Choosing the Right Software Architecture for Your Project
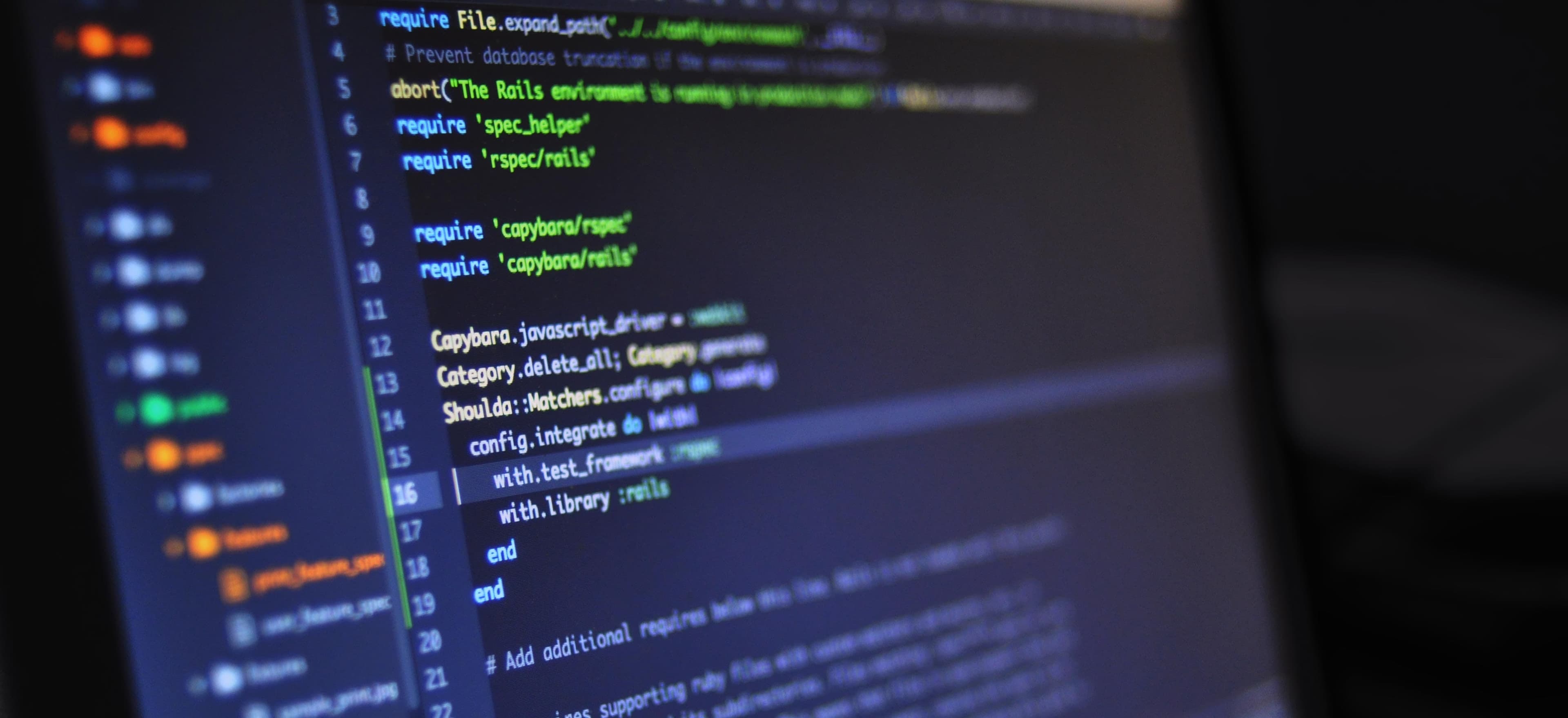
- Published on
Choosing the Right Software Architecture for Your Project
When embarking on a new software project, one of the most critical decisions you’ll face is selecting the appropriate software architecture. The architecture serves as a blueprint for both the development process and the system's future evolution, influencing everything from performance to maintainability. In this blog, we will explore the various software architecture styles, their implications, and how to choose the right one for your specific project needs.
What is Software Architecture?
Software architecture refers to the high-level structure of a software system. It encompasses the software components, their relationships, and the principles guiding its design and evolution. Establishing a sound architecture lays the foundation for both functionality and performance.
Key Factors Influencing Your Choice of Architecture
Before we dive into various architectural styles, it’s essential to understand the key factors that will influence your decision:
- Project Size and Complexity: Larger and more complex applications often require more sophisticated architectural patterns.
- Scalability: Consider whether your application will need to handle increased load over time and how the architecture supports scaling.
- Maintainability: Choose an architecture that will allow future developers to understand and modify the codebase easily.
- Team Skillset: Select an architecture that aligns with the technical expertise of your team.
- Time to Market: Sometimes, quicker solutions are necessary, making simpler architectures more appealing.
Common Software Architecture Patterns
Let’s look into various software architecture styles, starting from the simplest to more complex ones.
1. Monolithic Architecture
Monolithic architecture is a traditional model where all components of the application are tightly coupled into a single unit. This model facilitates simpler deployment routines and can be an excellent choice for smaller applications.
Code Example:
public class MonolithicApp {
public static void main(String[] args) {
UserService userService = new UserService();
userService.createUser("Alice", "password123");
// additional service calls...
}
}
class UserService {
public void createUser(String username, String password) {
// Logic to create a user
}
}
Why Use Monolithic Architecture?
- Simplicity: Developers can easily navigate through a single codebase.
- Performance: Calls between different modules don't incur network latency.
However, as the project grows, managing a monolithic application can become challenging due to its coupling.
2. Microservices Architecture
Microservices architecture breaks the application down into smaller, loosely coupled services. These services communicate over a network, typically using APIs. This architecture is ideal for large-scale applications requiring high scalability and frequent updates.
Code Example:
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
return new ResponseEntity<>(userService.createUser(user), HttpStatus.CREATED);
}
}
Why Use Microservices Architecture?
- Scalability: Individual services can scale independently.
- Flexibility: Different programming languages and frameworks can be used for different services.
However, it requires a robust infrastructure for managing communications, both for data consistency and service discovery.
3. Serverless Architecture
With serverless architecture, you can build and run applications without having to manage servers. You only pay for the compute time you consume. Popular platforms include AWS Lambda and Azure Functions.
Code Example:
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class Handler implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
return "Hello, " + input;
}
}
Why Choose Serverless Architecture?
- Cost-Effectiveness: You pay for actual usage, which can lead to cost savings.
- Reduced Operational Burden: No need for provisioning and managing servers.
However, you will need to consider cold starts and vendor lock-in issues.
4. Event-Driven Architecture
This architecture model is based on the production, detection, consumption of, and reaction to events. It’s suitable for applications that require immediate responses to various activities, such as payment processing systems.
Code Example:
public class EventProducer {
public void produceEvent(String event) {
// Logic to send the event to a message broker
}
}
Why Use Event-Driven Architecture?
- Decoupled Components: Systems can evolve independently while still being part of the same architecture.
- Real-time Processing: Events can be processed in real-time for rapid responses.
However, managing a distributed system can introduce its complexities, particularly regarding event handling and consistency.
How to Choose the Right Architecture for Your Project
Choosing the correct architecture isn't merely about understanding concepts; it involves a detailed analysis of your project requirements, team strengths, and future growth possibilities.
- Define Requirements: Gather functional and non-functional requirements.
- Evaluate Current Infrastructure: Analyze the existing systems to see what’s reusable and what needs to be changed.
- Consider Documentation and Support: Opt for architectures that have strong community support and extensive documentation.
- Prototyping: Build a prototype using a couple of architectures. Sometimes, hands-on testing will reveal the best approach.
- Future-Proofing: Think ahead. Can the architecture accommodate future changes and scaling needs?
Final Thoughts
Selecting the right software architecture is vital to the success of your project. Each architectural style comes with its own set of advantages and disadvantages. Whether you choose a monolithic, microservices, serverless, or event-driven architecture, make your decision based on thorough research, team capabilities, and project requirements.
Explore more about software architecture patterns through resources like Martin Fowler's website or Microsoft's cloud adoption framework to gain deeper insights.
By carefully weighing your options and adhering to your unique project needs, you can establish a solid architectural framework that supports your project both now and in the future.