Common Pitfalls in Using Drools with Pure Java Rules
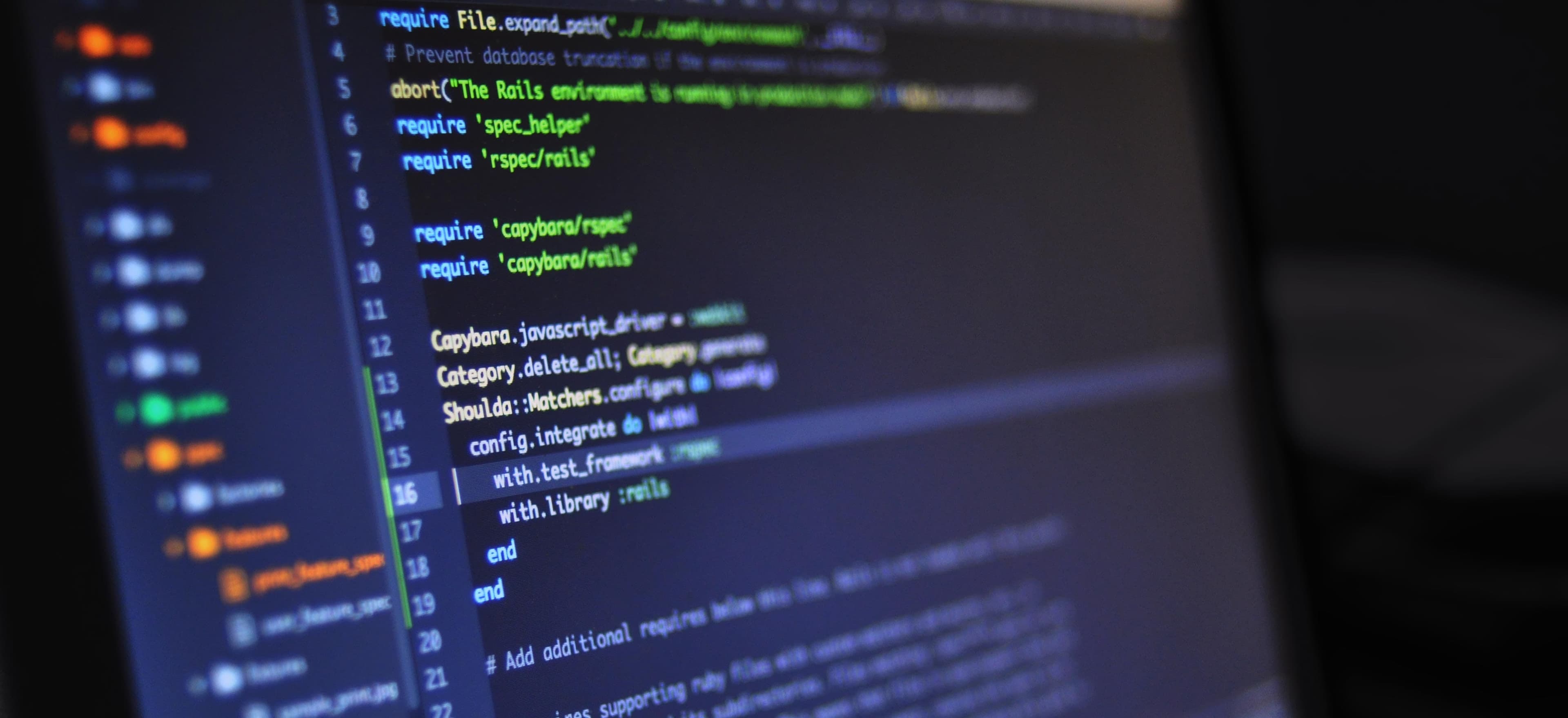
- Published on
Common Pitfalls in Using Drools with Pure Java Rules
In the world of business rules management systems (BRMS), Drools stands out as a powerful and flexible option. However, integrating Drools with pure Java rules can lead to some common pitfalls that developers should be wary of. In this article, we will explore these challenges, how to avoid them, and best practices for using Drools effectively in Java applications.
Getting Started to Drools
Drools is an open-source project that implements a production rule system using an inference-based agenda. It allows developers to define and manage complex business rules in a separate configuration file, which can be updated without changing the application code.
Understanding how to effectively integrate Drools with Java is crucial for developers looking to build scalable and maintainable applications. Although Drools offers numerous advantages, improper usage can lead to performance issues, increased complexity, and reduced maintainability.
Why Use Drools?
Before diving into the pitfalls, let’s briefly discuss why you might choose Drools for your Java application:
- Separation of Concerns: Keeping business logic outside of application code enhances maintainability.
- Dynamic Rule Changes: You can modify rules without redeploying your application.
- Advanced Matching Algorithms: Drools uses Rete algorithm which is highly efficient for large sets of rules.
However, effectively utilizing these advantages often comes with a learning curve.
Common Pitfalls
1. Overloading Rule Complexity
It can be tempting to write complex rules in one go, especially when dealing with intricate business logic. This leads to:
- Readability Issues: Code becomes hard to read and maintain.
- Performance Degradation: Complex rules can slow down the evaluation process.
Example: Overly Complex Rule Definition
Instead of one monolithic rule, consider breaking it down:
rule "Overly Complex Rule"
when
someConditionA
and someConditionB
and someConditionC
then
// Actions
end
Refactor to Multiple Readable Rules:
rule "Condition A Rule"
when
someConditionA
then
// Actions for A
end
rule "Condition B Rule"
when
someConditionB
then
// Actions for B
end
rule "Combined Actions Rule"
when
someConditionA
and someConditionB
then
// Combined Actions
end
Why Break It Down?
- Each rule can be tested individually.
- Easier to debug and understand.
- Improves the performance of the rule engine by allowing for quicker rule evaluations.
2. Ignoring Rule Activation Order
Drools operates on an agenda-based model. Rules can be activated in different orders based on their conditions and the salience you configure.
Example of Salience Misconfiguration:
When defining rules, the salience property can be used to prioritize rule execution:
rule "High Priority Rule" salience 100
when
someCondition
then
// High priority actions
end
Ignoring the setting can lead to unpredictable execution patterns.
Best Practice:
Always review and test the salience of your rules, especially when they have interdependencies. It ensures that the most critical rules execute first, leading to consistent behavior.
3. Not Leveraging Drools' Features
Drools comes with numerous features, such as:
- Global Variables: Share data across rules.
- Logical Queries: Process complex data queries easily.
Not using these features can lead to repetitive and inefficient code.
Example: Using Global Variables
global List<String> results;
rule "Example of Global Variable"
when
someCondition
then
results.add("This is a result");
end
Why Leverage Globals?
- Reduces redundancy.
- Keeps your rules focused on logic rather than data management.
4. Lack of Unit Testing
One of the most critical pitfalls is failing to properly test your rules. Like any code, your rules are subject to the same errors and complexities.
Best Practice:
Implement a suite of unit tests using Drools testing framework. You can utilize JUnit or similar libraries to ensure that your rules behave as expected.
@Test
public void testDroolsRules() {
// Setup Kie Session
KieSession kSession = kContainer.newKieSession("ksession-rules");
// Insert Facts
kSession.insert(new MyFact());
// Fire rules
kSession.fireAllRules();
// Assertions
assertEquals("Expected Result", ...);
}
5. Misunderstanding Rule Execution Context
Drools evaluates rules based on a specific context. This includes facts currently in the working memory, global variables, and activated rules. Mismanagement of these contexts leads to unexpected outcomes.
Example of Context Confusion:
In your rules, ensure that you understand the available facts at the time of execution.
rule "Contextual Rule"
when
$fact : MyFact(someProperty == "value")
then
// Perform actions based on current context
end
Why Context Matters:
- Logical errors may arise when assumptions about available facts differ from actual conditions.
- Ensuring clarity over context enables more predictable rule behavior.
Closing the Chapter
Drools is a robust system for managing complex business rules. However, improper usage or misunderstanding of its features can lead to maintenance headaches, performance issues, and unpredictable behavior. By avoiding common pitfalls—overloading rule complexity, ignoring activation order, underutilizing Drools features, neglecting testing, and misjudging the execution context—you can harness the full potential of Drools, ensuring a maintainable and efficient rule engine.
For further reading on Drools and its integration with Java, check out Drools Documentation and Effective Java for insights into best programming practices.
Implementing these strategies will not only hone your skills as a developer but also establish a solid foundation for building dynamic and robust Java applications using Drools. Happy coding!
Checkout our other articles