Boosting Apache Camel 3.6: Fixing Common Performance Issues
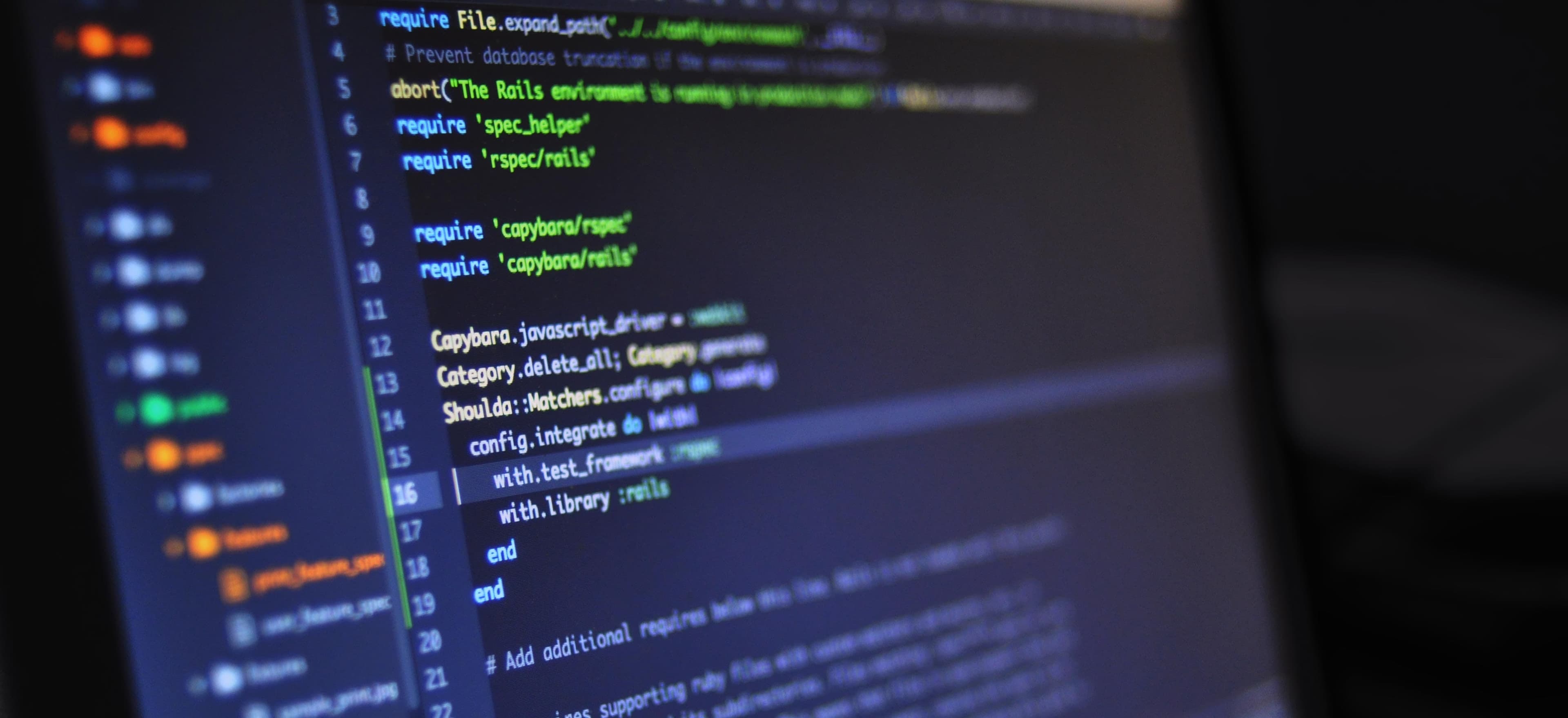
- Published on
Boosting Apache Camel 3.6: Fixing Common Performance Issues
Apache Camel is a powerful integration framework that allows developers to integrate various systems in a flexible and maintainable way. However, as with any framework, performance issues can arise that may hinder the efficiency of your applications. In this post, we will discuss common performance issues you may encounter while using Apache Camel 3.6, along with potential solutions to optimize your integration routes.
Understanding Performance Bottlenecks
Before diving into specific issues, it's crucial to understand what performance bottlenecks are. These are segments in a project that slow down execution times significantly, inhibiting the flow of data. Performance issues often stem from inefficient route configurations, resource constraints, or excessive memory consumption.
Identifying Common Performance Issues
-
Slow Routes: One of the most frequent concerns in Apache Camel projects is route slowness. This can be due to heavy processing in the route or improper configuration of components.
-
Memory Leaks: Over time, memory leaks can accumulate, leading to increased garbage collection (GC) times, which can further degrade performance.
-
Thread Management: Misconfigured thread pools can lead to either starvation or excessive resource usage, both of which can cripple a Camel application’s performance.
Optimizing Your Camel Routes
Optimizing your Camel routes can significantly improve application performance. Here are some effective strategies:
1. Reduce Processing Time with Route Optimization
One of the first steps you should consider is optimizing the route itself. This can involve modifying data transformation methods or accessing data less frequently.
Code Example: Filtering Data Early
from("direct:start")
.filter(simple("${body.size()} < 500"))
.process(exchange -> {
// Perform data transformation
})
.to("direct:end");
Why: Here, we use a filter to discard larger messages early in the route, reducing processing time for the subsequent pipeline and lowering the load on further processing stages.
2. Asynchronous Processing
If your application allows, implement asynchronous processing to free up resources. The ExecutorService
provides a simple way to manage concurrent tasks.
Code Example: Using ExecutorService
ExecutorService executorService = Executors.newFixedThreadPool(10);
from("direct:start")
.process(exchange -> {
Runnable task = () -> {
// Perform time-consuming operation
};
executorService.submit(task);
});
Why: Using ExecutorService
allows you to handle multiple tasks simultaneously, effectively utilizing available system resources and improving response time.
3. Avoid Memory Leaks
Memory leaks can significantly impact the performance of your Camel application. Regular use of profiling tools, such as VisualVM or Eclipse MAT, can help identify these issues.
Code Example: Use WeakReference
import java.lang.ref.WeakReference;
public class MyService {
private WeakReference<MyResource> myResourceRef;
public void initializeResource() {
myResourceRef = new WeakReference<>(new MyResource());
}
public MyResource getResource() {
return myResourceRef.get(); // Returns null if resource is GC'd
}
}
Why: Using WeakReference
allows the garbage collector to reclaim the reference if necessary, preventing memory leaks and optimizing resource utilization.
4. Configuring Thread Pools
Improper configuration of thread pools can lead to problems such as thread contention, which can bottleneck performance.
Code Example: Configuring Thread Pool in Camel Context
CamelContext context = new DefaultCamelContext();
ThreadPoolProfile profile = new ThreadPoolProfile();
profile.setPoolSize(20); // Set an optimal thread pool size
context.getExecutorServiceManager().updateThreadPoolProfile("myThreadPool", profile);
Why: Properly configuring the thread pool size allows the application to handle a larger number of requests concurrently, reducing latency.
5. Efficiently Handling Error Messages
Implementing robust error-handling strategies can also help mitigate performance slowdowns. When an error occurs, efficiently routing the error messages can save processing time.
Code Example: Using Dead Letter Channel
from("direct:start")
.errorHandler(deadLetterChannel("direct:error").maximumRedeliveries(3).redeliveryDelay(1000));
Why: Using a dead letter channel allows you to manage failed messages without overwhelming the primary processing logic, effectively avoiding unnecessary resource strain.
Monitoring and Testing Performance
It's essential to monitor your Camel applications continuously and test them periodically to identify and address performance issues proactively.
1. Monitoring Tools
Utilize tools like Prometheus and Grafana to keep an eye on system performance. Implementing metrics collection in Camel routes can provide insights into how various components perform.
Code Example: Metrics Route
from("direct:start")
.to("metrics:myRoute?metricType=timer");
Why: By capturing metrics such as response times, you can make informed decisions based on actual performance data rather than assumptions.
2. Load Testing
Utilize load testing tools like Apache JMeter or Gatling to simulate real-world conditions. This testing helps uncover issues that may not be evident during regular development.
In Conclusion, Here is What Matters
Performance is a critical component of any Apache Camel application. By understanding common performance issues and applying the strategies outlined in this post, you can boost the performance of your Apache Camel 3.6 routes significantly.
I encourage you to experiment with the provided code samples in your integration projects and to monitor their impact on performance. The integration world thrives on continuous improvement, so remain open to adjustments as your application and its requirements evolve.
By focusing on optimizing your routes, managing resources effectively, and implementing robust monitoring strategies, you'll set your Camel applications up for success.
For more information on Camel Best Practices, check the Apache Camel Documentation or explore community discussions on Stack Overflow to deep dive into optimization strategies.
By addressing these performance issues proactively, you can ensure that your Apache Camel applications operate efficiently, delivering reliable integration solutions that scale with your business needs.