Understanding ThreadLocal Memory Leaks in Java
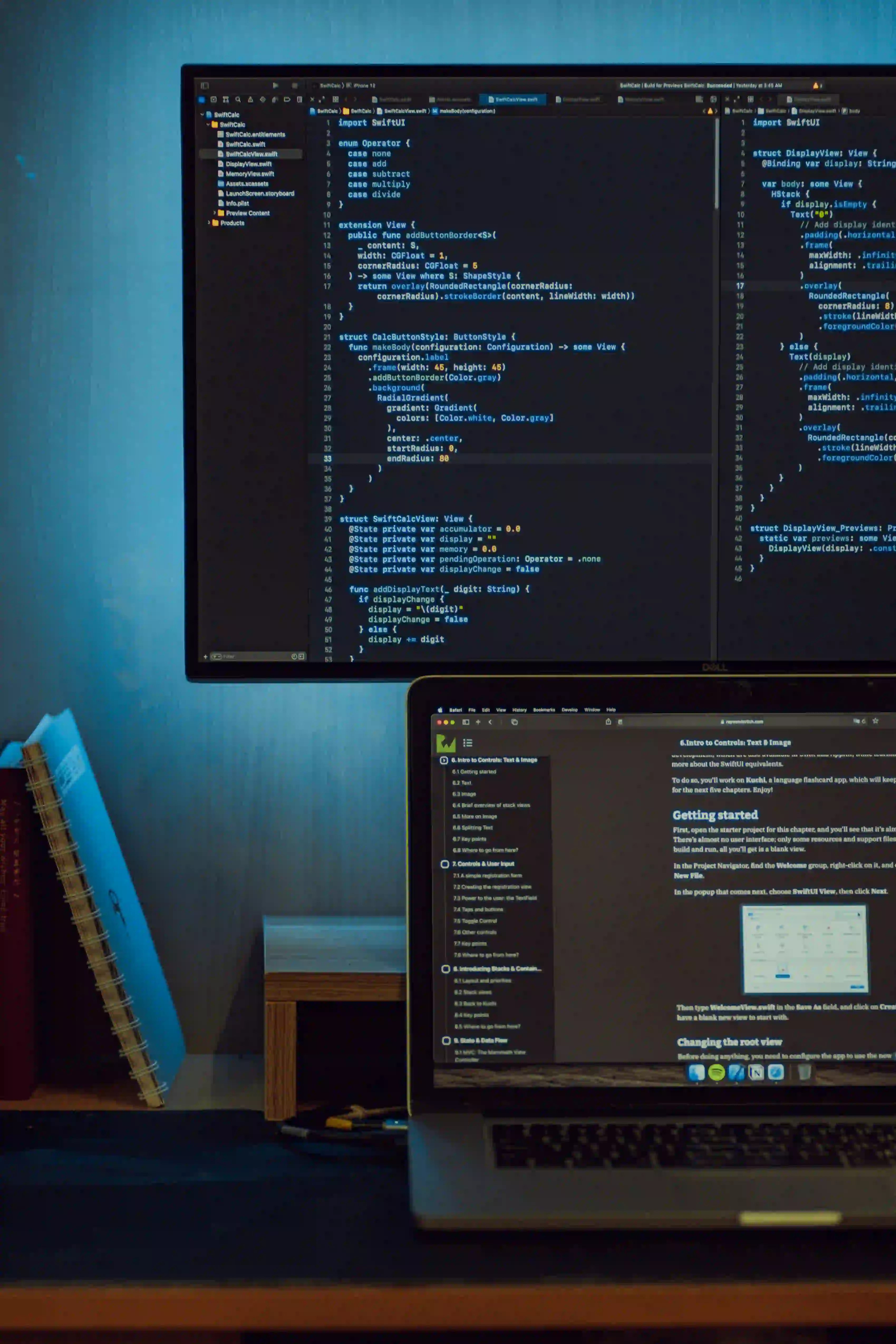
Understanding ThreadLocal Memory Leaks in Java
In the realm of Java programming, understanding memory management is key to creating scalable, efficient applications. One often overlooked aspect is the use of ThreadLocal
variables, which can lead to subtle and hard-to-track memory leaks. In this blog post, we will delve into what ThreadLocal is, why it is both useful and dangerous, and how to properly manage it to avoid memory leaks.
What is ThreadLocal?
ThreadLocal
is a Java construct that provides thread-local variables. Each thread accessing such a variable has its own, independently initialized copy of the variable. It is an effective way to maintain user session data without resorting to synchronization.
ThreadLocal<String> userContext = ThreadLocal.withInitial(() -> "Default User");
In the above example, a ThreadLocal variable named userContext
is created. Each thread that accesses this variable will see its own value, initialized to "Default User" if not set otherwise.
Why Use ThreadLocal?
Using ThreadLocal
can significantly simplify the handling of per-thread variables in a concurrent environment. Here are some reasons why developers use ThreadLocal:
-
Performance: Since the variable is inherently thread-safe and doesn't require synchronization for every access, it can improve performance in multithreaded applications.
-
Simplicity: It simplifies passing state during method calls. This avoids the "ThreadContext" pattern and cleans up method signatures.
-
Memory Isolation: The thread-local variables are not shared between threads, which can prevent unintended side effects.
However, with great power comes great responsibility. Improper handling of ThreadLocal variables can lead to memory leaks.
Understanding Memory Leaks with ThreadLocal
Memory leaks occur when the memory that is no longer needed is not released. In Java, this can happen with ThreadLocal when threads are managed improperly, especially in web applications.
How ThreadLocal Can Lead to Memory Leaks
Consider this scenario: you have a web server where new threads are spawned to handle incoming requests. These threads might use ThreadLocal variables to store user session information.
public class RequestProcessor implements Runnable {
private static ThreadLocal<UserSession> userSession = ThreadLocal.withInitial(UserSession::new);
@Override
public void run() {
// Access the user session
UserSession session = userSession.get();
// process the request
// We will *not* clean up the ThreadLocal after processing
}
}
In this example, the userSession
variable will hold a reference to the UserSession
object for every thread that accesses it. If the server or application does not properly clean up this reference when the thread completes its task, it leads to an accumulation of objects in memory. When threads get reused (as in a thread pool), the old references remain, leading to a memory leak.
What Happens During ClassLoader Leaks?
In web applications, the ThreadLocal
variable may also hold references to objects that are tied to a particular ClassLoader
. When a web application is undeployed or redeployed, if a thread holds a reference to a ThreadLocal variable, this can prevent the ClassLoader
from being garbage collected.
private static ThreadLocal<MyService> serviceThreadLocal = new ThreadLocal<>();
If MyService
instances are dependent on classes loaded by the web application's ClassLoader, the aforementioned scenario can lead to leaks that are difficult to debug.
Avoiding ThreadLocal Memory Leaks
- Explicit Cleanup: Always invoke
remove()
on a ThreadLocal variable when it is no longer needed. This is especially crucial in thread pools or web applications.
public void processRequest() {
try {
UserSession session = userSession.get();
// Process your session here
} finally {
userSession.remove(); // Ensure cleanup happens
}
}
- Custom Thread Factory: When using a thread pool, consider creating a custom thread factory that will clean up your ThreadLocal variables. This is particularly effective when using frameworks like Spring.
public class CustomThreadFactory implements ThreadFactory {
@Override
public Thread newThread(Runnable r) {
return new Thread(r) {
@Override
public void run() {
try {
super.run();
} finally {
userSession.remove();
}
}
};
}
}
-
Weak References: Although it is more complex, in certain scenarios, consider using
WeakReference
with ThreadLocal if you want to avoid memory leaks due to the ThreadLocal's strong reference. This should be approached with caution and is generally not the common practice. -
Use with Care: Lastly, assess the need for ThreadLocal. Often the use cases can be effectively managed using other state management techniques, such as Dependency Injection.
To Wrap Things Up
ThreadLocal
is a powerful resource when utilized correctly but it carries the risk of leaking memory, impacting application performance and scalability. By understanding how to manage ThreadLocal variables effectively and cleaning up after their use, developers can take advantage of their benefits while avoiding the pitfalls.
For deeper insights into memory management in Java, consider reviewing the official Java documentation. Moreover, if you want to dive deeper into the commonly encountered issues in memory management, you might find Java Memory Management a worthwhile read.
By ensuring that we handle ThreadLocal properly, we not only maintain application performance but also enhance the robustness of our Java applications. Keep these considerations in mind as you explore Java's rich concurrency features. Happy coding!