Why You Should Stop Using Java's Finalizer Today
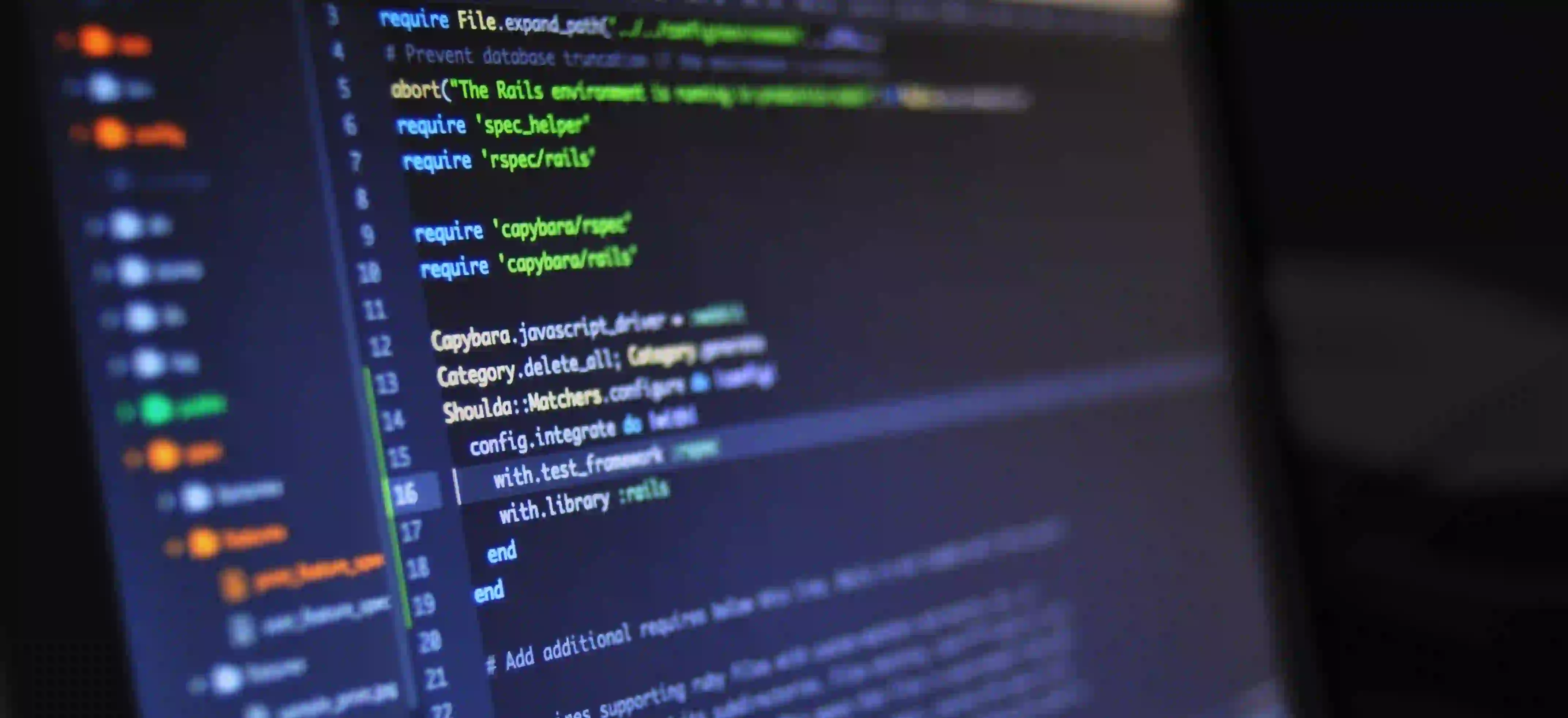
Why You Should Stop Using Java's Finalizer Today
Java, a language synonymous with portability, consistency, and reliability, has several features that developers have used over the years. One such feature is the finalizer method (finalize()
). Although it offers a way to perform cleanup operations on objects, this mechanism has become a source of complexity and inefficiency in Java applications. In this blog post, we will discuss why you should stop using Java's finalizer today and the alternatives that you can embrace for proper resource management.
What is a Finalizer?
A finalizer is a special method in Java, defined in the java.lang.Object
class, that allows you to clean up resources that an object is holding before it is removed from memory. When an object is about to be garbage collected, the JVM calls its finalize()
method. Here is a simple example:
public class Resource {
@Override
protected void finalize() throws Throwable {
try {
// Cleanup resources like closing a file or releasing a connection
System.out.println("Finalizing Resource");
} finally {
super.finalize();
}
}
}
In this code snippet, we override the finalize()
method to perform necessary cleanup operations. However, the complexity and unpredictability associated with using finalizers can outweigh their benefits.
The Problems with Finalizers
1. Unpredictability of Execution
You cannot predict when or even if the finalize()
method will be called. Finalizers run on a separate thread and are subject to the JVM's garbage collection schedule. Thus, it's not guaranteed that resources will be released promptly.
For instance, consider a scenario where a database connection is not released until the garbage collector decides to run. This may lead to resource exhaustion, particularly in systems with limited available connections.
2. Increased Pauses During Garbage Collection
The presence of finalizers incurs additional overhead. The garbage collector has to keep track of objects with finalizers and invoke their cleanup methods, which adds to the time taken for garbage collection. This can lead to noticeable pauses in your application's performance.
In high-performance applications, such as web servers, latency can impact user experience significantly. According to Oracle's documentation, the added complexity from finalizers can lead to longer GC pauses.
3. Complexity with Exceptions
If an exception occurs within a finalizer, it can prevent the object from being finalized properly. This can lead to other resources going unused or unreleased, further deteriorating the application's efficiency. Java's design does not provide a mechanism to recover from such exceptions, which can leave your application in an unstable state.
4. Memory Leaks
Most disturbingly, objects that hold references to other objects may avoid garbage collection if the finalizer is not executed. For example:
public class MemoryLeak {
private Object resource;
public MemoryLeak(Object resource) {
this.resource = resource;
}
@Override
protected void finalize() throws Throwable {
try {
resource = null; // Attempt to release the resource
} finally {
super.finalize();
}
}
}
In this scenario, if the finalize()
method is unable to run, the resource
object will never be cleared, leading to a memory leak.
Alternatives to Finalizers
Instead of relying on the finalize method, Java developers can use several better alternatives for managing resources:
1. Try-With-Resources Statement
The try-with-resources statement simplifies resource management by ensuring that each resource is closed at the end of the statement. It is available in Java 7 and later versions and is particularly useful for working with IO classes like FileInputStream
, BufferedReader
, etc.
Here is an example:
import java.io.*;
public class ResourceManagement {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this code, the BufferedReader
is automatically closed at the end of the try block, eliminating the risk of resource leaks.
2. Explicit Resource Management
For critical scenarios where you need more control, consider explicit resource management through manual close()
or dispose()
methods. This pattern requires you to define a method to release resources and always make sure it's called.
public class CustomResource {
private boolean isOpen;
public void open() {
isOpen = true;
}
public void close() {
if (isOpen) {
System.out.println("Cleaning up resources");
isOpen = false;
}
}
}
Call close()
when you're done with the resource, and ensure you handle it in a finally
block if necessary.
3. Use PhantomReferences
Java offers PhantomReferences
as a part of the reference hierarchy that can be useful for managing memory cleanups without the drawbacks of finalize. They allow you to execute code after an object has been garbage collected. However, they should be used with caution as they introduce complexity and may not be necessary in many scenarios.
Final Thoughts
Finalizers may have been a convenient feature in early Java development, but their drawbacks far outweigh their advantages today. From unpredictable execution to potential memory leaks and performance issues, the risks are considerable.
By using alternatives such as the try-with-resources statement and explicit resource management, you can write cleaner, more efficient, and more predictable Java code. For more information about resource management in Java, check out Java Documentation on try-with-resources and learn how to enhance your coding practices.
Stop relying on finalizers today – embrace cleaner, safer, and more efficient resource management techniques in your Java applications!