Mastering Sequential TestNG Execution with DataProvider
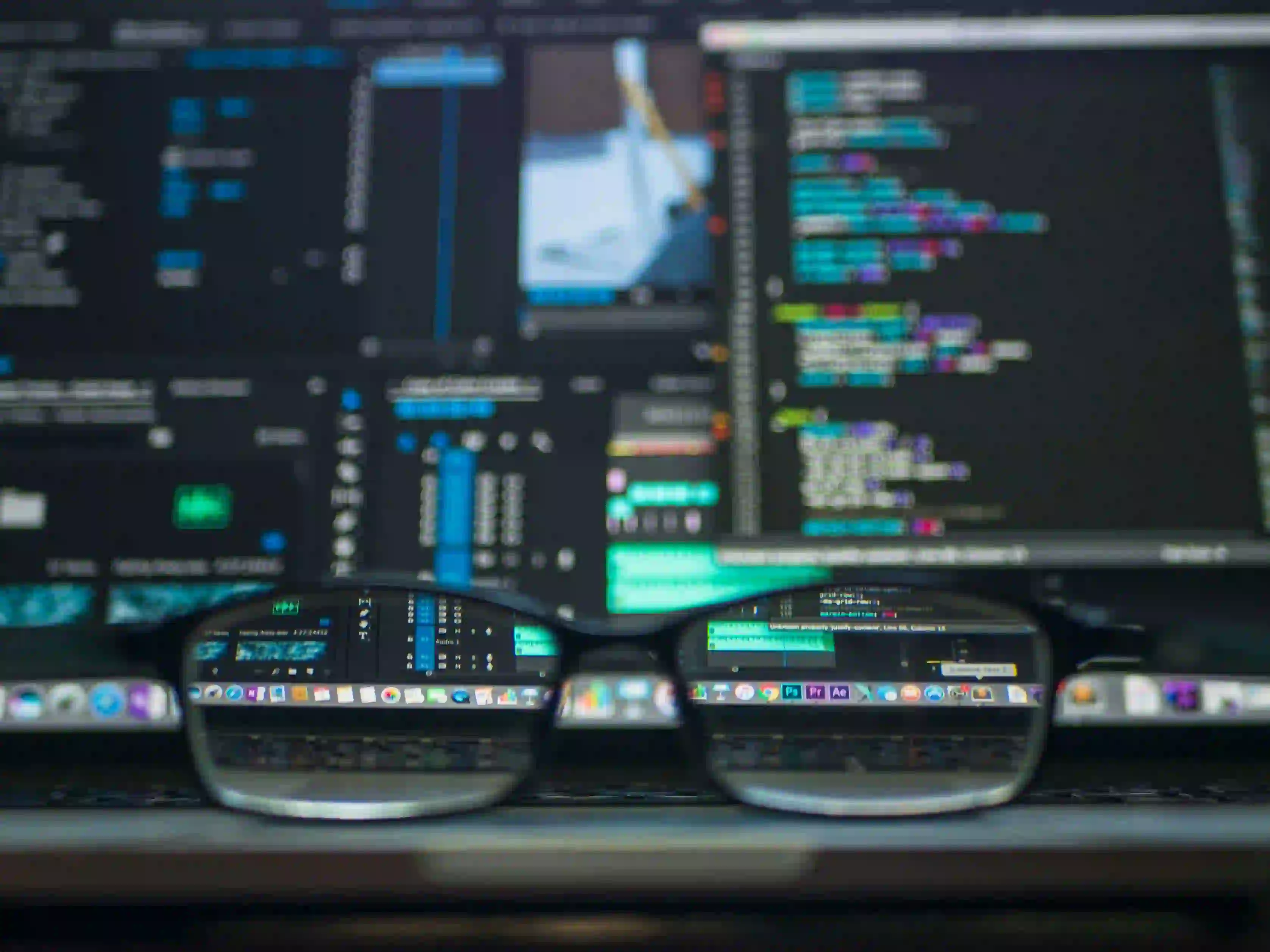
Mastering Sequential TestNG Execution with DataProvider
When it comes to automation testing in Java, TestNG stands out as one of the most comprehensive testing frameworks. It provides powerful tools for unit testing and includes features like annotations, grouping, parameterization, and reporting. One of the most critical functionalities in TestNG is the ability to run tests sequentially using the DataProvider
annotation. In this blog post, we’ll explore how to master sequential execution in TestNG with DataProvider.
What is TestNG?
TestNG (Test Next Generation) is inspired by JUnit and NUnit, but it introduces several new functionalities, making it more powerful and flexible. Key features of TestNG include:
- Annotations: Streamlining test configuration and execution.
- Parameterization: Facilitating dynamic inputs for test methods.
- DataProviders: Running a single test method multiple times with different input values.
- Test Order: Managing the execution order of tests.
This flexibility and control are essential in scenarios where the execution of one test is reliant on the successful completion of another.
The Need for Sequential Execution
In many testing scenarios, it's vital to maintain a specific order of execution. For instance:
- User login should occur before navigating to a profile page.
- Filling out a form before submitting it.
Running tests sequentially ensures that one test's output is the input for the next. This can lead to more reliable test results and easier debugging processes.
Setting Up TestNG in Your Project
Before diving into DataProvider
and sequential executions, let’s make sure TestNG is set up in your project. If you're using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
If you're not using Maven, you can download the TestNG JAR from TestNG’s official website and add it to your project’s build path.
Understanding DataProvider in TestNG
DataProvider
is one of the heavyweights of TestNG. It allows you to supply a test method with different data sets. This is immensely useful in sequential testing because it can encapsulate varying states for each subsequent test.
Example of Using DataProvider
Here's how to create a simple DataProvider
that supplies multiple sets of data to a test method sequentially.
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class SequentialTest {
@DataProvider(name = "userDataProvider")
public Object[][] userData() {
return new Object[][] {
{"user1", "pass1"},
{"user2", "pass2"},
{"user3", "pass3"}
};
}
@Test(dataProvider = "userDataProvider")
public void loginTest(String username, String password) {
System.out.println("Logging in with username: " + username + " and password: " + password);
// Insert login logic here (e.g., asserting login success)
}
}
Explanation of the Code
-
DataProvider Definition: The
userData()
method is annotated with@DataProvider
, allowing it to return an array of object arrays. Each inner array represents a set of parameters for theloginTest
method. -
Test Method: The
loginTest
method retrieves the username and password from theDataProvider
. For each set of data, the test will execute, thereby allowing a sequential flow. -
Logging In: In a real-world scenario, this method would contain assertions and logic to validate that a user has successfully logged in.
Executing Tests Sequentially
By default, TestNG executes test methods in parallel based on their configuration. To ensure sequential execution, configure your testng.xml
file:
<suite name="Sequential Tests" parallel="false">
<test name="Login Tests">
<classes>
<class name="SequentialTest"/>
</classes>
</test>
</suite>
Setting parallel
to false
ensures that all tests are executed in the order they appear.
Implementing a Sequential Workflow
Let’s expand our initial example into a complete sequential workflow. Suppose we want to log in a user and then navigate to their profile.
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class UserWorkflowTest {
@DataProvider(name = "userDataProvider")
public Object[][] userData() {
return new Object[][] {
{"user1", "pass1"},
{"user2", "pass2"},
{"user3", "pass3"}
};
}
@Test(dataProvider = "userDataProvider")
public void loginTest(String username, String password) {
// Simulating login operation
System.out.println("Logging in with username: " + username + " and password: " + password);
assert login(username, password); // Replace with actual login method
}
@Test(dependsOnMethods = "loginTest", dataProvider = "userDataProvider")
public void viewProfile(String username, String password) {
System.out.println("Viewing profile of user: " + username);
// Assert that the profile loads correctly
}
private boolean login(String username, String password) {
// Here add your actual login logic
return true; // Assume the login is always successful for simplicity
}
}
Explanation of the Sequential Workflow
-
Dependency: The
viewProfile
method has a dependency on theloginTest
. This means thatviewProfile
will only run afterloginTest
has executed successfully. -
Using DataProvider: Both methods utilize the same
DataProvider
, making it easy to manage test data while ensuring the sequential flow of tasks. -
Real-World Use Cases: In an actual application, you may want to replace the
login
method with a robust authentication procedure, including fetching data from a database.
In Conclusion, Here is What Matters
Mastering the DataProvider
in TestNG allows for comprehensive testing strategies including sequential execution. By understanding how to effectively utilize these features, testers can create more accurate and reliable test cases.
This not only enhances your testing framework but also makes it easier to debug issues that may arise from dependencies between tests. The next time you find yourself stuck with managing dependencies in your tests, consider leveraging TestNG's DataProvider
to streamline your workflow.
For more advanced concepts in TestNG, check out TestNG’s official documentation and explore how grouping tests can benefit your testing strategy.
Start implementing your sequential test execution today and elevate your automation testing to new heights!