Mastering JFreeChart: Overcoming Common Charting Pitfalls
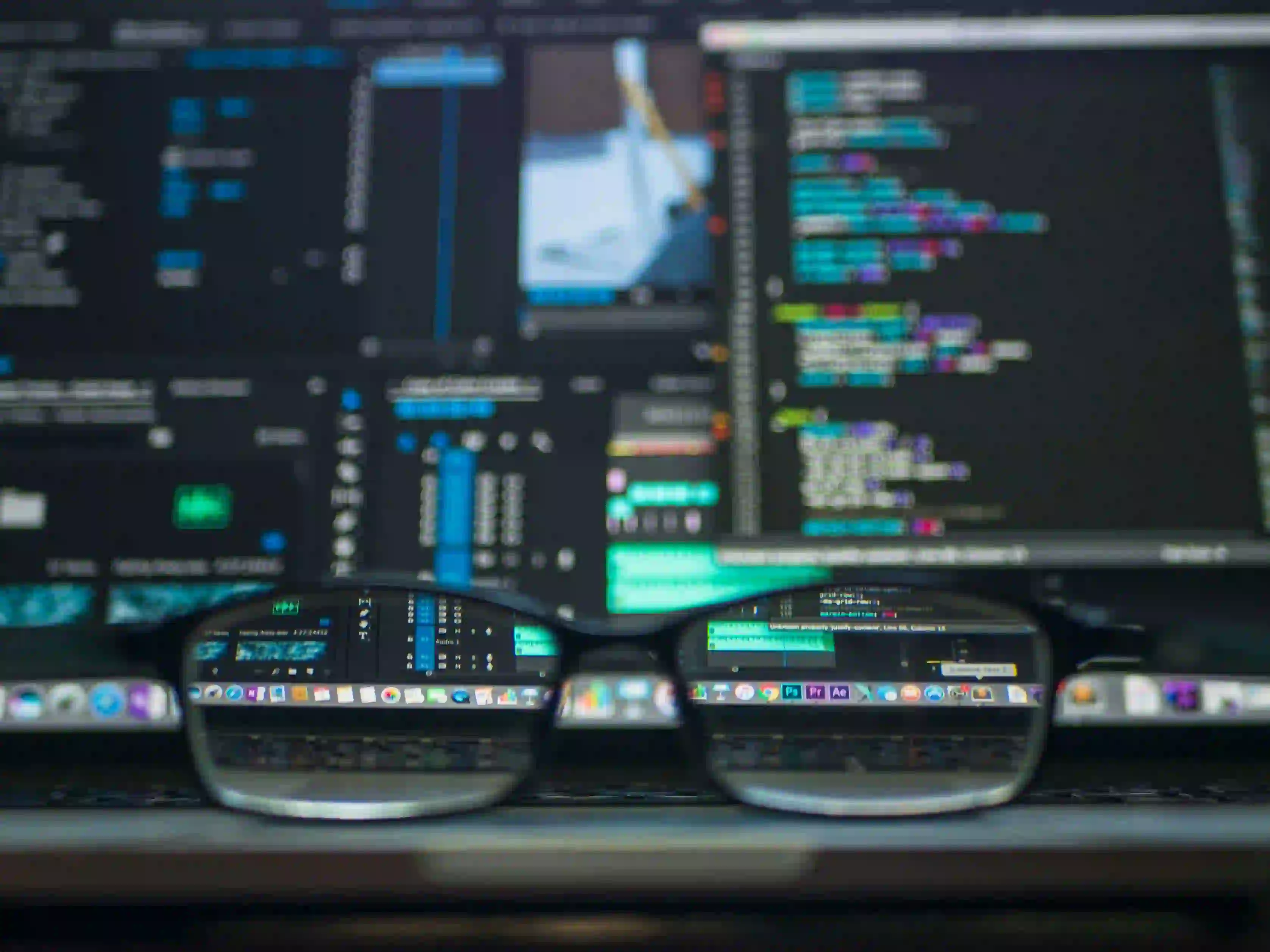
Mastering JFreeChart: Overcoming Common Charting Pitfalls
Charting is an essential feature in any software application aimed at data visualization. With numerous libraries available, one that stands out is JFreeChart, a powerful Java library well-suited for creating a variety of charts. However, while JFreeChart is robust, developers can encounter several pitfalls that may hinder their charting experience. In this post, we will cover common challenges and how to overcome them, ensuring that you can harness the full potential of JFreeChart.
What is JFreeChart?
JFreeChart is a widely used open-source library for creating a wide range of popular charts in Java, including pie charts, bar charts, line charts, and more. It allows developers to generate both static and dynamic charts seamlessly.
Setting Up JFreeChart
Before we dive into common issues, ensuring you have JFreeChart installed is crucial. You can download the library from the official JFreeChart website. After downloading, include the JAR files in your project’s build path.
Basic Example of a Line Chart
Let’s start with a simple example of creating a line chart. Here is a Java snippet that demonstrates this:
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.xy.XYSeries;
import org.jfree.ui.ApplicationFrame;
public class LineChartExample extends ApplicationFrame {
public LineChartExample(String title) {
super(title);
JFreeChart lineChart = createChart();
ChartPanel chartPanel = new ChartPanel(lineChart);
chartPanel.setPreferredSize(new java.awt.Dimension(800, 600));
setContentPane(chartPanel);
}
private JFreeChart createChart() {
XYSeries series = new XYSeries("Example Data");
series.add(1, 1);
series.add(2, 4);
series.add(3, 3);
series.add(4, 5);
return ChartFactory.createXYLineChart(
"Sample Line Chart",
"X Axis",
"Y Axis",
series
);
}
public static void main(String[] args) {
LineChartExample chart = new LineChartExample("Line Chart Example");
chart.pack();
chart.setVisible(true);
}
}
Commentary on the Code
- JFreeChart Initialization: We initialize the chart using
ChartFactory.createXYLineChart()
. This method simplifies chart creation and ensures best practices regarding chart aesthetics and functionality. - XYSeries: This class holds the data for the chart. It is crucial because it allows dynamic manipulation of data points.
Common Pitfall #1: Overlooking Data Visibility
One of the most common pitfalls developers face is the data not appearing on the chart. This can happen when the data series is either empty or outside the visible range of the chart.
Solution:
Always ensure that your data series are populated with valid data. Moreover, make sure that the axis ranges accommodate your data. We can set the ranges using the following code:
plot.getRangeAxis().setRange(0, 10);
plot.getDomainAxis().setRange(0, 5);
Setting these ranges enables you to control the view of the axes explicitly, thereby ensuring your data is visible.
Common Pitfall #2: Ignoring Chart Refresh
When dynamically updating data, many developers forget to refresh or redraw the chart. In such cases, a user may not see the updated data unless they manually refresh the application or window.
Solution:
Use ChartPanel
's repaint()
method after the data has been modified:
chartPanel.repaint();
This command signals the component to redraw itself, ensuring that the latest data is displayed.
Common Pitfall #3: Cluttered Charts
While visual appeal is important, cramming too much information into a single chart can lead to clutter and confusion. This can overwhelm users and obscure important data trends.
Solution:
- Use Legends and Titles Wisely: Following best practices can greatly enhance clarity.
- Limit the Data Series: Avoid adding too many data series. Consider breaking them into multiple charts or using interactive filters to manage data visibility.
Here’s how to add a legend:
chart.setLegend(new LegendTitle(chart.getPlot(), new Font("SansSerif", Font.PLAIN, 10)));
You can customize the position and appearance of the legend to improve readability.
Common Pitfall #4: Inaccessible Charts
Another significant issue can arise when creating charts that are not accessible for individuals with visual impairments.
Solution:
Make sure to incorporate accessibility features into your charts. One way to achieve this is through proper labeling and tooltips:
plot.getDomainAxis().setLabel("Time");
plot.getRangeAxis().setLabel("Values");
// Add tooltips
XYItemRenderer renderer = plot.getRenderer();
renderer.setBaseToolTipGenerator(new StandardXYToolTipGenerator());
This approach can help users understand the chart context even if they cannot see the details clearly.
Advanced Customization
Beyond the common pitfalls, JFreeChart allows for extensive customization. Here’s a snippet showing how to modify the appearance of a line chart:
// Changing line color and stroke
BasicStroke stroke = new BasicStroke(2.0f);
renderer.setSeriesStroke(0, stroke);
renderer.setSeriesPaint(0, Color.BLUE);
Changing the stroke and paint can help you direct the user's focus to specific data trends.
Final Thoughts
Mastering JFreeChart involves understanding both its powerful features and the common pitfalls associated with data visualization. By addressing these issues—like data visibility, chart refresh, clutter, and accessibility—you can create effective and engaging visualizations.
Experiment with the examples provided, and as you grow more comfortable with JFreeChart, feel free to dive into more advanced features to create intricate and tailored charts for your applications.
For further reading on JFreeChart and its capabilities, consider exploring the official JFreeChart documentation, where you can find additional examples and advanced functionalities.
Happy charting!