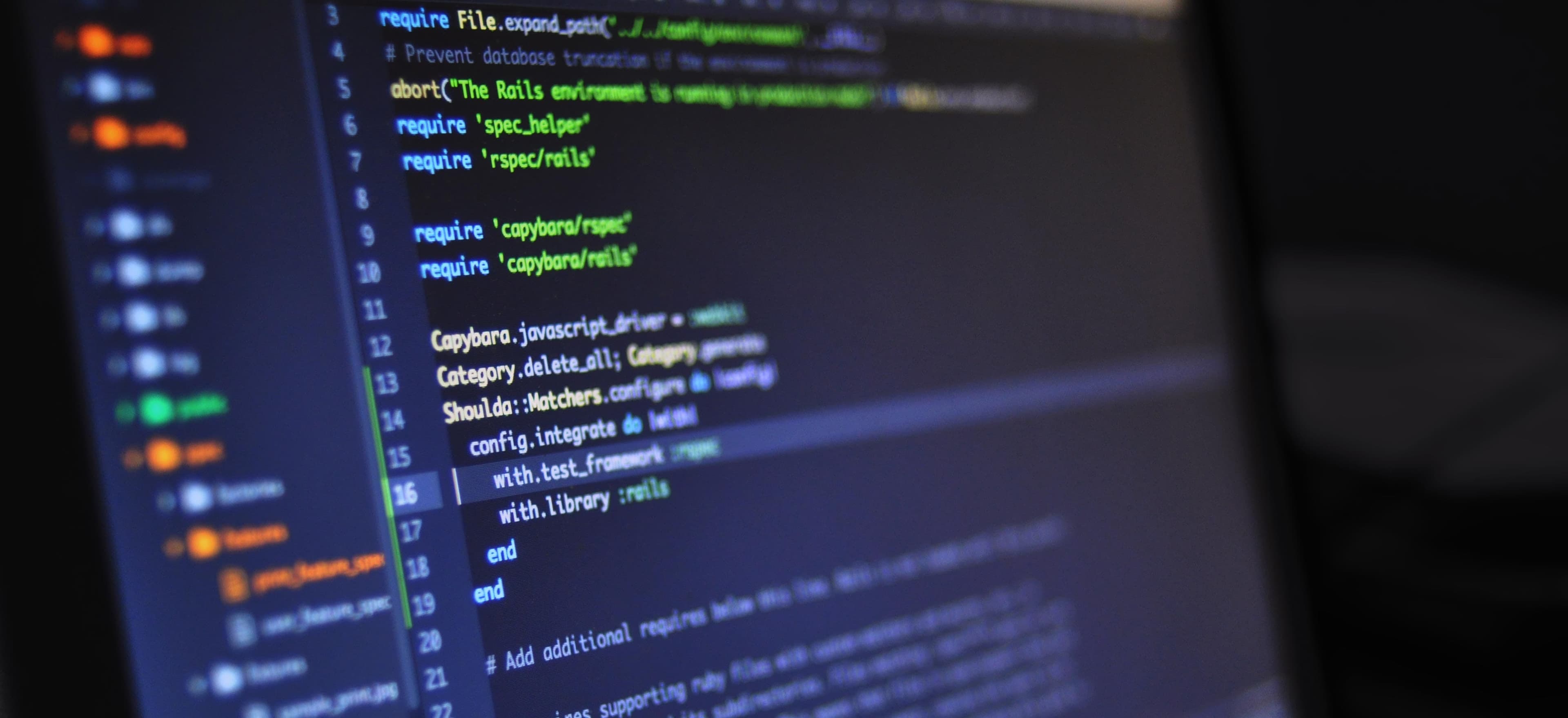
- Published on
Top 5 Database Security Flaws to Avoid at All Costs
In today's data-driven world, database security has become paramount. With cyberattacks escalating and data breaches making headlines, understanding the security vulnerabilities present in database systems is crucial. This blog will explore the top five database security flaws that can put your sensitive data at risk, and provide strategies to avoid them.
1. Lack of Proper Access Controls
Understanding Access Controls
Access control is the backbone of database security. It ensures that only authorized users can access sensitive data. This means implementing user authentication mechanisms and defining user permissions carefully.
Why It Matters
Without strict access controls, unauthorized users could have unrestricted access to critical data. This could lead to data leaks, manipulation, or even complete data loss.
Best Practices
- Implement Role-Based Access Control (RBAC): Different users should have different permissions based on their roles. For instance, an IT admin should have different access than an end-user.
public class UserAccess {
private String role;
public UserAccess(String role) {
this.role = role;
}
public boolean canAccessSensitiveData() {
return "ADMIN".equals(this.role);
}
}
This code snippet checks if a user has admin access. This simple check can help prevent unauthorized access.
- Regularly Review Access Rights: Conducting frequent audits of who has access to the database can help identify and mitigate risks before they lead to security breaches.
Additional Resource
For more on access control mechanisms, refer to the NIST guidelines.
2. Insecure Database Configurations
Importance of Proper Configurations
Insecure database configurations can leave your database vulnerable to various attacks. Default settings often do not prioritize security, leaving gaps for potential intrusions.
Why It Matters
When databases are not properly configured, they can expose your sensitive information to attackers.
Best Practices
- Change Default Credentials: Never use default usernames and passwords. Change them immediately after setting up the database.
# Change the default password in MySQL
ALTER USER 'root'@'localhost' IDENTIFIED BY 'NewPassword';
Changing default passwords is a straightforward yet critical step in securing your database.
- Disable Unused Features: If your database configuration has features you are not using, turn them off. Attackers often exploit these unused features.
Additional Resource
The OWASP Database Security Project offers comprehensive guidelines on securing your database environment.
3. Failure to Encrypt Sensitive Data
Understanding Data Encryption
Encryption is the process of converting data into a coded format that can only be read by authorized parties. This is crucial for protecting sensitive information both at rest and in transit.
Why It Matters
If an attacker gains access to your database, unencrypted sensitive data can be exploited immediately. Encryption adds another layer of protection.
Best Practices
- Encrypt Data at Rest: Use encryption techniques for storing sensitive information such as Personal Identifiable Information (PII).
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class DataEncryption {
public static byte[] encrypt(String data) throws Exception {
SecretKey key = KeyGenerator.getInstance("AES").generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data.getBytes());
}
}
This Java code snippet demonstrates how to encrypt data using AES encryption.
- Use TLS for Data in Transit: Always ensure that data transmitted between client and server is secured using Transport Layer Security (TLS).
Additional Resource
For detailed methodologies on database encryption, find compelling insights at Encrypting Databases.
4. Application-Layer Vulnerabilities
What They Are
Application-layer vulnerabilities arise from how applications communicate with databases. SQL Injection is a common example, where an attacker can execute arbitrary SQL code.
Why It Matters
Exploiting application-layer vulnerabilities can give an attacker access to sensitive data or allow them to manipulate your database.
Best Practices
- Use Prepared Statements: Prepared statements allow you to define SQL queries with placeholders. This minimizes the risk of SQL Injection.
import java.sql.Connection;
import java.sql.PreparedStatement;
public void insertUser(Connection connection, String username, String password) throws Exception {
String query = "INSERT INTO users (username, password) VALUES (?, ?)";
try (PreparedStatement stmt = connection.prepareStatement(query)) {
stmt.setString(1, username);
stmt.setString(2, password);
stmt.executeUpdate();
}
}
This Java snippet highlights using prepared statements to prevent SQL Injection attacks.
- Implement Input Validation: Always validate user inputs to ensure that they conform to expected patterns.
Additional Resource
To learn more about protecting against SQL Injection, check SQL Injection Prevention Cheat Sheet.
5. Insufficient Backup and Disaster Recovery Plans
Understanding Backup and Recovery
A robust backup and recovery plan ensures that you can recover data after a breach or system failure. Insufficient backups can lead to data loss.
Why It Matters
Without a reliable backup strategy, you risk significant downtime and financial loss if your data is compromised.
Best Practices
- Schedule Regular Backups: Automate the process of taking backups so that you have up-to-date copies of your data.
# Example command to backup a MySQL database
mysqldump -u user -p password database_name > backup_file.sql
This command creates a backup of your MySQL database.
- Test Recovery Procedures: Regularly test your backup recovery process to ensure you can restore your database when needed.
Additional Resource
For best practices surrounding backups, refer to the Backup and Recovery Plan Guide.
Final Thoughts
Prioritizing database security is not just a best practice; it is a necessity. By addressing these top five database security flaws, you can significantly mitigate the risks associated with data breaches. Regular audits, user education, and implementing the discussed best practices are essential steps in protecting your databases. Remember, a secure database adds a layer of trust with your users and protects your organization's reputation.
Take proactive measures today to safeguard your critical data. Start by assessing your current database security posture and make adjustments as necessary to create a more secure environment.
By heeding these guidelines, you can protect your database—and indeed, your entire organization—from the burgeoning threat landscape of cybercrime.