VBox(treeTableView);
Scene scene = new Scene(vBox, 300, 250);
primaryStage.setScene(scene);
primaryStage.setTitle("Autosizing TreeTableView Example");
primaryStage.show();
}
private TreeTableView<String> createTreeTableView() {
TreeTableView<String> treeTableView = new TreeTableView<>();
TreeItem<String> rootNode = new TreeItem<>("Root");
treeTableView.setRoot(rootNode);
treeTableView.setShowRoot(true);
TreeTableColumn<String, String> column = new TreeTableColumn<>("Node");
treeTableView.getColumns().add(column);
// Adding items
rootNode.getChildren().addAll(new TreeItem<>("Child with long text"), new TreeItem<>("Child 2"));
return treeTableView;
}
private void autoSizeColumns(TreeTableView<String> treeTableView) {
for (TreeTableColumn<String, ?> column : treeTableView.getColumns()) {
column.setPrefWidth(-1); // Reset the preferred width
column.setMinWidth(50); // Set a sensible minimum width
// Calculate the ideal width
double width = 0;
for (TreeItem<String> item : treeTableView.getExpandedItemCount() > 0 ? treeTableView.getRoot().getChildren() : treeTableView.getRoot().getChildren()) {
String cellText = column.getCellData(item);
if (cellText != null && cellText.length() > width) {
width = cellText.length() * 7; // Approximate width calculation
}
}
column.setPrefWidth(width); // Set the preferred width based on calculated width
}
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of Autosizing Code
- Resetting Widths: We start by resetting the preferred width to a default so that we can calculate a new ideal width.
- Calculate Width: We iterate through all the items in the column. The width is determined based on the maximum string length of the texts displayed in that column, multiplied by an approximation factor (here we use
7
). This factor should be adjusted based on your font metrics for the best fit. - Setting Column Width: After determining the maximum width, we assign it back to the column's preferred width.
Further Enhancements
While the basics of autosizing are covered, you might want to add functionality to resize dynamically as data changes. By listening to events on your data model, you can call autoSizeColumns()
to keep the UI responsive and up-to-date.
For example, if you're using a ChangeListener
or ListChangeListener
, you can trigger a recalculation of the column widths whenever your hierarchical data changes.
yourObservableList.addListener((ListChangeListener<MyObject>) change -> {
autoSizeColumns(treeTableView);
});
Closing Remarks
In this blog post, we explored the TreeTableView
in JavaFX and implemented a robust autosizing mechanism for its columns. A well-sized TreeTableView
improves the overall user interface and enhances user experience significantly.
JavaFX offers great flexibility and power in creating compelling applications. If you want to dive deeper into the features JavaFX provides, check out the official JavaFX documentation.
Remember to explore different customization possibilities that suit the needs of your applications, and keep your interfaces clean and user-friendly. Happy coding!
Checkout our other articles