Mastering Concurrency: Avoiding Common Pitfalls
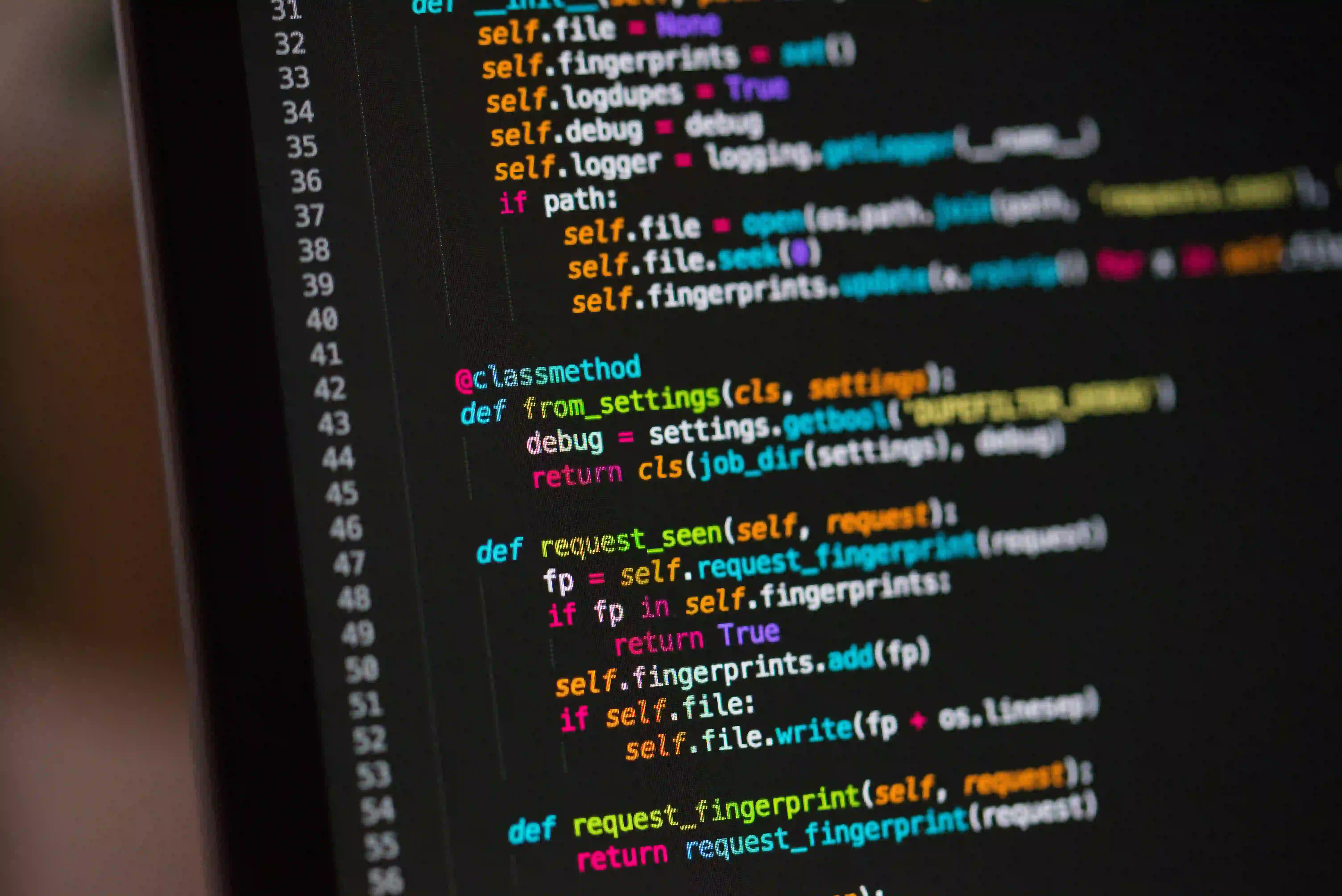
Mastering Concurrency: Avoiding Common Pitfalls
Concurrency in Java can be both powerful and challenging. By leveraging multiple threads, you can improve the efficiency and responsiveness of your applications, but it also introduces a suite of potential pitfalls. In this blog post, we will delve into common concurrency issues in Java and how to avoid them, providing code snippets to illustrate solutions and best practices.
Understanding Concurrency in Java
In Java, concurrency allows your program to execute multiple threads simultaneously. A thread is a lightweight process, and Java provides robust support for multithreading through the java.lang.Thread
class and the Runnable
interface. However, as you develop concurrent applications, you may encounter several issues, primarily related to shared resources and the unpredictable order of execution.
Common Pitfalls
-
Race Conditions
A race condition occurs when two or more threads access shared resources and try to change them simultaneously. The result depends on the timing of the threads, which can lead to inconsistent data.
Example
☕snippet.javapublic class Counter { private int count = 0; public void increment() { count++; // Not thread-safe } public int getCount() { return count; } }
In this example, if multiple threads call the
increment()
method, they may read and update thecount
variable simultaneously, resulting in an incorrect final count.Solution: Synchronization
To avoid race conditions, you can use the
synchronized
keyword.☕snippet.javapublic class Counter { private int count = 0; public synchronized void increment() { count++; } public int getCount() { return count; } }
By marking the
increment()
method assynchronized
, you ensure that only one thread can execute it at any given time, thus preventing concurrent modifications that may lead to errors. -
Deadlocks
A deadlock is a situation where two or more threads are waiting indefinitely for one another to release resources. This can halt your application and require a restart to resolve.
Example
☕snippet.javapublic class DeadlockExample { private final Object lock1 = new Object(); private final Object lock2 = new Object(); public void thread1() { synchronized (lock1) { try { Thread.sleep(100); } catch (InterruptedException e) { e.printStackTrace(); } synchronized (lock2) { // Critical section } } } public void thread2() { synchronized (lock2) { synchronized (lock1) { // Critical section } } } }
In this example,
thread1
lockslock1
and waits forlock2
, whilethread2
does the reverse, resulting in a deadlock.Solution: Lock Order
To resolve deadlock issues, maintain a strict order for acquiring locks.
☕snippet.javapublic void thread1() { synchronized (lock1) { synchronized (lock2) { // Critical section } } } public void thread2() { synchronized (lock1) { synchronized (lock2) { // Critical section } } }
With this approach, both threads will always acquire
lock1
beforelock2
, preventing deadlocks. -
Starvation
Starvation happens when a thread is perpetually denied access to the resources it needs for execution due to other threads consuming all available resources.
Example
☕snippet.javaclass StarvationExample { public void method() { // Thread that keeps acquiring lock synchronized (this) { while (true) { // Doing work } } } public void otherMethod() { synchronized (this) { // Less frequent access } } }
In this case, if the first thread continuously locks the resource, the second may never get a chance to execute.
Solution: Thread Priorities
Adjusting thread priorities may help, but it is not a guaranteed solution. A better approach is to ensure fair access to resources by utilizing Java’s
ReentrantLock
with a fair policy.☕snippet.javaimport java.util.concurrent.locks.ReentrantLock; class FairLockExample { private final ReentrantLock lock = new ReentrantLock(true); // fair public void method() { lock.lock(); try { // Critical section } finally { lock.unlock(); } } }
This helps in ensuring that threads get access to the shared resource in the order they requested it. You can read more about locks in Java Concurrency.
-
Livelocks
Livelock is similar to deadlock, but instead of threads being blocked, they continue to change state in response to each other without making progress.
Example
☕snippet.javapublic class LivelockExample { private final Object lock1 = new Object(); private final Object lock2 = new Object(); public void method1() { while (true) { synchronized (lock1) { // Avoid the other thread synchronized (lock2) { // Critical section } } } } public void method2() { while (true) { synchronized (lock2) { // Avoid the other thread synchronized (lock1) { // Critical section } } } } }
Both threads attempt to acquire locks and then back off when they detect contention, leading to an endless cycle without making any progress.
Solution: Back-off Strategy
A better strategy involves implementing a back-off algorithm, allowing threads to wait before retrying to acquire a lock.
☕snippet.javapublic void method() { while (true) { synchronized (lock) { // perform work break; // exit the loop once done } try { Thread.sleep(100); // Back-off } catch (InterruptedException e) { Thread.currentThread().interrupt(); } } }
-
Improper Use of Thread Pools
Thread pools are essential for managing concurrency efficiently. However, an improper configuration may lead to thread exhaustion or resource leaks.
Solution: Use Executors
Utilize the
Executors
framework to manage thread pools effectively.☕snippet.javaimport java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class ThreadPoolExample { public static void main(String[] args) { ExecutorService executor = Executors.newFixedThreadPool(5); for (int i = 0; i < 10; i++) { final int taskId = i; executor.submit(() -> { System.out.println("Task ID: " + taskId + " is executing."); }); } executor.shutdown(); } }
Here, an
ExecutorService
creates a thread pool of a specified number of threads. Tasks are submitted to the executor, which manages their lifecycle, ensuring efficient resource usage.
Closing Remarks
Mastering concurrency in Java is crucial for building efficient, responsive applications. Understanding and avoiding common pitfalls like race conditions, deadlocks, starvation, and improper thread management is essential.
By leveraging synchronization, following lock order principles, employing fair algorithms, and correctly configuring thread pools, you can mitigate these issues effectively. Always test your concurrent code thoroughly to ensure it behaves as expected under various conditions.
For more comprehensive insight on Java concurrency, consider exploring Java Concurrency in Practice by Brian Goetz, which delves deeper into concurrency patterns and solutions.
With these principles in hand, you are well on your way to mastering concurrency in Java. Happy coding!