Debugging CompletableFuture: Tackling Asynchronous Timeouts
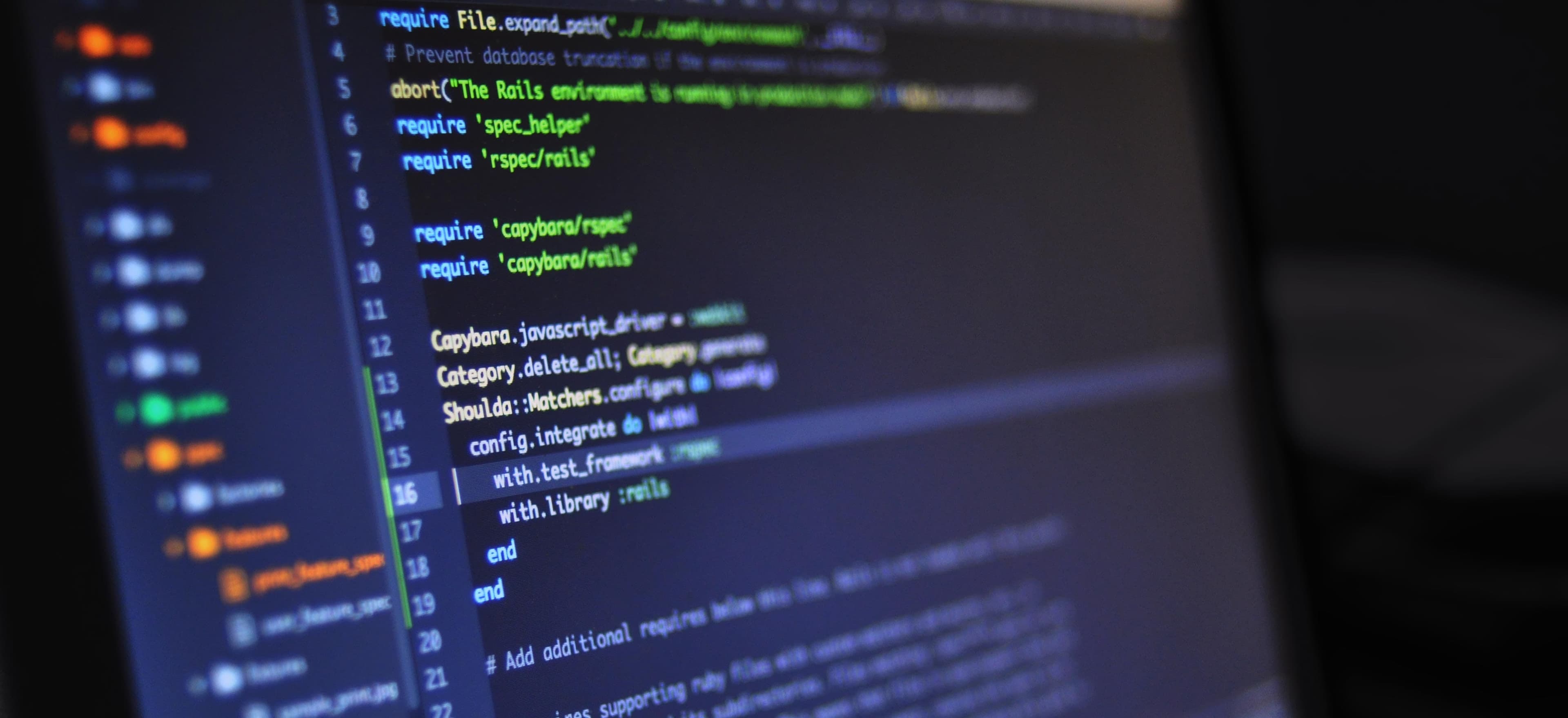
- Published on
Debugging CompletableFuture: Tackling Asynchronous Timeouts
In the realm of Java programming, one cannot underestimate the importance of concurrency and parallelism. With the rise of multi-core processors, asynchronous programming has become an essential part of modern Java applications. Among its plethora of features, Java's CompletableFuture
is a powerful tool that simplifies asynchronous programming, allowing developers to write non-blocking code with ease. However, working with CompletableFuture
can present its own set of challenges, particularly when dealing with timeouts. In this blog post, we will explore debugging strategies for handling asynchronous timeouts using CompletableFuture
.
Understanding CompletableFuture
Before diving deeper, let's understand what CompletableFuture
is. It is part of the Java 8 java.util.concurrent
package, which provides a way to work with asynchronously executed tasks.
The key features of CompletableFuture
include:
- Non-blocking execution: You can chain actions that will execute upon completion.
- Error Handling: You can handle exceptions gracefully.
- Combining futures: You can combine multiple futures using methods like
thenCombine
,thenCompose
, etc.
Here's a simple example of creating a CompletableFuture
:
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating a long-running task
try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); }
return "Hello, World!";
});
future.thenAccept(result -> System.out.println(result));
}
}
Explanation:
supplyAsync
schedules a task that simulates a long-running operation.- The
thenAccept
method processes the result once the computation is complete.
The Challenge of Timeouts
As with any asynchronous operation, there can be scenarios where a task may take longer than expected. This could be due to network latency, external service slowness, etc. In such cases, implementing a timeout mechanism becomes necessary.
Setting Timeout
To set a timeout on a CompletableFuture
, we can use the completeOnTimeout
method. This allows us to specify a fallback value if the future does not complete in the designated time.
Here’s an example:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.TimeUnit;
public class TimeoutExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating a long-running task
try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); }
return "Task completed";
});
String result = future
.completeOnTimeout("Timeout occurred", 3, TimeUnit.SECONDS)
.join();
System.out.println(result); // Output will be "Timeout occurred"
}
}
Explanation:
- In this case, if the computational task does not complete within 3 seconds, the fallback value "Timeout occurred" will be used.
Debugging Timeout Issues
While the completeOnTimeout
method is helpful, it is crucial to debug any issues that lead to timeouts. Here are some strategies for effectively debugging CompletableFuture
timeouts:
1. Logging
Adding logging statements provides insights into the flow of execution. By logging the start and end of tasks, we can ascertain whether they are taking more time than expected.
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.TimeUnit;
import java.util.logging.Logger;
public class LoggingExample {
private static final Logger logger = Logger.getLogger(LoggingExample.class.getName());
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
logger.info("Task started");
try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); }
logger.info("Task completed");
return "Task Result";
});
String result = future
.completeOnTimeout("Timeout occurred", 3, TimeUnit.SECONDS)
.join();
logger.info("Result: " + result);
}
}
2. Thread Pool Configuration
The configuration of thread pools can significantly impact how fast tasks complete. If the number of concurrent tasks exceeds the thread pool size, tasks may be queued, resulting in timeouts.
Here’s an example of adjusting the thread pool with Executors
:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class ThreadPoolExample {
private static final ExecutorService executor = Executors.newFixedThreadPool(2);
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
try { Thread.sleep(6000); } catch (InterruptedException e) { e.printStackTrace(); }
return "Long-running result";
}, executor);
String result = future
.completeOnTimeout("Timeout occurred", 3, TimeUnit.SECONDS)
.join();
System.out.println(result);
executor.shutdown();
}
}
Dealing with Exception Handling
Timeouts can also lead to exceptions if not handled properly. By using the exceptionally
method, you can gracefully handle these issues.
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.TimeUnit;
public class ExceptionHandlingExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
try { Thread.sleep(4000); } catch (InterruptedException e) { throw new RuntimeException("Interrupted"); }
return "Done";
});
String result = future
.completeOnTimeout("Default Value", 2, TimeUnit.SECONDS)
.exceptionally(ex -> {
System.out.println("An error occurred: " + ex.getMessage());
return "Error Occurred";
})
.join();
System.out.println(result);
}
}
Explanation:
In this snippet, if the task fails, it will log the error message and return "Error Occurred" instead of the default timeout value.
A Final Look
Managing timeouts when using CompletableFuture
is an integral part of ensuring robust asynchronous programming. By leveraging built-in timeout features, utilizing logging for tracking execution flow, configuring thread pools, and implementing comprehensive error handling, you can significantly improve the performance and reliability of your Java applications.
For more detailed information regarding Java's concurrency features, you can explore the official documentation here.
As you continue to work with asynchronous programming in Java, keep these strategies in mind. They not only help in debugging but also lead to better performance overall. Happy coding!