Overcoming Code Overwhelm: 3 Effective Strategies
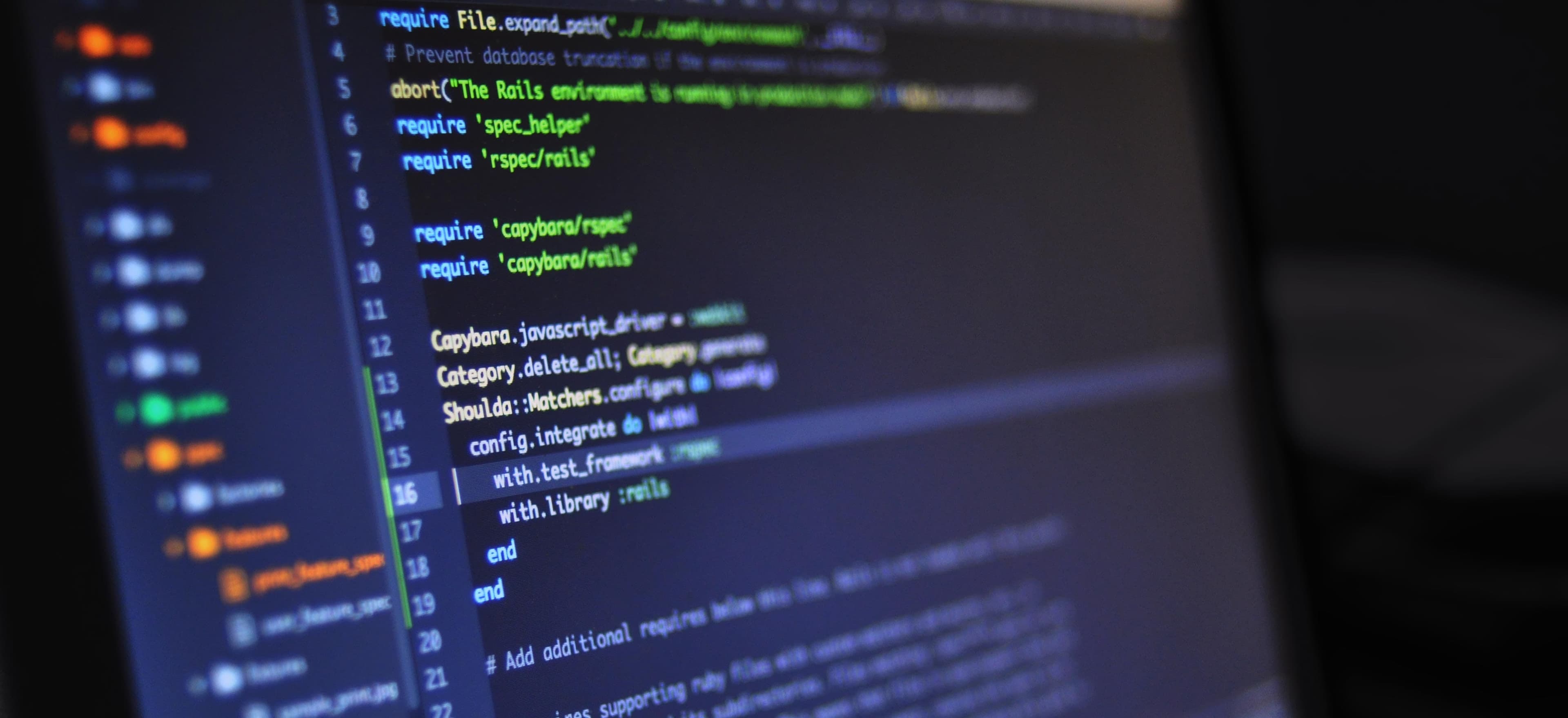
- Published on
Overcoming Code Overwhelm: 3 Effective Strategies
Java developers often encounter a sensation of code overwhelm, especially when dealing with large projects or complex codebases. Whether you are a novice programmer diving into your first project or a seasoned developer grappling with legacy systems, an abundance of code can be daunting. The good news is that there are effective strategies to manage and alleviate this stress.
In this post, we'll explore three effective strategies to help you overcome code overwhelm, ultimately enhancing your productivity and coding experience.
1. Break Code into Manageable Chunks
One of the most effective ways to tackle code overwhelm is to break down your project into smaller, manageable chunks. This approach is known as "modular programming," which involves dividing your code into distinct sections or modules.
Why Modular Programming?
By organizing your code into modules, you reduce cognitive load. Each module serves a specific purpose, making it easier to focus on one task at a time.
Example of Modular Programming:
Consider a simple Java application that performs user management. Instead of writing everything in a single file, you can create separate classes for each function.
// User.java
public class User {
private String username;
private String email;
public User(String username, String email) {
this.username = username;
this.email = email;
}
// Getters and setters
public String getUsername() {
return username;
}
public String getEmail() {
return email;
}
}
// UserService.java
import java.util.ArrayList;
import java.util.List;
public class UserService {
private List<User> users = new ArrayList<>();
public void addUser(User user) {
users.add(user);
}
public List<User> getUsers() {
return users;
}
}
In the example above, the User
class defines the user attributes, while the UserService
class manages user operations. This separation allows you to focus on user management without being overwhelmed by unrelated components.
For a deeper understanding of modular programming, check out this detailed guide.
2. Utilize Version Control Systems
Another effective method to overcome code overwhelm is to utilize version control systems (VCS) like Git. By integrating version control into your workflow, you can track changes, revert to previous versions, and branch out new features without fear of ruining existing functionality.
Why Use Version Control?
Version control allows you to experiment with your code and collaborate more effectively with team members. It creates a safety net, giving you the confidence to make changes without worrying about losing your work.
Basic Git Commands to Get Started:
Here are some essential Git commands that every developer should know:
# Initialize a new Git repository
git init
# Add files to the staging area
git add .
# Commit changes with a message
git commit -m "Initial commit"
# Create a new branch
git checkout -b feature/new-feature
# Merge changes from a branch
git checkout main
git merge feature/new-feature
# Push changes to a remote repository
git push origin main
The ability to create branches lets you isolate changes and focus on one feature at a time. Once completed, you can merge it back into the main branch when it’s ready. Explore more about version control with Git in this comprehensive tutorial.
3. Practice Test-Driven Development (TDD)
Test-Driven Development (TDD) is a software development process that relies heavily on testing before writing the actual code. This strategy encourages developers to create tests for their functions before implementing them.
Why Adopt TDD?
TDD not only helps to prevent bugs but also encourages a clearer understanding of the requirements of your code. You write tests for the expected outcomes, which provides a roadmap for your implementation. This process reduces feelings of overwhelm by clarifying what needs to be done.
Example of TDD in Java:
Let’s consider a simple example that demonstrates TDD:
- Write a Test:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
- Implement the Functionality:
public class Calculator {
public int add(int a, int b) {
return a + b; // Simple and clear
}
}
In the example, a test for adding two numbers is written first. The actual implementation follows the test. As a result, you gain clarity on what the add
method is supposed to do.
Conclusion on TDD:
Test-Driven Development facilitates a mindset shift; it encourages thinking about how your code will be used before you write it. To learn more about the nuances of TDD, visit Martin Fowler's detailed article.
The Last Word
Code overwhelm is a common experience for many developers, but it can be managed effectively through strategic practices. By breaking your code into manageable chunks, utilizing version control, and adopting Test-Driven Development, you can significantly reduce stress and enhance productivity.
Navigating the complexities of programming can be challenging, but with these strategies, you’ll find yourself equipped to tackle larger projects or intricate codebases with confidence.
Remember, coding is not just about writing lines of code; it's about creating a structured and sustainable developer experience. Happy coding!
Checkout our other articles