Why Java's String Joining Can Be Confusing
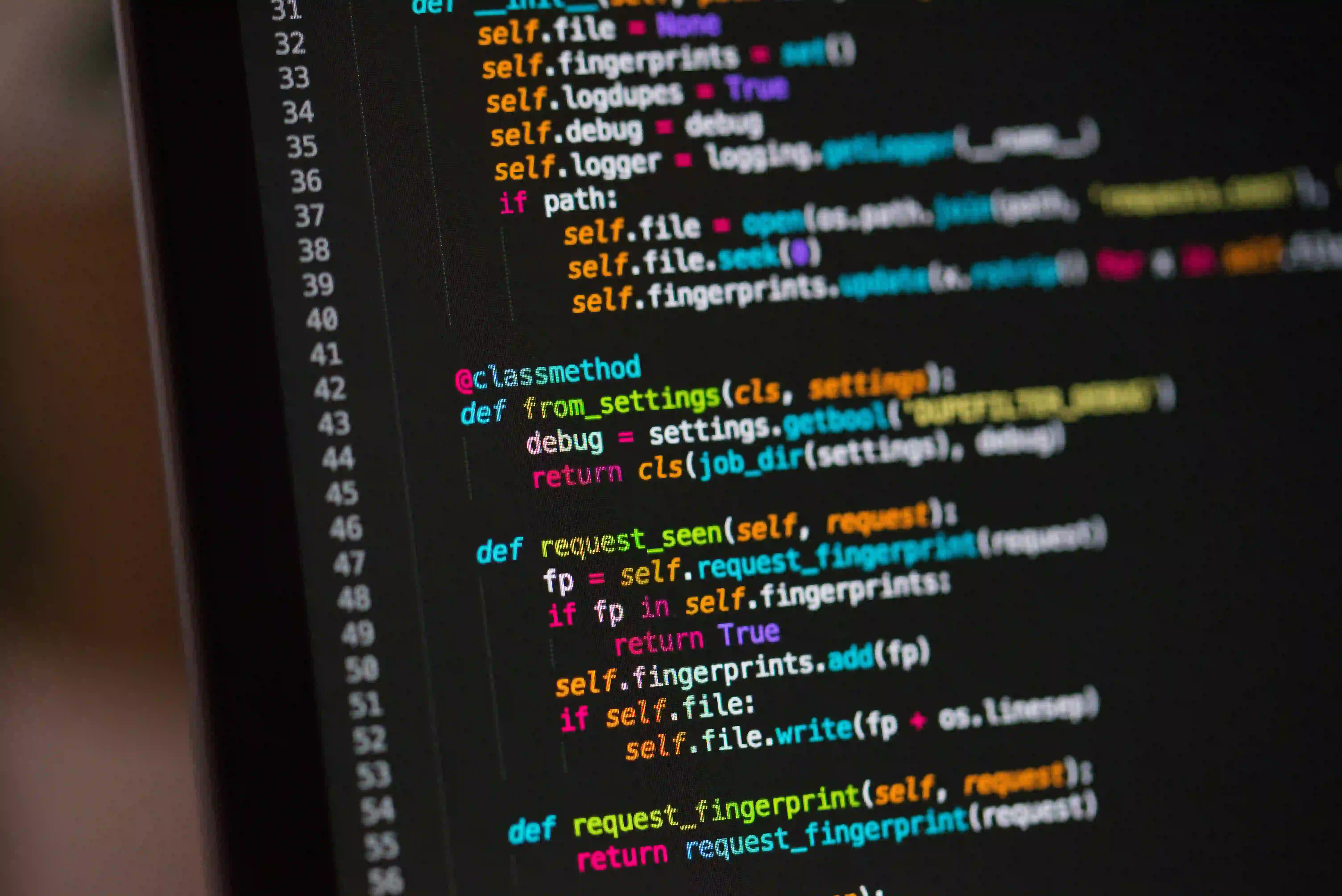
Why Java's String Joining Can Be Confusing
Java has long been a foundational programming language for developers around the world. One of its lesser-discussed yet critical features is the various ways it allows developers to join Strings. However, as straightforward as it sounds, the intricacies of string joining in Java can leave many perplexed. In this post, we'll delve into Java's string joining mechanisms, discussing the various methodologies, their nuances, and why they tend to confuse developers.
Understanding String Joining
String joining is the process of concatenating multiple strings into a single string. In Java, there are multiple ways of doing this, including using the +
operator, StringBuilder
, StringBuffer
, and the more recent String.join()
method introduced in Java 8.
1. Using the Plus Operator (+)
The simplest form of string joining is using the +
operator. Consider the following code snippet:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
This method is straightforward but can lead to performance issues when concatenating many strings, especially in loops. This is because each use of the +
operator creates a new string object. Strings in Java are immutable, meaning they cannot be changed once created. Thus, a new instance is created each time you use the +
operator.
Why It's Confusing
The +
operator appears simple and intuitive for joining strings, but it can become inefficient almost immediately as the number of concatenations increases. A loop concatenating strings can cause excessive memory usage and slow performance, leading many to seek alternatives.
2. Using StringBuilder
For cases where performance is key, Java provides the StringBuilder
class. This mutable sequence of characters allows for more efficient string concatenation. Here's how you use it:
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("John");
stringBuilder.append(" ");
stringBuilder.append("Doe");
String fullName = stringBuilder.toString();
Why It's Efficient
By using StringBuilder
, we avoid creating multiple intermediate string objects. The StringBuilder
class maintains a buffer, allowing you to manipulate the contents without creating new instances each time.
Why It's Confusing
The confusion arises from needing to remember to use toString()
to convert the StringBuilder
back to a standard string. Additionally, beginners might find the use of the append
method less intuitive compared to the +
operator.
3. Using String.format()
Another method for joining strings in Java is using String.format()
. This method allows developers to format strings in a structured manner.
String firstName = "John";
String lastName = "Doe";
String fullName = String.format("%s %s", firstName, lastName);
Why It's Powerful
String.format()
is incredibly useful for creating more complex strings. You can easily specify formats for different data types. It draws on C-style formatting, which many developers may already be familiar with.
Why It's Confusing
For those unfamiliar with the formatting syntax, String.format()
can appear overly complex. The format specifiers (like %s
) may not be immediately understandable.
4. Using String.join() (Java 8 and onwards)
As per Java’s official documentation, the String.join()
method is another powerful way to concatenate strings. This method takes a delimiter and an iterable of strings.
List<String> names = Arrays.asList("John", "Doe");
String fullName = String.join(" ", names);
Why It's Modern
With a more modern and cleaner syntax, String.join()
simplifies array or list joining. It’s concise and efficient, making the code easier to read.
Why It's Confusing
The introduction of a new method can add a layer of complexity, particularly for developers unfamiliar with Java 8 features. There are also nuances regarding null handling that can be problematic if not understood.
When to Choose Which Method
Choosing the proper string joining method often depends on the context:
- For single or few concatenations: Use the
+
operator. - For many concatenations (especially in loops): Use
StringBuilder
for better performance. - For formatted strings: Use
String.format()
, especially when dealing with complex data types. - For joining collections: Use
String.join()
, which is clean and efficient.
In Conclusion, Here is What Matters
While Java offers multiple ways to join strings, each method has its use cases, benefits, and pitfalls. Understanding them not only improves performance but also leads to cleaner, more maintainable code.
String joining can be confusing at times due to differences in performance, readability, and method signatures. However, once you grasp the strengths and weaknesses of each approach, you'll be able to select the most appropriate method quickly.
If you're interested in diving deeper into Java, check out other resources such as Oracle's Java Tutorials for more comprehensive information on Java programming practices.
Happy coding!