Overcoming Complexity: Simplifying Multilayered Architecture
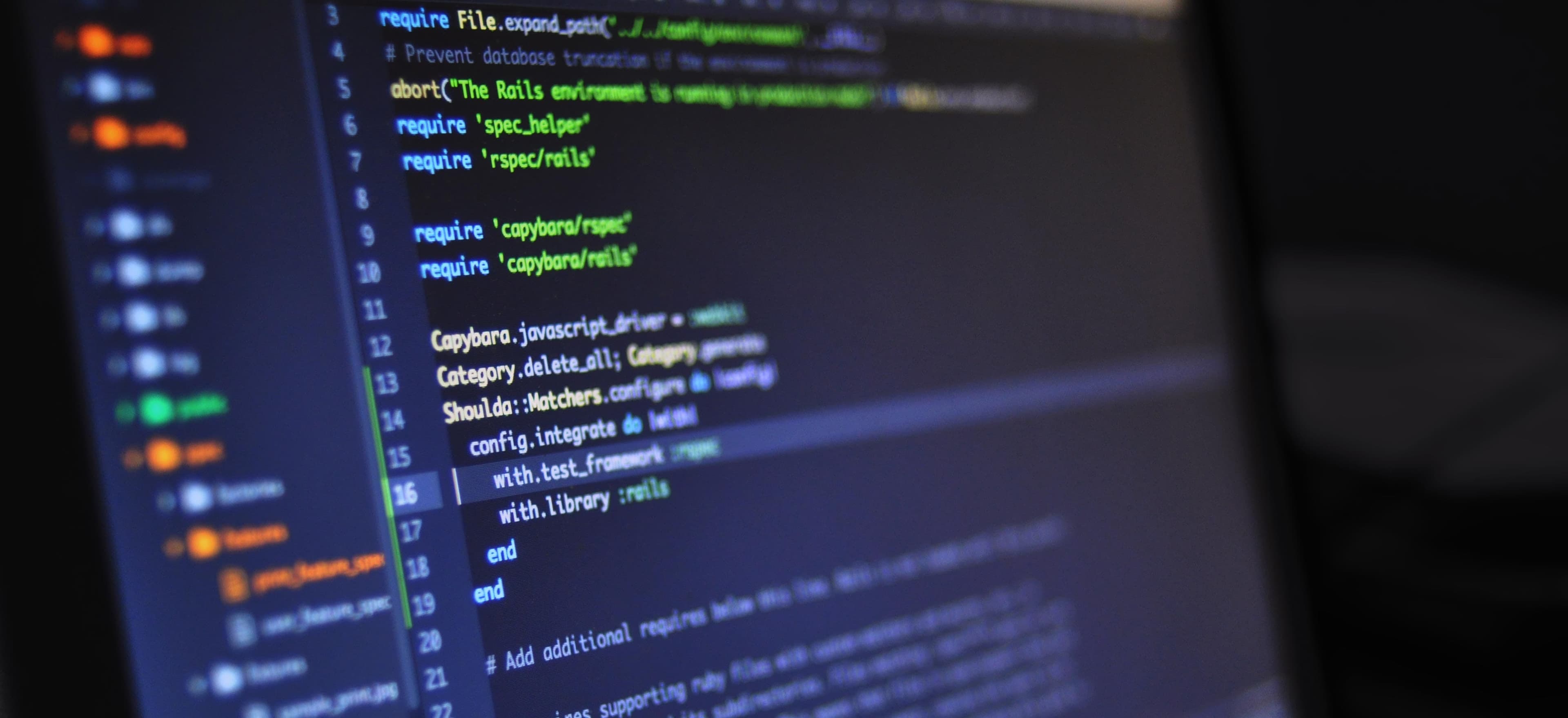
- Published on
Overcoming Complexity: Simplifying Multilayered Architecture in Java
In the modern landscape of software development, architects often grapple with a key challenge: complexity. This complexity often stems from multilayered architectures. In this post, we will delve into understanding multilayered architecture, exploring the underlying complexities, and provide strategies for simplifying it, specifically in the context of Java development.
What is Multilayered Architecture?
Multilayered architecture, often referred to as n-tier architecture, is a pattern where software is divided into several layers, each serving a distinct purpose. Typically, you might encounter these layers:
- Presentation Layer: This layer manages user interaction and display. It can be web-based, mobile, or desktop.
- Business Logic Layer: Also known as the service layer, this encapsulates the business rules and logic.
- Data Access Layer: This layer interacts with the data sources, often including databases or external APIs.
While this separation of concerns promotes maintainability and scalability, it can lead to increased complexity. Each layer introduces additional interactions, potential bottlenecks, and integration challenges that can be cumbersome to manage.
Understanding the Sources of Complexity
1. Increased Communication Overhead
With multiple layers, the number of interactions between them can exponentially increase. This can severely degrade performance and complicate debugging processes. The time spent in communication often outweighs the time spent in processing tasks.
2. Over-Engineering
Often developers over-engineer solutions, adding extra layers or abstractions that may not be necessary. This could lead to bloated codebases that are hard to traverse and maintain.
3. Dependency Management
As layers interact with each other, their dependencies can create tightly coupled code. Modifying a lower layer may unintentionally affect upper layers, making the system fragile.
Simplifying Multilayered Architecture in Java
1. Use of Design Patterns
Design patterns can help mitigate complexity by providing tried-and-true solutions for common problems. Here are a few patterns particularly useful in well-structured Java applications:
Repository Pattern
The Repository Pattern abstracts data access logic, allowing you to separate your data access code from the business logic.
public interface UserRepository {
User findById(Long id);
void save(User user);
}
public class UserRepositoryImpl implements UserRepository {
private EntityManager entityManager;
public UserRepositoryImpl(EntityManager entityManager) {
this.entityManager = entityManager;
}
@Override
public User findById(Long id) {
return entityManager.find(User.class, id);
}
@Override
public void save(User user) {
entityManager.persist(user);
}
}
Why? This pattern reduces the complexity of database interactions, making your code cleaner and more testable. By decoupling data access from business logic, you improve the maintainability of your application.
2. Implementing Service Interfaces
Using interfaces to define your service layer can provide clear contracts for how different components will interact. This structure encourages loose coupling and makes it easier to swap out implementations.
public interface UserService {
User getUser(Long id);
void createUser(User user);
}
public class UserServiceImpl implements UserService {
private UserRepository userRepository;
public UserServiceImpl(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Override
public User getUser(Long id) {
return userRepository.findById(id);
}
@Override
public void createUser(User user) {
userRepository.save(user);
}
}
Why? Separation of concerns is crucial. With this approach, you can replace the UserServiceImpl
without affecting the rest of your application, as long as the contract in the UserService
interface remains unchanged.
3. Dependency Injection
Frameworks like Spring allow for dependency injection, which simplifies complex wiring between classes. By allowing the framework to manage dependencies, you can focus on the logic rather than the configuration.
@Configuration
public class AppConfig {
@Bean
public UserRepository userRepository() {
return new UserRepositoryImpl(entityManager());
}
@Bean
public UserService userService() {
return new UserServiceImpl(userRepository());
}
@Bean
public EntityManager entityManager() {
// Configure your EntityManager
}
}
Why? Dependency injection reduces the boilerplate code required to instantiate classes and manage their lifecycles. It promotes a cleaner design and enhances testability by enabling easier mocking of dependencies.
4. Leveraging Frameworks
Frameworks such as Spring Boot can help simplify application configurations, allowing developers to focus on writing business logic without worrying about the boilerplate code.
For example, using Spring Boot, you can quickly set up a RESTful API:
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.getUser(id);
return ResponseEntity.ok(user);
}
@PostMapping
public ResponseEntity<Void> createUser(@RequestBody User user) {
userService.createUser(user);
return ResponseEntity.status(HttpStatus.CREATED).build();
}
}
Why? Using a framework streamlines the creation of web services. The annotations used (e.g. @RestController, @GetMapping) make the code more expressive and easier to understand.
5. Continuous Refactoring
One of the best practices in managing complexity is to commit to continuous refactoring. Regularly reviewing and simplifying your architecture as your application evolves will keep you ahead of potential complexity.
Encourage a culture of refactoring within your team. It prevents technical debt from accumulating and helps in the long-term health of the codebase.
6. Monitoring and Performance Analysis
Complexity can result in performance issues. Integrating monitoring tools will help identify bottlenecks or inefficiencies in your multilayered architecture. Tools such as Spring Boot Actuator can provide insights into application performance.
Wrapping Up
Overcoming the complexities of multilayered architecture in Java does not have to be daunting. By utilizing design patterns, dependency injection, and suited frameworks like Spring Boot, developers can streamline their code and enhance its maintainability.
Furthermore, a commitment to continuous refactoring and employing monitoring tools ensures that the application not only remains performant but also is easier to understand and modify.
As you evolve your Java applications, keep these strategies in mind to simplify your multilayered architecture, paving the way for more robust and scalable systems.
For more insights on Java best practices, consider checking out the Java Official Documentation or exploring Spring's Official Guides.
Is your application ready for a redesign? What strategies will you implement to reduce complexity? Share your thoughts in the comments!
Checkout our other articles