Boost Productivity: Mastering Time Blocks for Coding Success
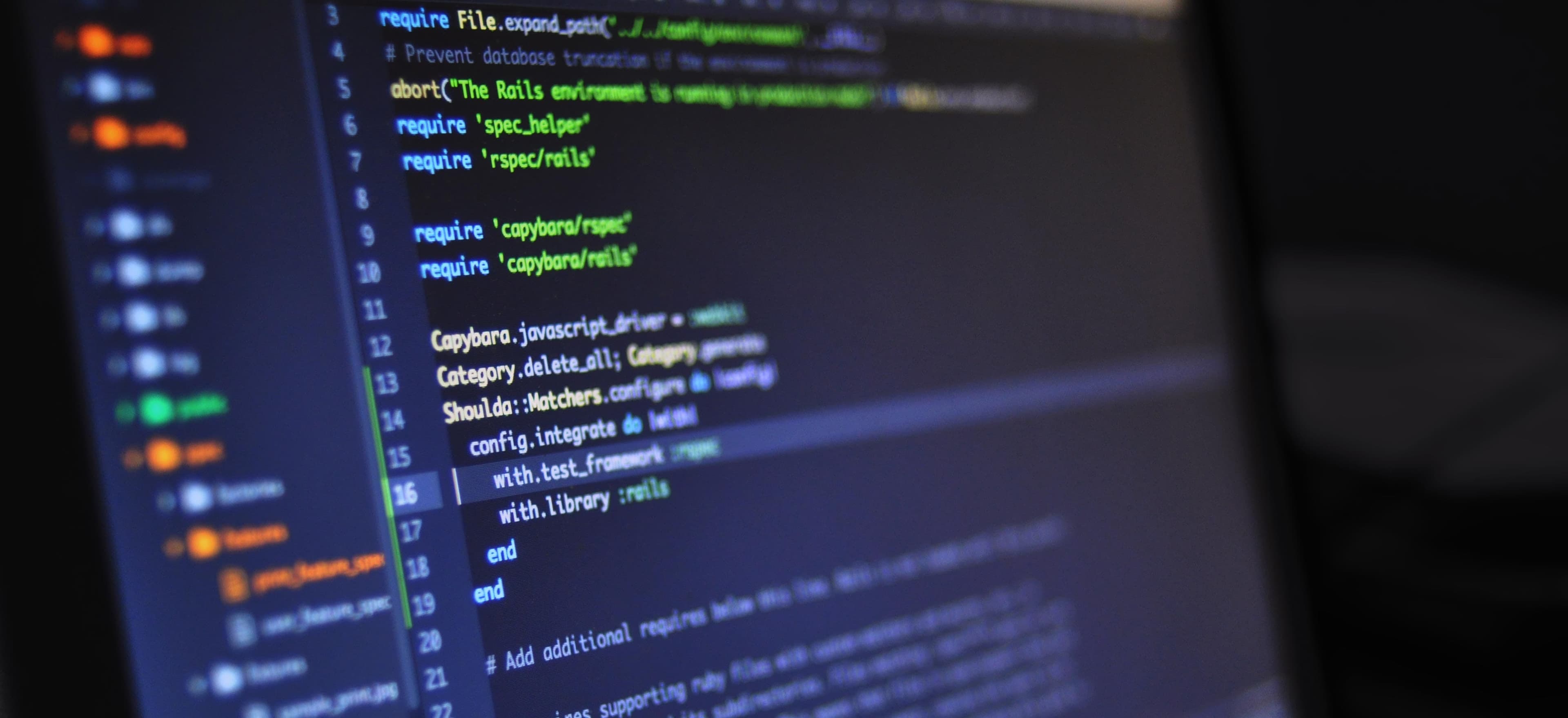
- Published on
Boost Productivity: Mastering Time Blocks for Coding Success
As programmers, the ability to focus and maintain a steady workflow is crucial for success. One method that has proven effective in this arena is the concept of time blocking. This structured approach to time management can enhance productivity, minimize distractions, and make coding sessions much more efficient. In this blog post, we'll delve into what time blocking is, its advantages, and how to apply it effectively in your coding practice.
What is Time Blocking?
Time blocking is a time management technique wherein you allocate specific blocks of time to different tasks throughout the day. This means you set aside uninterrupted periods for focused work, allowing you to dive into coding without distractions.
For example, you might have a schedule that includes:
- 9:00 AM - 10:00 AM: Code Review
- 10:00 AM - 12:00 PM: Feature Development
- 1:00 PM - 2:00 PM: Debugging
- 2:00 PM - 3:00 PM: Learning New Frameworks
By blocking out time, you help your brain prepare for focused work, increasing your overall efficiency.
Advantages of Time Blocking
- Improved Focus: When you know exactly what you're working on during a specific time frame, it reduces the tendency to procrastinate.
- Less Context Switching: Instead of jumping from one task to another, you stay in the zone. This flow state is invaluable, especially when coding.
- Increased Accountability: Establishing a clear plan for your day makes it easier to see when you've strayed from the course. You're more likely to stick to your commitments.
- Better Work-Life Balance: By organizing your time, you can allocate periods for personal activities, preventing work from spilling into your free time.
Implementing Time Blocking in Your Coding Workflow
Step 1: Identify Key Tasks
Start by listing your key tasks and responsibilities as a programmer. These might include debugging, coding new features, code reviews, and learning. Once you have a clear picture of your workload, prioritize these tasks based on urgency and importance.
Step 2: Create Your Time Blocks
Now that you, have your tasks identified, it's time to allocate time blocks. Below is a simple Java code snippet that implements a rudimentary task planner using a Map
, which can help you visualize how you might structure your time blocks:
import java.util.HashMap;
import java.util.Map;
public class TimeBlocking {
public static void main(String[] args) {
// Create a map to store tasks and their allocated time blocks
Map<String, String> tasks = new HashMap<>();
// Allocating time blocks for each task
tasks.put("9:00 AM - 10:00 AM", "Code Review");
tasks.put("10:00 AM - 12:00 PM", "Feature Development");
tasks.put("1:00 PM - 2:00 PM", "Debugging");
tasks.put("2:00 PM - 3:00 PM", "Learning New Frameworks");
// Print out the schedule for the day
tasks.forEach((time, task) -> System.out.println(time + ": " + task));
}
}
Commentary on Code
In the above example:
- We use a
HashMap
to store tasks alongside their allocated time slots. - This structure provides a simple way to visualize your schedule and makes it easy to adjust tasks as needed.
You can expand this Map
to include additional parameters like task priority, estimated effort, or even deadlines.
Step 3: Use the Pomodoro Technique
Integrating the Pomodoro Technique into time blocks can further enhance your productivity. The Pomodoro Technique consists of working for a specified period, typically 25 minutes, followed by a short break (5 minutes). After four iterations, take a longer break (15-30 minutes).
For instance, if you are implementing a feature during your allotted 10:00 AM - 12:00 PM block, you might work intensely for 25 minutes, then take a 5-minute break, and repeat. This can help in preventing burnout and maintaining high levels of engagement.
Here’s a simple Java class that represents the Pomodoro Timer concept:
import java.util.Scanner;
public class PomodoroTimer {
public static void main(String[] args) {
// Get user input for session duration and breaks
Scanner scanner = new Scanner(System.in);
System.out.print("Enter focus duration in minutes: ");
int focusDuration = scanner.nextInt();
System.out.print("Enter break duration in minutes: ");
int breakDuration = scanner.nextInt();
System.out.println("Focus for " + focusDuration + " minutes, then take a " + breakDuration + " minute break.");
// Here you could implement actual timer logic with Thread.sleep() for real pauses
// For simplicity, we are just illustrating user interaction
scanner.close();
}
}
Commentary on Code
In the Pomodoro Timer class:
- We use a
Scanner
to gather user input for focus and break durations. - This sets the stage for creating a more complex timer that could handle the actual timing mechanism.
Step 4: Analyze and Adjust
After implementing your time blocks for a week or two, take time to analyze what’s working and what’s not. Did you complete tasks efficiently? Were there unexpected distractions? Based on this reflection, you can adjust your blocks to better suit your workflow.
Step 5: Leverage Tools
In this digital age, there are numerous tools available that can help streamline the time-blocking process. Tools like Trello, Todoist, or calendar applications can provide visual cues to structure your time effectively.
For coding-specific tools, consider using IDE plugins that enable time tracking or task management directly within your coding environment. Additionally, there are resources available online that dive deeper into productivity techniques tailored for programmers. For example, check out Hackernoon's Ultimate Guide to Time Management for more strategies.
A Final Look
Time blocking is more than just managing hours; it's about maximizing the effectiveness of your coding efforts. By leveraging the focus provided through structured schedules and integrating techniques like the Pomodoro method, you can elevate your productivity significantly.
Stay proactive in understanding your working patterns, keep adapting your strategies, and you will find that mastering time blocks can lead to coding success and a healthier work-life balance. Your future self will thank you for it!
Is time blocking something you've tried? How did it work for you? Share your experiences and insights in the comments below!
Checkout our other articles