Why Inversion of Control Can Confuse New Developers
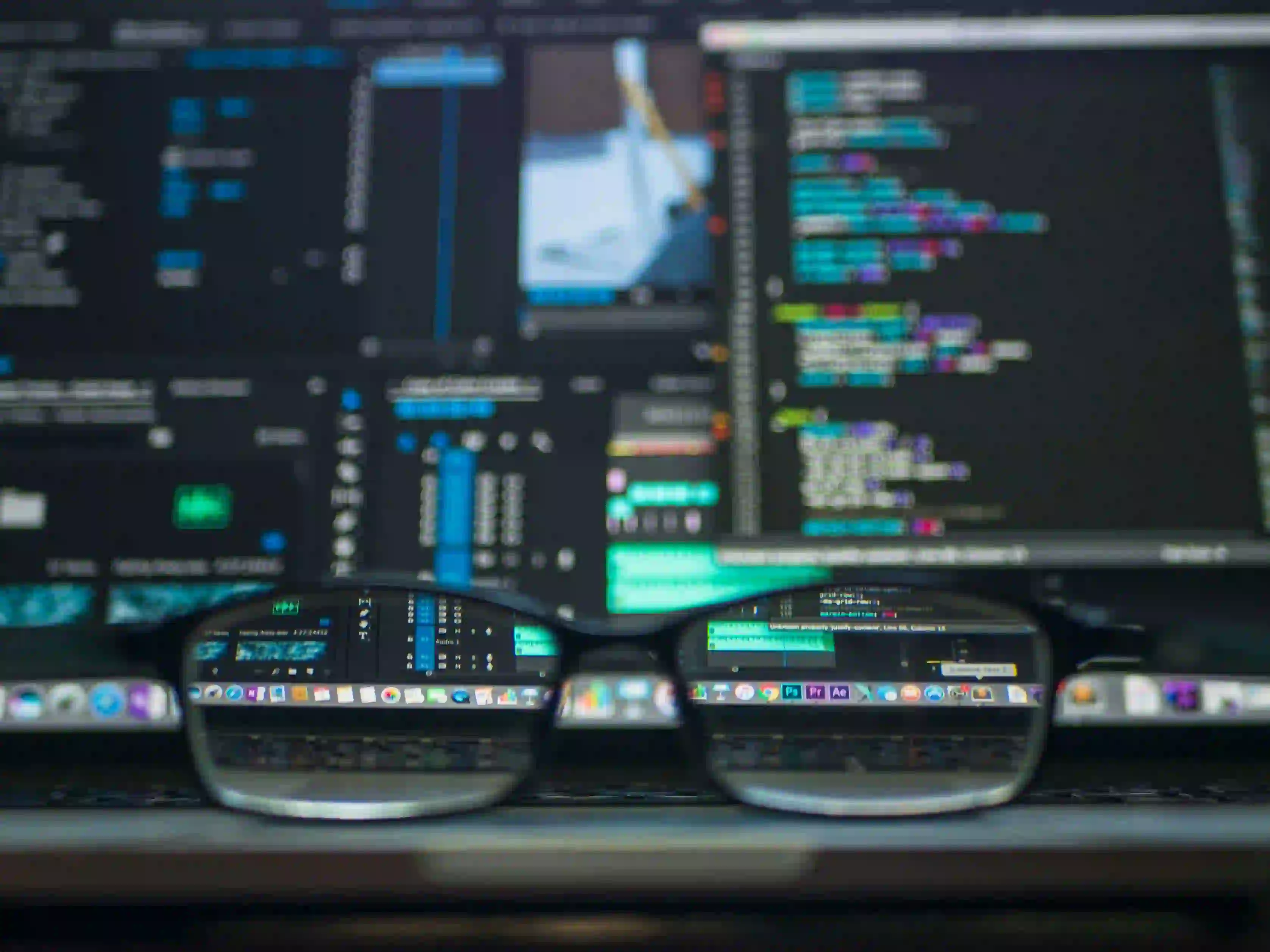
Why Inversion of Control Can Confuse New Developers
In the world of software development, particularly in Java, Inversion of Control (IoC) is a fundamental principle that enables developers to build more flexible and testable applications. Yet, while it's a powerful concept, it can often leave new developers scratching their heads. This blog post will delve into what IoC is, why it's important, and how to use it effectively.
What is Inversion of Control?
Inversion of Control refers to a design principle in which the control of object creation and management is inverted away from the application code, and instead is handled by a container or framework. In simpler terms, instead of writing code that dictates how components interact, you can rely on an external framework to do that for you.
How Does IoC Work?
To understand IoC better, let’s take a step back and differentiate between traditional control flow and the IoC approach.
In a traditional programming approach, a class might create its dependencies directly. For instance:
class UserService {
private final UserRepository userRepository;
public UserService() {
this.userRepository = new UserRepository(); // direct instantiation
}
}
In this example, UserService
is tightly coupled with UserRepository
. If you later need to change UserRepository
, you'd need to modify UserService
.
With IoC, dependencies are injected from an external source, usually a container like Spring:
class UserService {
private final UserRepository userRepository;
@Autowired // Using Spring's dependency injection
public UserService(UserRepository userRepository) {
this.userRepository = userRepository; // injected from Spring
}
}
This pattern increases flexibility, testability, and maintainability. Here’s why new developers often find IoC confusing:
1. New Concepts
IoC introduces several new concepts that new developers may not be familiar with, such as:
- Dependency Injection (DI): The process of providing an object with its dependencies rather than the object creating them itself.
- Service Locators: A registry that provides services to clients on demand.
These concepts can be overwhelming. When you're accustomed to a straightforward approach, switching to IoC can feel like learning a new language.
2. Ambiguity in Control Flow
In traditional programming, the flow of control is easy to follow. However, with IoC, control is transferred to the container.
For example, consider a Spring application:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In this snippet, Spring manages your application context. A new developer may ask: Where does execution start? Who creates the beans? This can lead to confusion if they aren't familiar with the framework's workings.
3. Complicated Debugging
Debugging IoC-based applications can often feel like looking for a needle in a haystack.
When you have complex object graphs and dependencies, understanding where an error occurs in the flow can be challenging. A stack trace may contain references to multiple layers of abstraction, masking the root cause.
Here’s a common scenario using Spring:
@Service
public class UserService {
// omitted for brevity
}
@Controller
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService; // injected UserService
}
}
If an error occurs while interacting with UserService
, it might surface in UserController
, leaving developers questioning the source.
4. Configuration Overhead
Configuring IoC containers (like Spring) can be tedious. Annotations, XML files, or Java-based configuration can feel redundant, especially for simple projects.
Consider a simple application.properties
for a Spring Boot project:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=secret
Quick Note
For detailed Spring Boot configuration, refer to the official Spring Documentation.
Managing this configuration increases complexity for a new developer who may prefer straightforward code.
5. Overhead of Learning Frameworks
To effectively implement IoC, developers are often required to learn specific frameworks.
Frameworks like Spring have extensive documentation, and new developers can feel lost as they navigate through various components like Spring MVC, Spring Data, and how they integrate with IoC. There's a learning curve associated with understanding how these frameworks use IoC.
Making IoC More Accessible
Although IoC can pose challenges for new developers, there are ways to make it more accessible:
1. Focus on Core Principles
Ensure you grasp the underlying concepts behind IoC and DI. Understanding these principles will enable you to see the practical benefits they offer in terms of flexibility and maintenance.
2. Start Small
Begin by working on small projects that utilize IoC. Experiment with manually injecting dependencies before moving on to a larger framework like Spring.
3. Leverage Community Resources
The Java community is vast. Read blogs, watch tutorials, and participate in forums like Stack Overflow for questions and clarifications.
4. Don’t Skip the Documentation
Framework documentation may feel dense, but it provides invaluable insights. Familiarize yourself with the fundamentals in the Spring Framework Documentation.
5. Practice Debugging
Use debugging tools within your IDE (like IntelliJ IDEA or Eclipse) to step through your code. This practice helps demystify what’s happening behind the scenes.
The Closing Argument
Inversion of Control is a powerful design principle that can tremendously improve the flexibility and maintainability of your Java applications. However, it's essential to approach it with an understanding of the potential challenges new developers face. With practice, learning, and exploration, you can harness IoC's true power and significantly enhance the quality of the software you create.
By acknowledging the confusion IoC can bring, new developers can take the necessary steps to overcome it and become more confident in their programming skills. Remember, every complex concept is simply a combination of simpler ideas that can become clear with the right practices and mindset. Happy coding!