Overcoming JavaFX Limitations in Fluent Design Progress Bars
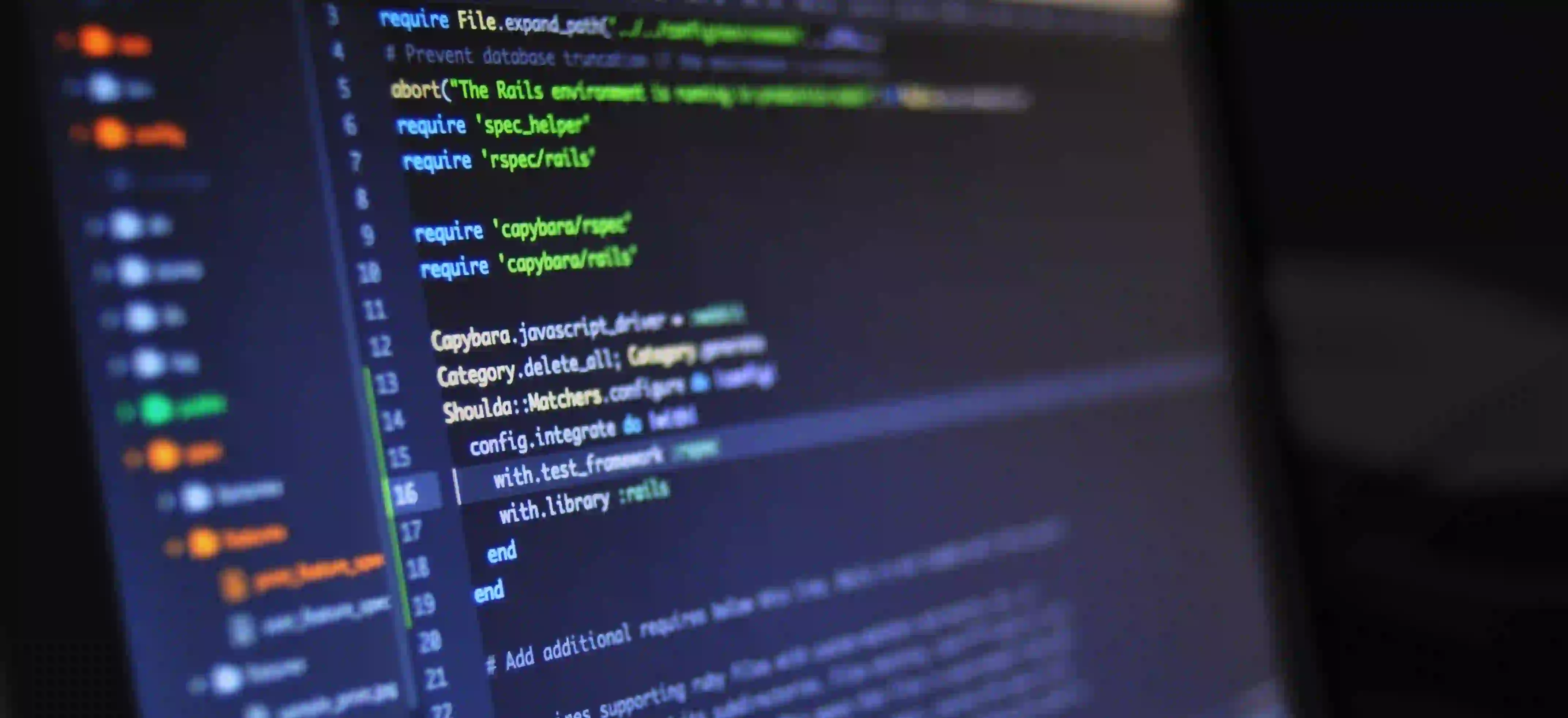
Overcoming JavaFX Limitations in Fluent Design Progress Bars
As we dive into the world of JavaFX, one of the most common challenges developers face is creating UI components that not only function well but also adhere to modern design trends. Fluent Design, conceptualized by Microsoft, incorporates layering, depth, motion, and responsive design which can be tricky to implement in JavaFX. Progress bars are essential UI elements that often lack the flexibility demanded by modern applications. This blog post will highlight strategies for overcoming the limitations of JavaFX in developing aesthetically pleasing and functional Fluent Design progress bars.
Understanding JavaFX UIs
JavaFX is a robust framework for creating rich internet applications in Java. However, it can be limited when it comes to following a specific design aesthetic. Native JavaFX components often do not provide the flexibility needed for a beautifully designed progress bar. To understand how to work around these limitations, let's look at the primary aspects that make up a progress bar.
Progress Bar Basics
At its core, a progress bar conveys loading or completion status to the user. In JavaFX, a basic progress bar can be created using the ProgressBar
class. The underlying functionality is simple, but achieving a Fluent Design aesthetic requires custom styling and behavior.
Creating a Basic JavaFX Progress Bar
Here's a basic implementation of a JavaFX progress bar:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ProgressBar;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class BasicProgressBar extends Application {
@Override
public void start(Stage primaryStage) {
ProgressBar progressBar = new ProgressBar();
progressBar.setProgress(0.5); // 50% completion
StackPane root = new StackPane(progressBar);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("Basic Progress Bar");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the code above, we establish a simple progress bar that is filled to 50%. While functional, it lacks the polish expected from modern UI designs.
Limitations of JavaFX Progress Bars
1. Lack of Customization
JavaFX's built-in progress bar does not support advanced stylistic adjustments easily. You may find that when you want a sleek, rounded, or gradient-style progress bar, you hit a wall.
2. Visual Feedback
For modern applications, visual feedback is essential. Using motion and changes in depth enhances the user experience, which the default JavaFX progress bar lacks.
Overcoming Limitations with Custom Styling
Styling with CSS
To create an engaging Fluent Design progress bar, using CSS for styling is a primary approach. JavaFX allows for external stylesheets, enabling developers to apply custom styles effortlessly.
Example: Custom CSS for a Progress Bar
Here’s a simple example of how to style a progress bar using CSS:
.progress-bar {
-fx-background-color: transparent; /* No background */
}
.progress-bar > .bar {
-fx-background-color: linear-gradient(to right, #0078D7, #00BFFF); /* Gradient effect */
-fx-background-radius: 10; /* Rounded corners */
}
Now to use this CSS in your JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ProgressBar;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class StyledProgressBar extends Application {
@Override
public void start(Stage primaryStage) {
ProgressBar progressBar = new ProgressBar();
progressBar.setProgress(0.5); // 50% completion
progressBar.getStyleClass().add("progress-bar"); // Apply custom CSS class
StackPane root = new StackPane(progressBar);
Scene scene = new Scene(root, 300, 200);
scene.getStylesheets().add("style.css"); // Link to CSS file
primaryStage.setTitle("Styled Progress Bar");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Important Notes on Custom CSS
- The
-fx-background-color
property allows for transparent backgrounds, crucial for layering effects. - Use
linear-gradient
to create smooth transitions between colors, enabling a modern feel. - Adjust the
-fx-background-radius
property to add rounded corners, consistent with Fluent Design principles.
Implementing Motion and Depth
Achieving the Fluent Design vision is not only about appearance. Adding a sense of motion and depth can significantly enhance user experiences. This can be achieved using transitions in JavaFX.
Example: Adding Animation to Progress Bar
JavaFX provides robust capabilities for animation. Here’s an example of how to add a simple animation to a progress bar.
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ProgressBar;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class AnimatedProgressBar extends Application {
private double progress = 0;
@Override
public void start(Stage primaryStage) {
ProgressBar progressBar = new ProgressBar(0); // Start at 0
AnimationTimer timer = new AnimationTimer() {
@Override
public void handle(long now) {
if (progress < 1) {
progress += 0.01; // Increment progress
progressBar.setProgress(progress);
} else {
stop(); // Stop when complete
}
}
};
timer.start();
StackPane root = new StackPane(progressBar);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("Animated Progress Bar");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Key Considerations for Animation
- Animation should be smooth and not intrusive. Continuous updates (as shown) create a visual signal that work is being done.
- Consider user experience—too much motion can be distracting.
Wrapping Up
Implementing Fluent Design elements into JavaFX progress bars is not without its challenges, but utilizing CSS for stylization and JavaFX’s animation capabilities can yield stunning results. By customizing components to suit design aesthetics, developers can provide better visual feedback to users.
Additional Resources
- JavaFX CSS Reference Guide
- Fluent Design System
- JavaFX Animation Documentation
Incorporating these practices into your JavaFX applications not only enhances functionality but importantly uplifts user experience and satisfaction. Fondly nodding to the principles of Fluent Design does not just make applications look modern; it ensures they feel that way too. Happy coding!