How to Safely Unescape HTML Characters in Java
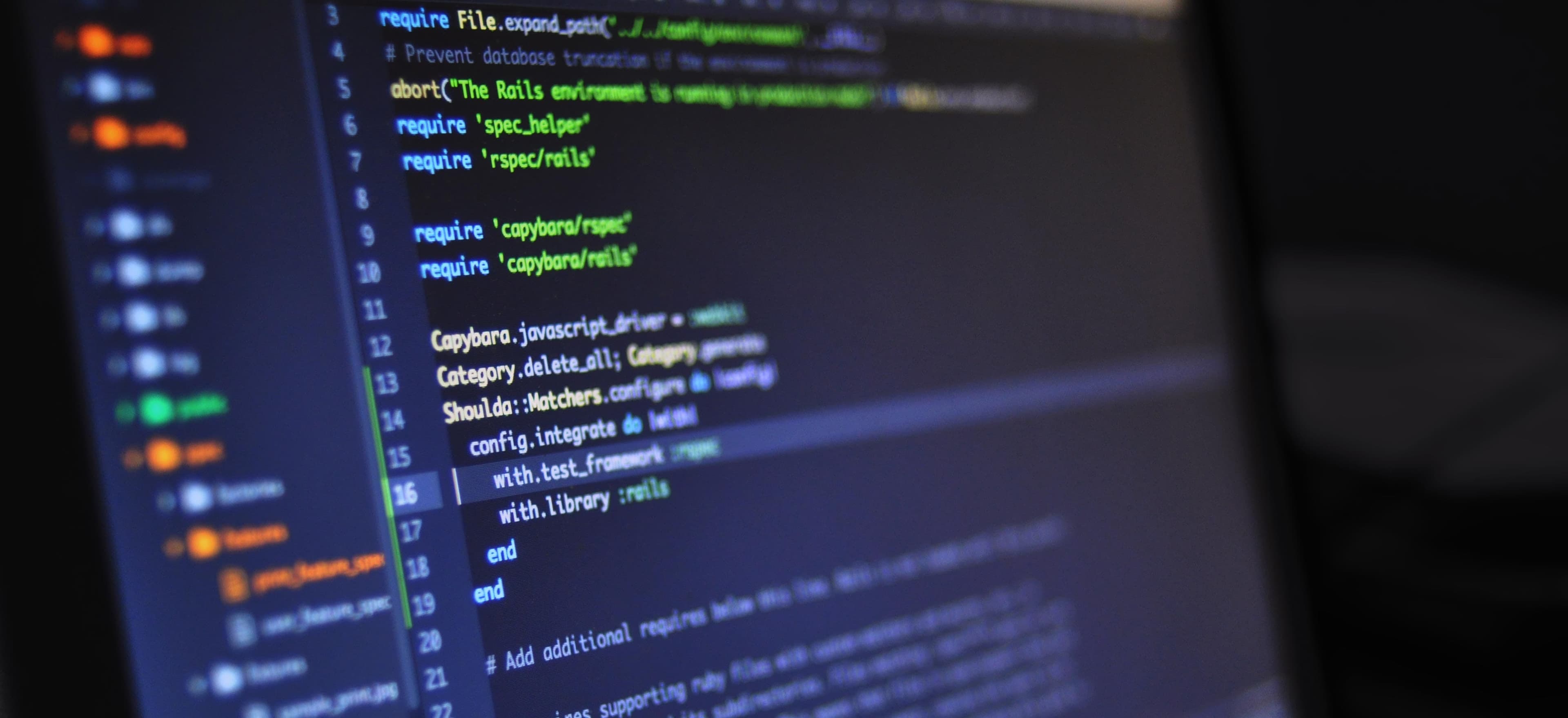
- Published on
How to Safely Unescape HTML Characters in Java
HTML escaping is an essential part of developing robust web applications. When user inputs include special HTML characters, those characters need to be escaped when rendering them to avoid security vulnerabilities, such as Cross-Site Scripting (XSS). Conversely, at times, you might need to unescape HTML characters back into their original representation. This blog post will guide you through safely unescaping HTML characters in Java, focusing on best practices and reliable libraries.
Understanding HTML Escaping and Unescaping
HTML escaping replaces characters like <
, >
, &
, etc., with their corresponding entity references, for example:
<
becomes<
>
becomes>
&
becomes&
Unescaping is the opposite process where these entity references are converted back to their original representation. In certain scenarios, failing to properly unescape may lead to broken page rendering or worse, security vulnerabilities.
Safe Unescaping: Why It Matters
Improper handling of HTML characters can lead to security vulnerabilities—specifically XSS attacks, where a malicious user might input scripts that are executed when unescaped. Therefore, it’s critical to have safe mechanisms for unescaping in place.
Libraries for Unescaping HTML Characters
Java offers various libraries to handle HTML unescaping safely. Here are a couple of notable ones:
- Apache Commons Lang: This is a widely-used library that provides the
StringEscapeUtils
class. - Jsoup: A library primarily used for parsing HTML but also includes methods for escaping and unescaping HTML.
Using Apache Commons Lang
If you are not already using Apache Commons Lang, you can add it to your project using Maven. You can include it in your pom.xml
:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version> <!-- Check for the latest version -->
</dependency>
Unescaping HTML with Apache Commons Lang
You can unescape HTML strings using the following code sample:
import org.apache.commons.text.StringEscapeUtils;
public class HtmlUnescapeExample {
public static void main(String[] args) {
String escapedHtml = "Hello, <world>! I love & enjoy coding.";
String unescapedHtml = StringEscapeUtils.unescapeHtml4(escapedHtml);
System.out.println("Unescaped HTML: " + unescapedHtml);
}
}
Why this works: The method unescapeHtml4()
efficiently converts all HTML entity references into their corresponding characters, making it simple and effective to render HTML content.
Using Jsoup
Jsoup is another fantastic library, specifically designed for working with real-world HTML. It provides a robust way to unescape HTML content.
Adding Jsoup to Your Project
You can add Jsoup to your Maven project by including the following dependency in your pom.xml
:
<dependency>
<groupId>org.jsoup</groupId>
<artifactId>jsoup</artifactId>
<version>1.14.3</version> <!-- Check for the latest version -->
</dependency>
Unescaping HTML with Jsoup
Here’s an example of how to unescape HTML strings using Jsoup:
import org.jsoup.Jsoup;
public class JsoupHtmlUnescapeExample {
public static void main(String[] args) {
String escapedHtml = "Welcome to <Jsoup>, where you can & learn!";
String unescapedHtml = Jsoup.parse(escapedHtml).text();
System.out.println("Unescaped HTML: " + unescapedHtml);
}
}
Why this works: Jsoup’s parse()
method takes in the HTML string, constructs a document, and text()
retrieves the plain text, automatically handling the unescaping of any HTML entities.
When to Unescape HTML
While unescaping can be helpful, it is equally important to know when you should do it. Here are some scenarios:
- Rendering user input in a webpage.
- Processing HTML that was previously saved in a database.
Make sure to verify that the source of the HTML content is safe. If user input is involved, validate it first to prevent XSS vulnerabilities.
Best Practices
-
Use Established Libraries: As demonstrated, Apache Commons Lang and Jsoup are both secure and reliable. Avoid manual implementation unless absolutely necessary.
-
Validate Input: Always validate user inputs that may contain HTML. Implementing sanitization measures can prevent harmful inputs from being processed.
-
Avoid unnecessary unescaping: If you don’t need to unescape the HTML, just keep it escaped. Only unescape when rendering or manipulating the content is absolutely necessary.
-
Test Extensively: Run various tests including corner cases to ensure that correct unescaping occurs as intended and does not leave vulnerabilities in your application.
Final Thoughts
Working with HTML in Java necessitates proper handling practices to ensure security and performance. Utilizing libraries such as Apache Commons Lang or Jsoup facilitates a seamless unescaping process while maintaining code clarity and efficiency. Always practice caution and validate input, as security vulnerabilities can greatly undermine a web application’s integrity.
For more in-depth exploration, check out the official documentation for Apache Commons Lang and Jsoup.
By adhering to these guidelines and employing reliable libraries, you'll be well-prepared to handle HTML character unescaping safely and effectively within your Java applications.
Checkout our other articles