Troubleshooting Common Android App Engine Integration Issues
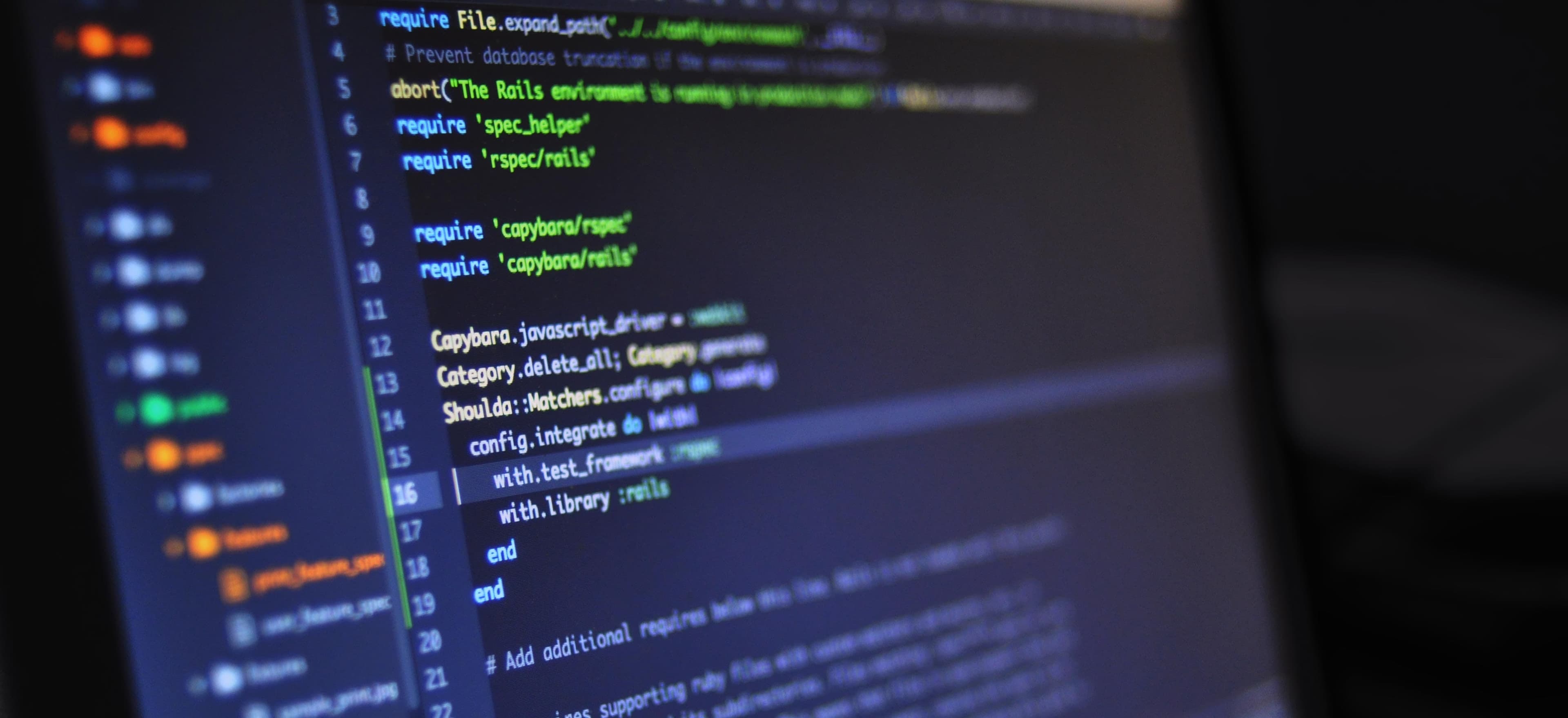
- Published on
Troubleshooting Common Android App Engine Integration Issues
When developing an Android application that utilizes Google Cloud's App Engine, you may encounter various integration challenges that can stall your development efforts. Understanding these hurdles is crucial for successful deployment. This blog post will provide insights into common App Engine integration issues with Android apps, actionable troubleshooting tips, and some best practices to ensure a smoother development experience.
Understanding Google Cloud App Engine
Before diving into challenges, let’s quickly recap what Google Cloud’s App Engine is. App Engine is a fully managed platform for building and deploying applications. It abstracts away the underlying infrastructure concerns and allows you to scale automatically based on traffic.
If you're new to App Engine, consider reading the official Google Cloud App Engine documentation to familiarize yourself with its features and capabilities.
Common Integration Issues
1. Authentication Errors
Authentication is a critical aspect of any application that connects to Google Cloud services. Often, you might face issues such as:
-
Invalid Credentials: Make sure that you have correctly set up your credentials, which usually involves downloading a service account key from your Google Cloud Console.
-
Access Scope Configuration: Ensure that proper access scopes are configured in your Google Cloud project, especially if you’re accessing sensitive resources.
Example Code Snippet
Here is a sample code illustrating how to set up authentication in your Android app:
import com.google.api.client.googleapis.auth.oauth2.GoogleAccountCredential;
private GoogleAccountCredential getCredential(String accountName) {
GoogleAccountCredential credential = GoogleAccountCredential.usingOAuth2(
this, Collections.singleton("https://www.googleapis.com/auth/cloud-platform"));
credential.setSelectedAccountName(accountName);
return credential;
}
Why this code is essential: This code snippet demonstrates how to initialize the Google Account Credential to authenticate your app. Correctly setting up authorization will resolve many access-related issues.
2. Network Configuration Issues
Another common issue arises from improper network configurations. Sometimes you may find that requests to your App Engine instance fail due to:
-
Firewall Rules: Ensure that your Google Cloud firewall settings permit traffic from your Android device.
-
Internet Permissions: Check your AndroidManifest.xml to ensure that your app has the necessary permissions to access the internet.
Example Code Snippet
Make sure your application has appropriate internet permissions:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapp">
<application
... >
...
</application>
<uses-permission android:name="android.permission.INTERNET"/>
</manifest>
Why this snippet matters: Missing internet permissions in your AndroidManifest.xml
can lead to network requests being blocked. Always validate these entries.
3. Incorrect API Endpoints
You can face issues when the Android app is not able to talk with APIs hosted on App Engine. These troubles commonly emerge from:
-
Wrong URL Format: Ensure that you are using the complete and correct endpoint URLs, including the correct method (GET, POST, etc.).
-
CORS Issues: If your app runs in the browser, make sure you have CORS enabled on your App Engine applications.
Example Code Snippet
Here's an example of how to make an API call:
String urlString = "https://<your-app-id>.appspot.com/api/data";
try {
URL url = new URL(urlString);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.setRequestMethod("GET");
urlConnection.connect();
// Handle the response in your app
} catch (IOException e) {
e.printStackTrace();
}
Why this is crucial: Using incorrect URL can lead to a 404 Not Found
error. This snippet exemplifies how to set up a basic GET request to your App Engine instance.
4. Incompatibility Issues with Libraries
Sometimes, dependency conflicts become a thorn in your side. Libraries used in your Android project might conflict with libraries utilized by your App Engine back-end.
- Version Compatibility: Ensure that your app’s dependencies are compatible with the versions used on App Engine.
Example: Gradle Dependency
dependencies {
implementation 'com.google.api-client:google-api-client:1.30.11'
implementation 'com.google.guava:guava:30.1.1-jre'
}
Why this matters: Always ensure that the libraries used in your app are compatible with same or higher versions and are documented. Failing to do so can result in runtime errors that may be hard to debug.
5. Google Cloud TTL Issues
A peculiar issue that some developers face occurs due to overly aggressive caching and TTL (Time to Live) settings. Sometimes, it can lead to the old versions of app static files being served.
- Set Correct Cache Headers: Configure your cache settings wisely within your app configuration files.
Example: Setting Cache Headers in App.yaml
You can adjust cache settings in your App Engine configuration:
handlers:
- url: /static
static_dir: static
expiration: "2h" # Time to live for static files
Why this is essential: Proper cache management allows your app to serve the latest files without undue delays. If you're constantly changing static assets and don’t see the updates, this could be the culprit.
Best Practices for Smooth Integration
-
Use Environment Variables: Store sensitive data and configuration options in environment variables rather than hardcoding them in the source code.
-
Regularly Update Dependencies: Make it a practice to update your libraries and SDKs. New versions often fix bugs and improve performance.
-
Monitor Logs: Utilize the Google Cloud Logging service to keep an eye on logs from your App Engine instance for real-time insights when your app runs into issues.
-
Comprehensive Testing: Implement both unit and integration tests on your API endpoints to catch errors before they make their way into production.
-
Consult the Community: If all else fails, don't hesitate to visit forums like Stack Overflow or the Google Cloud Community for advice.
Final Thoughts
Troubleshooting common integration issues between your Android application and Google Cloud's App Engine may seem daunting, but understanding typical problems and their solutions can dramatically simplify the experience. The above strategies and code examples should equip you with the knowledge to overcome these hurdles effectively.
Stay informed, experiment, and, when in doubt, don't hesitate to reach out to communities and official documentation. Happy coding!