Costly Consequences of Overusing Code Reuse
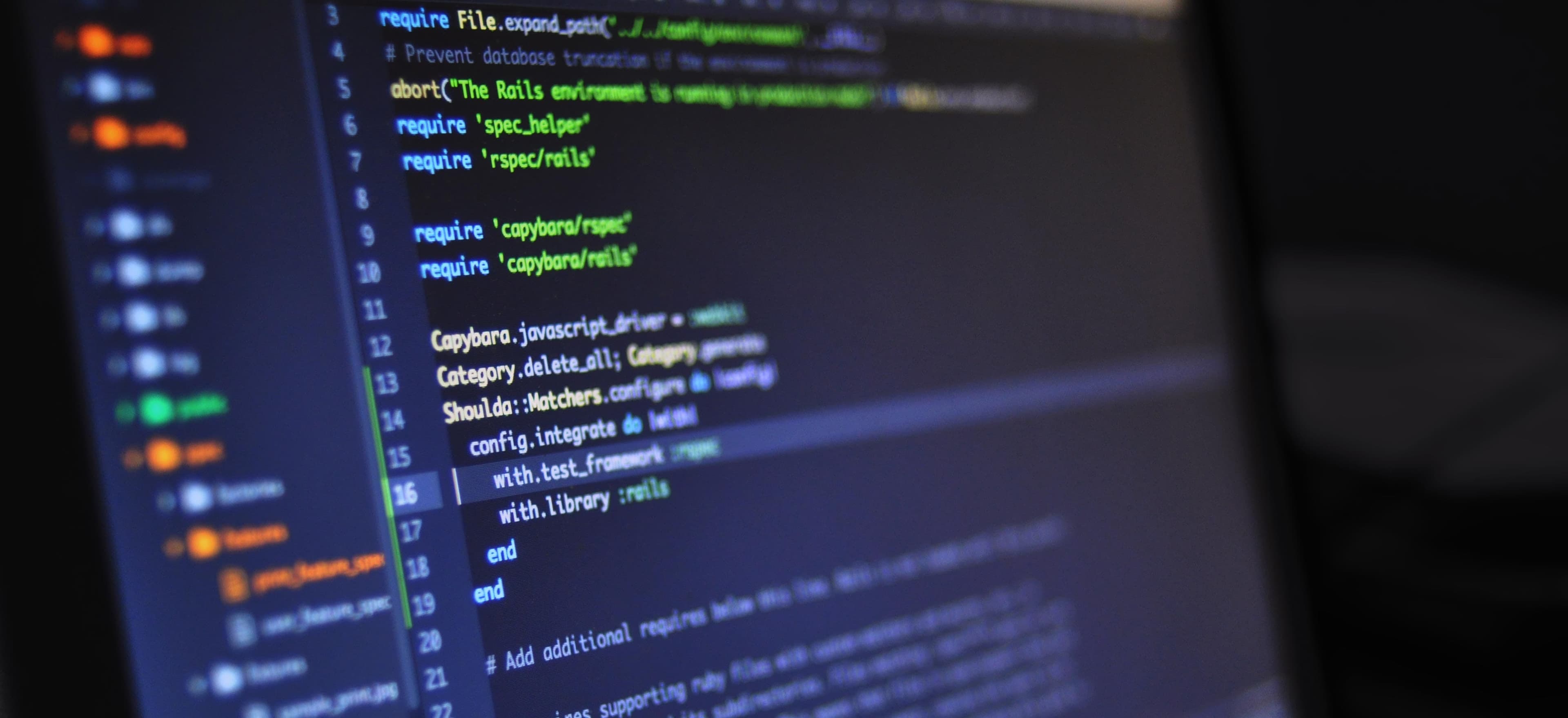
- Published on
Costly Consequences of Overusing Code Reuse in Java
In the world of software development, code reuse is often hailed as a best practice. The idea is simple: why reinvent the wheel? However, while code reuse can bring about efficiency and reduce redundancy, overdoing it can lead to significant pitfalls. In this blog post, we'll explore the detrimental impacts of excessive code reuse, particularly within the Java programming language. Additionally, we will delve into some balanced approaches that can help you maintain code quality and robustness.
Understanding Code Reuse
Code reuse refers to the practice of using existing code for new functions or applications instead of creating new code from scratch. In Java, it can take many forms, including:
- Inheritance - Utilizing parent classes to share functionalities.
- Interfaces - Defining methods that can be implemented by multiple classes.
- Libraries and Frameworks - Using pre-built code from external sources.
Although these strategies can save time and avoid redundancy, they can also have unintended consequences.
Drawbacks of Code Reuse
-
Tight Coupling When code is reused without careful consideration, it can lead to tight coupling. This means that different modules of your application become heavily dependent on each other. Tight coupling can make your codebase fragile and challenging to maintain.
class Vehicle { void startEngine() { // Engine starting code } } class Car extends Vehicle { void drive() { startEngine(); // Tight coupling with Vehicle class } }
In the example above, if changes are made to the
Vehicle
class, you may inadvertently break theCar
class, leading to a cascading set of issues. -
Increased Complexity Over-relying on inherited code can often lead to complicated class hierarchies that are difficult to decipher. As the architecture becomes dense, debugging and testing become increasingly cumbersome.
-
Higher Maintenance Costs While it might save time initially, maintaining reused code often incurs additional costs. If a bug found in a shared utility function affects multiple modules, it may require refactoring across several areas of the application.
-
Breaking Changes External libraries can change or become deprecated. Continued reliance on these external codes means you might have to deal with breaking changes that could affect the core functionalities of your application.
Example of Breaking Changes
Consider a scenario where a Java library’s function signature changes:
// Original function in external library
public void performTask(String taskName) {
// Implementation
}
// New version of external library
public void performTask(String taskName, int priority) {
// Implementation
}
If your code relies on the old signature, it will result in runtime errors. Keeping track of these changes can be difficult, especially when you are dealing with multiple dependencies.
Addressing the Challenges of Code Reuse
Now that we’ve discussed the problems tied to overusing code reuse, how can we address these challenges while still leveraging the benefits? Here are some practical strategies:
1. Favor Composition over Inheritance
Composition encourages building complex types by combining simpler ones rather than creating a rigid class hierarchy that often results from inheritance.
class Engine {
void start() {
// Engine starting code
}
}
class Car {
private Engine engine = new Engine();
void drive() {
engine.start(); // Using composition
}
}
In this example, Car
simply contains an Engine
. This decouples the two classes and allows changes to be made independently, improving flexibility and maintainability.
2. Use Interfaces Wisely
Interfaces can provide a flexible way to promote code reuse. However, be mindful of overuse. Limit the number of methods in your interfaces to maintain clarity.
interface Drivable {
void drive();
}
class Car implements Drivable {
public void drive() {
// Drive implementation
}
}
class Bicycle implements Drivable {
public void drive() {
// Drive implementation
}
}
By keeping your interfaces simple and focused, you reduce tight coupling and streamline class implementations.
3. Code Reviews and Refactoring
Regular code reviews can help identify areas where code reuse may be overdone. During these reviews, focus on the following:
- Redundant Code: Code that performs the same function multiple times can often be refactored into a single utility method.
- Common Patterns: Usage of design patterns can also promote code reuse while maintaining flexibility and clarity.
Refactoring is key to maintaining a healthy codebase. For more on clean code practices, check out Clean Code Principles.
Final Considerations
While code reuse is undoubtedly a powerful tool in software development, engineers must approach it carefully. The consequences of overusing this practice can range from tight coupling and increased complexity to escalated maintenance costs. By favoring composition over inheritance, using interfaces judically, and regularly conducting code reviews, you can strike a balanced path towards effective code reuse.
Remember, the goal is not to reduce duplication at all costs, but to enhance the overall quality and maintainability of your code.
Next time you’re tempted to reuse code indiscriminately, think twice. Sometimes the new code is just what you need to keep your codebase clean and efficient.
Additional Resources
For developers looking for further insights into managing code reuse, consider checking:
- Effective Java - A comprehensive guide that offers best practices for coding in Java.
- Refactoring Guru - A resource for learning about code refactoring and design patterns.
By keeping these principles in mind, you can enjoy the benefits of code reuse without falling victim to its pitfalls. Happy coding!